Overcoming JavaFX Performance Issues in Real-World Apps
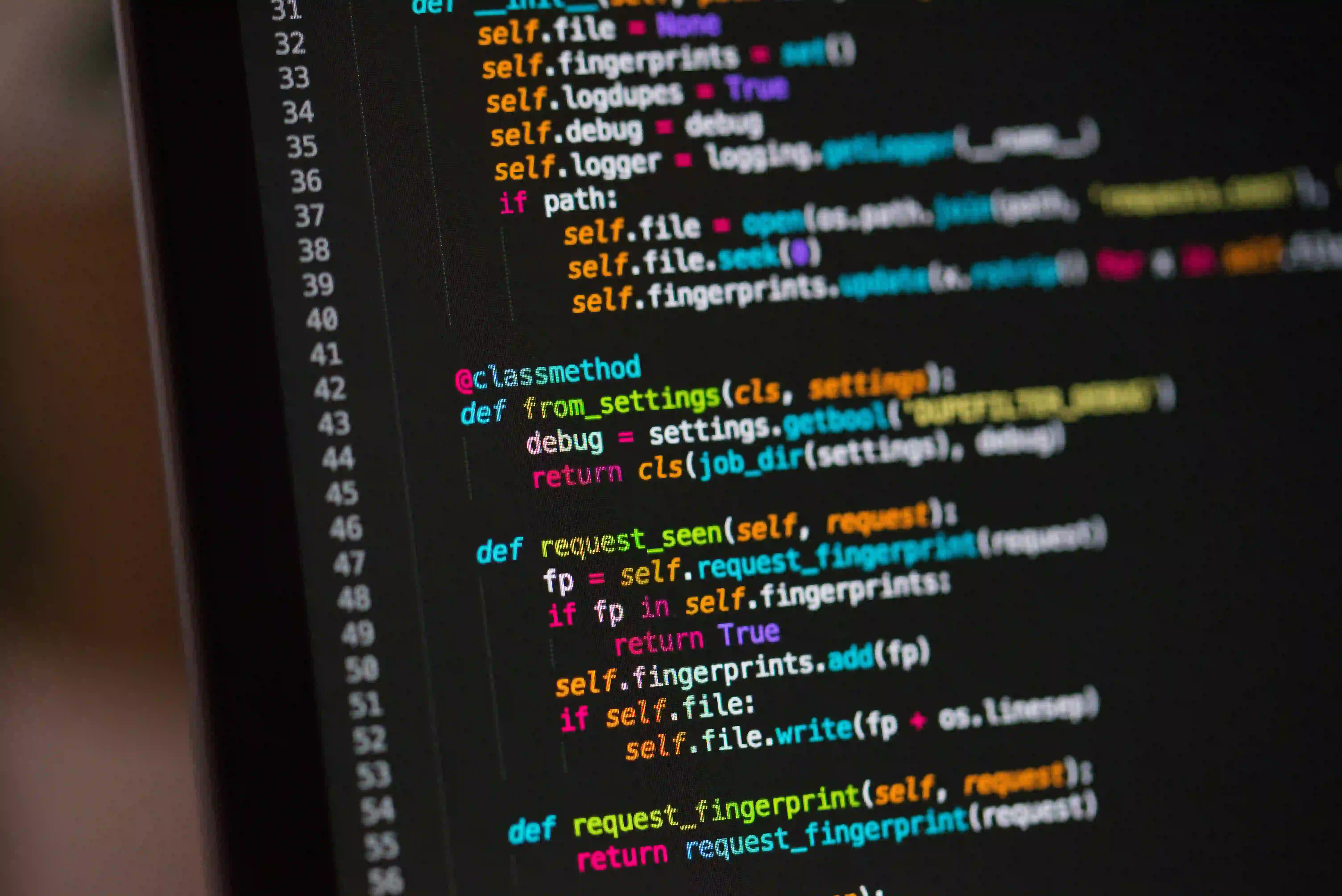
Overcoming JavaFX Performance Issues in Real-World Apps
JavaFX is a powerful framework for building rich client applications in Java. While it provides a modern way to design user interfaces and offers robust support for graphics, video, and audio, developers sometimes face performance issues when deploying JavaFX applications in real-world scenarios. In this blog post, we will delve into the common performance problems that can arise in JavaFX applications and explore practical solutions.
Understanding JavaFX Performance Issues
JavaFX applications can experience slow performance due to various factors, including:
- Heavy UI Thread Usage: The JavaFX framework relies on a single Application Thread for rendering graphics. Tasks that take too long on this thread can lead to UI freezes.
- Inefficient Scene Graph: The JavaFX scene graph is a tree structure that represents all visual elements. A complex scene graph can significantly slow down rendering.
- Resource Management: Poor resource management, such as large images or a large number of nodes in the scene graph, can lead to slow performance and increased memory consumption.
- Animation and Rendering: Improper use of animations or transitions can cause janky effects and frame drops.
Now, let’s explore some strategies to overcome these performance challenges in JavaFX applications.
1. Optimize Background Tasks
Performing long-running tasks on the JavaFX Application Thread will cause the user interface to become unresponsive. Use the Task
class in JavaFX to run these operations in the background.
Example: Using Task for Background Operations
import javafx.concurrent.Task;
public class DataFetchTask extends Task<List<String>> {
@Override
protected List<String> call() throws Exception {
List<String> data = new ArrayList<>();
// Simulate a long-running operation
for (int i = 0; i < 1000; i++) {
// This represents a time-consuming task
data.add("Data " + i);
updateProgress(i + 1, 1000);
}
return data;
}
}
In the example above, the Task
class is used to allow the application to remain responsive while the data is being fetched. The updateProgress
method can be called to inform the UI about the task’s progress.
Why This Matters
Running long tasks in the background prevents the UI from freezing, ensuring a seamless user experience. Leveraging JavaFX’s Task
system ensures that users can interact with the application without interruption.
2. Optimize Scene Graph
A complex scene graph can slow down rendering. Here are a few techniques to optimize it:
- Minimize Node Usage: Use fewer nodes by combining UI elements where possible.
- Use Groups: If you have multiple similar nodes, consider grouping them. Grouping reduces the complexity of your scene graph.
- Lazy Loading: Load only the portions of the UI needed at the moment (e.g., in a tabbed interface).
Example: Grouping Nodes
import javafx.scene.Group;
import javafx.scene.shape.Circle;
Group root = new Group();
for (int i = 0; i < 100; i++) {
Circle circle = new Circle(10, Color.BLUE);
circle.setTranslateX(i * 20);
root.getChildren().add(circle);
}
In this code snippet, we create a group of circles instead of managing each circle individually, which reduces the overall complexity and memory footprint of the UI.
Why This Matters
Optimizing the scene graph reduces the workload on the rendering engine, leading to a more responsive UI and smoother performance.
3. Efficient Resource Management
Resources like images, videos, and icons can consume significant memory. Manage them wisely:
- Use Scaled Images: Load images in the resolution they will be displayed at, using the appropriate scaling method.
- Use ImageView Efficiently: Instead of creating numerous ImageView instances, reuse existing ones.
Example: Efficient Image Loading
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
ImageView imageView = new ImageView();
Image image = new Image("imagePath.png", 100, 100, false, true); // Load at display size
imageView.setImage(image);
In this example, we load the image at the size it will be displayed, which conserves memory and improves loading time.
Why This Matters
Efficient resource management can significantly reduce memory overhead, leading to quicker load times and better overall performance.
4. Optimize Animations and Effects
Animations and visual effects can enrich user experience, but poorly implemented animations can hurt performance.
- Use Timeline or Animation: Use JavaFX's built-in animation capabilities instead of creating custom render loops.
- Limit Node Effects: Use effects like shadows and lighting only when necessary, as these can be costly in terms of performance.
Example: Using Timeline for Animation
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.util.Duration;
Timeline timeline = new Timeline(new KeyFrame(Duration.seconds(1), actionEvent -> {
// Animation logic here
}));
timeline.setCycleCount(Timeline.INDEFINITE);
timeline.play();
This example shows how to implement a simple animation using the Timeline
class. This class is optimized for performance, in contrast to manual frame rendering.
Why This Matters
By utilizing JavaFX’s optimized animations, you ensure smoother performance, reducing frame drops and janky effects.
5. Use CSS for Styling
JavaFX supports CSS for styling user interfaces. Using CSS can offload some processing from the JavaFX Application Thread, leading to better performance.
Example: Styling with CSS
.button {
-fx-font-size: 14pt;
-fx-background-color: #4CAF50;
-fx-text-fill: white;
}
By defining styles in an external CSS file, you can minimize inline styling and improve performance.
Why This Matters
CSS provides a clear separation of styling and logic, enabling cleaner code and potentially reducing the strain on the JavaFX Application Thread.
Lessons Learned
Optimizing JavaFX applications for performance requires a multi-faceted approach. By efficiently managing background tasks, optimizing the scene graph, handling resources wisely, and utilizing JavaFX's built-in capabilities, you can create smooth, responsive applications.
If you're just starting with JavaFX or looking to fine-tune your existing applications, remember that small optimizations can lead to significant performance improvements. For further reading on JavaFX performance optimization techniques, consider visiting the Oracle JavaFX Documentation and the JavaFX GitHub repository.
By embracing these strategies, you'll ensure that your JavaFX applications not only look good but also perform efficiently, providing users with the best experience possible.