Overcoming Challenges in Java for DOCX Document Comparison
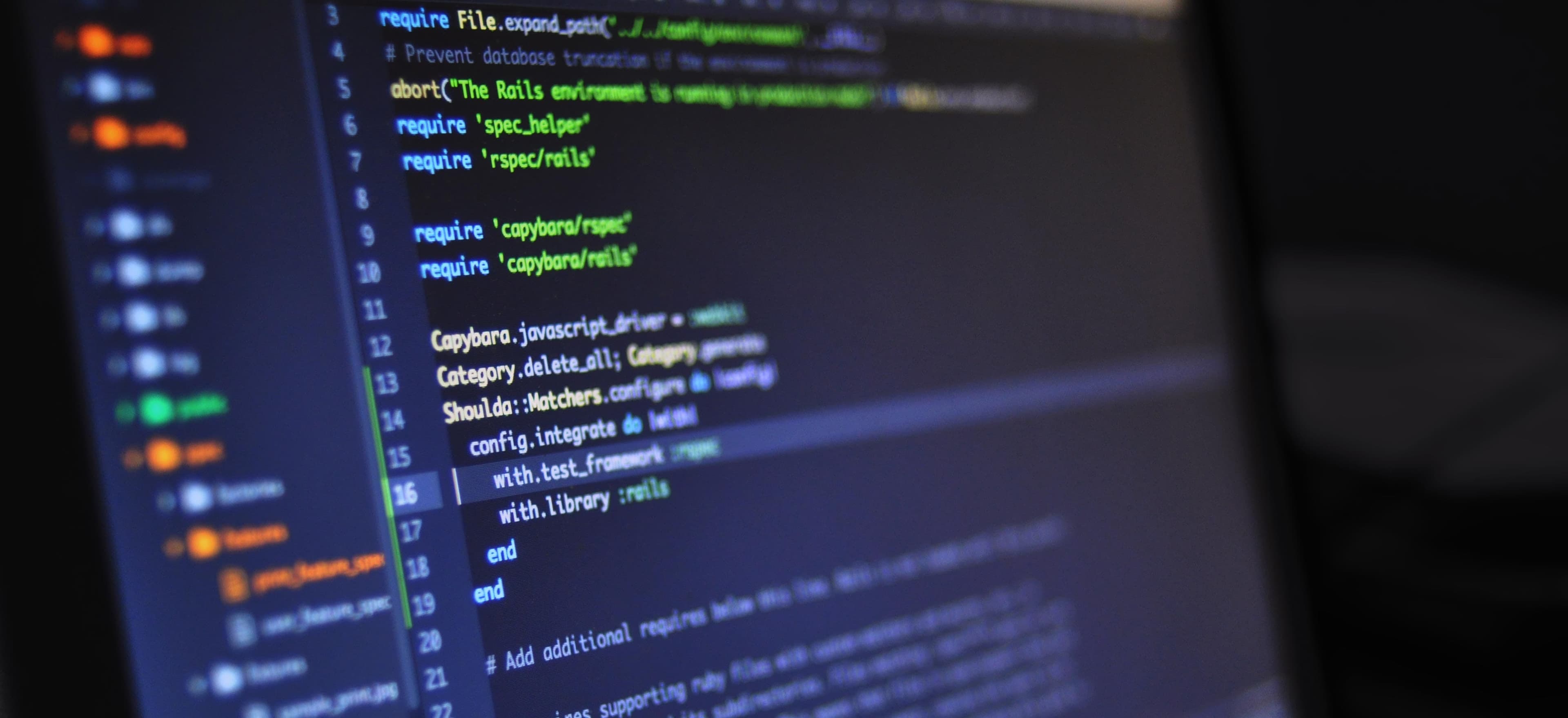
- Published on
Overcoming Challenges in Java for DOCX Document Comparison
In today's fast-evolving tech landscape, document management and comparison are pivotal aspects for businesses and software developers alike. As more organizations rely on digital communication and documentation, the need for effective tools that can compare, highlight differences, and synchronize changes becomes paramount. Java provides a robust platform for creating such tools. However, developers often encounter challenges in implementing efficient DOCX document comparison functionalities.
In this blog post, we will highlight the common challenges faced in Java for DOCX document comparison, present solutions, and provide exemplary code snippets. By the end, you'll have a clearer understanding of how to tackle these challenges effectively.
Understanding DOCX File Structure
Before delving into comparisons, it’s essential to grasp what a DOCX file is and its underlying structure. DOCX files are essentially zip archives containing various XML files representing document data, styles, media, relationships, and more.
document.xml
: Contains the main content of the document.styles.xml
: Holds the styles used throughout the document.settings.xml
: Contains document settings.
Understanding this structure is fundamental because it might dictate your comparison strategy. For more information on DOCX structures, you can refer to the Microsoft Documentation.
Challenge #1: Accessing the DOCX File
Solution: Using Apache POI
The first hurdle involves accessing and reading DOCX files programmatically. A popular and efficient library to handle DOCX files in Java is Apache POI. It provides an easy way to manipulate both .xls
(Excel) and .docx
(Word) files.
To start, you need to include the Maven dependency in your project:
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.3</version>
</dependency>
Here’s how you can read the content of a DOCX file:
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.List;
public class DocxReader {
public static void main(String[] args) {
try (FileInputStream fis = new FileInputStream("document.docx");
XWPFDocument document = new XWPFDocument(fis)) {
List<XWPFParagraph> paragraphs = document.getParagraphs();
for (XWPFParagraph paragraph : paragraphs) {
System.out.println(paragraph.getText());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Use Apache POI?
Apache POI streamlines the process of working with Microsoft file formats. It abstracts away much of the complexity of parsing the DOCX structure, allowing developers to focus on the logic behind comparison rather than file parsing intricacies.
Challenge #2: Comparing Text Content
Once you have the content of both DOCX files, the next challenge lies in accurately comparing the text. It’s easy to compare strings; however, DOCX documents often contain various formatting styles and embedded objects that complicate direct comparison.
Solution: Implementing a Text Comparison Algorithm
One effective way to perform text comparison is to use the Levenshtein distance algorithm, which calculates the difference between two sequences. This allows us to identify additions, deletions, and substitutions between documents.
Here’s a simplistic implementation:
public class StringComparer {
public static int levenshteinDistance(String a, String b) {
int[][] dp = new int[a.length() + 1][b.length() + 1];
for (int i = 0; i <= a.length(); i++) {
for (int j = 0; j <= b.length(); j++) {
if (i == 0) {
dp[i][j] = j; // Deletions
} else if (j == 0) {
dp[i][j] = i; // Additions
} else if (a.charAt(i - 1) == b.charAt(j - 1)) {
dp[i][j] = dp[i - 1][j - 1]; // Match
} else {
dp[i][j] = 1 + Math.min(Math.min(dp[i - 1][j], dp[i][j - 1]), dp[i - 1][j - 1]); // Min of delete, add, replace
}
}
}
return dp[a.length()][b.length()];
}
public static void main(String[] args) {
String text1 = "This is an example document.";
String text2 = "This is a sample document.";
int distance = levenshteinDistance(text1, text2);
System.out.println("Levenshtein Distance: " + distance);
}
}
Why Use Levenshtein Distance?
Using the Levenshtein distance allows you to quantify how similar or different two strings are. This can be particularly useful in distinguishing minor changes in a DOCX document.
Challenge #3: Handling Formatting and Embedded Objects
Text comparison is only one piece of the puzzle. DOCX files often contain formatted text, images, tables, charts, and other elements that require special handling. Ignoring these can lead to incomplete comparisons.
Solution: Comprehensive Document Parsing
A robust comparison system needs to account for more than plain text. You can extract and normalize various elements using Apache POI. Here’s a basic approach to include formatting and links:
import org.apache.poi.xwpf.usermodel.XWPFRun;
import java.util.List;
public class DocxFormatter {
public void printFormattedText(XWPFParagraph paragraph) {
for (XWPFRun run : paragraph.getRuns()) {
String text = run.getText(0);
if (text != null) {
// Print with basic formatting
if (run.isBold()) {
System.out.print("**" + text + "** ");
} else {
System.out.print(text + " ");
}
}
}
System.out.println();
}
}
Why Account for Formatting?
Formatting details often convey significant information. For example, bold text might indicate headings or important notes that shouldn’t be omitted during comparison.
Challenge #4: Performance
When dealing with large documents, performance can become an issue. Comparing large volumes of text and elements can lead to slow execution times, particularly when using naive algorithms.
Solution: Memory Management
To enhance performance, consider applying optimization techniques like lazy loading and batch processing. Process sections of the document iteratively instead of loading everything into memory at once. Here’s an example of lazy loading:
public class OptimizedDocxReader {
public void readAndProcess(String filePath) {
try (FileInputStream fis = new FileInputStream(filePath);
XWPFDocument document = new XWPFDocument(fis)) {
// Process one paragraph at a time
for (XWPFParagraph paragraph : document.getParagraphs()) {
processParagraph(paragraph);
}
} catch (IOException e) {
e.printStackTrace();
}
}
private void processParagraph(XWPFParagraph paragraph) {
// Processing logic for individual paragraphs
System.out.println(paragraph.getText());
}
}
Why Optimize for Performance?
Performance optimizations help maintain a responsive user experience. Users expect quick comparisons, and large document processing can lead to frustration if managed inefficiently.
The Closing Argument
Document comparison in Java, especially for DOCX files, comes with a unique set of challenges. By employing libraries like Apache POI, implementing comparison algorithms, and optimizing your application's performance, you can effectively overcome these hurdles.
For further reading on document processing, explore resources like Apache POI’s Official Documentation and Open XML SDK Documentation.
Start implementing these solutions, and empower your applications with robust document comparison functionalities. Happy coding!