Troubleshooting Common Issues with mTLS in Java Calls
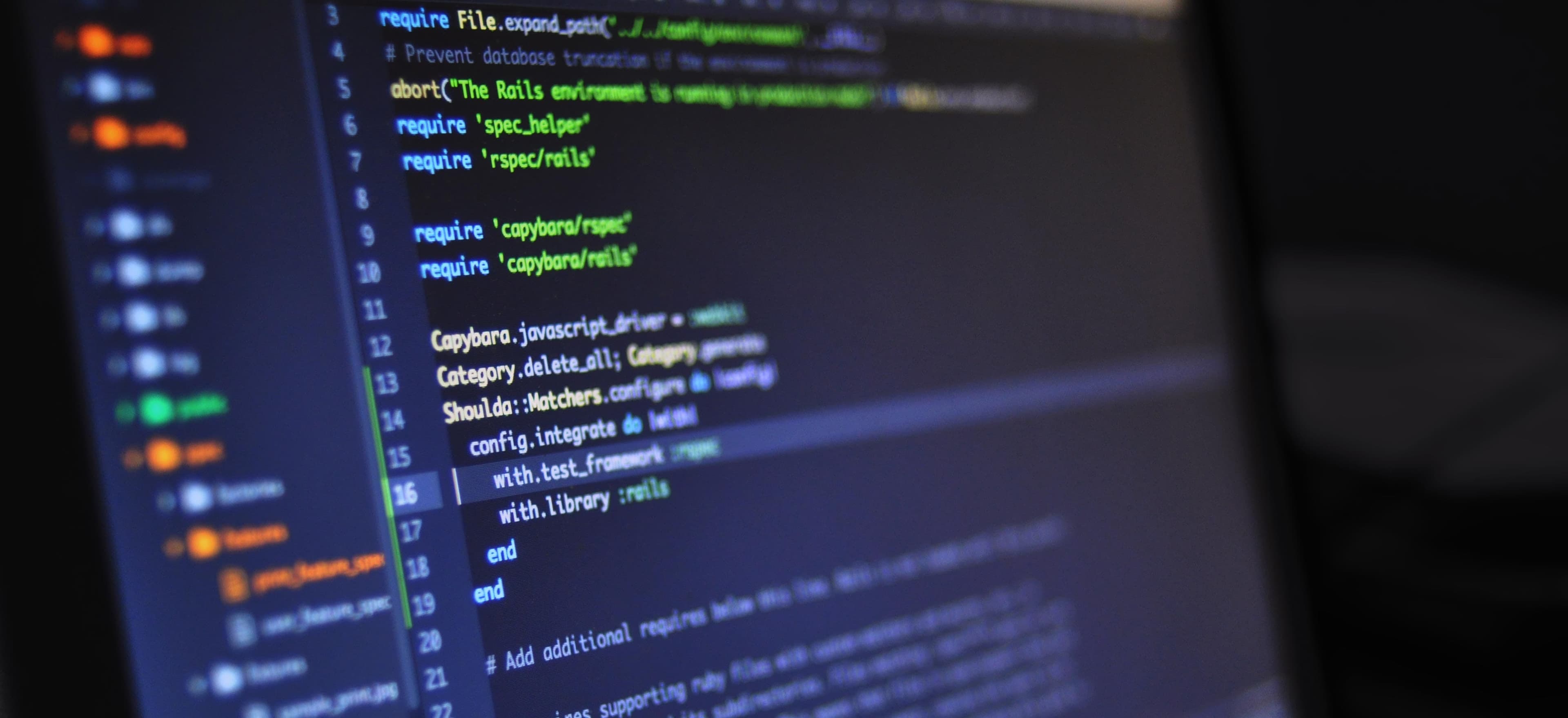
- Published on
Troubleshooting Common Issues with mTLS in Java Calls
Mutual Transport Layer Security (mTLS) is a powerful method for securing communications between clients and servers. It goes beyond standard TLS by requiring both parties to present certificates relative to one another, ensuring identity verification on both sides before establishing a connection. However, implementing mTLS in Java applications can introduce unique challenges.
In this blog post, we'll explore common issues faced when implementing mTLS in Java and provide practical solutions. We'll also include relevant code snippets to help clarify key concepts.
Table of Contents
- Understanding mTLS
- Setting up mTLS in Java
- Common Issues with mTLS
- Certificate Issues
- Protocol Mismatches
- Configuration Errors
- Debugging Tips
- Conclusion
1. Understanding mTLS
Before diving into troubleshooting, it's essential to grasp the fundamentals of mTLS. In standard TLS, the server presents a certificate to the client. In mTLS, both the client and the server provide certificates, creating a two-way verification process. This is crucial for applications that require high security, such as banking or healthcare systems.
How mTLS Works
- Client Initiates Connection: The process begins with the client initiating a connection to the server.
- Server Responds with Certificate: The server sends its digital certificate to the client.
- Client Validates Server Certificate: The client verifies the server's certificate against trusted Certificate Authorities (CAs).
- Client Sends Its Certificate: Once the server's identity is confirmed, the client sends its certificate back to the server.
- Server Validates Client Certificate: The server validates the client's certificate.
- Encrypted Communication Begins: Upon successful verification, they establish a secure connection.
2. Setting up mTLS in Java
To set up mTLS in a Java application, you typically use libraries like Spring or Apache HttpClient. Below is a basic setup using HttpClient
.
Maven Dependencies
First, ensure you have the necessary dependencies in your pom.xml
:
<dependencies>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
</dependencies>
Example Code Snippet
Here's a simplified example of how you could implement mTLS with Apache HttpClient:
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.ssl.SSLContextBuilder;
import java.io.File;
public class MTLSClient {
public static void main(String[] args) {
try {
// Load the client certificate and the trusted CA
CloseableHttpClient httpClient = HttpClients.custom()
.setSSLContext(SSLContextBuilder.create()
.loadTrustMaterial(new File("path/to/truststore.jks"), "password".toCharArray())
.loadKeyMaterial(new File("path/to/keystore.jks"), "password".toCharArray(), "password".toCharArray())
.build())
.build();
HttpGet request = new HttpGet("https://your-secure-server.com/api");
CloseableHttpResponse response = httpClient.execute(request);
// Handle response
System.out.println("Response Code: " + response.getStatusLine().getStatusCode());
response.close();
httpClient.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why Use These Settings?
Using SSLContextBuilder
allows you to configure the SSL context with specific key materials and trust stores. It’s crucial because:
- Security: It ensures that only trusted certificates are accepted, which protects against Man-in-the-Middle attacks.
- Flexibility: You can specify different stores for keys and trusted certificates as per your application requirements.
3. Common Issues with mTLS
Certificate Issues
Problem
One of the most common issues arises from misconfigured or invalid certificates. For instance, if the certificate has expired, it will fail verification.
Solution
Check the following:
- Ensure the certificates are not expired.
- Confirm that the Common Name (CN) in the certificate matches the host name of the server.
- Verify the certificate chain to ensure all intermediate and root CAs are trusted by the application.
Protocol Mismatches
Problem
Sometimes, the client and server might attempt to use different protocols or cipher suites, leading to handshake failures.
Solution
Enable logging of the SSL handshake by setting the following system property when launching your Java application:
-Djavax.net.debug=ssl,handshake
Examine the logs for any protocol mismatches and make sure that both the server and client support common configurations.
Configuration Errors
Problem
Misconfigurations in your keystore or truststore can often lead to SSLHandshakeException
.
Solution
Double-check your configurations:
- Ensure the paths to the keystore and truststore are correct.
- Verify that you are using the correct passwords and file formats (typically JKS or PKCS12).
- Use the
keytool
command to list entries in your keystore to ensure the correct certificates are present.
keytool -list -v -keystore path/to/keystore.jks
4. Debugging Tips
When troubleshooting mTLS issues, consider these best practices:
Check the Full Stack Trace
When you run into exceptions, check the full stack trace. It often provides valuable context on what went wrong.
Use SSL Debugging
As mentioned, enabling SSL debugging can provide insights into handshake failures and certificate issues. Review these logs carefully.
Simplify with Localhost
If possible, simplify initial tests by using localhost. This way, you can isolate the problem from network issues.
Utilize Tools
Utilize tools like Postman or cURL with mTLS support to test API endpoints. This can help verify whether the server denies or accepts connections due to mTLS.
curl --key path/to/client.key --cert path/to/client.crt --cacert path/to/ca.crt https://your-secure-server.com/api
5. Conclusion
Implementing mTLS in Java not only enhances security but also ensures a trusted communication channel between clients and servers. However, as we've discussed, it comes with its fair share of challenges.
Be proactive in managing certificates, meticulously configure your SSL context, and adhere to best practices for debugging any issues that arise.
For further reading, check out the official documentation on the Java security architecture and Apache HttpClient for more information.
By keeping these strategies in mind, you can effectively troubleshoot and overcome common mTLS issues, paving the way for a more secure application environment. Happy coding!