Complexity in Java: Tackling Decorator Pattern Challenges
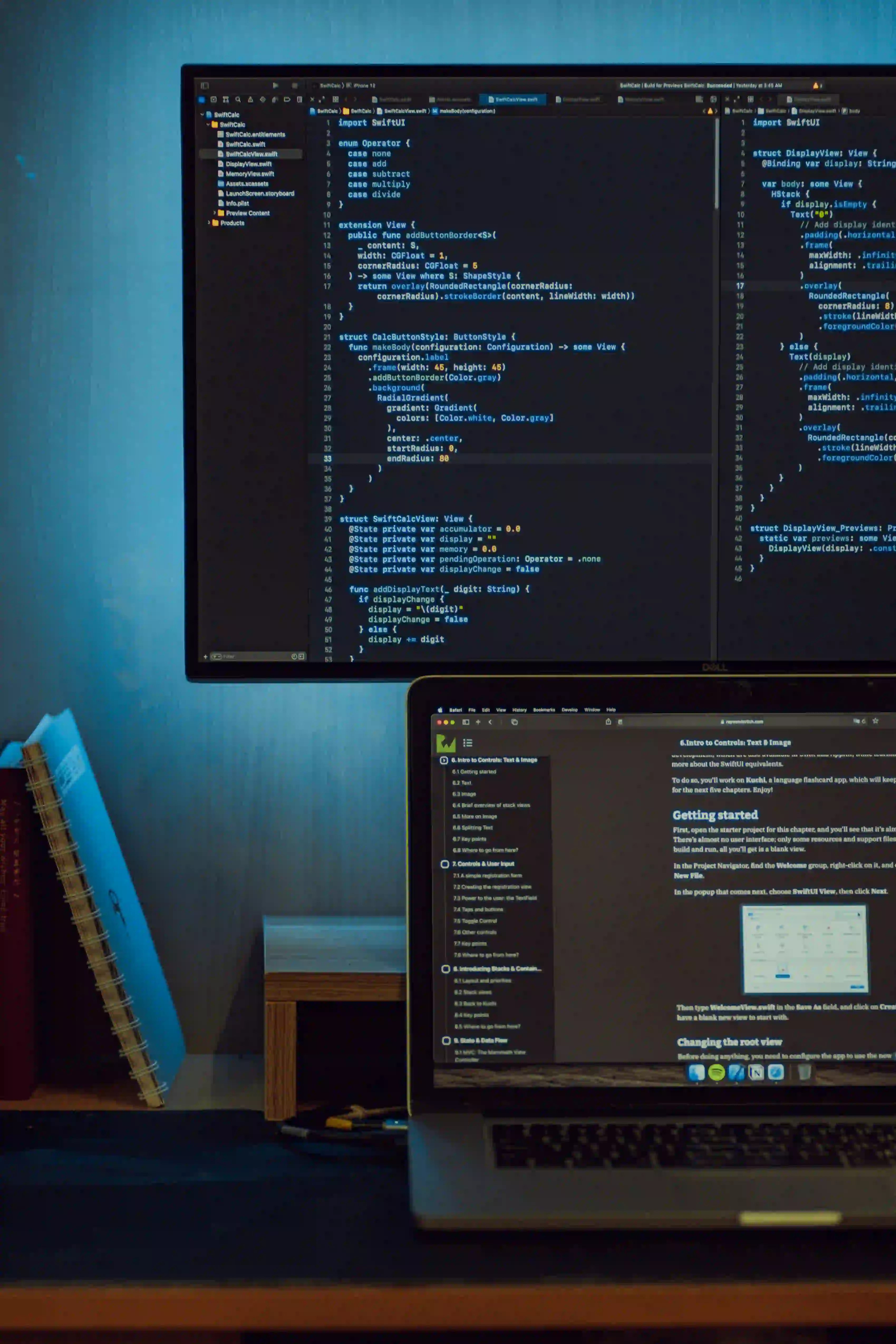
Complexity in Java: Tackling Decorator Pattern Challenges
In software development, patterns are crucial for solving common problems. One such pattern—the Decorator Pattern—offers a flexible way to extend the functionality of objects at runtime. In this blog post, we will delve deeply into the complexities of the Decorator Pattern in Java. We will explore real-life scenarios, code examples, and the benefits of employing this design pattern. By the end of this post, you will have a solid understanding of how to effectively utilize the Decorator Pattern in your Java applications.
What is the Decorator Pattern?
The Decorator Pattern falls under the category of structural design patterns, which are concerned with how classes and objects compose to form larger structures. This pattern enables additional responsibilities to be added to individual objects dynamically without altering their structure.
Key Components
Here are the key components of the Decorator Pattern:
- Component: An interface or abstract class defining the common interface for both the concrete components and decorators.
- Concrete Component: A class that implements the component interface. This is the object to which additional responsibilities can be added.
- Decorator: A class that implements the component interface and has a reference to a component object. It wraps the component and extends its functionality.
Why Use the Decorator Pattern?
Using the Decorator Pattern provides several advantages:
- Single Responsibility Principle: You can adhere to this principle by separating the core functionality from additional responsibilities.
- Flexibility: You can add responsibilities dynamically at runtime when needed, which helps in avoiding class explosion.
- Open/Closed Principle: You can extend the behavior of objects without modifying their structure.
Implementing the Decorator Pattern in Java
Let’s illustrate the Decorator Pattern through a classic example involving a simple pizza ordering system. Our initial objective is to create a pizza class with various toppings that can be added dynamically.
Step 1: Define the Component Interface
First, let’s define our Pizza
component interface.
public interface Pizza {
String getDescription();
double cost();
}
Why? This acts as our blueprint that other pizza-related classes will follow, ensuring they all implement common methods like getDescription
and cost
.
Step 2: Create the Concrete Component
Now, we can create a class that implements the Pizza
interface:
public class BasicPizza implements Pizza {
@Override
public String getDescription() {
return "Basic Pizza";
}
@Override
public double cost() {
return 8.00; // Base price
}
}
Why? The BasicPizza
class is the core element of our system. It provides the essential structure we will build upon.
Step 3: Create the Decorator
Next, we create an abstract decorator class that implements the Pizza
interface.
public abstract class PizzaDecorator implements Pizza {
protected Pizza tempPizza;
public PizzaDecorator(Pizza newPizza) {
this.tempPizza = newPizza;
}
@Override
public String getDescription() {
return tempPizza.getDescription();
}
@Override
public double cost() {
return tempPizza.cost();
}
}
Why? The PizzaDecorator
class allows decorators to wrap other Pizza
objects (like BasicPizza
) while still adhering to the component interface.
Step 4: Create Concrete Decorators
Now, let’s create some concrete decorators for pizza toppings like cheese and olives.
public class CheeseDecorator extends PizzaDecorator {
public CheeseDecorator(Pizza newPizza) {
super(newPizza);
}
@Override
public String getDescription() {
return tempPizza.getDescription() + ", Cheese";
}
@Override
public double cost() {
return tempPizza.cost() + 1.50; // Additional cost for cheese
}
}
public class OliveDecorator extends PizzaDecorator {
public OliveDecorator(Pizza newPizza) {
super(newPizza);
}
@Override
public String getDescription() {
return tempPizza.getDescription() + ", Olives";
}
@Override
public double cost() {
return tempPizza.cost() + 1.00; // Additional cost for olives
}
}
Why? Each decorator class adds its flavor to the pizza while calling the methods of the wrapped object. This allows for continuous extension without altering the original BasicPizza
.
Step 5: Using the Decorators
Now, let's put all the pieces together:
public class PizzaStore {
public static void main(String[] args) {
Pizza myPizza = new BasicPizza(); // Create a basic pizza
System.out.println(myPizza.getDescription() + " $" + myPizza.cost());
myPizza = new CheeseDecorator(myPizza); // Add cheese
System.out.println(myPizza.getDescription() + " $" + myPizza.cost());
myPizza = new OliveDecorator(myPizza); // Add olives
System.out.println(myPizza.getDescription() + " $" + myPizza.cost());
}
}
Output:
Basic Pizza $8.0
Basic Pizza, Cheese $9.5
Basic Pizza, Cheese, Olives $10.5
Why? This demonstrates how you can add multiple layers of decorators to enrich the functionality of your core pizza object. Each addition stays compliant with the original interface, allowing for dynamic scaling according to your requirements.
Challenges of the Decorator Pattern
While the Decorator Pattern opens up a world of flexibility and scalability, it does come with its challenges:
- Increased Complexity: The more decorators you create, the higher the complexity of your system can climb. This may lead to issues in maintenance and readability of your code.
- Overhead: Additional layers can introduce performance overhead since each decorator will have its own instance, leading to increased memory usage.
To mitigate these challenges, it’s essential to apply the pattern judiciously. Use it when you truly need dynamic behavior and wrap necessary functionalities without necessity overextension.
My Closing Thoughts on the Matter
The Decorator Pattern is a valuable asset in the Java developer's toolkit. By allowing enhancements to be added dynamically and flexibly, it adheres to key software design principles while mitigating the risks associated with class bloat.
For a deeper exploration of design patterns, consider checking out Refactoring Guru's design patterns to augment your understanding further.
As with any pattern, a balanced approach is crucial. Always consider maintainability and readability against the flexibility you may gain. By grasping the benefits and limitations of the Decorator Pattern, you will craft better Java applications that are both elegant and effective.
This blog post aims to provide a comprehensive overview while being a resource for practical implementation, ensuring that you’re well-equipped to tackle the complexities of the Decorator Pattern in your Java projects.