Fixing the Pitfalls of Continuous Deployment: My Story
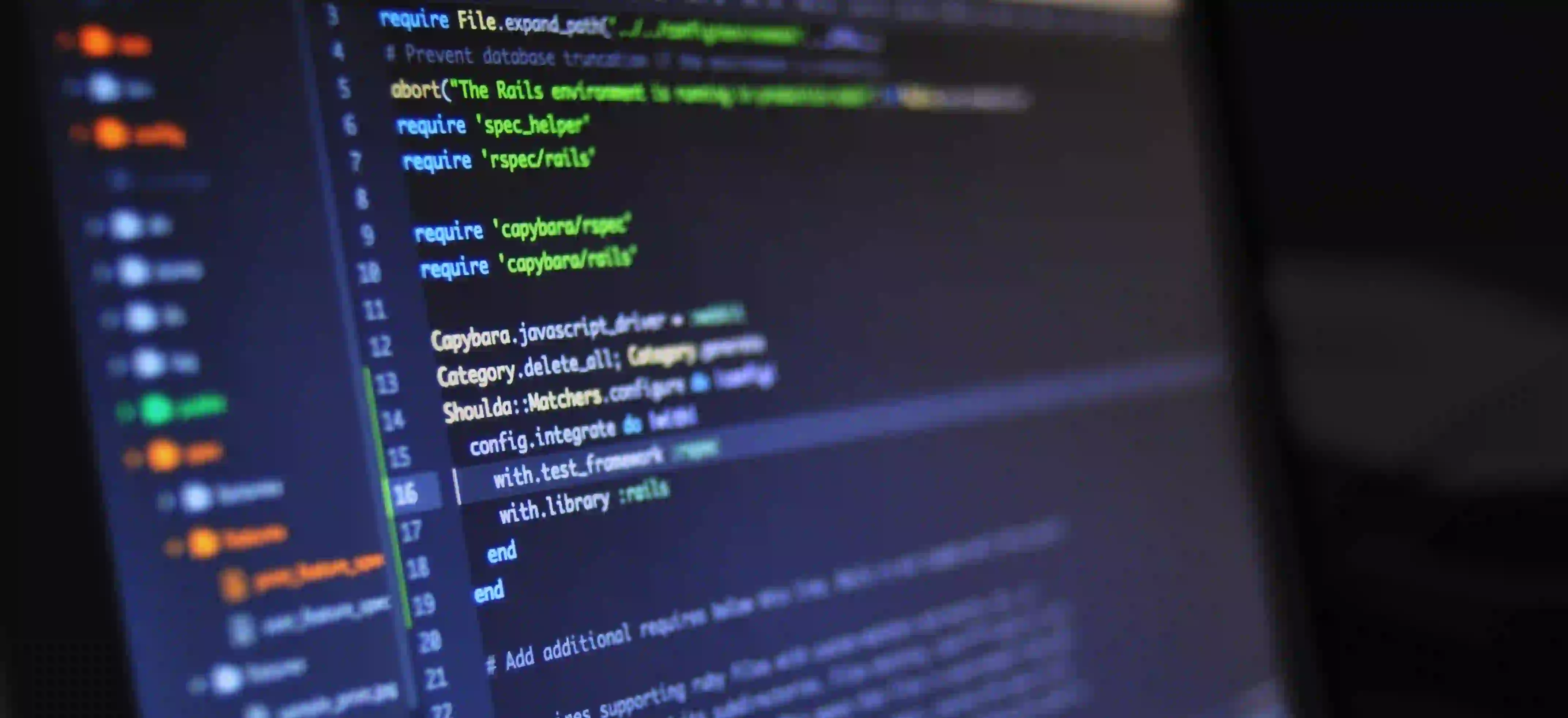
Fixing the Pitfalls of Continuous Deployment: My Story
Continuous Deployment (CD) has transformed how we deliver software. What once took teams weeks or even months now occurs in hours, thanks to automated systems and robust pipelines. However, with speed comes responsibility. Throughout my journey in the tech industry, I encountered several pitfalls of continuous deployment that almost derailed projects. In this blog post, I will share my experiences, the common problems I faced, and the solutions that helped navigate these challenges.
Understanding Continuous Deployment
Before diving into the pitfalls, let's clarify what continuous deployment is. Continuous Deployment is a software release process where every change made to the codebase is automatically deployed to production after passing predefined tests. This practices promotes rapid iteration and timely feature rollouts, ensuring that users always have access to the latest functionality.
Initial Array of Problems
When our team first implemented Continuous Deployment, the excitement was palpable. We envisioned rapid delivery to our users, eliminating the long cycles of traditional deployment. However, our enthusiasm quickly met reality, and several issues arose:
- Fragile Systems: Rapid deployments led to unstable systems.
- Unclear Communication: Teams began losing synchronization on release processes.
- Overwhelming Feedback: With quick releases, user feedback began pouring in, but actionable insights became difficult to discern.
- Testing Burden: Automated tests sometimes failed, but deploying to production was still prioritized.
Pitfall #1: Fragile Systems
One of the earliest issues we faced was system fragility. Deployments were happening so often that bugs could slip through the cracks unnoticed, causing instability.
My Solution: Enhanced Testing
To counteract this, we adopted a robust testing strategy using the following test types:
- Unit Tests: These tests cover individual components of the application to ensure their correctness.
- Integration Tests: These tests check how well different components work together.
- End-to-End Tests: These simulate user scenarios to validate the entire application flow.
Here’s a simple example of a unit test written in Java using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
void addTwoNumbers() {
Calculator calculator = new Calculator();
int result = calculator.add(5, 3);
assertEquals(8, result, "Adding 5 and 3 should equal 8");
}
}
Why This Works
Unit and integration tests allowed us to identify bugs early in the development cycle. By validating the integrity of each code change, we significantly improved our deployment stability.
Pitfall #2: Unclear Communication
With more frequent deployments came confusion. The team members were increasingly unaware of what changes were being released.
My Solution: Enhanced Communication Protocols
To tackle this communication breakdown, we implemented structured release notes alongside a Release Calendar that highlighted upcoming features, bug fixes, and version changes. We utilized tools like Slack and JIRA to facilitate ongoing conversations about deployments.
Slack Example:
*Release Note - Version 1.5.0*
*Date:* 2023-10-10
*New Features:*
- User profile customization
- Enhanced search capabilities
*Bug Fixes:*
- Resolved crashes in profile view
- Fixed pagination issues in user lists
Why It Matters
This level of transparency allowed developers and stakeholders to know what changes were being made and when they would go live. It minimized surprises and fostered a culture of accountability.
Pitfall #3: Overwhelming Feedback
User feedback is critical to product improvement, but when released too often, it can become overwhelming. We struggled to sift through various user comments to gather actionable insights.
My Solution: Prioritize Feedback
To overcome this hurdle, we introduced a user feedback assessment rubric that allowed us to categorize feedback based on urgency, feature requests, and overall theme. Using a structured approach, we would classify feedback as:
- Critical: Needs immediate attention.
- High Priority: Important but not urgent.
- Low Priority: Nice to have, but not essential.
Sample Feedback Categorization in Java:
enum FeedbackPriority {
CRITICAL,
HIGH,
LOW
}
class UserFeedback {
String userId;
String feedback;
FeedbackPriority priority;
public UserFeedback(String userId, String feedback, FeedbackPriority priority) {
this.userId = userId;
this.feedback = feedback;
this.priority = priority;
}
}
Why Organize Feedback
Prioritization helped our product team focus on what truly mattered. It prevented overload and ensured that the most critical bugs and features got attention first. As a result, we enhanced our overall development process.
Pitfall #4: The Testing Burden
Automated tests are supposed to reduce the burden on developers. However, we found that when tests failed, it often led to a frantic rush to fix issues instead of deploying valuable features.
My Solution: Implementing Test Flakiness Policies
To address this, we established a policy for identifying flaky tests. Here’s how it worked:
- Track Test Reliability: We kept track of test pass/fail rates using CI/CD pipeline tools.
- Create a ‘Pending’ State: If a test failed three times in a row, it was moved to a "Pending" state while the team investigated.
- Review Regularly: Scheduled reviews of flaky tests helped us determine if they needed fixes or if they should be deprecated.
Example CI/CD Configuration Snippet:
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Run tests
run: |
./gradlew test
if [ $? -ne 0 ]; then
echo "Test failed, moving to pending state";
# Custom logic here to handle flaky tests
fi
The Result
By addressing flaky tests, we ensured that our tests provided reliable feedback and that we maintained a constant deployment cadence without unnecessary delays.
Bringing It All Together
Continuous Deployment is undoubtedly a powerful aspect of modern software development. However, it’s crucial to remain vigilant about its potential pitfalls. From fragile systems to unclear communication, overwhelming feedback, and testing burdens, each challenge can derail progress if not properly managed.
By adopting enhanced testing strategies, improving communication, prioritizing user feedback, and handling flaky tests, we transformed our deployment processes. Our experiences are testament to the fact that, while Continuous Deployment speeds up development, a thoughtful approach ensures that quality, communication, and visionary alignment remain at the forefront.
For more information on Continuous Deployment methodologies, I recommend these resources:
- Continuous Deployment: What It Is and How to Implement It
- Automated Testing Best Practices
Whether you are considering implementing Continuous Deployment or if you're already on this path, take a moment to reflect on these potential pitfalls. A proactive approach will set your team up for success. Happy deploying!