Sorting HashMap Values in Java 8: Common Pitfalls to Avoid
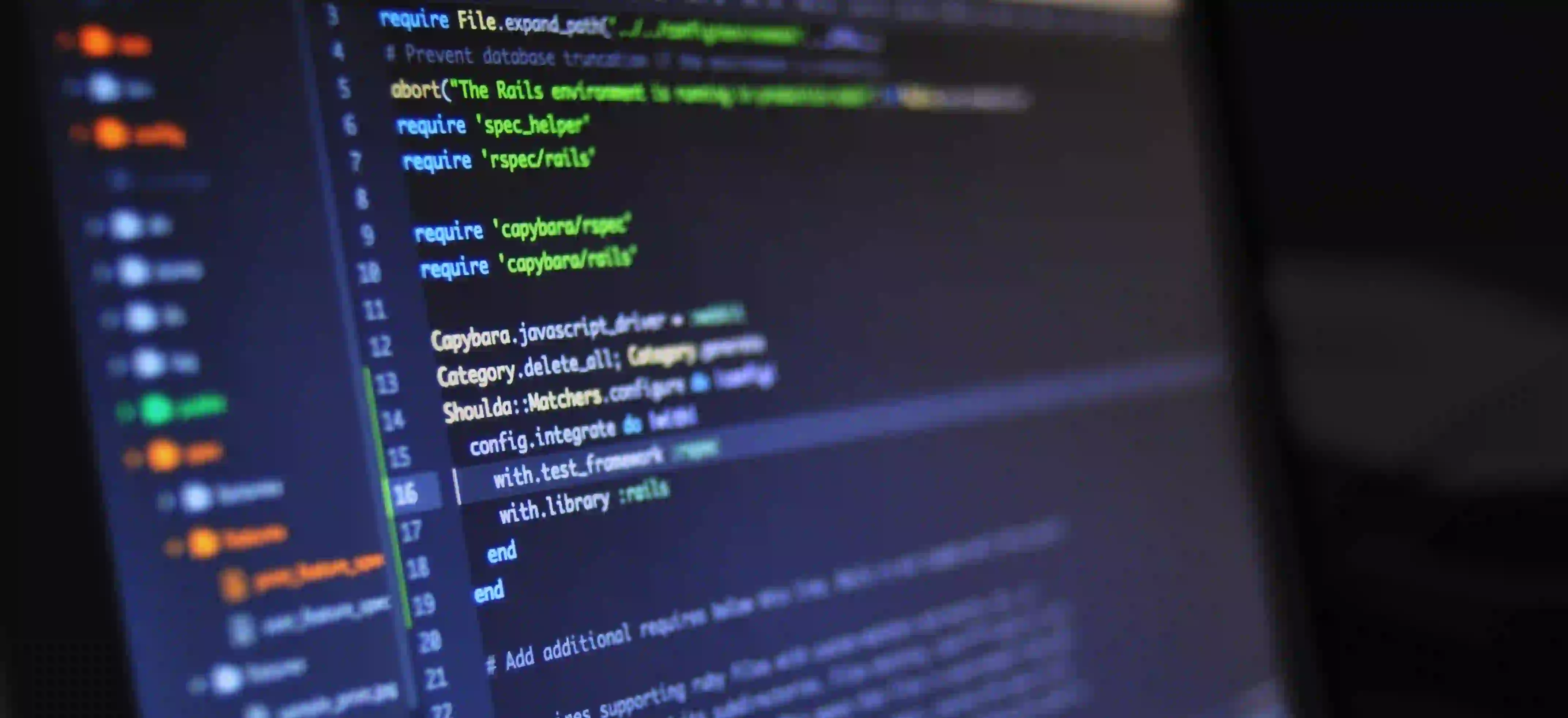
Sorting HashMap Values in Java 8: Common Pitfalls to Avoid
In the realm of Java programming, efficiently working with data structures is a must. One common scenario developers face is sorting values within a HashMap. Although sorting might seem straightforward, Java 8 introduced additional features that can lead to both elegant solutions and common pitfalls. In this blog post, we will explore these nuances and provide practical examples to ensure you avoid mistakes.
Understanding HashMap and Ordering
Before diving into sorting techniques, it’s crucial to understand what a HashMap is. A HashMap is a collection that maps keys to values. The main characteristic is that it does not guarantee any order of its elements. Thus, when you need to sort the values, you need to first convert this unordered structure into a sorted one.
Sorting Strategies in Java 8
Java 8 brought in the Stream API
, which allows for more functional-style programming. Sorting values in a HashMap can be done using streams, but developers often trip over subtleties. Below, we explore the correct approach and common pitfalls.
Example HashMap
Let's start with a simple HashMap that we will sort.
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("A", 3);
map.put("B", 1);
map.put("C", 2);
// The map is unsorted: {A=3, B=1, C=2}
}
}
Sorting the Values
To sort the values of the above HashMap, we can convert it into a stream and then sort it:
import java.util.*;
import java.util.stream.*;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("A", 3);
map.put("B", 1);
map.put("C", 2);
List<Map.Entry<String, Integer>> sortedEntries = map.entrySet()
.stream()
.sorted(Map.Entry.comparingByValue())
.collect(Collectors.toList());
// Output the sorted entries
sortedEntries.forEach(entry -> System.out.println(entry.getKey() + ": " + entry.getValue()));
}
}
What Does this Code Do?
- Streams the HashMap:
map.entrySet().stream()
converts the HashMap entries into a stream. - Sorting:
sorted(Map.Entry.comparingByValue())
sorts the entries based on their values. - Collecting Results: Finally,
collect(Collectors.toList())
gathers the sorted entries back into a List.
Common Pitfalls to Avoid
While the above approach works efficiently, there are common pitfalls developers encounter when sorting HashMap values.
1. Confusing Key-Value Pairs with Streams
A frequent mistake is treating the stream of entries as a stream of keys or values, which can lead to exceptions or incorrect sort conditions. Make sure to always work with Map.Entry<K, V>
when dealing with entries.
2. Modifying the Original HashMap
Another classic error is modifying the original HashMap while sorting. Doing so can lead to unexpected behavior. For example:
// Risky: Modifying map during sorting
map.put("D", 4);
Instead, create a copy of the original map if you plan to modify it during the sorting process.
3. Assumptions About Unique Values
When sorting, you might assume that all values are unique, but that isn't always the case. If there are duplicates, the sorted output may not preserve the original insertion order for equal elements.
To handle duplicates, consider using a more advanced structure like LinkedHashMap
if you want to maintain insertion order after sorting.
Handling Sorting with Duplicates
To demonstrate how you can handle situations where values are not unique, consider the following example:
import java.util.*;
import java.util.stream.*;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("A", 3);
map.put("B", 1);
map.put("C", 2);
map.put("D", 3); // Duplicate value
List<Map.Entry<String, Integer>> sortedEntries = map.entrySet()
.stream()
.sorted(Map.Entry.comparingByValue())
.collect(Collectors.toList());
sortedEntries.forEach(entry -> System.out.println(entry.getKey() + ": " + entry.getValue()));
}
}
In this case, keys "A" and "D" will appear next to each other when sorting by value.
Sorting by Multiple Criteria
Sometimes you might want to sort by multiple criteria. For example, if two entries have the same value, sorting them by key name would be another layer of sorting. Here is how you can achieve that:
List<Map.Entry<String, Integer>> sortedEntries = map.entrySet()
.stream()
.sorted(Map.Entry.<String, Integer>comparingByValue()
.thenComparing(Map.Entry.comparingByKey()))
.collect(Collectors.toList());
In this code:
- We first sort by
value
. - Then, in case of ties, we sort by
key
.
A Final Look
Sorting values in a HashMap can be performed elegantly using Java 8’s Stream API, but caution is needed. Common pitfalls include confusing pairs, modifying the original HashMap, and overlooking unique values.
By adhering to the best practices discussed—such as using streams thoughtfully and knowing how to handle duplicates—you can ensure your code remains robust and efficient.
Further Reading: For deeper insights into Java collections and best practices, you may find these resources useful:
By understanding these concepts, you will not only be able to sort HashMaps effectively but also elevate your overall programming skill set.