Preventing External Resource Delays in Java Applications
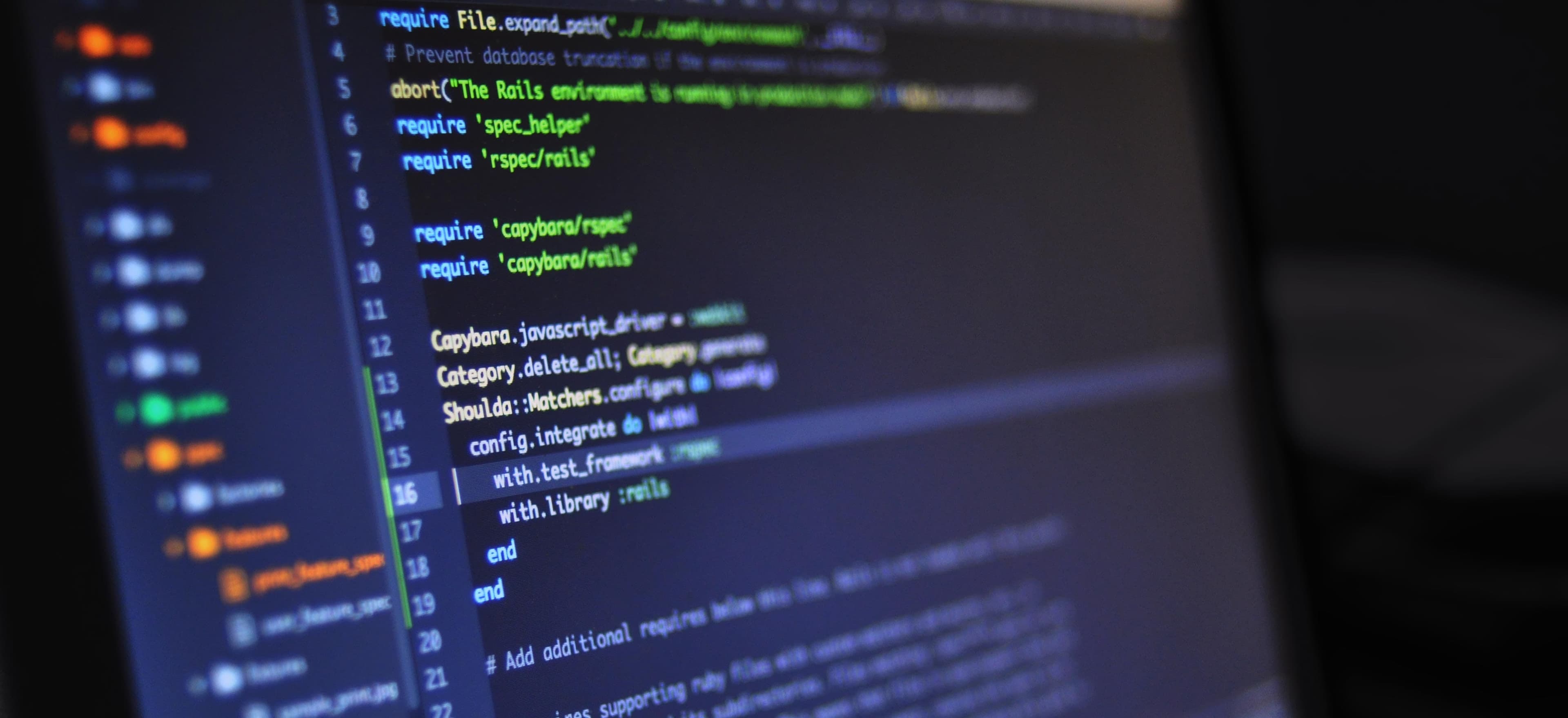
- Published on
Preventing External Resource Delays in Java Applications
In today's hyper-connected world, the performance of web applications hinges not just on the efficiency of the server-side logic but also on how external resources are fetched and managed. Slow external resources can create a lag, frustrating users and potentially harming your site's SEO. This article delves into best practices for preventing external resource delays specifically in Java applications.
Understanding External Resource Delays
External resource delays occur when a Java application tries to fetch data or assets from third-party services, APIs, or CDNs. This process can introduce latency that, if not managed properly, will slow down your application and degrade user experience. You can learn more about this in the article How to Stop External Links from Disrupting Your Site's Load.
Key Best Practices
Here are some important strategies you can adopt to minimize external resource delays in your Java applications.
1. Asynchronous Calls
One of the most effective methods to prevent blocking your application's threads while waiting for external resources is to make asynchronous calls. Java provides several techniques for achieving this, from using simple threads to using more advanced frameworks like CompletableFuture or Spring's @Async annotation.
Example with CompletableFuture
import java.util.concurrent.CompletableFuture;
public class ExternalServiceCaller {
public CompletableFuture<String> fetchDataAsync(String url) {
return CompletableFuture.supplyAsync(() -> fetchData(url));
}
private String fetchData(String url) {
// Simulating an external REST API call
try {
// Replace this with your actual HTTP call
Thread.sleep(2000); // Simulate delay
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Data fetched from " + url;
}
}
Why this matters: By using CompletableFuture
, we allow the main thread to continue executing without waiting for the external request to complete. This method promotes efficient resource use and enhances the responsiveness of your application.
2. Caching Responses
Caching is a straightforward way to deal with repeated requests for the same resource. When you have previously fetched data and are likely to fetch it again, storing this data temporarily can dramatically improve performance.
Example with Guava Cache
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.Cache;
import java.util.concurrent.TimeUnit;
public class CacheExample {
private Cache<String, String> cache = CacheBuilder.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(100)
.build();
public String getData(String url) {
return cache.get(url, () -> fetchData(url));
}
private String fetchData(String url) {
// Fetch data as needed. This method might take time due to external API call.
return "Data fetched from " + url;
}
}
Why this matters: Caching not only reduces the number of calls sent to external services but also speeds up response time for users by serving pre-fetched results from memory.
3. Timeout Management
Setting proper timeouts on external service calls can help your application remain responsive, even when the external resource is slow. You can specify time limits for how long to wait for a response before declaring a failure.
Example Using HttpURLConnection
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class NetworkClient {
private static final int TIMEOUT = 5000; // 5 seconds
public String fetchFromExternalApi(String endpoint) throws Exception {
URL url = new URL(endpoint);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(TIMEOUT);
connection.setReadTimeout(TIMEOUT);
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
try (BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()))) {
StringBuilder response = new StringBuilder();
String line;
while ((line = in.readLine()) != null) {
response.append(line);
}
return response.toString();
}
}
throw new Exception("Failed to fetch data: " + responseCode);
}
}
Why this matters: By implementing an adequate timeout, you prevent your application from waiting indefinitely for an external service's response, enabling it to handle other requests efficiently without becoming unresponsive.
4. Load Balancing and Failover
When using external services, it's crucial to implement load balancing and failover strategies. This way, if one resource is slow or unresponsive, you can gracefully failover to a different resource or retry the request.
Example with Retry Logic
import java.util.Random;
public class ExternalServiceWithRetry {
private static final int MAX_RETRIES = 3;
public String fetchDataWithRetry(String url) {
int attempt = 0;
while (attempt < MAX_RETRIES) {
try {
return fetchData(url);
} catch (Exception e) {
attempt++;
System.out.println("Attempt " + attempt + ": Failed to fetch data. Retrying...");
}
}
throw new RuntimeException("Failed to fetch data after retries");
}
private String fetchData(String url) {
if (new Random().nextBoolean()) {
throw new RuntimeException("Simulated fetch failure");
}
return "Data fetched from " + url;
}
}
Why this matters: By implementing retry logic, you prepare your application to handle external service failures more resiliently, thereby maintaining an enhanced user experience during outages.
5. Profiling and Monitoring
To effectively prevent external resource delays, regularly profiling and monitoring your application’s performance is essential. Tools like JVisualVM or New Relic can help identify slow requests.
How to do this effectively:
- Use APM (Application Performance Monitoring) tools to track external API call times.
- Setup alerts for abnormal latency, enabling swift action or adjustments.
To Wrap Things Up
Improving the performance of Java applications that rely on external resources involves several strategies, including asynchronous calls, caching, timeout management, load balancing, and continuous monitoring. Implementing these strategies will not only enhance user experience but also optimize overall application performance.
As the digital landscape continues to evolve, keeping abreast of best practices—like those discussed herein—will help keep your Java applications running smoothly. And remember, if you want to dive deeper into managing external links and their effects on site loading times, check out How to Stop External Links from Disrupting Your Site's Load.
By following these strategies and continuously improving your application architecture, you'll be well-equipped to prevent external resource delays, elevating your project's success.
Feel free to explore each of these concepts further in your projects, with the understanding that great user experiences stem from attention to detail in how your applications interact with the world outside.