Navigating Async Issues in Java with CompletableFuture
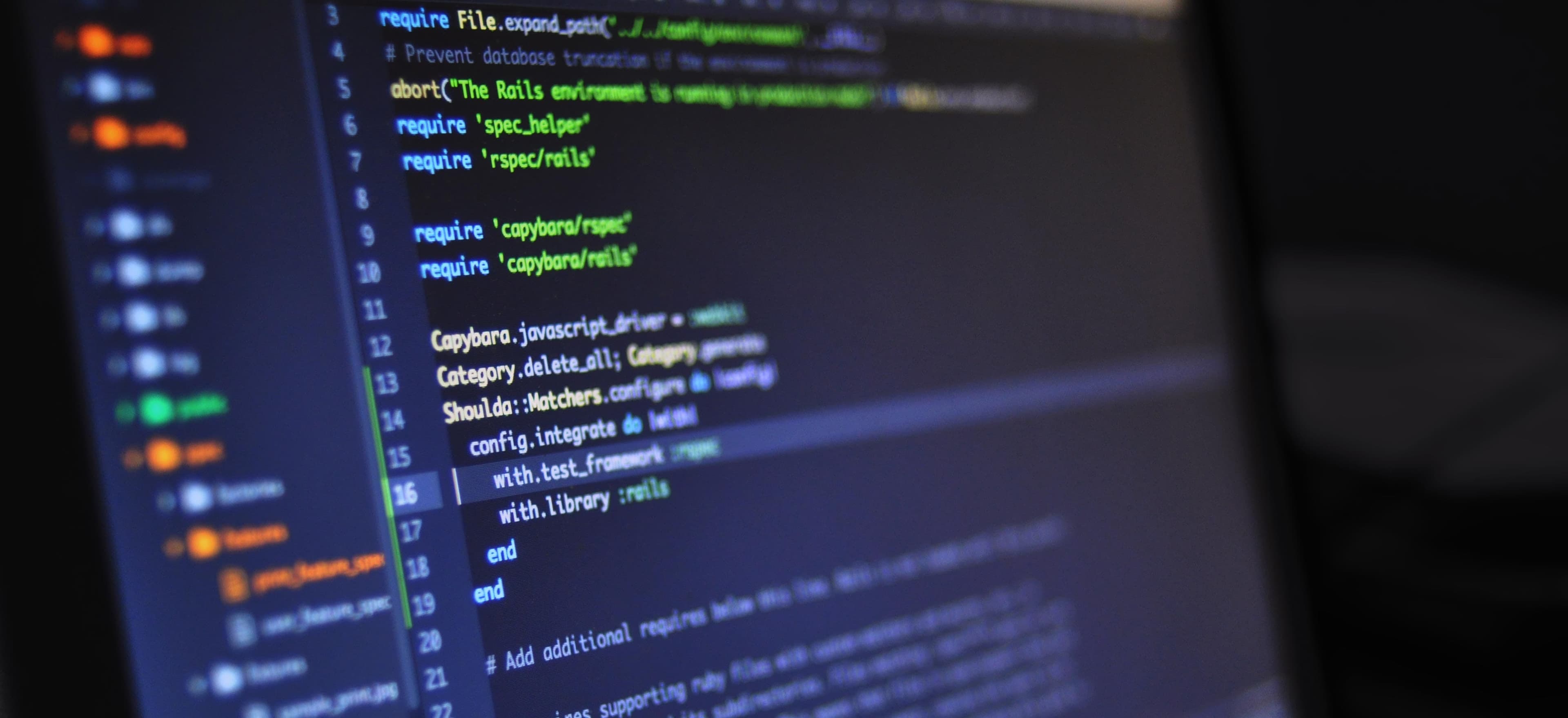
- Published on
Navigating Async Issues in Java with CompletableFuture
As technology evolves, the way we handle concurrent programming is becoming increasingly important in Java. One of the most significant advancements in Java's approach to asynchronous programming is the introduction of CompletableFuture
. In this blog post, we will delve into how to use CompletableFuture
effectively to manage asynchronous tasks without falling into the pitfalls of callback hell.
Understanding CompletableFuture
CompletableFuture
is part of the java.util.concurrent
package and was introduced in Java 8. Unlike traditional futures, which can only be completed once, a CompletableFuture
can be manually completed with the complete
method. This flexibility allows for better control when dealing with asynchronous operations.
Why Use CompletableFuture?
- Non-blocking: While traditional futures are blocking,
CompletableFuture
allows for non-blocking operations. - Chaining: You can chain multiple asynchronous operations together, making your code cleaner.
- Error Handling: It provides straightforward options for handling exceptions.
- Integration: It integrates well with the Stream API, making it a powerful tool for data processing tasks.
Getting Started with CompletableFuture
To start utilizing CompletableFuture
, ensure you're using Java 8 or above. Here is a simple example of creating and running a CompletableFuture
.
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulating long-running task
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result of the asynchronous task";
});
future.thenAccept(result -> {
// This block runs after the asynchronous task completes
System.out.println(result);
});
// Keep main thread alive until future is complete
future.join();
}
}
Explanation of the Code
In this code snippet, we perform a long-running task asynchronously using supplyAsync
. The thenAccept
method is called once the asynchronous operation completes. The future.join()
call blocks the main thread until the future is complete, ensuring we get the result before the program exits.
Chaining Multiple Asynchronous Tasks
One of the core benefits of CompletableFuture
is the ability to chain multiple operations. Let’s take a look at how we can achieve this.
import java.util.concurrent.CompletableFuture;
public class ChainingExample {
public static void main(String[] args) {
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
return 5;
}).thenApply(result -> {
// We square the result
return result * result;
}).thenApply(result -> {
// We add 10 to the squared result
return result + 10;
});
// Block and get the final result
Integer finalResult = future.join();
System.out.println("Final Result: " + finalResult);
}
}
Explanation of the Chaining Example
This code demonstrates how thenApply
can be chained. The first supplyAsync
provides an initial value (5), which is then squared and 10 is added to it. Each stage is executed only after the prior stage completes.
Handling Exceptions
With the power of asynchronous programming also comes the need for robust error handling. CompletableFuture
provides several methods for this purpose, such as exceptionally
and handle
.
import java.util.concurrent.CompletableFuture;
public class ExceptionHandlingExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
if (true) {
throw new RuntimeException("Something went wrong!");
}
return "Result";
})
.exceptionally(ex -> {
// Handle the exception and return a default value
return "Error: " + ex.getMessage();
});
System.out.println(future.join());
}
}
Explanation of the Exception Handling Example
In this example, the supplyAsync
method throws a RuntimeException
. We use the exceptionally
method to handle this exception and return a meaningful response instead of failing silently. This approach enhances the robustness of your code.
Integration with Stream API
CompletableFuture
can also be used in combination with Java’s Stream API for processing collections of data asynchronously.
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", "Charlie", "David");
List<CompletableFuture<String>> futureNames = names.stream()
.map(name -> CompletableFuture.supplyAsync(() -> processName(name)))
.collect(Collectors.toList());
// Wait for all futures to complete
List<String> results = futureNames.stream()
.map(CompletableFuture::join)
.collect(Collectors.toList());
results.forEach(System.out::println);
}
private static String processName(String name) {
return "Hello, " + name;
}
}
Explanation of the Stream Example
In this code snippet, we process a list of names asynchronously. Each name is processed in a separate CompletableFuture
, demonstrating how you can leverage both the Stream API and CompletableFuture for efficient asynchronous processing.
Real-World Applications
The CompletableFuture
class is particularly useful in scenarios such as:
- Web Services: Making multiple API calls concurrently and aggregating results.
- Database Operations: Performing CRUD operations without blocking the main thread.
- File Processing: Reading and transforming large files in chunks.
By adopting these practices, you can create responsive applications that handle asynchronous tasks with ease.
For further reading on handling callbacks in JavaScript and finding inspiration for perfecting asynchronous programming, you may refer to this enlightening article: Unraveling Callback Hell: Mastering Async JS & Promises.
Wrapping Up
CompletableFuture
is a powerful tool that simplifies asynchronous programming in Java. By utilizing features such as chaining, error handling, and integration with the Stream API, you can create robust and scalable applications. Embrace asynchronous programming, and unleash the full potential of Java with CompletableFuture
!
By understanding and applying these concepts, you will not only enhance your Java skills but also deliver higher-quality applications that can operate seamlessly in a concurrent environment. Happy coding!
Checkout our other articles