Java Solutions for Managing Water Quality Data Efficiently
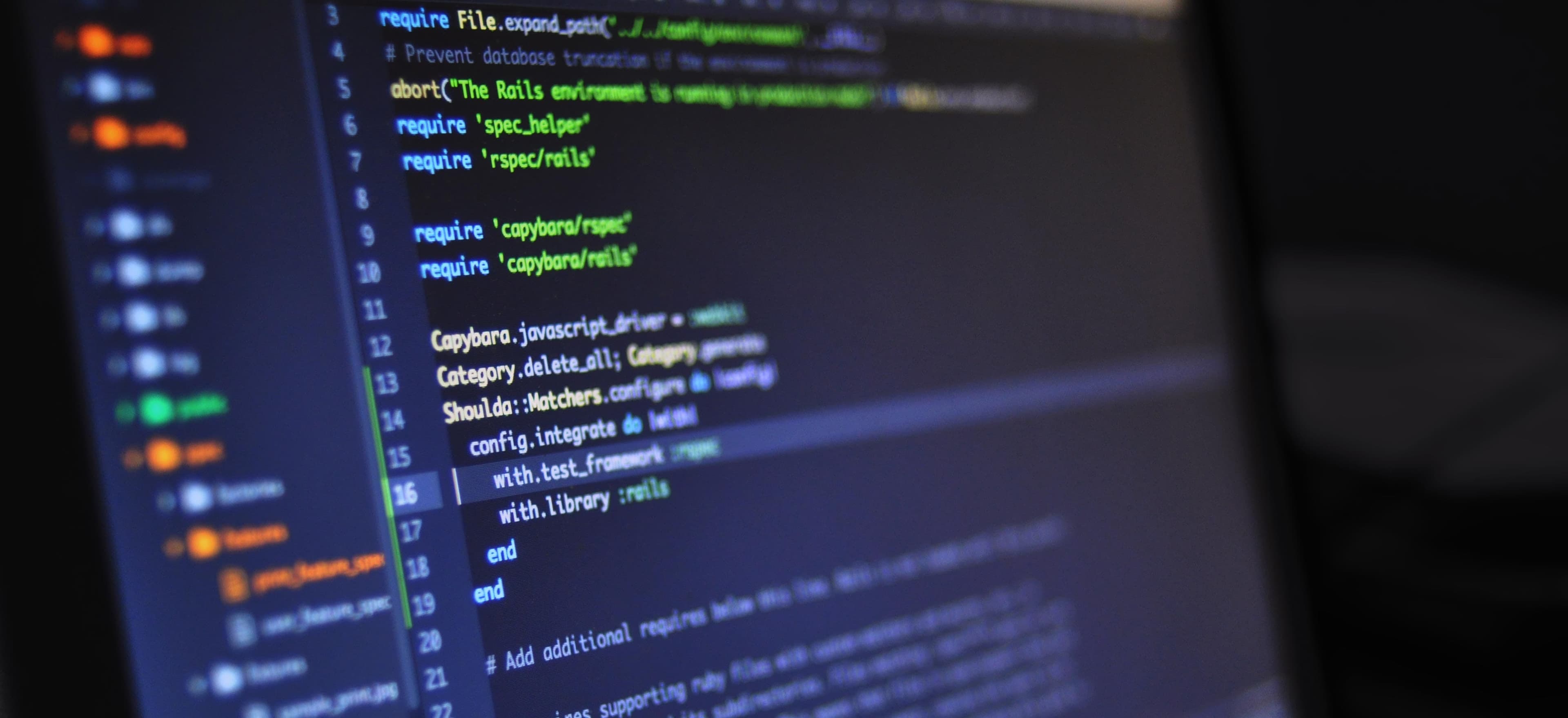
- Published on
Java Solutions for Managing Water Quality Data Efficiently
Water quality data is crucial for environmental monitoring, public health, and resource management. As the demand for clean water rises, efficient data handling becomes imperative. This is where Java, with its robustness and extensive libraries, steps in as an ideal choice for developing applications to manage water quality data effectively. In this blog post, we will explore how Java can be utilized for this purpose, along with code snippets and real-world applications.
Understanding Water Quality Data
Water quality data typically includes various parameters such as pH levels, turbidity, conductivity, and the presence of contaminants. Organizations and researchers collect this data over time to assess the health of water bodies and ensure safe drinking water. Managing this data effectively requires systems that can process, analyze, and visualize the data with ease.
Why Choose Java for Water Quality Data Management?
Java comes with a host of benefits that make it ideal for managing complex data sets:
-
Platform Independence: Java applications can run on any device that supports the Java Virtual Machine (JVM). This is essential for data management systems that may be deployed across various environments.
-
Robust Libraries: The language provides extensive libraries and frameworks such as JavaFX for GUI development and JDBC for database connectivity. These tools streamline the development of comprehensive data management systems.
-
Strong Community Support: With a vast community, developers have access to a wealth of resources, libraries, and frameworks that can speed up the development process.
-
Scalability: As data volumes grow, Java applications can be scaled efficiently without compromising performance.
Key Components of a Water Quality Data Management System
- Data Collection: Using sensors or manual input to gather data from water sources.
- Data Storage: Storing data in a database for retrieval and analysis.
- Data Analysis: Performing calculations and generating reports based on the data.
- Data Visualization: Providing graphical interpretations of the data.
Example Implementation
Let's create a simple water quality data management application. We'll focus on how to collect, store, and retrieve water quality data using Java.
Step 1: Define a Water Quality Data Model
Creating a model helps represent our water quality data consistently.
public class WaterQualityData {
private double pH;
private double turbidity;
private double conductivity;
private String date;
// Constructor
public WaterQualityData(double pH, double turbidity, double conductivity, String date) {
this.pH = pH;
this.turbidity = turbidity;
this.conductivity = conductivity;
this.date = date;
}
// Getters
public double getPH() { return pH; }
public double getTurbidity() { return turbidity; }
public double getConductivity() { return conductivity; }
public String getDate() { return date; }
// Display method
public String displayData() {
return "Date: " + date + ", pH: " + pH + ", Turbidity: " + turbidity + " NTU, Conductivity: " + conductivity + " µS/cm";
}
}
Why a Data Model? A structured model allows us to encapsulate the properties of water quality data, making it easier to manipulate and transfer data between different parts of our application.
Step 2: Database Connectivity Using JDBC
To store our data, we will use a simple SQLite database. First, we need to create a database that can hold our water quality data.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class DatabaseConnection {
private static final String URL = "jdbc:sqlite:waterquality.db";
public static Connection connect() {
Connection conn = null;
try {
conn = DriverManager.getConnection(URL);
System.out.println("Connection to SQLite has been established.");
} catch (Exception e) {
e.printStackTrace();
}
return conn;
}
public void createTable() {
String sql = "CREATE TABLE IF NOT EXISTS water_quality ("
+ "id INTEGER PRIMARY KEY,"
+ "pH REAL,"
+ "turbidity REAL,"
+ "conductivity REAL,"
+ "date TEXT);";
try (Connection conn = this.connect(); PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
public void insertData(WaterQualityData data) {
String sql = "INSERT INTO water_quality(pH, turbidity, conductivity, date) VALUES(?,?,?,?)";
try (Connection conn = this.connect(); PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setDouble(1, data.getPH());
pstmt.setDouble(2, data.getTurbidity());
pstmt.setDouble(3, data.getConductivity());
pstmt.setString(4, data.getDate());
pstmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
public void fetchData() {
String sql = "SELECT * FROM water_quality";
try (Connection conn = this.connect(); PreparedStatement pstmt = conn.prepareStatement(sql); ResultSet rs = pstmt.executeQuery()) {
while (rs.next()) {
System.out.println("Record: " + new WaterQualityData(rs.getDouble("pH"), rs.getDouble("turbidity"), rs.getDouble("conductivity"), rs.getString("date")).displayData());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why Use JDBC? JDBC (Java Database Connectivity) allows for seamless interaction with databases. Using a library like SQLite provides a lightweight solution suitable for local data storage.
Step 3: Putting It All Together
Finally, we’ll create a simple application to collect and store water quality data.
import java.util.Scanner;
public class WaterQualityApp {
public static void main(String[] args) {
DatabaseConnection db = new DatabaseConnection();
db.createTable();
Scanner scanner = new Scanner(System.in);
System.out.println("Enter water quality data:");
System.out.print("pH: ");
double pH = scanner.nextDouble();
System.out.print("Turbidity (NTU): ");
double turbidity = scanner.nextDouble();
System.out.print("Conductivity (µS/cm): ");
double conductivity = scanner.nextDouble();
// Using current date as an example
String date = new java.util.Date().toString();
WaterQualityData data = new WaterQualityData(pH, turbidity, conductivity, date);
db.insertData(data);
System.out.println("Data inserted successfully.");
System.out.println("Fetching all data records:");
db.fetchData();
scanner.close();
}
}
Why User Input? Gathering user input allows for real-time data collection, ensuring that the application can be used flexibly in different scenarios.
Wrapping Up
In summary, Java offers an efficient and structured way to manage water quality data. By leveraging its robust libraries and scalability, developers can create comprehensive data management systems that enhance the monitoring and analysis of water quality.
For further insights on the implications of water purification systems, such as Does Your Berkey Filter Strip Water of Essential Minerals? visit youvswild.com/blog/berkey-filter-essential-minerals.
By adopting these technologies, organizations and researchers can ensure they are working with accurate and reliable water quality data, ultimately contributing to better environmental and public health outcomes.
Checkout our other articles