Evaluating Java Networking Solutions for Real-Time Data Streams
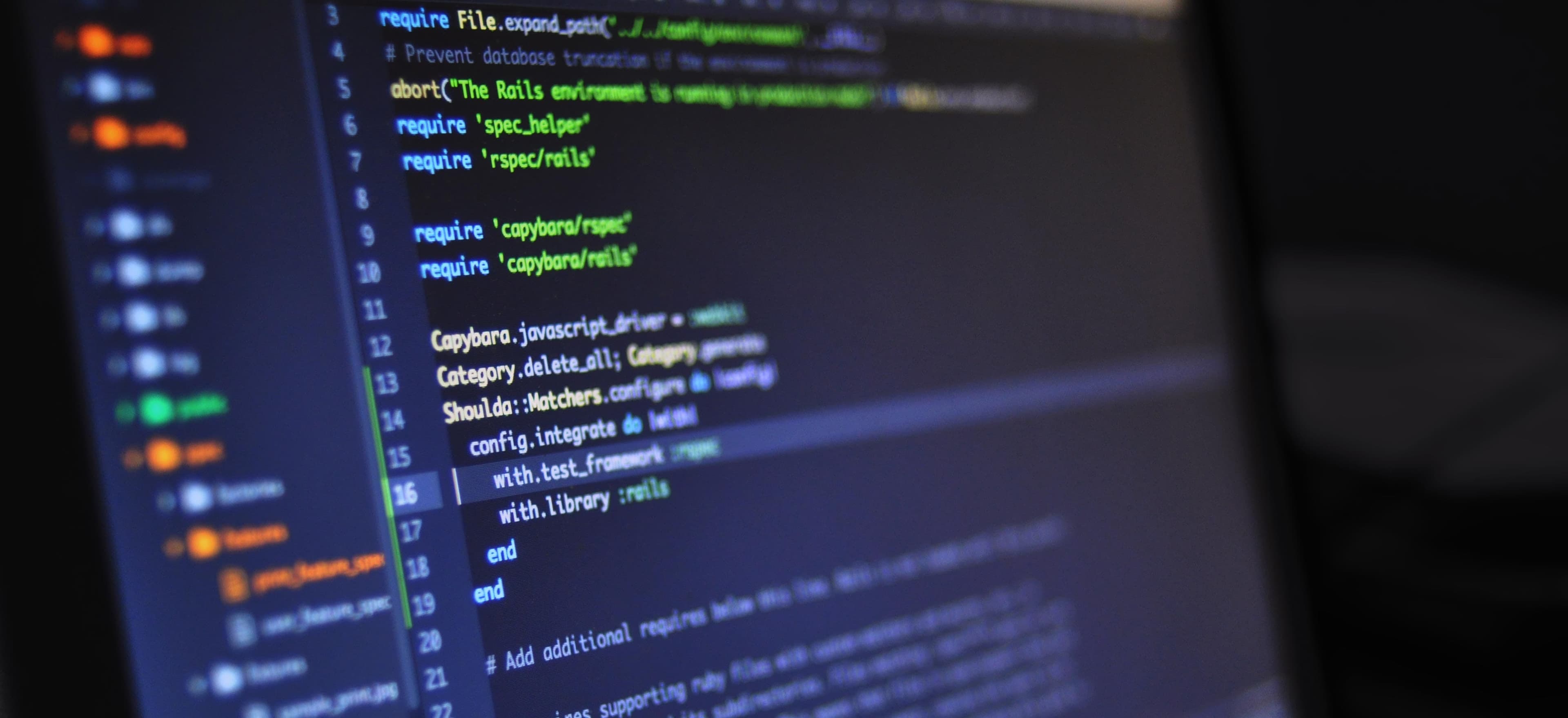
- Published on
Evaluating Java Networking Solutions for Real-Time Data Streams
In the era of rapid technological advancement, the demand for efficient real-time data streaming is ever-increasing. Java, a popular programming language, offers various networking solutions that can effectively manage and process data streams in real-time. In this blog post, we will evaluate different Java networking solutions that are apt for real-time data streaming, discuss their advantages and implementation details, and provide useful examples to showcase their capabilities.
Understanding Real-Time Data Streaming
Before we dive into Java networking solutions, let's define what real-time data streaming means. In essence, real-time data streaming refers to the continuous flow of data, allowing users and applications to process information instantly as it's generated. This capability is vital in various sectors, ranging from finance and healthcare to weather monitoring and IoT devices.
As an example, if a weather application needs to fetch data regarding weather conditions like temperature or humidity, a real-time data streaming solution will allow the app to receive this information without delay. For further reading on how real-time data can impact specific applications, you might find the article "NOAA Weather Radio Woes: Finding the Right Channel" insightful. You can read it here.
Java Networking Solutions
Java's rich set of networking libraries enables developers to build applications that can handle real-time data streams. Here, we will discuss some prominent solutions:
- Java Sockets
- Java NIO (Non-blocking I/O)
- WebSockets
- Apache Kafka
- Spring WebFlux
1. Java Sockets
Java Sockets provide the foundational building blocks for network communication. Using sockets, you can establish a client-server architecture for sending and receiving data.
How Do Java Sockets Work?
Sockets establish a communication endpoint. The client and server create a socket, bind it to a port, and begin exchanging data.
Example Code Snippet
Below is a simple server-client interaction using Java Sockets:
Server Code:
import java.io.*;
import java.net.*;
public class SimpleServer {
public static void main(String[] args) {
try (ServerSocket serverSocket = new ServerSocket(1234)) {
System.out.println("Server is listening on port 1234");
Socket socket = serverSocket.accept();
System.out.println("Client connected");
// Data input and output stream
InputStream input = socket.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(input));
String message = reader.readLine();
// Print received message
System.out.println("Message from client: " + message);
// Close connections
socket.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
Why this code is significant:
- The
ServerSocket
listens for incoming client connections on port 1234. - The socket accepts client connections, reading data directly as it arrives.
Client Code:
import java.io.*;
import java.net.*;
public class SimpleClient {
public static void main(String[] args) {
try (Socket socket = new Socket("localhost", 1234)) {
// Output stream for sending messages
PrintWriter writer = new PrintWriter(socket.getOutputStream(), true);
writer.println("Hello, Server!");
// Close connection
socket.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
Why this code is significant:
- The client initiates a connection to the server and sends a message, allowing real-time data exchange.
2. Java NIO (Non-blocking I/O)
Java NIO can offer enhanced performance for real-time data streaming, especially when dealing with multiple channels. Non-blocking I/O allows handling multiple connections with a single thread, which is more efficient compared to the traditional blocking I/O method.
Example Code Snippet
Here’s a basic example of Java NIO in action:
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
public class NIOServer {
public static void main(String[] args) throws IOException {
Selector selector = Selector.open();
ServerSocketChannel serverSocketChannel = ServerSocketChannel.open();
serverSocketChannel.bind(new InetSocketAddress(1234));
serverSocketChannel.configureBlocking(false);
serverSocketChannel.register(selector, serverSocketChannel.validOps());
while (true) {
selector.select();
Iterator selectedChannel = selector.selectedKeys().iterator();
while (selectedChannel.hasNext()) {
SocketChannel socketChannel = (SocketChannel) selectedChannel.next();
// Read Input
ByteBuffer buffer = ByteBuffer.allocate(256);
socketChannel.read(buffer);
String received = new String(buffer.array()).trim();
System.out.println("Received: " + received);
selectedChannel.remove();
}
}
}
}
Why this code is significant:
- Using
Selector
allows the server to manage multiple client connections in an efficient manner. - It reduces thread management overhead, crucial for applications that require high-performance data streaming.
3. WebSockets
WebSockets offer a modern approach to real-time communication, enabling bi-directional data flow between clients and servers. This protocol is particularly useful for web applications, chat services, and real-time updates.
Example Code Snippet
To illustrate, here is an example of a basic WebSocket server using the javax.websocket
API:
Server Code:
import javax.websocket.*;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/echo")
public class WebSocketServer {
@OnOpen
public void onOpen(Session session) {
System.out.println("Connected: " + session.getId());
}
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("Received: " + message);
session.getAsyncRemote().sendText("Echo: " + message);
}
@OnClose
public void onClose(Session session) {
System.out.println("Disconnected: " + session.getId());
}
}
Why this code is significant:
- The
@ServerEndpoint
annotation indicates a WebSocket server endpoint. - It allows multiple clients to connect and communicate simultaneously, making it suitable for real-time communications.
4. Apache Kafka
When it comes to handling vast amounts of streaming data, Apache Kafka shines. It is a distributed streaming platform that allows applications to publish and subscribe to streams in real-time.
Example Code Snippet
Setting up a basic Kafka producer and consumer in Java might look like this:
Producer Code:
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
import java.util.Properties;
public class SimpleKafkaProducer {
public static void main(String[] args) {
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
producer.send(new ProducerRecord<>("topicName", "key", "Hello, Kafka!"));
producer.close();
}
}
Why this code is significant:
- The producer sends messages to Kafka to a specified topic, making it highly scalable and effective for real-time data streaming.
5. Spring WebFlux
For reactive programming, Spring WebFlux provides a robust framework. It allows building non-blocking applications, making it perfect for real-time data processing.
Example Code Snippet
Here is a simple WebFlux controller:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Flux;
@RestController
public class ReactiveController {
@GetMapping("/stream")
public Flux<String> streamFlux() {
return Flux.interval(Duration.ofSeconds(1))
.map(i -> "Current time: " + LocalDateTime.now());
}
}
Why this code is significant:
- The controller exposes an endpoint that streams data every second.
- This is useful for applications needing constant updates, such as dashboards or monitoring tools.
Lessons Learned
Evaluating Java networking solutions for real-time data streams reveals a diverse array of options. Java Sockets provide basic functionality, while Java NIO and WebSockets offer more advanced performance benefits. For heavy data loads, Apache Kafka is the go-to choice, whereas Spring WebFlux excels in reactive programming scenarios.
Each solution has its strengths and should be chosen based on the specific requirements of your application. Make sure to assess your requirements, architecture, and expected load to select the best technology for real-time data streaming.
As you explore these solutions, be mindful of the underlying principles driving real-time data systems. For further insights, don't forget to check the article "NOAA Weather Radio Woes: Finding the Right Channel" here.
By understanding these Java networking solutions, you're now better equipped to implement an effective real-time data strategy in your applications. Happy coding!
Checkout our other articles