Streamlining Java Apps: Handling Channel Conflicts Efficiently
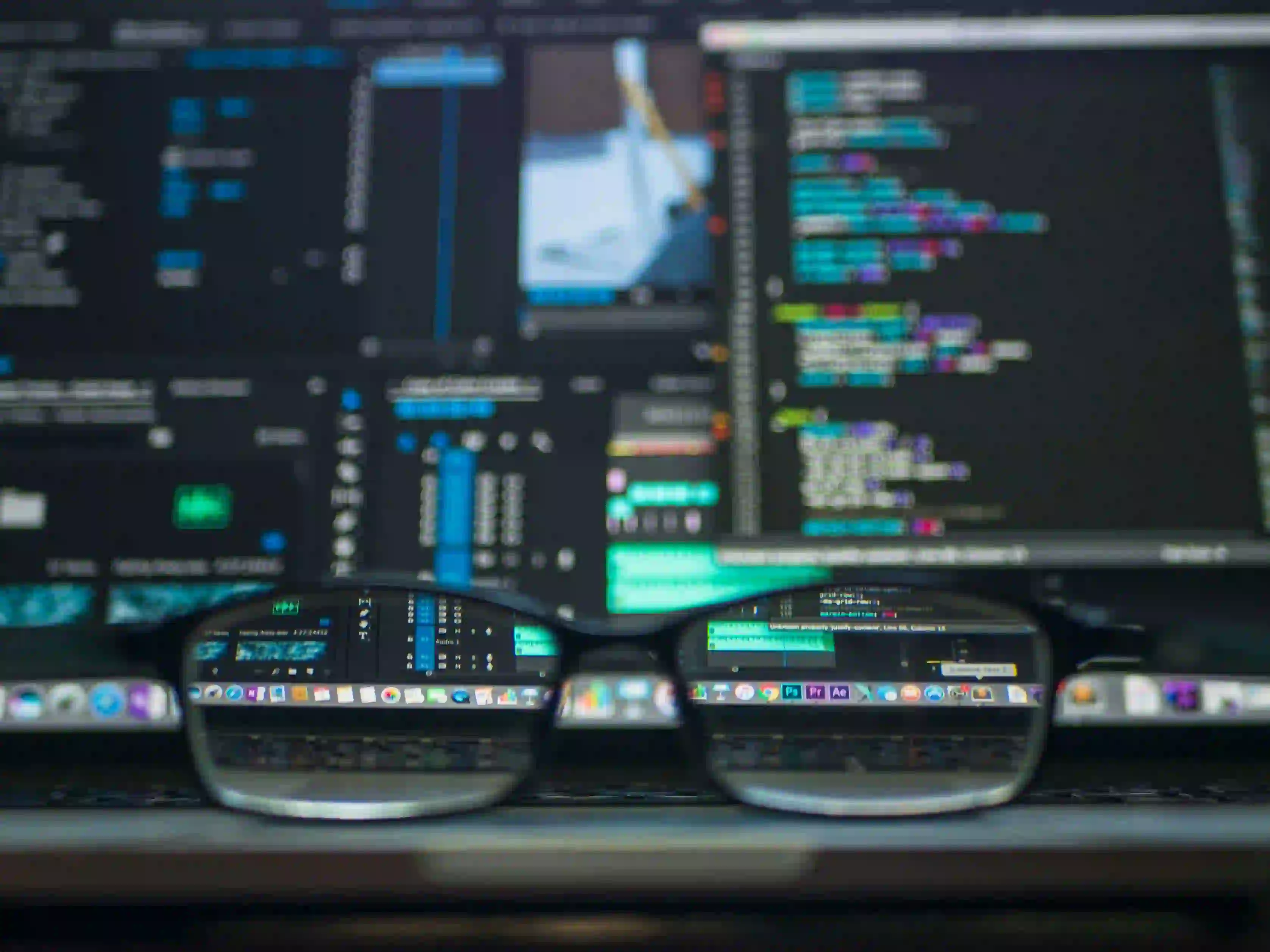
Streamlining Java Apps: Handling Channel Conflicts Efficiently
In today's interconnected world, channel management and conflict resolution are vital, especially when dealing with data streams and communication protocols. This article will delve into streamlining Java applications by effectively handling channel conflicts, drawing inspiration from concepts discussed in articles like "NOAA Weather Radio Woes: Finding the Right Channel" (youvswild.com/blog/noaa-weather-radio-woes-right-channel).
Through the use of practical examples and code snippets, we’ll explore how to optimize your Java applications for better performance and lower latency when dealing with channel conflicts.
Understanding Channel Conflicts
Before we jump into solutions, it’s essential to understand what channel conflicts are. Simply put, a channel conflict occurs when two or more processes attempt to communicate or send data over the same channel simultaneously. This can lead to data loss, transmission errors, or even application crashes if not handled efficiently.
In our case, Java applications often rely on threads and concurrent data handling to optimize performance. When multiple threads attempt to send data through a shared resource, conflicts arise. Therefore, we need robust mechanisms to ensure data integrity and boost performance in multi-threaded operations.
Importance of Synchronization
In Java, one can leverage synchronization to handle channel conflicts. Synchronization ensures that only one thread can access a particular piece of code or resource at a time, preventing a state where multiple threads may disrupt each other's operations.
Here's a simple example:
class SharedChannel {
private int data;
public synchronized void updateData(int newData) {
this.data = newData;
}
public synchronized int readData() {
return this.data;
}
}
Why Use Synchronization?
-
Data Integrity: This guarantees that only one thread is updating or reading data at any time, effectively preventing data corruption.
-
Thread Safety: By modifying the
updateData
andreadData
methods to be synchronized, we assure that operations are safe and predictable.
Implementing Locks for Advanced Control
While synchronization is straightforward, Java offers other mechanisms for more granular control. Enter Java's ReentrantLock
, which provides additional features for managing locks and improving performance.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
class AdvancedChannel {
private int data;
private final Lock lock = new ReentrantLock();
public void updateData(int newData) {
lock.lock(); // Acquire the lock
try {
this.data = newData;
} finally {
lock.unlock(); // Ensure that the lock is released
}
}
public int readData() {
lock.lock(); // Acquire the lock
try {
return this.data;
} finally {
lock.unlock(); // Ensure that the lock is released
}
}
}
Why Use Locking?
-
Fairness:
ReentrantLock
can be configured for fairness, allowing threads to access resources in the order they requested them. -
Condition Variables: Locks support condition variables, which can be beneficial in complex scenarios where threads need to wait for certain conditions to be met before proceeding.
Handling Channel Conflicts in Java Applications
Now that we have a grasp on synchronization and locking, let’s talk about integrating conflict resolution strategies into Java applications.
Example: Concurrent Data Streams
Imagine a scenario in which multiple data pipelines process information and send it through a common channel. Here’s how you could manage these channels while avoiding conflicts.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
class DataPipeline implements Runnable {
private final SharedChannel channel;
private final int dataToSend;
public DataPipeline(SharedChannel channel, int dataToSend) {
this.channel = channel;
this.dataToSend = dataToSend;
}
public void run() {
channel.updateData(dataToSend);
System.out.println("Data sent: " + dataToSend);
}
}
public class Main {
public static void main(String[] args) {
SharedChannel sharedChannel = new SharedChannel();
ExecutorService executorService = Executors.newFixedThreadPool(3);
for (int i = 0; i < 5; i++) {
executorService.execute(new DataPipeline(sharedChannel, i));
}
executorService.shutdown();
}
}
Why Use an Executor Service?
-
Thread Management: Instead of manually creating and managing threads, using an
ExecutorService
simplifies the process, allowing you to focus on your application logic. -
Scalability: You can easily adjust the number of threads based on your application's needs without rewriting significant portions of code.
Exploring Alternative Conflict Resolution Strategies
While synchronization and locks are powerful techniques, it’s also worth exploring other strategies, such as using message queues or frameworks designed to handle concurrent processing like Apache Kafka. These can further streamline your Java applications.
Using Message Queues
Here’s a conceptual overview of using a message queue for channel management:
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
class MessageQueueApp {
private BlockingQueue<Integer> queue = new ArrayBlockingQueue<>(10);
public void send(int message) throws InterruptedException {
queue.put(message);
System.out.println("Message sent: " + message);
}
public int receive() throws InterruptedException {
int message = queue.take();
System.out.println("Message received: " + message);
return message;
}
}
Why Use a Message Queue?
-
Decoupling: A message queue decouples the sender and receiver, which can significantly improve application responsiveness and throughput.
-
Buffering: It allows for buffering data, helping manage bursts in data transmission without overloading the receiving application.
The Bottom Line
Handling channel conflicts efficiently is crucial in developing robust Java applications. By using proper synchronization techniques, locking mechanisms, and potentially integrating message queues, you can maintain data integrity and boost application performance. These strategies are not just theoretical; they are vital for real-world applications that require reliability under concurrency.
If you're interested in further examples of managing communication channels effectively, you might find our insights in "NOAA Weather Radio Woes: Finding the Right Channel" (youvswild.com/blog/noaa-weather-radio-woes-right-channel) particularly relevant. These principles of communication apply beyond weather radios to any system relying on effective channel management.
By effectively applying these concepts, you can streamline your Java applications, eliminate conflicts, and ensure a smooth flow of data in multi-threaded environments. Start implementing these strategies today and watch your application's performance soar!