Mastering Java for Real-time Weather Channel Updates
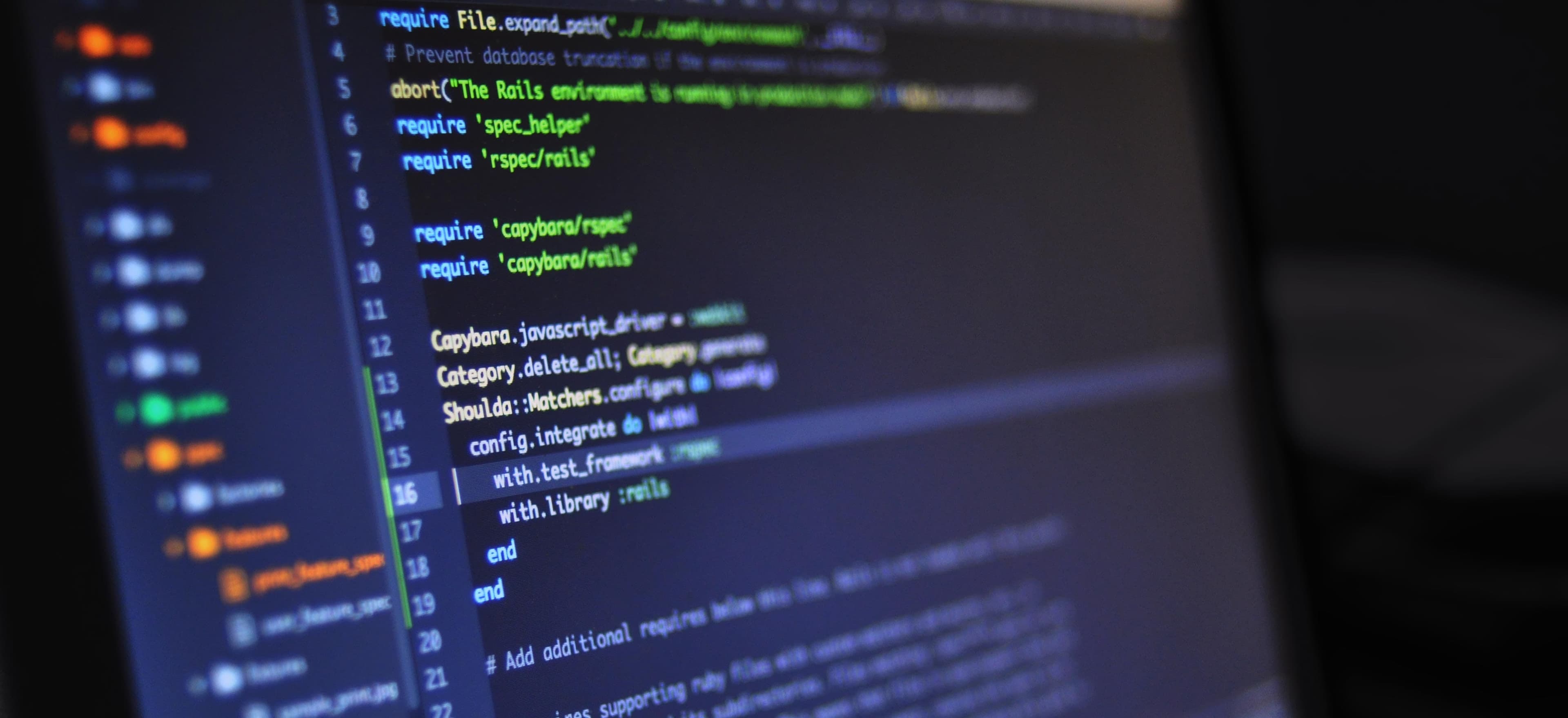
- Published on
Mastering Java for Real-time Weather Channel Updates
In today’s tech-savvy world, the need for real-time data is more prominent than ever. From stock market updates to weather predictions, the applications are endless. One specific area of interest is creating a Java application that provides real-time weather channel updates, reminiscent of the struggles highlighted in the article "NOAA Weather Radio Woes: Finding the Right Channel" (youvswild.com/blog/noaa-weather-radio-woes-right-channel). The significance of accurately receiving and processing weather data cannot be overstated.
This blog post will walk you through the essential steps to build a Java application that fetches live weather updates using an API. We'll delve into Java's strength in creating reliable applications, explore some best practices, and consider the ethical use of APIs.
Why Use Java for Weather Applications?
Java is a powerful, versatile programming language that’s platform-independent due to the JVM (Java Virtual Machine). This means any Java application can run on any system that has the JVM installed, which is crucial for applications like a weather updater that should be universally compatible. Additionally, the extensive libraries available in Java help simplify networking tasks and data parsing.
Key Benefits of Java
- Platform Independence: Write once, run anywhere (WORA) capability.
- Robust Libraries: Rapid development using libraries like Apache HttpClient and Gson for API calls and JSON parsing.
- Strong Community Support: Java has a mature community, providing a wealth of resources and libraries.
- Scalability: Applications can grow alongside business requirements without major rewrites.
Getting Started: Setting Up Your Java Environment
Before we can create our weather application, you'll need to set up your environment.
Prerequisites
- Java Development Kit (JDK)
- An Integrated Development Environment (IDE) such as IntelliJ IDEA, Eclipse, or NetBeans
- A weather API key from a service like OpenWeatherMap or WeatherAPI
Setting Up Your Project
- Create a new Java project in your IDE.
- Add dependencies for HTTP requests and JSON parsing. If you are using Maven, you can include the following dependencies in your
pom.xml
:
<dependencies>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version>
</dependency>
</dependencies>
These libraries will allow us to make REST API calls and parse JSON responses easily.
Making HTTP Requests to the Weather API
Sample Code Snippet for Fetching Weather Data
Now that your environment is set up, let’s write the code to request weather data.
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class WeatherClient {
private static final String API_KEY = "YOUR_API_KEY";
private static final String CITY = "London"; // Change city as needed
private static final String BASE_URL = "http://api.openweathermap.org/data/2.5/weather";
public static void main(String[] args) {
String url = BASE_URL + "?q=" + CITY + "&appid=" + API_KEY;
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
HttpGet request = new HttpGet(url);
try (CloseableHttpResponse response = httpClient.execute(request)) {
BufferedReader reader = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
String line;
StringBuilder result = new StringBuilder();
while ((line = reader.readLine()) != null) {
result.append(line);
}
System.out.println("Weather Data: " + result.toString());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation of the Code
-
HttpClient Initialization: We start by creating a
CloseableHttpClient
instance to enable us to send requests to the weather API. -
HttpGet Request: This defines the type of request (GET) and specifies the full URL to which we are making the request—including the API key.
-
Response Handling: We read the response from the InputStream and append each line to a
StringBuilder
for easy printing.
Why Use Try-with-Resources?
Using try-with-resources ensures that CloseableHttpClient
and CloseableHttpResponse
are properly closed after being used, preventing potential memory leaks.
Parsing JSON Response
Once we have our weather data, we need to extract relevant information from the JSON response format.
JSON Structure Example
Typically, the API returns data in a JSON format that looks something like this:
{
"coord": {
"lon": -0.13,
"lat": 51.51
},
"weather": [
{
"id": 300,
"main": "Drizzle",
"description": "light intensity drizzle",
"icon": "09d"
}
],
"base": "stations",
"main": {
"temp": 280.32,
"pressure": 1012,
"humidity": 81
},
"name": "London"
}
Sample Code Snippet for JSON Parsing
Using Gson
, we can parse this JSON effortlessly.
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class WeatherUpdater {
// Assuming 'jsonResponse' contains the JSON data received from the API
public static void parseWeatherData(String jsonResponse) {
JsonObject jsonObject = JsonParser.parseString(jsonResponse).getAsJsonObject();
String cityName = jsonObject.get("name").getAsString();
double temperature = jsonObject.get("main").get("temp").getAsDouble();
String weatherDescription = jsonObject.get("weather")
.getAsJsonArray()
.get(0)
.getAsJsonObject()
.get("description").getAsString();
System.out.println("City: " + cityName);
System.out.println("Temperature: " + temperature);
System.out.println("Weather: " + weatherDescription);
}
}
Explanation of the Code
-
Use of
JsonParser
: We create aJsonObject
from the JSON response for easy navigation. -
Extracting Data: By calling
get
, we can easily access values corresponding to specific keys. -
Structured Output: Finally, the data is printed in a structured format, making it easier to visualize.
Continuous Updates and Best Practices
In a real-world application, you’d want to fetch weather data at regular intervals. Consider using a polling mechanism or a scheduled executor service to automate this task.
Utilizing ScheduledExecutorService
Here’s how you can implement periodic updates.
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class WeatherScheduler {
public static void main(String[] args) {
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
Runnable task = () -> {
String weatherData = fetchWeatherData(); // Implement this method
parseWeatherData(weatherData); // Parse the weather data
};
scheduler.scheduleAtFixedRate(task, 0, 10, TimeUnit.MINUTES); // Update every 10 minutes
}
}
Best Practices to Follow
-
API Rate Limits: Always check the API’s rate limits to avoid overloading their servers.
-
Error Handling: Implement robust error handling to manage network issues gracefully.
-
Testing: Unit tests for your methods will ensure reliability and ease future updates.
Key Takeaways
Building a real-time weather application in Java is not only feasible but also a rewarding experience. By leveraging Java's capabilities alongside REST APIs, you can fetch, parse, and display live weather data effortlessly. Just like the challenges discussed in the article "NOAA Weather Radio Woes: Finding the Right Channel" (youvswild.com/blog/noaa-weather-radio-woes-right-channel), ensuring accurate and timely weather updates requires good practices and solid coding skills.
This journey provides a remarkable platform for enhancing your Java skills while contributing to the broader community by developing useful applications. As you continue to master Java, consider branching out into other areas, such as cloud services or microservices, to further enrich your programming arsenal. Happy coding!
Checkout our other articles