Engaging Java Presentations: Tips for Effective Teaching
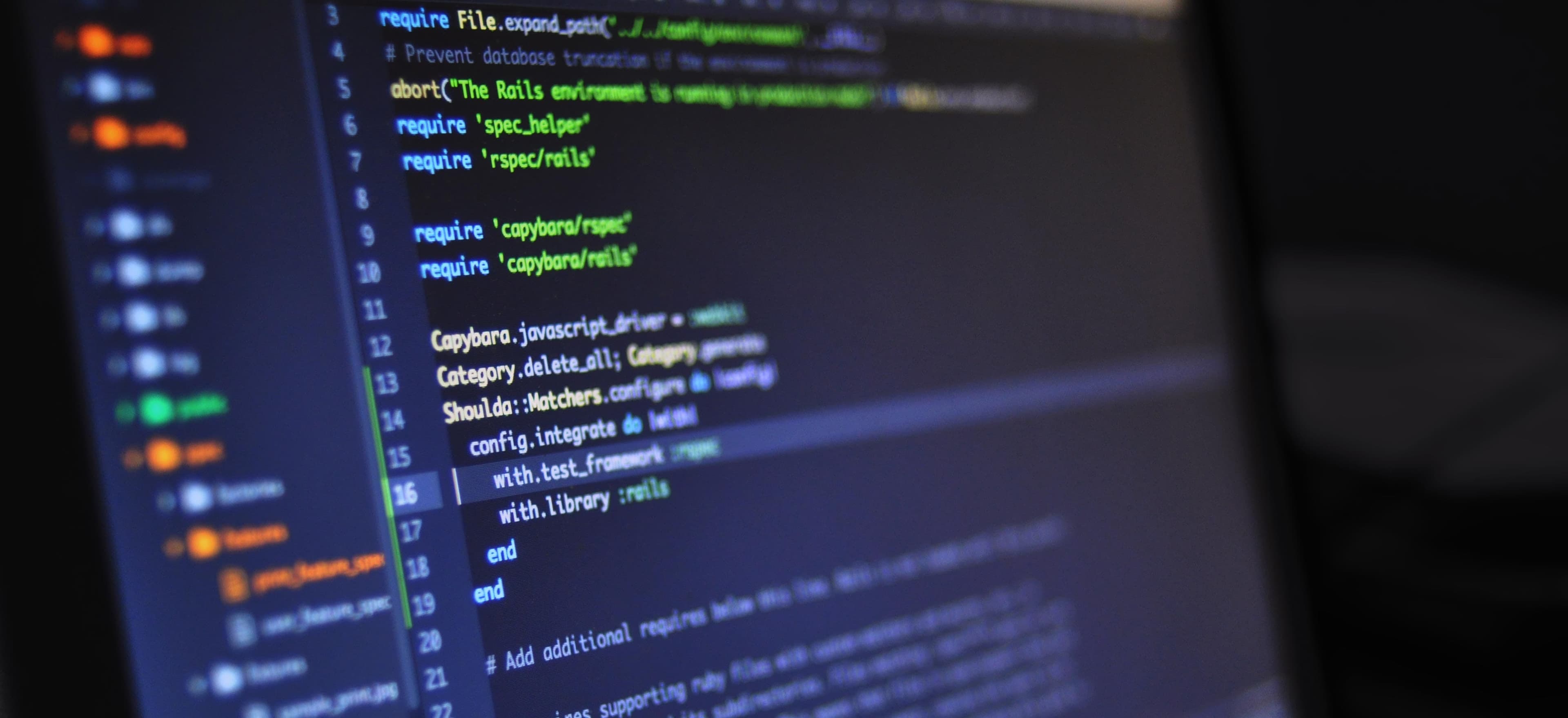
- Published on
Engaging Java Presentations: Tips for Effective Teaching
When it comes to teaching programming languages like Java, an effective presentation can make a world of difference. The key to a successful Java presentation is not just in the content you provide but also in how you engage your audience. This blog post will explore various strategies and techniques to enhance your Java presentations, ensuring that your students grasp complex concepts while remaining attentive and motivated.
Understanding Your Audience
Before diving into presentation techniques, it's crucial to understand your audience. Are they beginners, intermediate, or advanced learners? This knowledge will allow you to tailor your content and complexity accordingly.
For beginners, focus on fundamental concepts and practical examples. If your audience comprises more advanced learners, you can delve into intricate topics such as Java streams, lambda expressions, or concurrency techniques.
Structured Content is Key
A well-structured presentation helps maintain flow and keeps the audience engaged. Here’s a simple format that works well:
- Introduction: Give an overview of what you will cover.
- Core Concepts: Break down the topics into manageable sections.
- Practical Examples: Use code snippets to demonstrate how concepts are applied.
- Conclusion: Summarize key takeaways and open the floor for questions.
Example Structure:
# Engaging Java Presentation Techniques
## 1. Introduction
- Overview of Java and its relevance
- Outline of presentation topics
## 2. Core Concepts
### a. Object-Oriented Programming (OOP)
- Importance of OOP in Java
### b. Java Collections Framework
- Utilizing Lists, Sets, and Maps
## 3. Practical Examples
```java
// Example of creating a simple Java class demonstrating OOP
public class Car {
private String color;
private String model;
// Constructor
public Car(String color, String model) {
this.color = color;
this.model = model;
}
// Method to display car details
public void displayDetails() {
System.out.println("Car Model: " + model + ", Color: " + color);
}
}
// Usage of Car class
public class Main {
public static void main(String[] args) {
Car myCar = new Car("Red", "Toyota");
myCar.displayDetails(); // Outputs: Car Model: Toyota, Color: Red
}
}
- Why: This example introduces OOP by illustrating how to create a class and an object, encouraging students to think in terms of OOP principles.
4. Conclusion
- Recap of what was covered
- Encourage further exploration of Java
## The Power of Visual Aids
Utilizing visual aids can significantly enhance understanding. Diagrams, flowcharts, and slides can break down complex ideas and keep the audience engaged. For instance, when explaining the Java Collections Framework, a visual representation of different data structures can clarify how and when to use them.
### Sample Visual Aid
Here’s a simple diagram that could accompany a presentation on Collections:
+------------------+ | Java Collections | +------------------+ | List | | - ArrayList | | - LinkedList | +------------------+ | Set | | - HashSet | | - TreeSet | +------------------+ | Map | | - HashMap | | - TreeMap | +------------------+
## Interactive Learning
Encouraging interaction during your presentation can greatly enhance retention. Consider incorporating hands-on activities where attendees can practice coding based on what you've discussed. Using platforms like Replit or Jupyter Notebooks enables real-time coding collaboration.
### Sample Activity Idea
1. **Pair Programming**: Divide the audience into pairs and give them simple tasks related to the concepts you've covered. For example:
- Create a list of objects from the Car class.
- Sort them based on a specific attribute (e.g., model).
## Incorporating Real-World Examples
Relating Java concepts to real-world applications can make the learning experience more relatable. When discussing the Java Collections Framework, you could mention how major companies like Google and Amazon use these data structures in their systems.
### Example Code Snippet with Context
```java
// Example of using a HashMap to store user data
import java.util.HashMap;
public class UserDatabase {
private HashMap<String, String> users = new HashMap<>();
public void addUser(String username, String email) {
users.put(username, email);
}
public String getUserEmail(String username) {
return users.get(username);
}
}
- Why: The HashMap here serves as an efficient way to store and retrieve user information, showcasing practical use in real-world applications.
Keeping Engagement High
Another critical factor is to keep the energy up during your presentation. Here are some quick tips:
- Use Humor: A light joke or an amusing anecdote can break the ice.
- Ask Questions: Encourage questions throughout the presentation to foster a dialogue.
- Use Technology: Platforms like Kahoot can facilitate quick quizzes that reinforce learning.
Reference to Existing Content
For those interested in creative presentation techniques beyond Java, consider checking out this article: "10 kreative Ideen für Präsentationen im Deutschunterricht". It provides innovative ideas that can also apply to programming education settings. You can view it here.
Lessons Learned
Creating engaging Java presentations is an art that requires a combination of structured content, visual aids, interactive learning, and real-world applications. By making your presentations relevant and dynamic, you can cultivate a deeper understanding and appreciation for Java among your students. Use the techniques outlined here to elevate your teaching approach and inspire your audience.
Explore the art of teaching Java, and you'll not only impart knowledge but also ignite a passion for programming. Happy teaching!