Choosing Between Java Development and a Design Career Path
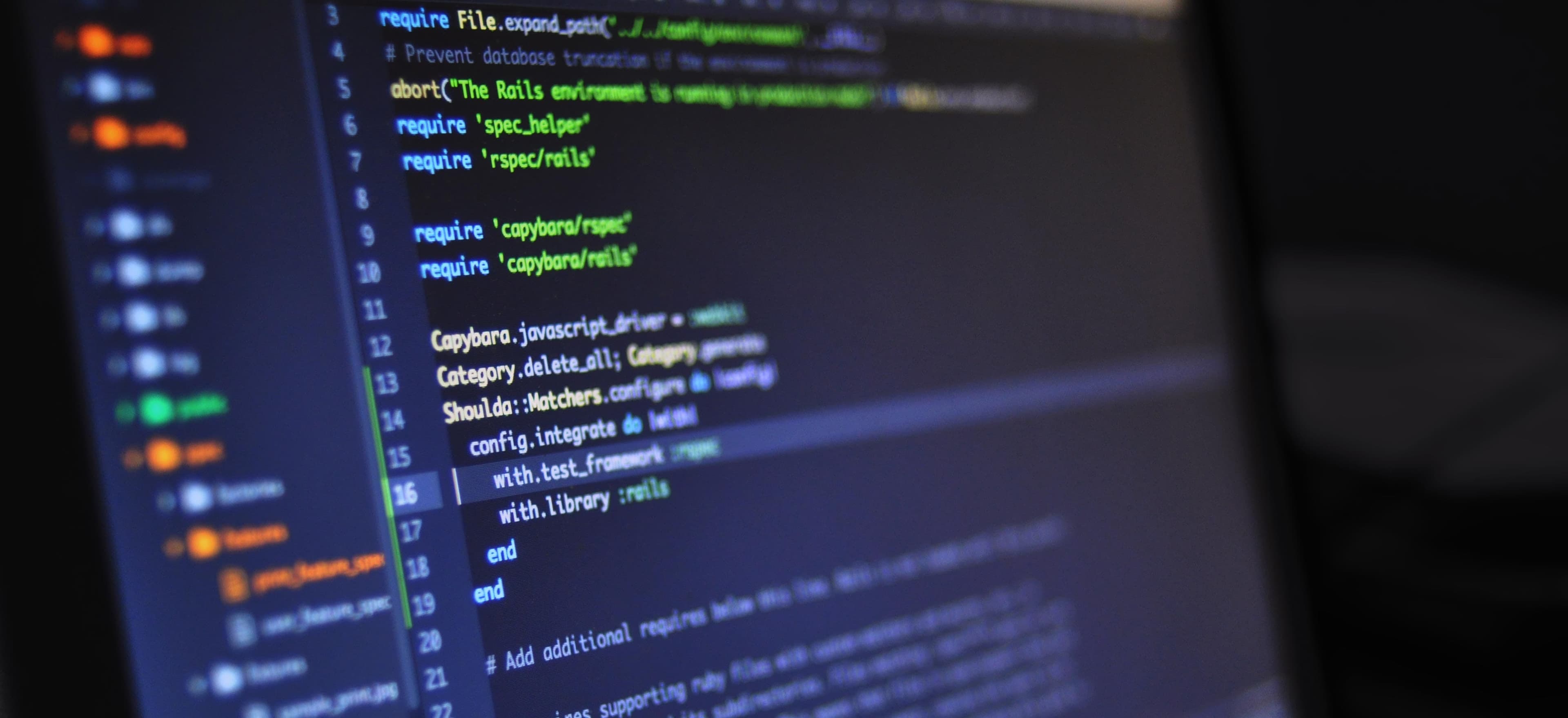
- Published on
Choosing Between Java Development and a Design Career Path
In today's digital landscape, the choice between a career in Java development and one focused on web design can be daunting. Each path offers unique challenges, opportunities, and rewards. While Java development focuses on creating robust, scalable software applications, web design centers on creating visually appealing and user-friendly interfaces. This article aims to dissect these two career paths and help you decide which one aligns better with your interests and goals.
Understanding Java Development
Java is a versatile and powerful programming language widely used in various applications, ranging from mobile apps to large-scale enterprise systems. As a Java developer, you will primarily be involved in writing and maintaining code, understanding algorithms, and solving complex problems.
Why Choose Java Development?
-
Job Demand: There's a strong demand for Java developers, especially in enterprise environments. Many large businesses rely on Java due to its reliability and security features.
-
Performance: Java applications are typically faster and more efficient. As the basis for many server-side applications, knowledge of Java positions you favorably in the job market.
-
Community and Resources: The Java community is vast, boasting countless libraries, frameworks, and resources for learning. This can significantly reduce the time it takes to develop applications.
Key Skills for a Java Developer
To excel as a Java developer, you need to acquire a specific set of skills:
-
Java Frameworks: Familiarity with frameworks like Spring and Hibernate is crucial to streamline the development process.
-
Understanding of OOP: Java is an object-oriented programming language, so grasping the four principles of OOP (encapsulation, inheritance, polymorphism, and abstraction) is essential.
-
Database Knowledge: Knowledge of SQL and how to interact with databases through Java is necessary for full-stack development.
Code Example
Here’s a simple example of a Java program that defines a User class and demonstrates encapsulation:
// User.java
public class User {
// Private fields
private String username;
private String password;
// Constructor
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getter for username
public String getUsername() {
return username;
}
// Method to validate password
public boolean validatePassword(String inputPassword) {
return this.password.equals(inputPassword);
}
}
In this code snippet, we've encapsulated the username
and password
properties within the User
class. This design not only protects the state of the object but also simplifies the maintenance and refactoring of code.
Exploring Web Design
On the other side of the spectrum, web design focuses on the aesthetics and usability of a website or application. This career path is ideal for individuals who have an eye for detail and a passion for creativity. Web designers combine technical skills with artistic flair to create engaging user experiences.
Why Choose Web Design?
-
Creativity: If you have a passion for art and design, a career in web design allows you to express your creativity daily.
-
User Experience Focus: Designers play a crucial role in ensuring that users have a seamless and enjoyable experience, which can significantly impact a business's success.
-
Tools and Technologies: Web design utilizes a range of tools such as Adobe Creative Suite, Figma, and various web design software, allowing for a diverse workflow.
Key Skills for a Web Designer
To stand out as a web designer, consider honing the following skills:
-
Responsive Design: Understanding how to create layouts that work across multiple devices and screen sizes is essential.
-
HTML & CSS Proficiency: Knowledge of HTML and CSS is foundational for any web designer.
-
User Interface (UI) Design: Skills in creating visually appealing and functional user interfaces are key to great web design.
Code Example
Here’s a basic HTML/CSS example showing how to create a simple responsive web layout:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Responsive Layout</title>
<style>
body {
font-family: Arial, sans-serif;
}
.container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.header, .footer {
padding: 20px;
background-color: #4CAF50;
color: white;
text-align: center;
width: 100%;
}
.content {
padding: 20px;
background-color: #f4f4f4;
width: 90%;
max-width: 800px;
}
@media screen and (min-width: 600px) {
.container {
flex-direction: row;
}
.content {
width: 60%;
}
}
</style>
</head>
<body>
<div class="container">
<div class="header">Header</div>
<div class="content">This is the main content area.</div>
<div class="footer">Footer</div>
</div>
</body>
</html>
In this example, we create a responsive layout using CSS Flexbox. This makes the design adaptable to different screen sizes—a necessity in modern web design.
Comparative Insights
When contemplating a career path, you should consider a few critical factors:
-
Passion for Coding vs. Design: Do you find joy in solving problems with code, or do you prefer creating visually appealing designs?
-
Work Environment: Java developers might work in more traditional corporate settings, while web designers may find themselves in creative agencies or freelance environments.
-
Learning Curve: Java development often involves a steeper learning curve due to programming concepts, while web design might be easier to grasp initially but requires ongoing learning about design trends and tools.
Real-World Applications
Both Java developers and web designers are critical to creating great digital experiences. Java powers the backend of applications, ensuring that everything runs smoothly, while web designers create the user interfaces that engage users. Learning both skills can provide a competitive advantage in the job market.
Bridging the Gap
If you're passionate about both areas, there are numerous opportunities to merge these skill sets. Full-stack development, which combines backend and frontend work, is a growing field. This path allows you to leverage the strengths of both Java development and web design.
Resources such as online courses, coding boot camps, and community forums can help you gain expertise in either domain. Websites like Codecademy, freeCodeCamp, and Coursera offer plenty of options for aspiring developers and designers alike.
The Bottom Line
Choosing between Java development and web design ultimately hinges on your interests and career aspirations. Java development is for those who enjoy coding and problem-solving, while web design caters to those with a creative eye and a passion for user experience.
When making your decision, consider your strengths, the skills you wish to develop, and the kind of work environment you hope to be part of. For further insights on career choices in the tech field, you might also want to check out the article titled "Web Dev or Web Design? Unraveling the Career Dilemma" at infinitejs.com/posts/web-dev-vs-web-design-career-dilemma.
Whichever path you choose, both careers present incredible opportunities for growth and success in our increasingly digital world.
Checkout our other articles