Java Strategies: Overcoming Asynchronous Testing Challenges
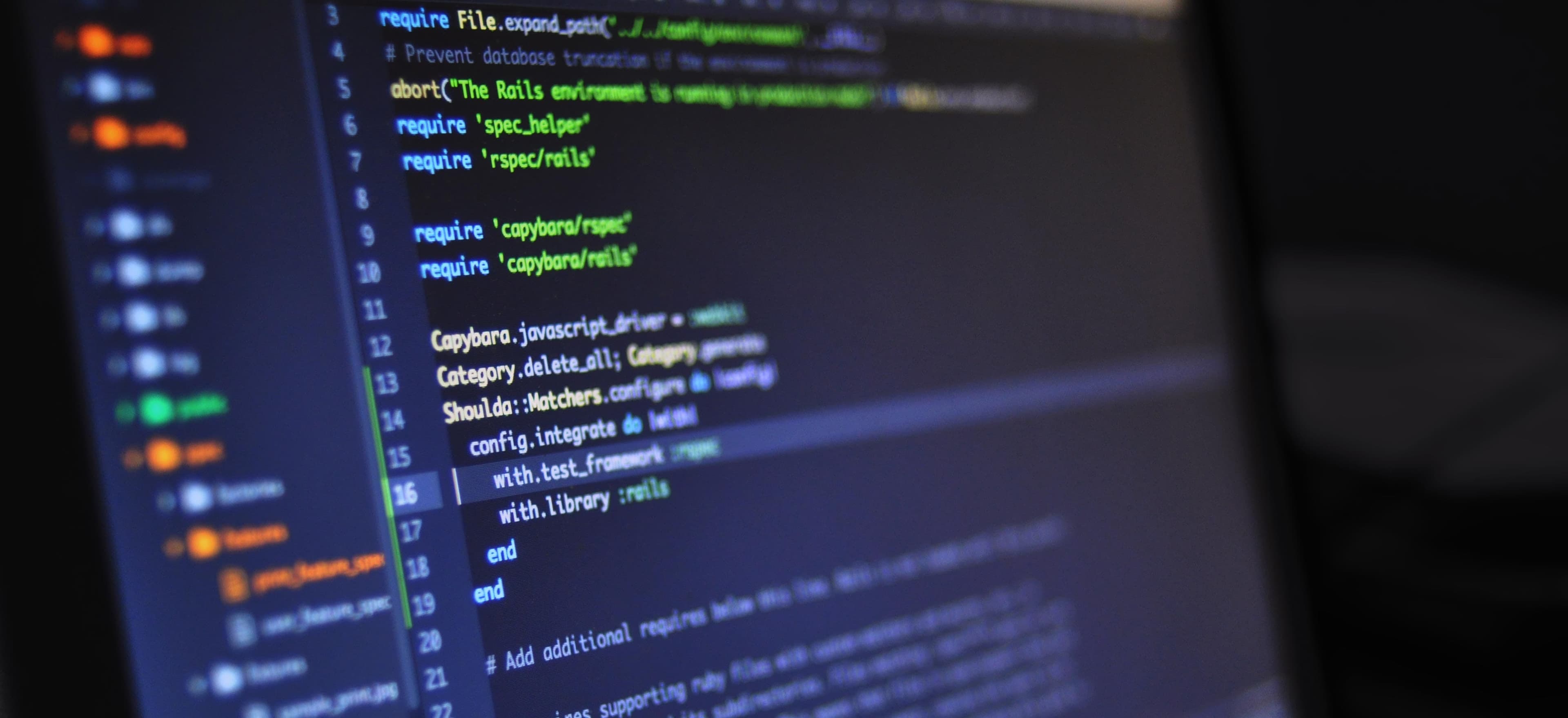
- Published on
Java Strategies: Overcoming Asynchronous Testing Challenges
As software development continues to evolve, so do the strategies required for effective testing. One of the most complex challenges that developers face today is asynchronous testing, especially when dealing with web applications where user interactions and server responses happen at different times. In this blog post, we’ll explore practical Java strategies that can help you successfully navigate these asynchronous testing challenges.
We'll delve deep into various concepts, provide examples, and share best practices that can elevate your testing outcomes.
Understanding Asynchronous Testing
Asynchronous programming allows operations to run in the background, freeing up the main thread to continue executing other tasks. However, when it comes to testing, this can pose several challenges:
- Timing Issues: Tests may complete before asynchronous tasks have fully executed.
- Unpredictable Results: Results can vary depending on the timing of operations, making debugging difficult.
- Complex Dependencies: Asynchronous calls can lead to complicated interdependencies that are hard to replicate in tests.
Considering these factors, it becomes imperative to have robust strategies in place to handle asynchronous testing effectively, particularly in a language like Java.
Using Java Testing Frameworks
Java developers often rely on various testing frameworks such as JUnit and TestNG. These frameworks offer built-in support for assertions and test initialization, which can be extremely useful when writing tests that deal with asynchronous code.
Example: Testing Asynchronous Methods with JUnit
Here, we will create a simple example to demonstrate how you can test an asynchronous method using JUnit.
import org.junit.jupiter.api.Test;
import java.util.concurrent.CompletableFuture;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class AsyncTest {
private CompletableFuture<String> asyncMethod() {
return CompletableFuture.supplyAsync(() -> {
// Simulating a long-running task
try {
Thread.sleep(2000); // Simulate delay
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Hello World!";
});
}
@Test
public void testAsyncMethod() throws Exception {
CompletableFuture<String> future = asyncMethod();
// Wait for the asynchronous task to complete and get the result
String result = future.get();
// Validate the result
assertEquals("Hello World!", result);
}
}
Why This Approach Works
- CompletableFuture: This Java API is designed for handling asynchronous tasks. Using
supplyAsync
, we can quickly run background tasks without blocking the main thread. - Future's get() method: The
get
method blocks until the CompletableFuture completes, effectively synchronizing the rest of your test with the asynchronous code.
This structured manner of testing ensures that your tests reflect true execution times, improving reliability.
Leveraging Mocking Frameworks
When testing complex asynchronous interactions, mocking frameworks such as Mockito can be invaluable. They allow you to simulate the behavior of system components that interact with your asynchronous code without needing to execute the actual implementation.
Example: Using Mockito with Asynchronous Code
Here’s a scenario where you might want to check if an asynchronous task is called correctly within a given service.
import org.junit.jupiter.api.Test;
import org.mockito.Mockito;
import java.util.concurrent.CompletableFuture;
import static org.mockito.Mockito.verify;
class AsyncService {
private Dependency dependency;
public AsyncService(Dependency dependency) {
this.dependency = dependency;
}
public CompletableFuture<String> performAsyncAction() {
return CompletableFuture.supplyAsync(() -> {
return dependency.getData();
});
}
}
class Dependency {
public String getData() {
return "Real Data";
}
}
public class AsyncServiceTest {
@Test
public void testAsyncActionCallsDependency() {
Dependency mockDependency = Mockito.mock(Dependency.class);
AsyncService service = new AsyncService(mockDependency);
service.performAsyncAction();
// Verifying that getData() was called on the mock dependency
verify(mockDependency).getData();
}
}
Why Mocking is Essential
- Isolation: By mocking dependencies, we isolate the code under test, allowing us to focus on the logic and behavior without external interference.
- Behavior Verification: It enables the verification of interactions, ensuring that your code correctly communicates with its dependencies – a crucial aspect of asynchronous code.
Managing Asynchronous Workflows
The complexity of asynchronous testing often comes from multiple asynchronous calls depending on one another. Managing these workflows effectively can be crucial for success.
Using CompletableFutures for Chained Async Calls
Let’s examine how to compose multiple chained asynchronous calls.
import java.util.concurrent.CompletableFuture;
public class AsyncChaining {
public CompletableFuture<Integer> computeFirst() {
return CompletableFuture.supplyAsync(() -> {
// Simulating time-consuming computation
return 20;
});
}
public CompletableFuture<Integer> computeSecond(int value) {
return CompletableFuture.supplyAsync(() -> {
return value * 2;
});
}
public CompletableFuture<Integer> computeFinalResult() {
return computeFirst()
.thenCompose(this::computeSecond);
}
}
The Power of Chaining
- thenCompose: This method allows you to chain asynchronous calls, where the result of one call serves as the input to the next. It ensures results are passed seamlessly from one step to another, making your tests clearly indicative of the workflow.
Testing Chained Async Calls
To validate chained calls, you can write the following test:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class AsyncChainingTest {
@Test
public void testChainedAsyncCalls() throws Exception {
AsyncChaining asyncChaining = new AsyncChaining();
// Invoke the final computation and wait for the result
int result = asyncChaining.computeFinalResult().get();
// Validate the result
assertEquals(40, result);
}
}
Strategies for Robustness
To ensure resilience in your asynchronous tests, consider the following strategies:
- Use Timeouts: Ensure tests time out to prevent indefinite waits.
- Simulate Failures: Mock components to throw exceptions, verifying error paths.
- Test Order Independence: Make your tests independent from one another to prevent hidden dependencies.
- Leverage Awaitility: This library can help to write tests that wait for specific conditions, simplifying asynchronous testing further.
Wrapping Up
Asynchronous testing in Java poses unique challenges that require innovative approaches. By leveraging modern Java features like CompletableFuture
, utilizing mocking frameworks, and employing chaining techniques, you can create reliable tests that accurately reflect the behaviors of your application.
For those seeking to expand beyond Java into the realm of web testing, you may also find value in the existing article titled Mastering Cypress: Tackling Asynchronous Testing Issues. It addresses similar concepts within a different context, emphasizing how to write effective tests for asynchronous code in JavaScript applications.
In conclusion, mastering asynchronous testing in Java is essential for delivering robust applications that meet modern standards. By implementing the strategies outlined in this post, you'll be well-equipped to tackle even the most complex scenarios. Happy testing!
Checkout our other articles