Mastering Number Stripping in Java: A Developer's Guide
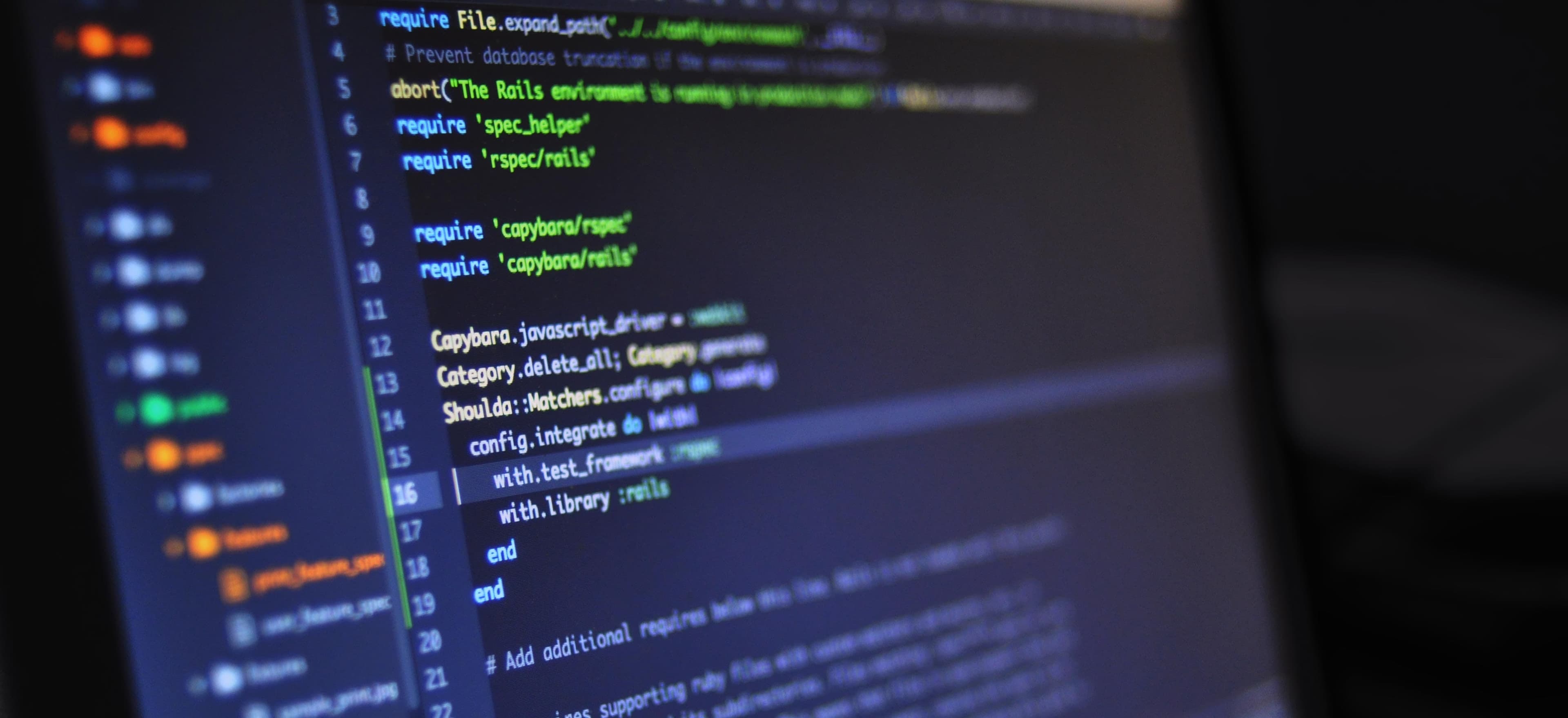
- Published on
Mastering Number Stripping in Java: A Developer's Guide
Java, a robust object-oriented programming language, has numerous applications, one of which involves data manipulation and parsing. In many applications, you may encounter situations where you need to separate numbers from strings or strip them before specific delimiters. Understanding how to effectively manage such tasks is vital in mastering Java.
This guide not only covers the basics of number stripping in Java but also illustrates strategies that elevate your programming skills. If you're looking for additional techniques, check out our previous article, Stripping Numbers Before Delimiters: A How-To Guide.
Understanding the Problem Context
In software development, particularly those dealing with text processing, it is common to find strings with embedded numbers. For instance, consider an input string as follows:
"The temperature is 25 degrees Celsius."
In scenarios like this, you may want either to extract the number (25) or to strip it out, focusing only on the string content. This brings us to the significance of ‘number stripping’. Number stripping refers to the process of identifying and removing numbers from strings based on specific rules or delimiters.
Delimiters in String Processing
Delimiters are characters or sequences of characters that provide boundaries within the strings. They signify where data should be separated for processing. In many cases, these delimiters could be spaces, commas, or even special characters. The task might involve removing or manipulating data relative to these delimiters.
Setting Up Your Java Environment
Before we delve into the code, ensure your Java development environment is set up. Use an IDE like IntelliJ IDEA or Eclipse, or code in a simple text editor. If you've not already installed Java Development Kit (JDK), download and install it here.
Maven Initialization
To manage dependencies more easily, we can utilize Maven for our Java project. Start by creating a new Maven project. Your pom.xml
file might look something like this:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>NumberStripping</artifactId>
<version>1.0-SNAPSHOT</version>
</project>
Writing the Code for Number Stripping
'Number stripping' isn't a one-size-fits-all solution. Depending on your requirements, you may employ different techniques. Below, we discuss a basic approach—using regex (regular expressions)—to extract numbers from strings.
Using Regular Expressions
Regular expressions (regex) are sequences of characters that form a search pattern. They are extremely powerful for string manipulation tasks. In the context of number stripping, regex can identify patterns associated with digits.
Here’s a simple code snippet demonstrating how to use regex to extract numbers from a string:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class NumberStripping {
public static void main(String[] args) {
String input = "The price is 45 dollars and 30 cents.";
System.out.println("Extracted numbers: " + extractNumbers(input));
}
public static String extractNumbers(String input) {
String regex = "\\d+"; // Pattern to match one or more digits
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(input);
StringBuilder numbers = new StringBuilder();
while (matcher.find()) {
numbers.append(matcher.group()).append(" "); // Append each found number with a space
}
return numbers.toString().trim(); // Return extracted numbers as a trimmed string
}
}
What This Code Does
-
Pattern Compilation: The regex
\\d+
matches sequences of one or more digits. This is compiled into a Pattern object. -
Matcher Creation: The Matcher object is created to find occurrences of the regex pattern in the input string.
-
Loop Through Matches: As we utilize a while loop, we check if any more digits are found, appending them to the
numbers
StringBuilder. -
Output: Finally, the extracted numbers are returned as a single space-separated String.
Why Use Regex?
Using regex is efficient as it allows complex pattern matching with minimal code. In most scenarios where number stripping is required, regex can prove to be faster than manually iterating over each character and checking if it is a number.
Stripping Numbers Before Delimiters
Sometimes, you might need to strip numbers before specific delimiters rather than extracting them. Let's enhance our previous example to remove numbers from a string before a specified delimiter.
public static String stripNumbersBeforeDelimiter(String input, String delimiter) {
String regex = "\\d+(?=" + Pattern.quote(delimiter) + ")"; // Matches digits before the given delimiter
return input.replaceAll(regex, ""); // Replace found numbers with an empty string
}
public static void main(String[] args) {
String input = "The price is 45 dollars, and the discount is 10% off.";
String result = stripNumbersBeforeDelimiter(input, "dollars");
System.out.println("Stripped string: " + result);
}
Breakdown of the Above Code
-
Positive Lookahead: The regex
\\d+(?=dollars)
utilizes a lookahead assertion. It matches digits that are followed by "dollars". -
String Replacement: With the
replaceAll
method, we replace all occurrences of this regex with an empty string, effectively stripping the numbers while retaining the rest of the content.
Why It's Important
By understanding how to strip numbers based on delimiters, you can create more sophisticated string-processing applications. This technique is valuable not only in parsing but also in data cleansing and validation scenarios.
Advanced Techniques: Handling Edge Cases
Multiple Delimiters
In practice, you may have multiple delimiters. One could utilize Java’s StringTokenizer
or simply modify regex to accommodate various delimiters. For example:
public static String stripNumbersBeforeMultipleDelimiters(String input) {
String regex = "\\d+(?=[,;:.])"; // Match digits before any of these delimiters
return input.replaceAll(regex, ""); // Replace found numbers with an empty string
}
Performance Considerations
When dealing with large datasets or complex strings, performance is crucial. Consider these factors:
- Optimize regex patterns to reduce backtracking.
- Profile your application to detect bottlenecks in string manipulation.
The Last Word
Mastering number stripping in Java involves a blend of regex skills and a strong grasp of string manipulation techniques. The examples discussed provide a solid foundation, whether you're extracting numbers or removing them based on delimiters.
For further reading and a deeper dive into the intricacies of string processing, revisit our article, Stripping Numbers Before Delimiters: A How-To Guide.
As you grow more comfortable with these techniques, you'll find your ability to handle real-world data processing tasks will enhance significantly. Happy coding!
Checkout our other articles