Tackling API Challenges in Java with Storyblok Integration
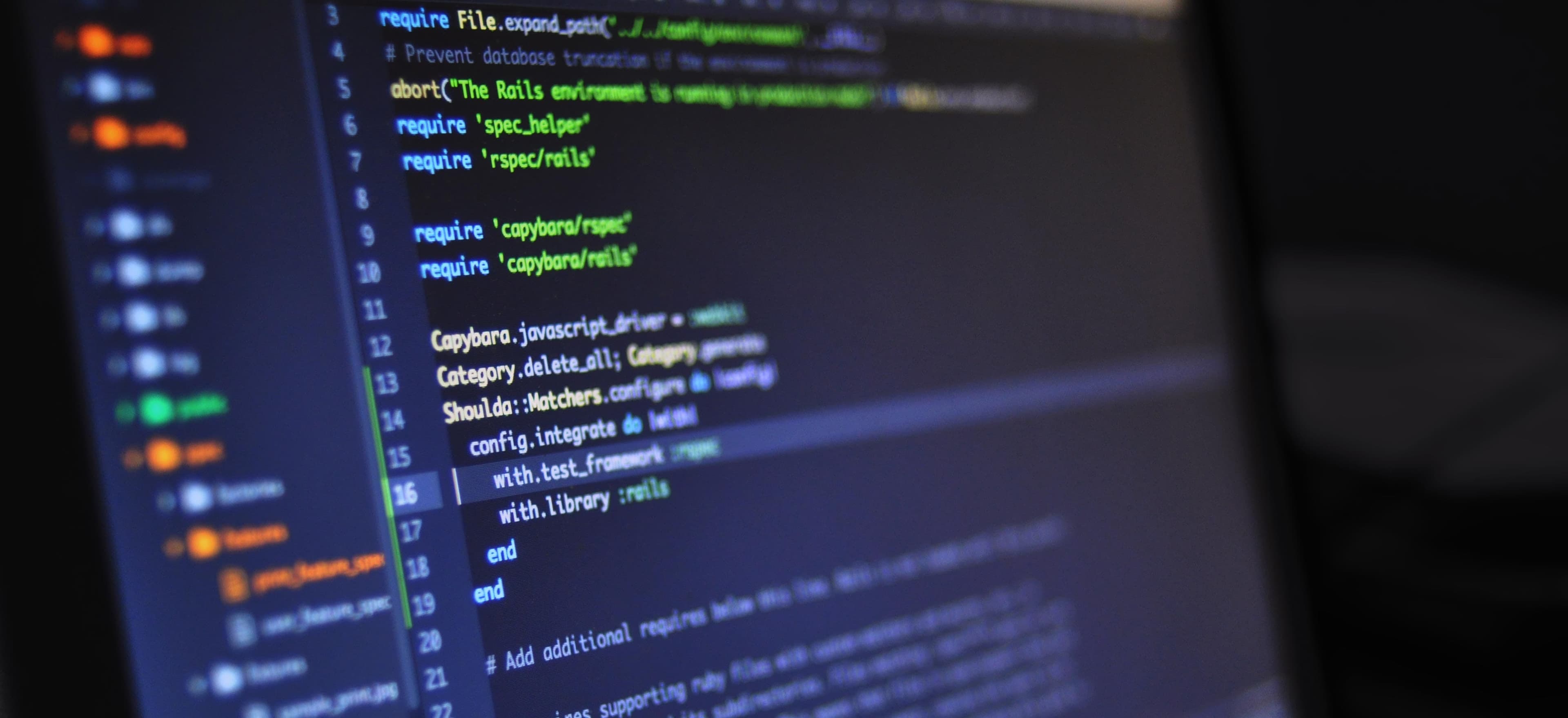
- Published on
Tackling API Challenges in Java with Storyblok Integration
Java has long been a favorite programming language among developers for its versatility and robustness. However, when it comes to integrating with APIs, challenges can arise, especially when working with Headless CMS like Storyblok. In this post, we will explore ways to effectively tackle such API challenges in Java, using Storyblok as an example.
Understanding Headless CMS and Storyblok
A Headless Content Management System (CMS) allows you to manage your content separately from your presentation layer. Storyblok is a popular Headless CMS that provides a powerful API for managing content dynamically. By using Storyblok, developers can create flexible architecture, decoupled from the front-end framework.
Why Integrate with Storyblok?
Using Storyblok allows developers to:
- Have full control over the presentation layer without being locked into any specific technology.
- Deliver dynamic content to various platforms including web, mobile, and IoT devices.
- Utilize a visual editor to manage content, which enhances productivity.
However, integrating Storyblok with Java can present some challenges. In this article, we will explore common obstacles and how to effectively navigate them.
Setting Up Your Java Project
Dependencies and Libraries
Before diving into API integration, ensure your Java environment is properly set up. You will need to include relevant libraries in your Maven pom.xml
or Gradle build file to work with HTTP requests.
For Maven, add the following dependencies:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version>
</dependency>
Here, we are using Apache HttpClient for handling HTTP requests, and Gson for parsing JSON data.
Making API Calls to Storyblok
The first step in integrating Java with Storyblok is to make API calls to fetch data. Below is an example of how to do this:
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import com.google.gson.Gson;
public class StoryblokAPI {
private static final String SPACE_ID = "your_space_id";
private static final String API_KEY = "your_api_key";
private static final String API_URL = "https://api.storyblok.com/v1/cdn/stories/";
public static void main(String[] args) {
String storyId = "your_story_id";
String response = getStoryblokData(storyId);
System.out.println(response);
}
public static String getStoryblokData(String storyId) {
String url = API_URL + storyId + "?token=" + API_KEY;
String jsonResponse = "";
try (CloseableHttpClient client = HttpClients.createDefault()) {
HttpGet request = new HttpGet(url);
try (CloseableHttpResponse response = client.execute(request)) {
jsonResponse = new Gson().toJson(response.getEntity().getContent());
}
} catch (Exception e) {
e.printStackTrace();
}
return jsonResponse;
}
}
Explanation
In this code, we defined a method getStoryblokData
, which constructs the API URL along with your space ID and API key (make sure to replace placeholders with actual values). The method makes a GET request to fetch a specific story using its ID and returns the JSON response.
Why Use Apache HttpClient?
Apache HttpClient is a popular and reliable library to make HTTP requests. Its flexible API makes it easy to handle different types of requests and responses, which is essential when working with APIs.
Handling Responses and Errors
While fetching data, it’s equally important to manage responses effectively. Below is an updated version of our earlier example that includes error handling.
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.entity.ContentType;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import com.google.gson.Gson;
public class StoryblokAPI {
// ... Previous code remains the same ...
public static String getStoryblokData(String storyId) {
String url = API_URL + storyId + "?token=" + API_KEY;
String jsonResponse = "";
try (CloseableHttpClient client = HttpClients.createDefault()) {
HttpGet request = new HttpGet(url);
try (CloseableHttpResponse response = client.execute(request)) {
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == 200) {
HttpEntity entity = response.getEntity();
jsonResponse = EntityUtils.toString(entity, ContentType.getOrDefault(entity).getCharset());
} else {
jsonResponse = "Error: " + statusCode;
}
}
} catch (Exception e) {
jsonResponse = "Error: " + e.getMessage();
}
return jsonResponse;
}
}
Explanation
In this version, the code checks the HTTP status code of the response. If it is not 200, it returns an error status. This practice is crucial for debugging and handling potential issues when dealing with an external API.
Final Considerations: The Power of Integration
Integrating Java with Storyblok can be smooth if you understand not just the mechanics of making API calls, but also how JSON responses work. The strategies mentioned above, paired with effective error handling, set a strong foundation for building flexible and dynamic applications.
For those who might still be struggling with integrating Storyblok, I recommend checking out the article titled "Struggling with Eleventy and Storyblok Integration?" which can provide more insights into integrating this powerful Headless CMS effectively. You can find it here.
By mastering API interactions, you unlock a world of possibilities, making your applications scalable and user-driven. Happy coding!
Further Reading
To continue improving your skills in Java and API integrations, consider the following resources:
With practice and a solid understanding of these concepts, you can ensure a smooth integration experience with various APIs in your Java applications.
Checkout our other articles