Are Your Tests Clean Enough? The Verification Dilemma
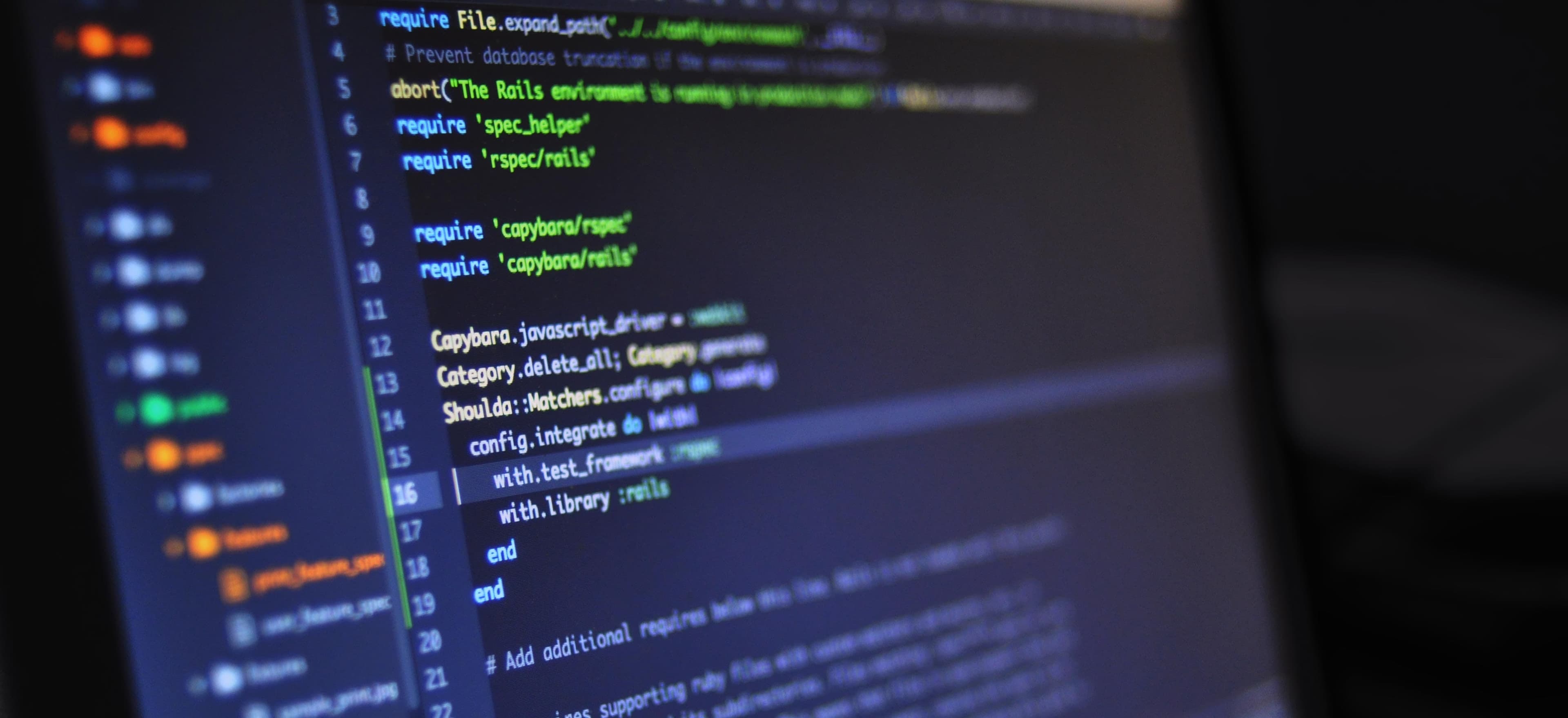
- Published on
Are Your Tests Clean Enough? The Verification Dilemma
In the world of software development, the importance of clean code extends far beyond writing functional algorithms. Just as clear communication is vital in human interactions, clean tests are essential during the software verification process. In this blog post, we'll explore the reasons why clean tests matter, how to maintain their clarity, and some practical strategies to enhance the quality of your testing suite.
Understanding Clean Tests
Clean tests are a hallmark of maintainable software. They are easy to read, concise, and effectively communicate their purpose. When tests are clean, it becomes easier for teams to understand the code's intent, facilitating the identification of bugs and issues during development.
Why Clean Tests Matter
-
Maintainability: Clean tests can be easily modified as code changes evolve. They allow developers to isolate problems faster.
-
Collaboration: In teams, clear tests enable developers to work together more effectively. They serve as documentation that helps teammates understand the code.
-
Debugging: When tests clearly convey their intentions, pinpointing the source of a failure becomes more straightforward.
-
Business Value: The quicker you can detect and fix issues, the more business value you deliver.
Principles of Clean Tests
Before diving into examples, let’s explore some fundamental principles of clean tests.
1. Descriptive Naming
Just as functional methods need descriptive names, test methods should convey exactly what they are testing. A good test name describes both the behavior and the expected outcome.
Example:
@Test
public void shouldReturnSumWhenAddingTwoNumbers() {
// Code for test here...
}
In the above example, the method name tells you not only the method's purpose but also the expected behavior when two numbers are added.
Refer to JUnit Naming Conventions for further insights on how to name your tests correctly.
2. Keep Tests Isolated
Tests should not depend on each other. Each test should be able to run independently. This prevents cascading failures, which can make debugging a nightmare.
Example:
@Test
public void shouldReturnFalseForInvalidInput() {
Validator validator = new Validator();
boolean result = validator.isValid("invalid input");
assertFalse(result);
}
@Test
public void shouldReturnTrueForValidInput() {
Validator validator = new Validator();
boolean result = validator.isValid("valid input");
assertTrue(result);
}
In this example, the tests for the Validator
class can be run separately. Therefore, if one test fails, it will not impact the outcome of the other.
3. Use Assertions Wisely
Assertions are the bedrock of unit tests. They should clearly express the condition that is expected to be true at a specific point in the code.
Example:
@Test
public void shouldThrowExceptionWhenInputIsNull() {
Exception exception = assertThrows(IllegalArgumentException.class, () -> {
new Calculator().divide(10, null);
});
assertEquals("Input cannot be null", exception.getMessage());
}
Using assertThrows
not only checks for exceptions but also asserts that the error message is what you expect. This makes your tests more readable and informative.
Strategies to Maintain Clean Tests
Here are some strategies to ensure that your tests remain clean over time.
1. Refactoring Tests Regularly
Just as production code should be refactored to improve its structure and readability, so should your test code. Regularly revisiting your tests encourages clarity and maintainability.
2. Avoid Duplicate Code
Duplicate code in tests can lead to confusion and makes maintenance harder. If the same setup appears in multiple tests, consider using helper methods or test fixtures to DRY (Don't Repeat Yourself) them up.
Example:
private Validator validator;
@BeforeEach
public void setUp() {
validator = new Validator();
}
@Test
public void testCaseOne() {
assertTrue(validator.isValid("input1"));
}
@Test
public void testCaseTwo() {
assertTrue(validator.isValid("input2"));
}
In this setup, we initialize the Validator
once and use it in multiple test cases instead of creating a new instance repeatedly.
3. Adopt Behavior-Driven Development (BDD)
Using BDD tools, like Cucumber, allows for writing tests in a human-readable format. This approach can bridge the gap between technical and non-technical team members.
4. Review and Pair Programming
Conduct regular code reviews of your tests. These reviews can highlight areas for improvement and ensure adherence to best practices. Pair programming can also support this process.
In Conclusion, Here is What Matters
Clean tests are not just a developer’s wish list; they are essential components of a healthy software development lifecycle. By following these principles and strategies, your tests can provide assurance of quality, maintainability, and business value.
As the saying goes, “A chain is only as strong as its weakest link.” In software development, your tests can serve as one of the strongest links in maintaining functionality and achieving scalability.
If you want to dive deeper into the topic of testing in Java, resources like The Art of Unit Testing by Roy Osherove are invaluable. Emphasizing best practices, this resource can help you take your tests to the next level.
Remember, continuous improvement is key. Always strive to make your tests cleaner, and your code will thank you!