Boosting Performance: Optimize Your Spring Data JPA Queries
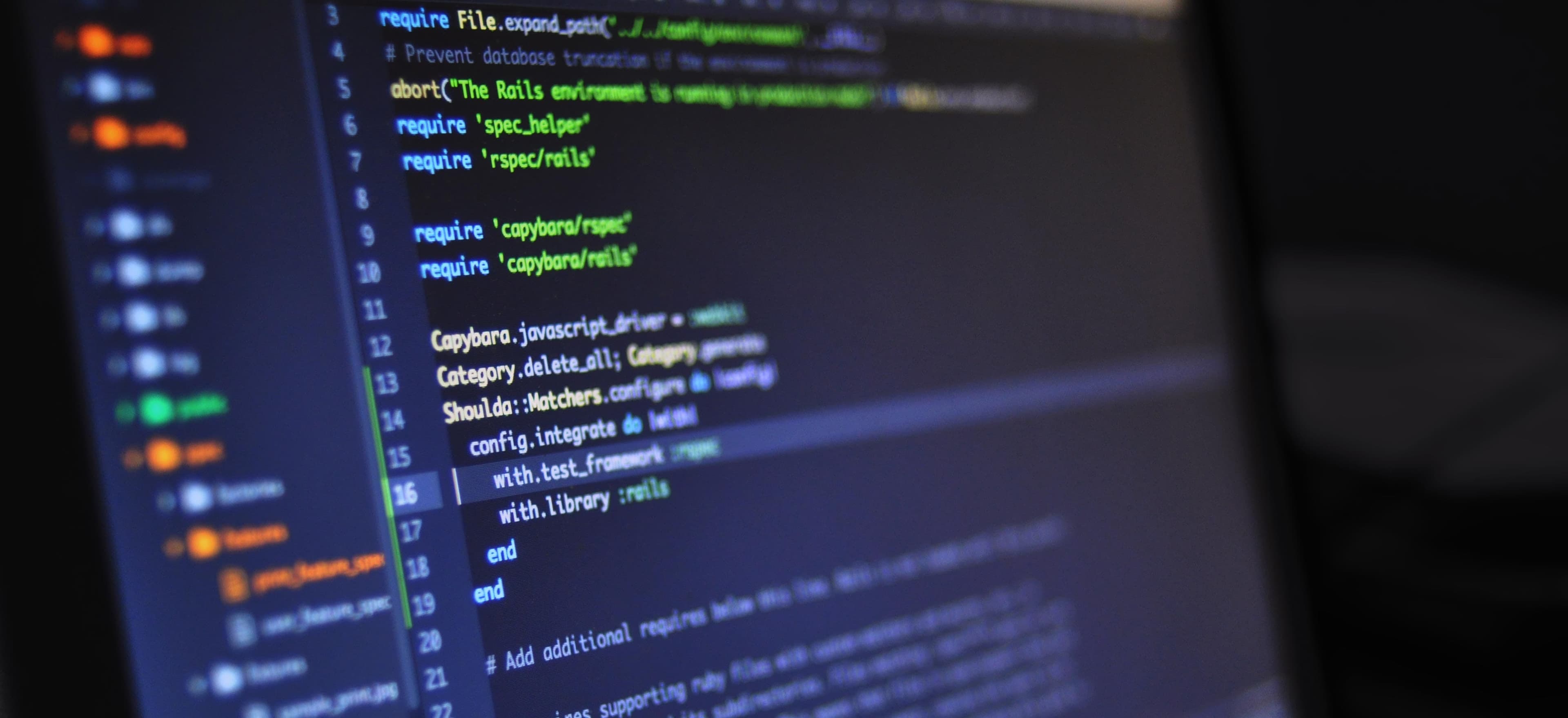
- Published on
Boosting Performance: Optimize Your Spring Data JPA Queries
As web applications evolve, handling data efficiently becomes paramount. When using Spring Data JPA for database interactions, it's essential to ensure your queries are optimized to boost performance. This blog post delves into practical strategies you can use to optimize your Spring Data JPA queries, elevating your application's performance while maintaining code clarity.
Understanding Spring Data JPA
At its core, Spring Data JPA simplifies database access through the use of repositories and entity models. While this abstraction simplifies coding, it can also inadvertently lead to performance pitfalls if not handled properly.
Common Pitfalls in Spring Data JPA
Here are a few common pitfalls developers encounter:
- The N+1 Select Problem: This is an issue caused by loading an entity and then loading its associated entities in a separate query for each entity.
- Fetching Unnecessary Data: Sometimes, you might be fetching more columns than you need, making your queries slower.
- Lack of Pagination: Loading large datasets without pagination can cause delays and lead to poor user experiences.
Now, let's explore ways to address these challenges.
Optimize Your Queries
1. Use Projections
Projections allow you to fetch only the fields you need from the database. This minimizes the amount of data transferred, leading to faster queries.
public interface UserProjection {
String getUsername();
String getEmail();
}
public interface UserRepository extends JpaRepository<User, Long> {
List<UserProjection> findByAgeGreaterThan(int age);
}
In this example, instead of fetching the entire User
entity, we only fetch the username
and email
fields. This is particularly useful when your entities have many attributes but you only need a subset.
2. Leverage Fetch Joins
To combat the N+1 problem effectively, use fetch joins. This retrieves related entities in one query rather than multiple.
@Query("SELECT u FROM User u JOIN FETCH u.posts WHERE u.age > :age")
List<User> findUsersWithPosts(@Param("age") int age);
With JOIN FETCH
, you're instructing JPA to load both User
and its associated posts
in a single call, enhancing efficiency.
3. Implement Pagination and Sorting
Handling large datasets without pagination can lead to performance issues. Use Spring Data’s built-in support for pagination to slice your data into manageable chunks.
Page<User> findAll(Pageable pageable);
You can then call this method with a PageRequest
, which allows you to specify the page number and size:
PageRequest pageRequest = PageRequest.of(0, 10); // Fetching the first 10 records
Page<User> userPage = userRepository.findAll(pageRequest);
This not only optimizes the query performance by reducing the data load but also improves user experience.
4. Avoid FetchType.EAGER
While EAGER loading may seem convenient, it's often inefficient. By default, related entities are fetched immediately with the parent entity, which can pull more data than necessary.
Opt for FetchType.LAZY
when defining relationships:
@Entity
public class User {
@OneToMany(fetch = FetchType.LAZY)
private Set<Post> posts;
}
With LAZY loading, you only fetch the related entities when they're actually needed. This drastically reduces the amount of data fetched upfront.
Use Query Hints
Spring Data JPA allows developers to pass hints to queries, which can help optimize performance in specific scenarios:
@QueryHints({
@QueryHint(name = "org.hibernate.cacheable", value = "true"),
@QueryHint(name = "org.hibernate.comment", value = "Optimizing User Query")
})
List<User> findActiveUsers();
Here, the @QueryHints
annotation improves performance by enabling second-level caching.
Batch Processing
If your application needs to perform bulk operations, consider batch processing to minimize the number of database calls.
@Transactional
public void saveAllUsers(List<User> users) {
int batchSize = 50;
for (int i = 0; i < users.size(); i++) {
userRepository.save(users.get(i));
if (i % batchSize == 0 && i > 0) {
userRepository.flush(); // Flush and clear to minimize memory usage
}
}
}
Batch processing reduces the overhead of multiple calls to the database, thus enhancing performance.
Consider QueryDSL or Specifications
For dynamic and complex queries, consider using QueryDSL or Specifications in Spring Data JPA. This approach provides a more flexible way to construct queries based on varying input parameters.
public List<User> findUsersByCriteria(UserCriteria criteria) {
JPAQuery<User> query = new JPAQuery<>(entityManager);
QUser user = QUser.user;
BooleanBuilder builder = new BooleanBuilder();
if (criteria.getUsername() != null) {
builder.and(user.username.eq(criteria.getUsername()));
}
if (criteria.getAge() != null) {
builder.and(user.age.goe(criteria.getAge()));
}
return query.select(user).from(user).where(builder).fetch();
}
Here, the BooleanBuilder
allows for dynamic query construction, making your data fetching rules adaptable to different scenarios.
A Final Look
Optimizing your Spring Data JPA queries is a multilayered process. It involves understanding the underlying pitfalls like the N+1 issue, judicious use of fetch types, implementing pagination, and employing projections effectively.
By utilizing strategies like batch processing, query hints, and leveraging tools such as QueryDSL, you can significantly enhance your application performance and provide a smoother user experience.
For more in-depth reading, check Spring Data JPA Reference and Optimizing Your JPA Queries.
By taking the time to optimize your queries, you'll not only improve performance but also maintain cleaner and more maintainable code. Happy coding!
Checkout our other articles