Mastering Max and Min Date Calculations with Java Streams
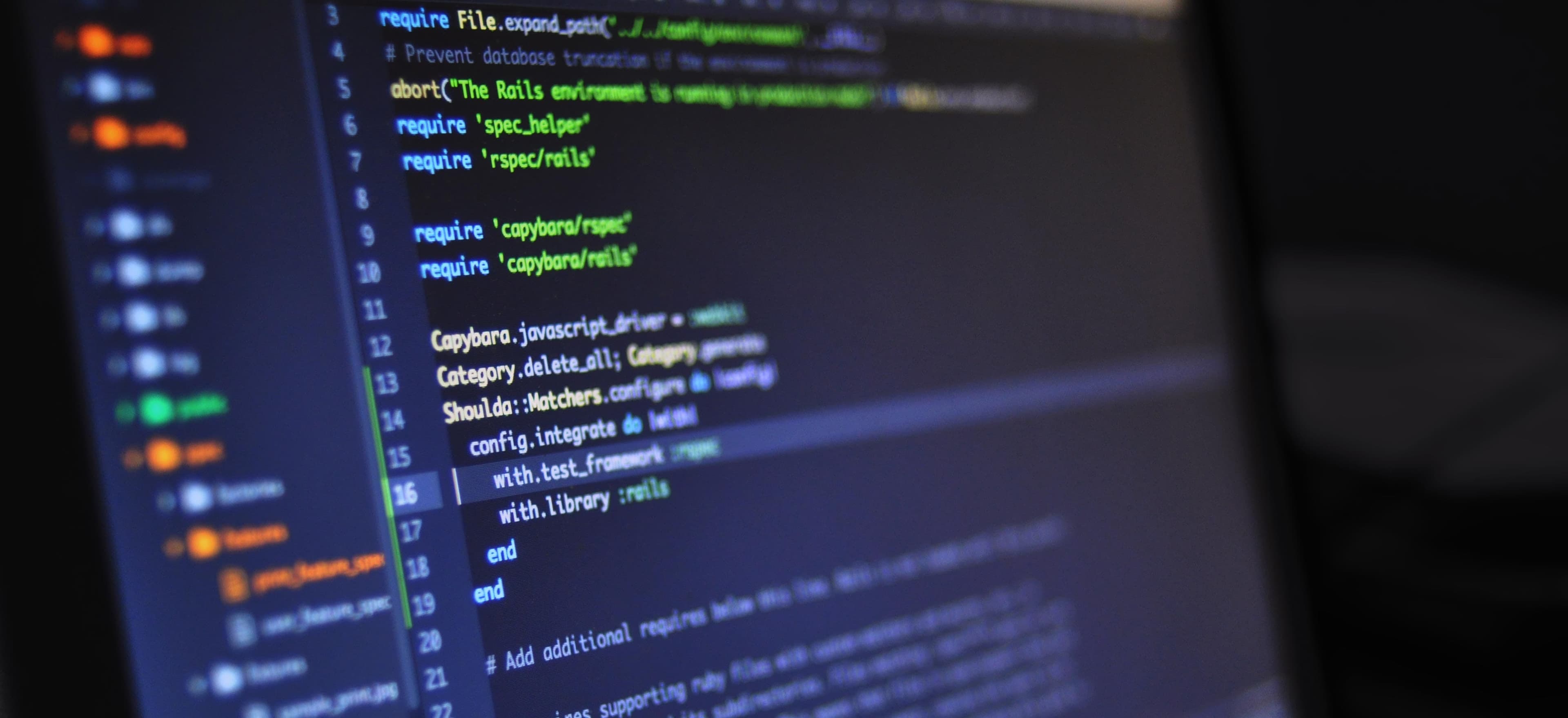
- Published on
Mastering Max and Min Date Calculations with Java Streams
In today's programming landscape, working with date and time is fundamental. Whether you're building an application to manage events, logging, or scheduling tasks, having the ability to find the maximum and minimum dates quickly and efficiently is crucial. Java Streams provide an elegant and powerful way to process sequences of elements, and they can greatly simplify date manipulations.
In this blog post, we will learn how to utilize Java Streams to compute the maximum and minimum dates from a list. We'll cover relevant use cases, discuss important concepts, and provide exemplary code snippets with explanations.
Understanding Java Streams
Java Streams, introduced in Java 8, facilitate functional-style operations on collections. They enable you to process data in a declarative way, making your code cleaner and more maintainable. The key advantages of using streams include:
- Less Boilerplate: Streams reduce the need for traditional loops.
- Lazy Evaluation: Streams are processed only when needed.
- Pipeline Processing: You can combine multiple operations into a single pipeline.
Example Use Case
Imagine you have a list of events, each associated with a date. Your task is to find the latest and earliest event dates. Instead of writing extensive loops, we can leverage the expressive power of Java Streams.
Setting Up the Environment
To follow along, ensure you have a Java Development Kit (JDK) installed. For this example, we will use Java 11 or later. You'll also want to use any IDE of your choice, such as IntelliJ IDEA or Eclipse.
Maven Dependency
If you're using Maven, make sure you have the following dependency in your pom.xml
:
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<scope>compile</scope>
</dependency>
Lombok is optional but can help with reducing boilerplate code in Java.
Creating the Event Class
Let's start with a simple Event
class that contains a name and a date.
import java.time.LocalDate;
public class Event {
private String name;
private LocalDate date;
public Event(String name, LocalDate date) {
this.name = name;
this.date = date;
}
public LocalDate getDate() {
return date;
}
public String getName() {
return name;
}
}
Why This Structure?
By encapsulating the properties of our event into a class, we can easily extend it in the future. We are ensuring our data is well-organized, making it easier to manage.
Creating a List of Events
Next, let's create a list of events with varying dates.
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
public class EventUtils {
public static List<Event> createSampleEvents() {
List<Event> events = new ArrayList<>();
events.add(new Event("Conference", LocalDate.of(2023, 5, 23)));
events.add(new Event("Meeting", LocalDate.of(2023, 3, 17)));
events.add(new Event("Workshop", LocalDate.of(2023, 8, 13)));
events.add(new Event("Seminar", LocalDate.of(2023, 1, 10)));
return events;
}
}
Explanation of the Sample Events
Here, we established a straightforward data structure that represents events occurring at different times. This will allow us to effectively apply stream operations to find maximum and minimum dates.
Finding the Latest and Earliest Dates
Now, let's get into the core functionality. We will utilize the Stream API to find the latest and earliest events.
Finding the Maximum Date
The following code demonstrates how to use streams to find the maximum date.
import java.util.Comparator;
public class EventProcessor {
public static void main(String[] args) {
List<Event> events = EventUtils.createSampleEvents();
LocalDate maxDate = events.stream()
.map(Event::getDate) // Extract the dates from events.
.max(Comparator.naturalOrder()) // Find the maximum date.
.orElse(null); // Handle the case where there are no events.
System.out.println("Latest Event Date: " + maxDate);
}
}
Explanation
- Stream Creation:
events.stream()
creates a stream from the events list. - Mapping:
map(Event::getDate)
transforms the stream of events into a stream of dates. - Finding Maximum:
max(Comparator.naturalOrder())
determines the maximum date. - Handling Absence of Elements:
orElse(null)
returnsnull
if the list is empty.
Finding the Minimum Date
You can easily find the minimum date by following similar steps:
public class EventProcessor {
public static void main(String[] args) {
List<Event> events = EventUtils.createSampleEvents();
LocalDate minDate = events.stream()
.map(Event::getDate) // Extract the dates from events.
.min(Comparator.naturalOrder()) // Find the minimum date.
.orElse(null); // Handle the case where there are no events.
System.out.println("Earliest Event Date: " + minDate);
}
}
Explanation
The logic mirrors the maximum date calculation. We instead call the min
method, which effectively retrieves the earliest date from the list of events.
Combining Maximum and Minimum in One Go
To further improve efficiency, we can calculate both the maximum and minimum dates in a single stream operation. Here’s how:
import java.util.Optional;
public class EventProcessor {
public static void main(String[] args) {
List<Event> events = EventUtils.createSampleEvents();
Optional<LocalDate> maxDate = events.stream()
.map(Event::getDate)
.max(Comparator.naturalOrder());
Optional<LocalDate> minDate = events.stream()
.map(Event::getDate)
.min(Comparator.naturalOrder());
System.out.println("Latest Event Date: " + maxDate.orElse(null));
System.out.println("Earliest Event Date: " + minDate.orElse(null));
}
}
Explanation
In this example, we conduct two separate stream operations for maximum and minimum date calculations. This may be inefficient if the list of events becomes significantly large. Angular improvements may be required here for performance, depending on the use case.
Best Practices and Further Reading
- Immutability: Prefer immutable data structures where possible.
- Stream Limitation: Always consider the size of the data being processed for scalability.
- Parallel Streams: For large datasets, consider using parallel streams to enhance performance.
For more on Java Streams, check out the Java Documentation on Streams.
In Conclusion, Here is What Matters
In this blog post, we mastered the methodology for calculating maximum and minimum dates in Java using the Stream API. By leveraging streams, our code became not only more readable but also more efficient.
As you continue working with dates in Java, remember to exploit the powerful features Java Streams offer. They can streamline your code and enhance overall productivity.
Feel free to share your thoughts in the comments below or reach out for any clarifications. Happy coding!
This post hits the sweet spot between technical depth and clarity, seamlessly guiding you through Java Streams and date calculations. I hope it serves you well in your journey of mastering Java!
Checkout our other articles