Solving Cache Invalidation Issues with Memcached in Spring
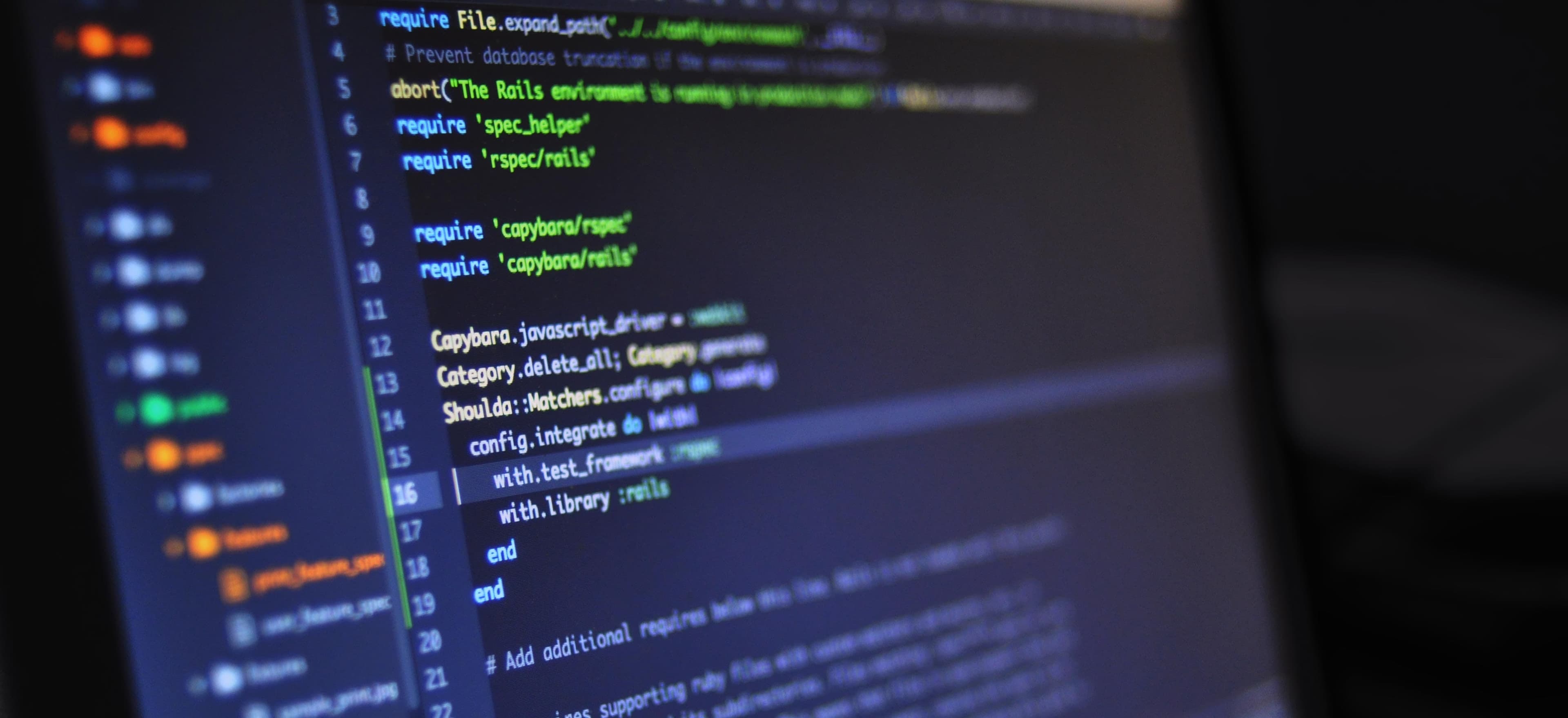
- Published on
Solving Cache Invalidation Issues with Memcached in Spring
Caching is crucial for enhancing application performance, especially when working with high-volume data access. However, cache invalidation can pose various challenges, particularly when data updates occur frequently. In this blog post, we'll examine how to utilize Memcached within a Spring application to solve cache invalidation issues effectively. We'll discuss key strategies and provide practical code snippets to illustrate these concepts.
What is Memcached?
Memcached is a high-performance, distributed memory caching system. It is used to reduce the database load, improve response times, and ensure efficient resource utilization. Memcached stores data in a key-value format, allowing applications to quickly retrieve frequently accessed data.
The Importance of Cache Invalidation
Cache invalidation is the process of updating or removing data from the cache when the underlying data changes. Failure to implement proper cache invalidation can lead to stale data being served to users, which may result in inconsistencies and degraded user experience.
Why Cache Invalidation is Challenging
- Data Volatility: Frequently changing data complicates the tracking of changes.
- Complex Inter-relationships: Related data may need invalidation, but the system may not predict all relevant changes.
- Concurrency Issues: Multiple application instances may lead to conflicting updates.
To tackle these challenges, we can use various strategies for cache invalidation, especially in a Spring context.
Setting Up Memcached with Spring
To integrate Memcached, we can use the Spring framework along with the Spymemcached client. Below are the steps for setting up Memcached within a Spring application.
Step 1: Maven Dependencies
First, add the necessary dependencies to your pom.xml
:
<dependency>
<groupId>net.ravendb</groupId>
<artifactId>spymemcached</artifactId>
<version>2.12.3</version>
</dependency>
Step 2: Configuring Memcached
Next, we need to configure Memcached in our Spring application. We will create a MemcachedClient
bean:
import net.ravendb.maven.MemcachedClient;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MemcachedConfig {
@Bean
public MemcachedClient memcachedClient() {
return new MemcachedClient("localhost:11211");
}
}
Step 3: Using Memcached in the Service Layer
Once the configuration is complete, you can utilize the Memcached client within your service layer. Here is a simple example for caching user data.
import net.ravendb.maven.MemcachedClient;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private MemcachedClient memcachedClient;
public User getUserById(String userId) {
String cacheKey = "user::" + userId;
User user = (User) memcachedClient.get(cacheKey);
if (user == null) {
user = fetchUserFromDatabase(userId);
memcachedClient.set(cacheKey, 3600, user); // Cache for 1 hour
}
return user;
}
private User fetchUserFromDatabase(String userId) {
// Fetch user from the database
// ...
return new User(userId, "John Doe");
}
}
Why Use Caching in This Example?
In the above getUserById
method:
- We first attempt to retrieve the user from Memcached.
- If the user is not present (cache miss), we fetch it from the database, and then cache it.
- This method improves performance, reduces the number of database calls, and ensures that frequently requested data is quickly available.
Cache Invalidation Strategies
1. Time-Based Expiry
One of the simplest strategies for cache invalidation is to set expiration times on cached entries. In the above code sample, we cached the user for one hour. This means that the cache will be refreshed automatically after the specified duration, mitigating stale data.
2. Explicit Invalidation on Data Changes
Another effective strategy is to clear or update cache entries explicitly whenever related data changes. For example, when a user’s information is updated:
public void updateUser(User user) {
updateUserInDatabase(user);
String cacheKey = "user::" + user.getId();
memcachedClient.delete(cacheKey);
// Optional: Set the updated user in cache
memcachedClient.set(cacheKey, 3600, user);
}
In this method, notice how we delete the cache entry after updating it in the database. This explicitly ensures that users always fetch the most recent data after an update.
3. Event-Driven Invalidation
For larger applications, you may implement an event-driven approach using Spring's event listeners to handle updates. This allows your application to become more reactive.
import org.springframework.context.ApplicationEvent;
import org.springframework.context.ApplicationListener;
public class UserUpdatedListener implements ApplicationListener<UserUpdatedEvent> {
@Autowired
private MemcachedClient memcachedClient;
@Override
public void onApplicationEvent(UserUpdatedEvent event) {
String cacheKey = "user::" + event.getUserId();
memcachedClient.delete(cacheKey);
}
}
In this setup, whenever a UserUpdatedEvent
is triggered, it automatically invalidates the cached user data.
4. Composite Keys for Relational Data
When working with related data, using a composite key can help in managing complex dependencies. This approach allows for more precise cache invalidation strategies.
public class PostService {
public Post getPostById(String postId) {
String cacheKey = "post::" + postId;
Post post = (Post) memcachedClient.get(cacheKey);
if (post == null) {
// Fetch post from the database
}
// Invalidate related keys
invalidateRelatedCache("user::" + post.getUserId());
return post;
}
private void invalidateRelatedCache(String userId) {
memcachedClient.delete(userId);
}
}
In this example, deleting related user data whenever a specific post is accessed ensures that any post updates reflect accurately in user posts.
Lessons Learned
Caching is an essential optimization technique for any high-traffic application, but it requires robust cache invalidation strategies to maintain data integrity. Memcached, combined with Spring, provides an excellent framework for implementing these strategies.
Implementing effective cache invalidation will allow your application to scale and respond efficiently to user requests, all while preserving accurate data.
For more information on caching and performance improvements, refer to the official Spring Caching documentation and explore Memcached here.
Feel free to share your experiences and strategies for cache invalidation in the comments below. Happy coding!