Common Errors When Importing CSVs into Neo4j from Spark
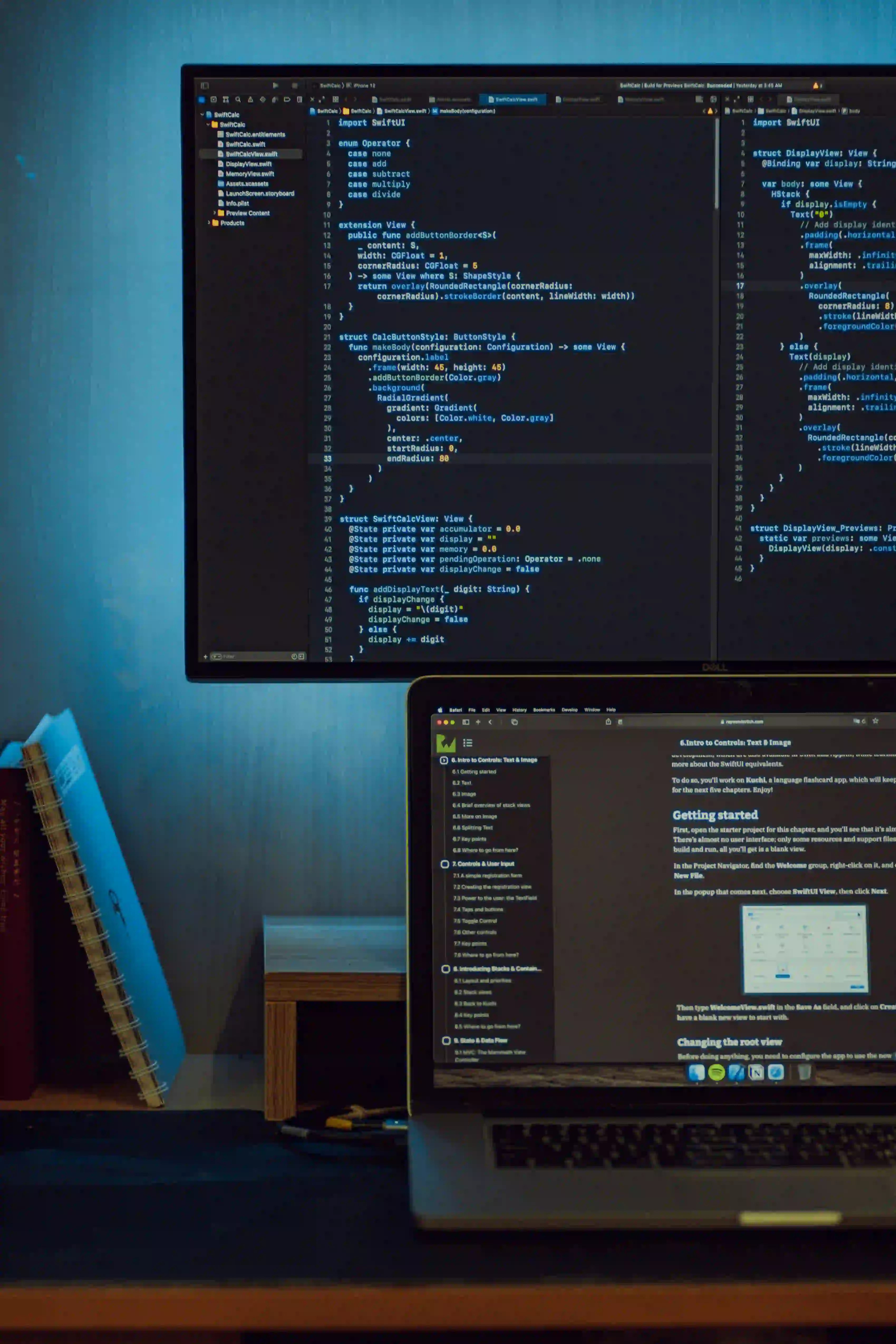
Common Errors When Importing CSVs into Neo4j from Spark
Transferring data from big data processing tools like Apache Spark to graph databases such as Neo4j can be a smooth process when everything is set up correctly. However, it's not uncommon to run into several typical errors during the importation. This blog post will illuminate some common pitfalls and provide solutions to make your data importation process easier and more efficient.
Why Use Neo4j and Spark Together?
Neo4j is a leading graph database that enables you to model and visualize data in a flexible way. Spark, on the other hand, is a powerful distributed computing framework designed to handle large-scale data processing tasks. When combined, they offer a formidable solution for both data analytics and storage.
Basic Concept of Importing CSVs from Spark to Neo4j
Importing CSVs from Spark to Neo4j involves several steps, including data transformation, file saving, and finally, executing the import in Neo4j. Before diving into common errors, here's a simple example of how you can write data from Spark to a CSV.
import org.apache.spark.sql.SparkSession;
public class SparkToCSV {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("Spark to Neo4j Example")
.master("local")
.getOrCreate();
// Creating a sample DataFrame
Dataset<Row> data = spark.read().option("header", "true").csv("input_data.csv");
// Writing to CSV
data.write()
.option("header", "true")
.csv("output_data.csv"); // Output path for CSV
}
}
In this example, we first create a Spark session, read a CSV, and then output it to another CSV file. The "why" behind this setup is that we want a well-structured dataset before importing it into Neo4j.
Common Errors and Their Solutions
1. CSV Format Issues
Error
One common issue is that the CSV file does not conform to standard format rules.
Solution
Ensure your CSV follows these guidelines:
- Consistent use of delimiters (commonly commas).
- Properly escaped characters and strings, especially those containing commas.
You can check this using Spark DataFrame's .show()
and .printSchema()
methods.
data.show(); // displays the DataFrame content
data.printSchema(); // displays the schema of DataFrame
This basic validation helps identify the structure of the data before you write it out to a CSV.
2. Incorrect File Paths
Error
Users may often specify incorrect paths when saving output files.
Solution
Double-check your file path. Consider using absolute paths or verifying relative paths with:
String outputPath = "output_data.csv";
data.write().option("header", "true").csv(outputPath);
Log the output path to confirm:
System.out.println("CSV output path: " + outputPath);
This extra verification can save hours of debugging.
3. Data Type Mismatches
Error
If the data types in your DataFrame do not match the expected types in Neo4j, errors can occur during import.
Solution
Ensure a type check before you write out your DataFrame. Use the following example for casting:
import org.apache.spark.sql.types.DataType;
import org.apache.spark.sql.types.StringType;
Dataset<Row> castedData = data.withColumn("column_name", data.col("column_name").cast(StringType));
By casting the data into the correct type before saving, you can ensure compatibility with Neo4j’s expectations.
4. Missing Headers
Error
If the CSV lacks headers, then column names become problematic in Neo4j as it relies on header information for node and relationship creation.
Solution
Always ensure your DataFrame has headers defined. If exporting without headers, add them explicitly:
data.write()
.option("header", "true")
.csv("output_data.csv");
5. Incorrect Cypher Queries on Import
Error
After importing CSVs into Neo4j, the Cypher query might produce errors due to incorrect syntax or structure.
Solution
Always verify your Cypher syntax. Test your query on smaller datasets to ensure it works properly.
For instance, a common import command looks like this:
LOAD CSV WITH HEADERS FROM 'file:///output_data.csv' AS row
CREATE (:Node {name: row.name, age: toInteger(row.age)});
Make sure that your property names from the CSV match exactly with what you are referencing in your Cypher query.
6. Memory Limits
Error
When dealing with large CSV files, you may run into memory issues, resulting in Spark jobs failing.
Solution
Optimize your Spark configuration parameters beforehand to handle bigger datasets:
spark.conf().set("spark.executor.memory", "4g");
spark.conf().set("spark.driver.memory", "4g");
Adjust the memory settings according to your available system resources, ensuring that Spark has enough memory for operation.
7. Misconfigured Neo4j Database
Error
The Neo4j database must be configured correctly (e.g., required plugins).
Solution
Ensure that the following configurations are checked:
- Enable APOC library for better handling of your data import process.
- Verify your database is running and that access permissions are set.
You can confirm this by visiting your Neo4j console (typically at http://localhost:7474
).
My Closing Thoughts on the Matter
Navigating CSV imports from Apache Spark into Neo4j doesn’t have to be an uphill battle. By being aware of common errors and implementing solutions proactively, you can transform this data import process into a more seamless operation. The integration of Spark and Neo4j allows you to elevate your data processing capabilities tremendously. For more in-depth knowledge and best practices, refer to the Neo4j Import Documentation and Apache Spark Documentation.
Happy importing!