When Refactoring Fails: Recognizing Its Limits
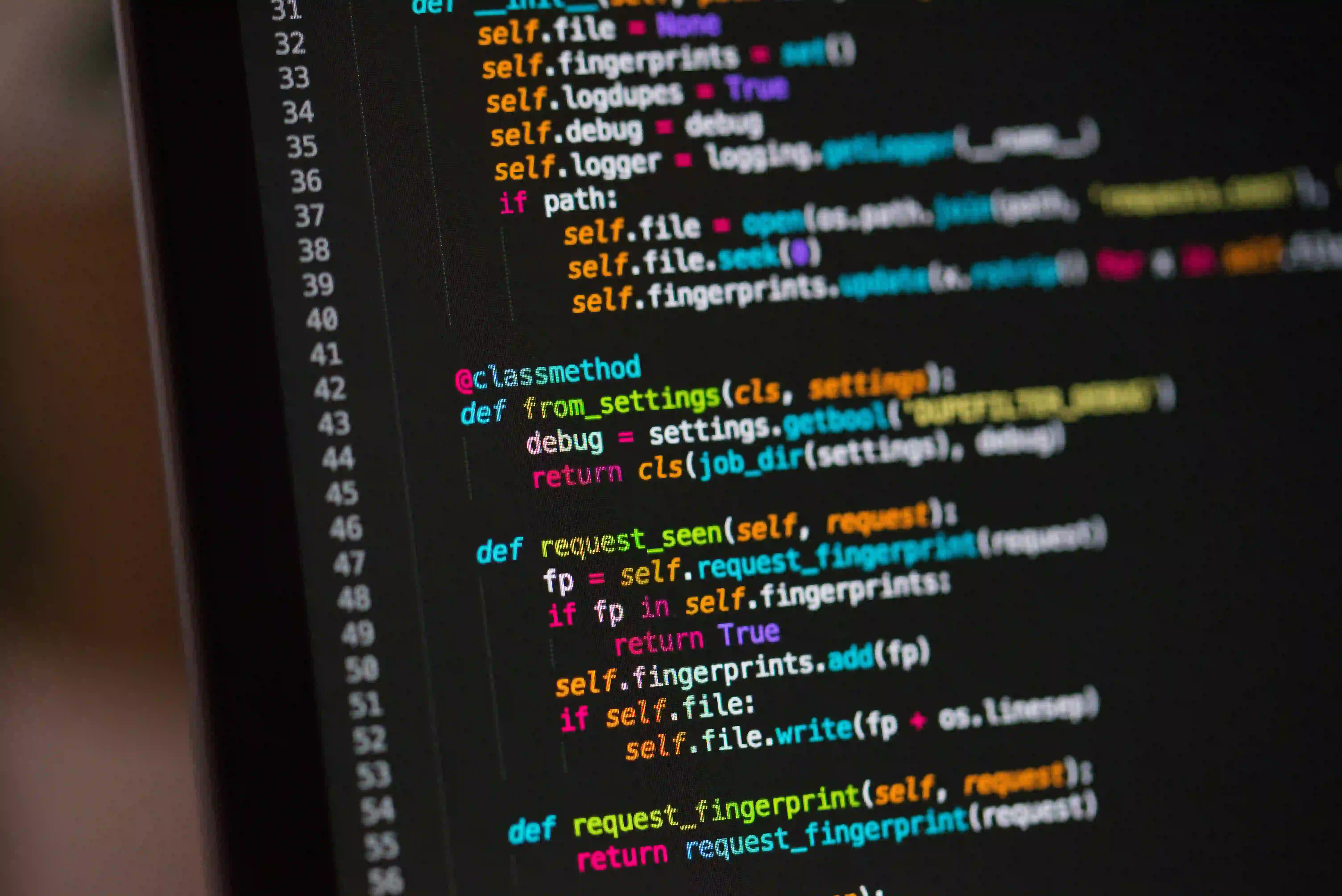
When Refactoring Fails: Recognizing Its Limits
Refactoring is often hailed as the silver bullet in software development—a timeless strategy to improve the internal structure of code without altering its external behavior. However, it is essential to understand that refactoring has its limits. At times, a refactoring effort can backfire, leading to decreased code quality, wasted resources, and even business disruption. This blog post delves into the circumstances under which refactoring fails, and how developers can recognize these limits early on.
Understanding Refactoring
Before we dive into the pitfalls, let's clarify what refactoring is. In essence, refactoring is the process of restructuring existing code without changing its functionality. The primary goals are to improve code readability, reduce complexity, and enhance maintainability.
It is, however, not a panacea. Let us examine when and why refactoring can stall or go astray.
1. Over-Refactoring
The Problem
One of the most common issues arises from over-refactoring. When developers refactor without a clear understanding of the existing system, they may introduce complexity instead of reducing it.
Example Code Snippet
Consider a simple function that calculates the sum of an array:
public int calculateSum(int[] numbers) {
int sum = 0;
for(int number : numbers) {
sum += number;
}
return sum;
}
In an attempt to make this code "better," one might refactor it like so:
public int calculateSum(int[] numbers) {
return Arrays.stream(numbers).sum();
}
While using a stream is a more modern approach, it could lead to performance issues, especially for large datasets. The initial version is straightforward and performs well for its purpose.
Why This Matters
Refactoring should serve as an improvement. When aiming to use new techniques or libraries—like Java Streams—scrutinize whether they genuinely benefit your project. Always assess the trade-offs involved.
2. Lack of Proper Testing
The Problem
Refactoring without an effective testing strategy is a recipe for disaster. Changes made to the structure of the code without tests can inadvertently introduce bugs.
The Solution
Make comprehensive tests a non-negotiable part of your development workflow. Unit tests, integration tests, and user acceptance tests should be in place before undertaking any refactoring.
Example Code Snippet
Here is a simple example of a unit test for the initial sum calculation:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class SumTest {
@Test
public void testCalculateSum() {
int[] numbers = {1, 2, 3, 4, 5};
int expectedSum = 15;
assertEquals(expectedSum, calculateSum(numbers));
}
}
Using testing frameworks like JUnit ensures you can safely refactor your code without fear of breaking existing functionality. For more about unit testing in Java, check out JUnit documentation.
3. Poor Understanding of Domain Requirements
The Problem
Poor comprehension of domain requirements can lead to incorrect assumptions about what the code does or should do. This misunderstanding can drive unnecessary refactoring efforts that yield no actual benefit.
Why It Happens
- Lack of contact with stakeholders.
- Insufficient documentation of requirements.
- Limited knowledge of domain-specific conventions.
The Solution
Always have a clear understanding of what the code is supposed to achieve. Work closely with product managers and stakeholders to gather accurate requirements.
User stories and requirement documents should be your guiding light rather than just focusing on code quality. Avoid altering parts of the system that do not require refactoring or that you don’t fully understand.
4. Too Many Dependencies
The Problem
The more dependencies you have, the more cautious you should be when refactoring. It becomes much harder to isolate changes when many components are intertwined.
The Solution
Implement techniques such as Dependency Injection (DI) to manage dependencies more effectively. With DI, you can provide classes with their dependencies externally rather than as hard-coded values. This increases modularity and makes testing easier.
Example Code Snippet
public class Calculator {
private SumStrategy sumStrategy;
public Calculator(SumStrategy sumStrategy) {
this.sumStrategy = sumStrategy;
}
public int calculate(int[] numbers) {
return sumStrategy.sum(numbers);
}
}
With Dependency Injection, you can easily swap out the SumStrategy
implementation during testing, helping to maintain the purity of your testing environment.
5. Inflexible Design Patterns
The Problem
Using design patterns inappropriately can lead to inflexible code. Certain patterns may not apply well to your architecture, causing unexpected repercussions during refactoring.
The Solution
Carefully evaluate the patterns you adopt. Make sure they align cohesively with your project's goals.
For instance, consider the following class structure utilizing the Singleton pattern:
public class Singleton {
private static final Singleton instance = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return instance;
}
}
While it is well-implemented, if your application actually requires multiple instances, this pattern could create a bottleneck and limit functionality.
A Final Look
Refactoring is undoubtedly a powerful technique that can lead to the creation of clean, efficient code. However, recognizing its limits ensures that you reap its benefits without falling into pitfalls.
Always ask the following questions:
- Are the changes genuinely improving code quality?
- Do I have a solid suite of tests in place?
- Do I fully understand the requirements of my code?
- How do dependencies impact the changes I'm making?
- Am I using design patterns appropriately?
When you recognize the limits of refactoring, you are better equipped to craft a maintainable codebase that stands the test of time.
For further reading on refactoring best practices, refer to Martin Fowler's book on Refactoring. It's packed with insights that can radically enhance your software development approach.
In summary, take a balanced approach, remain vigilant, and ensure that refactoring serves to enhance your code rather than complicate it.