Overcoming JVM Heap Limitations for Heavy Memory Apps
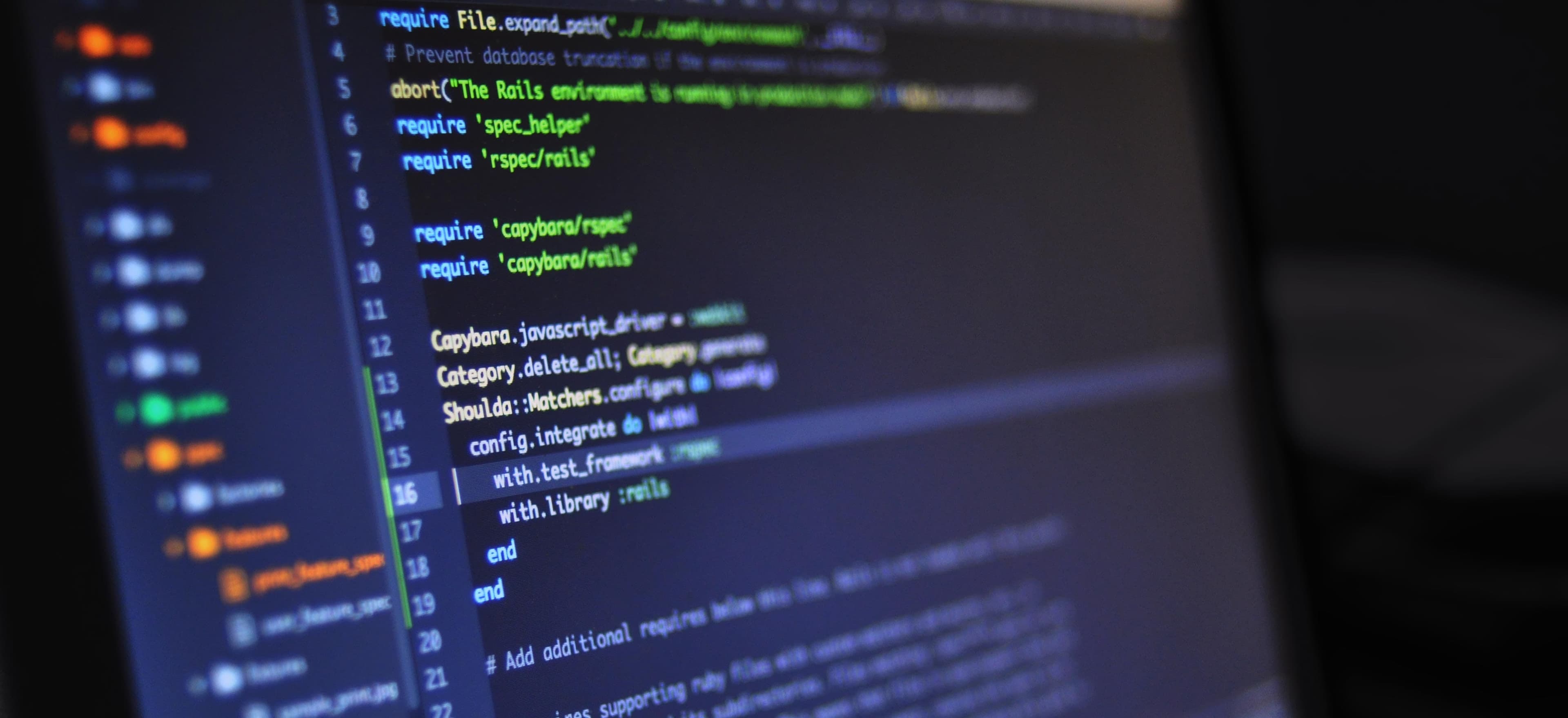
- Published on
Overcoming JVM Heap Limitations for Heavy Memory Apps
The Java Virtual Machine (JVM) is a cornerstone of the Java ecosystem. It offers a plethora of features that aid developers in building robust applications. However, one limitation that often hampers performance, particularly in memory-intensive applications, is the JVM heap size. In this article, we will explore techniques to overcome JVM heap limitations effectively, ensuring your heavy memory applications run smoothly.
Understanding the JVM Heap
The JVM heap is the runtime data area where Java objects are allocated. It's divided into several regions:
- Young Generation: Where new objects are created and garbage-collected quickly.
- Old Generation (Tenured Generation): Where long-lived objects reside after surviving several garbage collections.
- Permanent Generation (Metaspace in Java 8 and above): Where metadata about classes and methods is stored.
The maximum size of the heap can be defined using the -Xmx
flag. However, exceeding these limits can lead to performance degradation and OutOfMemoryError exceptions.
Why Heap Limitations Matter
When your application fails to manage memory efficiently, you may encounter various problems, including:
- Sluggish Performance: Excessive garbage collection (GC) cycles can drastically reduce application speed.
- OutOfMemoryError: This error results from exceeding the allocated memory limits, causing applications to crash.
- Inefficient Resource Utilization: Suboptimal memory allocation can lead to wasted resources and increased costs.
Before applying strategies to expand heap usage, it's essential first to collect all relevant data about memory consumption through monitoring or profiling tools.
Strategies to Overcome JVM Heap Limitations
1. Increasing the Heap Size
The most straightforward approach to address heap limitations is simply to increase the heap size. This can be done by adjusting the JVM parameters:
java -Xms512m -Xmx4g -jar YourApp.jar
In the command above, -Xms
sets the initial heap size to 512MB, while -Xmx
sets the maximum heap size to 4GB.
Why Increase Heap Size?
Larger heap sizes can accommodate more objects before garbage collection occurs, leading to fewer pauses in your application's execution. However, this is only a temporary solution and may not resolve underlying memory issues.
2. Use of G1 Garbage Collector
In Java 9 and later, the G1 Garbage Collector (Garbage First) can be a game changer for heavy memory applications. This collector is designed to optimize garbage collection for large heaps.
java -XX:+UseG1GC -Xmx4g -jar YourApp.jar
Why G1 GC?
G1 GC breaks the heap into regions and collects garbage more intelligently. This capability reduces long pause times, making it more suited for applications requiring high throughput. It also allows for predictable short pause times, which is beneficial for interactive applications.
3. YugabyteDB and Partitioning Data
If your application manages substantial data, consider leveraging sharded databases like YugabyteDB. By horizontally sharding your data, you can reduce the amount of memory required for in-memory operations.
Example of Sharding Strategy
Instead of loading all user data into memory, divide it based on region or user type before loading it into your application.
public void loadUsersByRegion(String region) {
List<User> users = database.fetchUsersByRegion(region);
// Process users...
}
Why Use Sharding?
Sharding partitions the data, enabling your application to handle smaller data sets in memory rather than loading everything at once. This not only minimizes memory consumption but also improves application performance.
4. Off-Heap Memory Management
Java provides several off-heap memory management options, allowing you to allocate memory outside of the JVM heap. Libraries such as Apache Ignite and Java Memory Model (JVM) enable off-heap data management.
import org.apache.ignite.Ignite;
import org.apache.ignite.Ignition;
Ignite ignite = Ignition.start();
ignite.cache("myCache").put("key", "value");
Why Off-Heap Memory?
Off-heap memory can hold large amounts of data without imposing strict limits from JVM heap size. These techniques may involve using native memory through APIs or employing specialized libraries, resulting in improved performance.
5. Memory-Efficient Coding Practices
Improving the way your application handles memory can also yield significant benefits.
- Avoid Memory Leaks: Use
WeakReference
when you want to allow the garbage collector to clear objects when memory is low. - Use Primitive Data Types: When working with collections, prefer primitive types to avoid unnecessary boxing and memory overhead.
List<Integer> numbers = new ArrayList<>();
for (int i = 0; i < 10000; i++) {
numbers.add(i); // Using primitive int directly
}
Why Use Efficient Coding Practices?
Optimizing memory usage can reduce the size of the heap or slow the rate at which it's filled. Efficient coding helps maximize resource usage and can substantially improve application responsiveness.
6. Monitoring and Profiling
Regularly monitor and profile your application for memory usage using tools like VisualVM or Eclipse Memory Analyzer (MAT).
Code Implementation for Monitoring
You can integrate monitoring libraries such as Micrometer for better observability:
import io.micrometer.core.instrument.MeterRegistry;
public class MemoryService {
private MeterRegistry meterRegistry;
public MemoryService(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
public void trackMemoryUsage() {
Runtime runtime = Runtime.getRuntime();
long usedMemory = runtime.totalMemory() - runtime.freeMemory();
meterRegistry.gauge("memory.used", usedMemory);
}
}
Why Monitoring Matters?
Monitoring provides insights into your application's memory behavior, allowing you to identify patterns and potential problems before they result in significant downtime.
My Closing Thoughts on the Matter
In this guide, we've explored various strategies to overcome JVM heap limitations in heavy memory applications. From increasing heap size using JVM parameters to implementing efficient coding practices and employing off-heap memory management, the techniques discussed can significantly enhance performance. Furthermore, don't underestimate the importance of monitoring your application. Regular profiling can make the difference between a smooth-running application and one plagued with performance issues.
To wrap up, it’s essential to remember that there is no one-size-fits-all solution. The right combination of techniques will depend on your specific application requirements and workload. By understanding the intricacies of memory management in the JVM, you are better equipped to build scalable and resilient Java applications.
For further reading, consider exploring:
- Java Garbage Collection Explained
- Memory Management in Java
By refining your understanding and continuously adjusting your application strategies, you can ensure optimal performance, even in the face of heavy memory demands.
Checkout our other articles