Common Challenges When Using Apache Ignite Client
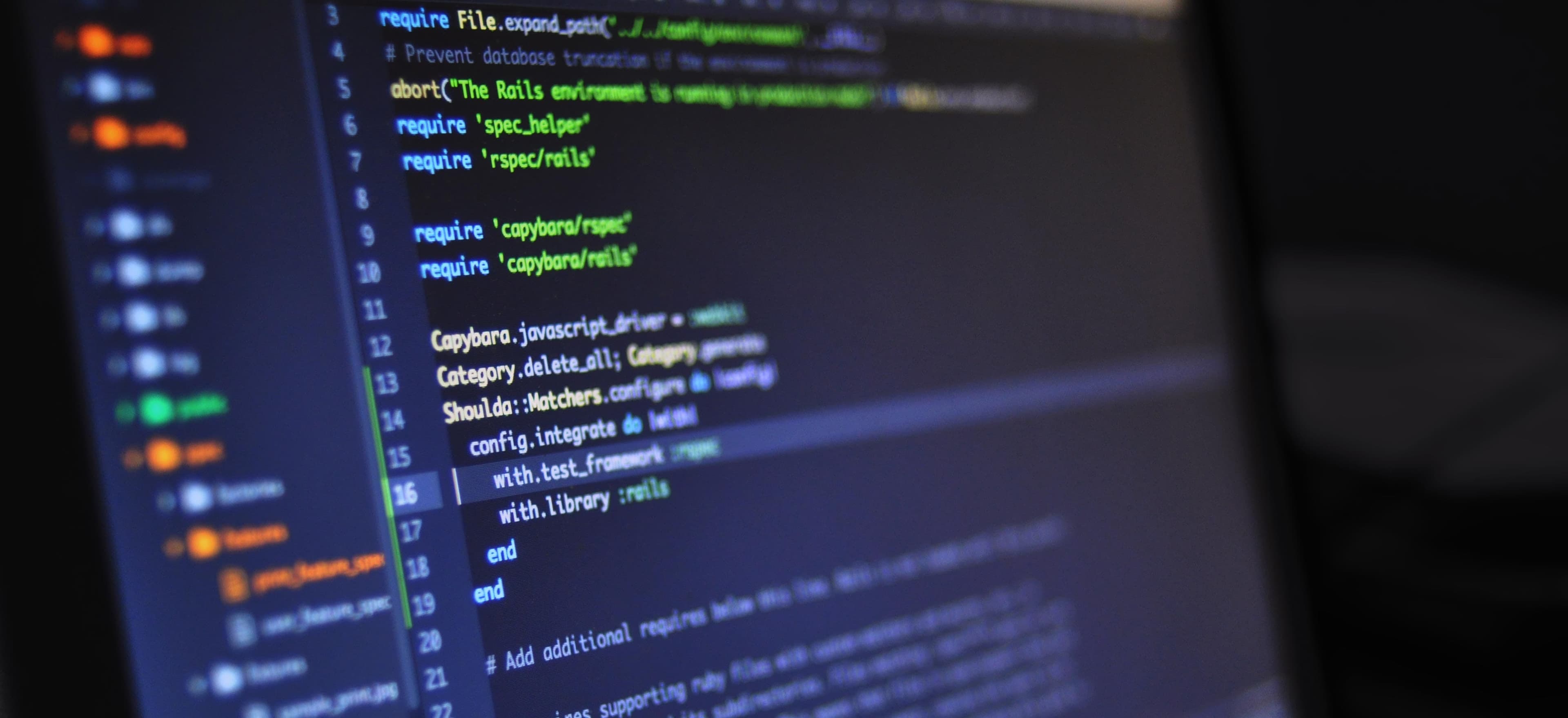
- Published on
Common Challenges When Using Apache Ignite Client
Apache Ignite is an in-memory computing platform that provides a high-performance, distributed architecture for processing data. It enables businesses to scale their applications and access data in real-time. While it offers numerous benefits, developers often encounter common challenges when using the Apache Ignite Client.
In this blog post, we will discuss these challenges and offer practical solutions.
Overview of Apache Ignite
Before diving into the challenges, let’s briefly recap what Apache Ignite is.
- In-Memory Data Grid: Apache Ignite is designed to provide fast, low-latency data access through in-memory data storage.
- Durability: It supports persistent storage, allowing data to be retained even after server restarts.
- Distributed Computing: Ignite allows the distribution of data and processing across multiple nodes, thereby increasing scalability.
For more information on Apache Ignite, the official Apache Ignite Documentation is an excellent resource.
Advantages of Using Apache Ignite Client
To set the context, let’s list some advantages:
- Speed: Keeps frequently accessed data in memory, drastically reducing access times compared to traditional databases.
- Scalability: Horizontal scaling is straightforward as adding new nodes can be done with minimal configuration.
- SQL Support: Ignite supports ANSI SQL, which allows developers to leverage their existing SQL skills.
Common Challenges
Though Ignite is powerful, using it effectively comes with its own set of challenges. Let’s explore these challenges one by one.
1. Configuration Complexity
Challenge: Apache Ignite has a flexible architecture that can be complex to configure effectively. This complexity increases as you scale your deployment.
Solution: Utilize Ignite's IgniteConfiguration
object to streamline your configuration. Here’s a simple code snippet that demonstrates how to create a basic Ignite configuration.
import org.apache.ignite.Ignite;
import org.apache.ignite.Ignition;
import org.apache.ignite.configuration.IgniteConfiguration;
// Create an IgniteConfiguration instance
IgniteConfiguration cfg = new IgniteConfiguration();
// Set the communication SPI
cfg.setCommunicationSpi(new TcpCommunicationSpi());
// Start the Ignite node
try (Ignite ignite = Ignition.start(cfg)) {
System.out.println("Ignite node started with configuration.");
}
Why This Matters: By customizing the IgniteConfiguration
, you can enhance performance according to your specific needs. Missed settings can result in suboptimal performance and unnecessary overhead.
2. Data Serialization
Challenge: Apache Ignite requires data objects to be serializable to store them in the grid. Improper serialization can lead to performance issues.
Solution: Implement the Serializable
interface in your data classes or use Ignite's built-in DataStreamer
. Below is an example of a serializable data class:
import java.io.Serializable;
public class Person implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
// Constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getter and Setter methods
public String getName() { return name; }
public int getAge() { return age; }
}
Why This Matters: Proper serialization ensures that objects can be efficiently marshaled over the network, which is critical for distributed systems. This affects data retrieval times and overall application performance.
3. Query Performance
Challenge: Querying large datasets can lead to performance bottlenecks. Inefficient indexing can exacerbate this issue.
Solution: Use Ignite SQL queries and ensure proper indexing strategies. Here’s how to create an index in Apache Ignite:
import org.apache.ignite.cache.query.Index;
IgniteCache<Integer, Person> cache = ignite.getOrCreateCache("personCache");
// Create an index for the 'name' field
cache.query(new SqlFieldsQuery("CREATE INDEX idx_name ON Person(name)"));
Why This Matters: Indexing can significantly speed up query performance, especially for large datasets. Ignoring indexing can lead to full table scans, which severely degrade performance.
4. Cluster Management
Challenge: Managing a distributed cluster can be challenging. Node failures can lead to data inconsistencies if not properly handled.
Solution: Use Ignite’s built-in fault tolerance and data replication features. Implement consistent hashing algorithms to distribute data evenly. An example for configuring backups is as follows:
cacheConfiguration.setBackups(1); // Set the number of backups to 1
Why This Matters: Proper backup configurations ensure that even if a node fails, your data remains accessible. This is essential for high-availability applications.
5. Monitoring and Diagnostics
Challenge: Monitoring the performance of a distributed system is often more complex than a standalone application.
Solution: Leverage tools like Apache Ignite’s Management Console to monitor statistics and performance metrics. You can also integrate third-party monitoring tools like Prometheus or Grafana.
Example Usage:
Apache Ignite provides an embedded web console you can access at http://localhost:8080/
.
Why This Matters: Regular monitoring helps you identify performance bottlenecks and allows for quicker resolution of issues, ensuring your application runs smoothly.
6. Memory Management
Challenge: Boosting performance through in-memory data often leads to memory management issues. Improperly configured memory settings can lead to OutOfMemoryError.
Solution: Use Ignite’s memory configuration features to fine-tune memory allocation.
MemoryConfiguration memCfg = new MemoryConfiguration();
memCfg.setMemoryPolicyConfigurations(new MemoryPolicyConfiguration[] {
new MemoryPolicyConfiguration("default", 10L * 1024 * 1024 * 1024, 0.9f)
});
cfg.setMemoryConfiguration(memCfg);
Why This Matters: Proper memory management is crucial in a system where performance relies heavily on memory utilization. This can prevent unexpected application crashes and provide a more stable performance.
7. Client-Side Logic
Challenge: Writing client-side logic that interacts smoothly with the Ignite server can sometimes be tricky, especially when dealing with distributed computation.
Solution: Make sure to isolate logic to be run on the server-side. Use Ignite's compute grid for distributed processing.
IgniteCompute compute = ignite.compute();
String result = compute.call(() -> {
// Perform tasks on the cluster
return "Task completed on server.";
});
Why This Matters: Offloading tasks to the Ignite server helps minimize network latency. This allows developers to take advantage of the distributed capabilities of Apache Ignite effectively.
Key Takeaways
While Apache Ignite offers powerful solutions for data-intensive applications, developers may face various challenges when using the Ignite Client.
By understanding these common challenges and deploying the provided solutions, you can maximize the performance and stability of your distributed applications.
For more in-depth knowledge and discovering new features, check the official Apache Ignite GitHub Repository.
By being proactive in addressing these issues, you can harness the full potential of Apache Ignite and build robust, high-performance applications tailored to your business needs.
Feel free to share your experiences or challenges you've faced while working with Apache Ignite in the comments below. Happy coding!
Checkout our other articles