Mastering Custom Exception Testing in JUnit
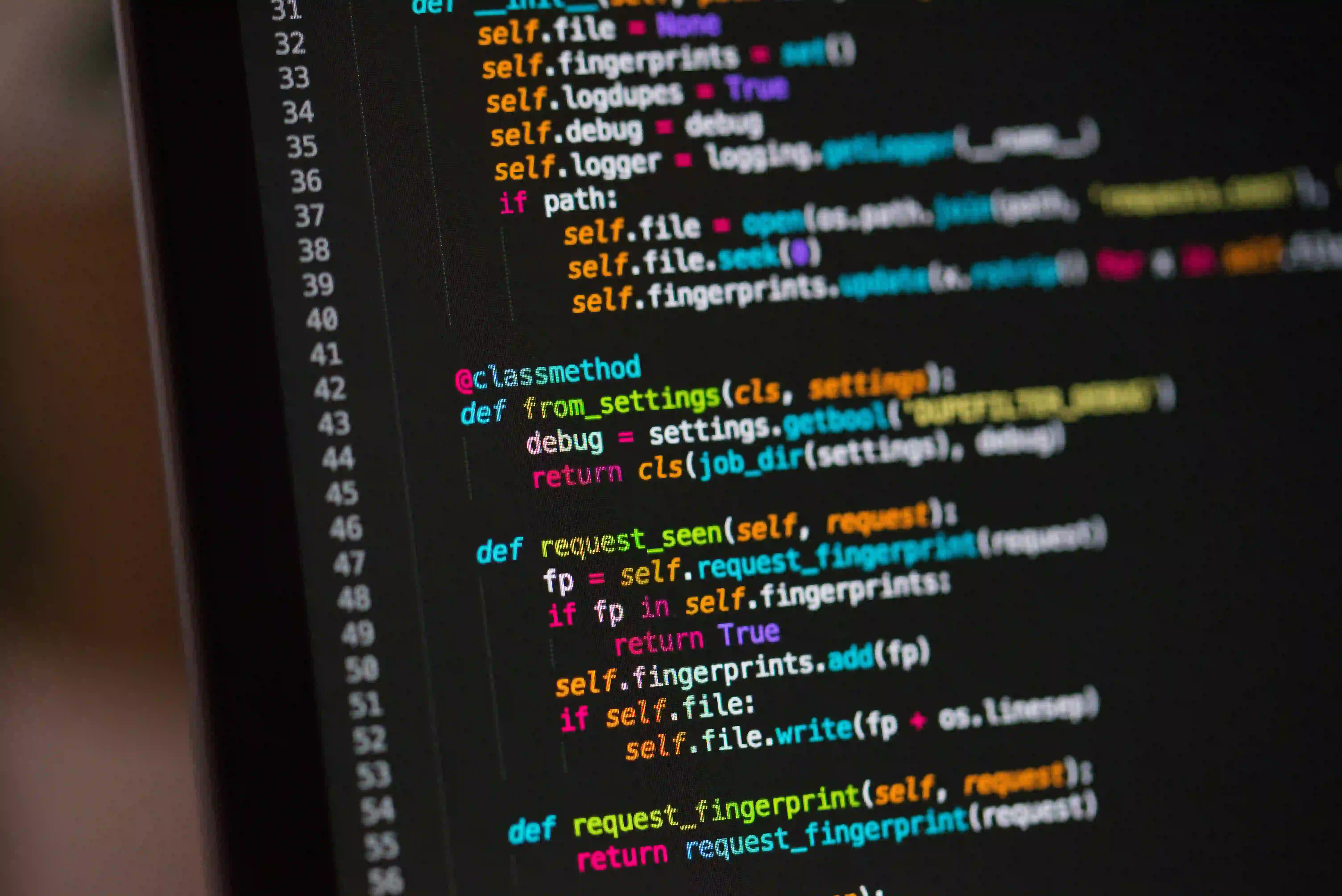
Mastering Custom Exception Testing in JUnit
In the realm of Java development, managing exceptions effectively is paramount. Custom exceptions allow developers to throw meaningful errors and simplify debugging. However, ensuring that these exceptions are tested correctly can be challenging. In this post, we will dive deep into how to create and test custom exceptions using JUnit. You will also learn best practices along the way.
What Are Custom Exceptions?
Custom exceptions are user-defined exceptions that extend the standard Java Exception class. They provide clarity and context to error handling in your applications. When an error occurs, a custom exception can communicate the issue more succinctly than a standard exception.
Why Use Custom Exceptions?
- Clarity: Custom exceptions can reflect specific business logic conditions.
- Separation of Concerns: They isolate error handling related to particular components.
- Enhanced Traceability: Easier to understand the flow and context of the error.
Creating a Custom Exception
Let's start with a simple example. Here’s how to create a custom exception that signals an invalid operation within our application.
public class InvalidOperationException extends Exception {
public InvalidOperationException(String message) {
super(message);
}
public InvalidOperationException(String message, Throwable cause) {
super(message, cause);
}
}
In this example, we defined a custom exception called InvalidOperationException
that takes a message and an optional cause. This allows the exception to express both high-level error details and the underlying reason.
Throwing Custom Exceptions
Once you've defined a custom exception, the next step is to throw it in your code. Below is a simple bank account example that checks for a negative balance operation.
public class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
this.balance = initialBalance;
}
public void withdraw(double amount) throws InvalidOperationException {
if (amount > balance) {
throw new InvalidOperationException("Insufficient funds for withdrawal");
}
balance -= amount;
}
}
In this code snippet, if an attempt is made to withdraw more than the current balance, we throw an InvalidOperationException
. This helps ensure that the caller is informed of the specific error.
Testing Custom Exceptions with JUnit
One of the most effective ways to validate the behavior of your custom exceptions is through unit testing. JUnit provides excellent support for exception testing. Let's explore how to do this effectively.
Basic Exception Testing with JUnit 5
To test custom exceptions, we can use the assertThrows
method from the JUnit 5 library. Here’s how you can test that our BankAccount
class throws an InvalidOperationException
for insufficient funds.
import static org.junit.jupiter.api.Assertions.assertThrows;
import org.junit.jupiter.api.Test;
public class BankAccountTest {
@Test
public void testWithdrawThrowsInvalidOperationException() {
BankAccount account = new BankAccount(100.0);
InvalidOperationException exception = assertThrows(InvalidOperationException.class, () -> {
account.withdraw(200.0);
});
assertEquals("Insufficient funds for withdrawal", exception.getMessage());
}
}
Breaking Down the Test
- assertThrows: This method checks that the specified exception is thrown during the execution of a lambda expression.
- getMessage: Validating the exception message ensures that the correct error context is being communicated.
Complete Testing: Edge Cases
When dealing with exceptions, it is crucial to check various scenarios. Ensure you also test for valid operations:
@Test
public void testWithdrawValidAmount() throws InvalidOperationException {
BankAccount account = new BankAccount(100.0);
account.withdraw(50.0); // Should not throw an exception
assertEquals(50.0, account.getBalance(), 0.001);
}
Make sure to add a method in the BankAccount
class to retrieve the balance:
public double getBalance() {
return balance;
}
Testing Multiple Scenarios
Testing only the expected or the happy paths can lead to missed edge cases. Use parameterized tests if you anticipate variations in input values. For example, if you want to ensure that negative withdrawal amounts also throw exceptions:
import static org.junit.jupiter.params.provider.Arguments.arguments;
import static org.junit.jupiter.params.provider.ArgumentsProvider;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.MethodSource;
import java.util.stream.Stream;
public class BankAccountParameterizedTest {
private static Stream<Arguments> withdrawalArguments() {
return Stream.of(
arguments(-10.0),
arguments(150.0)
);
}
@ParameterizedTest
@MethodSource("withdrawalArguments")
public void testWithdrawInvalidInputs(double amount) {
BankAccount account = new BankAccount(100.0);
InvalidOperationException exception = assertThrows(InvalidOperationException.class, () -> {
account.withdraw(amount);
});
assertEquals("Insufficient funds for withdrawal", exception.getMessage());
}
}
This provides a robust approach to ensure coverage of various invalid inputs and edge cases.
Best Practices for Custom Exception Testing
- Keep It Simple: Ensure your custom exceptions are clear and positioned in the correct context within the code.
- Specific Messages: Include informative error messages in your exceptions that can guide debugging.
- Unit Test Coverage: Aim for complete test coverage on both expected and edge cases.
Conclusion
Custom exceptions are a powerful tool in Java, enabling more effective error handling and communication. Testing these exceptions with JUnit is essential to maintaining the reliability of your applications. The examples above illustrate how to create and test custom exceptions thoroughly and efficiently.
For further reading about exception handling in Java, you can check out the Java Tutorials on Exception Handling.
Additionally, the JUnit documentation provides a comprehensive guide to learning more about testing in Java. Visit the JUnit 5 User Guide for more details.
By mastering custom exception testing in JUnit, you can ensure that your applications are robust and easy to maintain, ultimately leading to a better software development experience. Happy coding!