Common Spring Boot Mistakes That Can Derail Your Launch
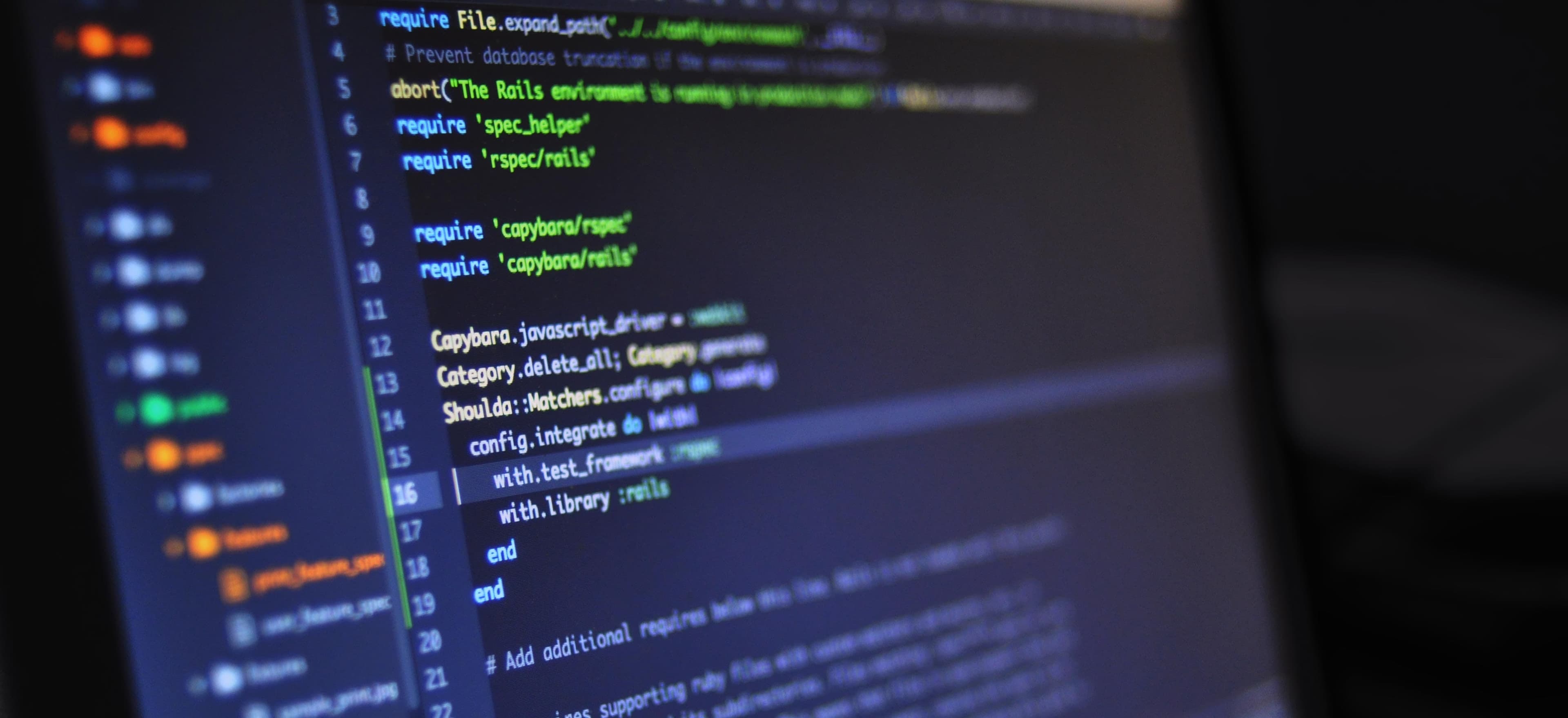
- Published on
Common Spring Boot Mistakes That Can Derail Your Launch
Spring Boot has revolutionized Java development by simplifying the process of building production-ready applications. However, despite its powerful features and convenience, many developers stumble into pitfalls that can significantly affect application performance, maintainability, and scalability. In this article, we'll explore common Spring Boot mistakes that can derail your project launch, and how to avoid them.
1. Ignoring Application Properties
One of the most prevalent mistakes in Spring Boot development is neglecting the application.properties
or application.yml
file. This configuration file allows you to customize various aspects of your application, from database connections to security settings.
Why it matters: Misconfigurations can lead to application failures, especially in production.
Example
server.port=8080
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
In this example, ensure your database configurations are updated before launching. Failing to do so might mean your application will not connect to the correct database, leading to failed requests or data inconsistencies.
Best Practice: Always double-check your configuration files and consider using environment variables for sensitive data.
2. Not Leveraging Spring Boot Starters
Spring Boot provides a set of "starters" that streamline dependency management by bringing together configurations and library dependencies needed for specific functionalities.
Why it matters: This approach reduces boilerplate and simplifies build files.
Example
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
</dependencies>
In this snippet, the inclusion of spring-boot-starter-web
automatically brings in Spring MVC and Tomcat, while spring-boot-starter-data-jpa
includes JPA and Hibernate dependencies. Not using starters can lead to missing dependencies and runtime errors.
Best Practice: Always use Spring Boot starters to simplify dependency management and reduce compatibility issues.
3. Hardcoding Values
While code sometimes requires constants, hardcoding values—especially those related to configurations—can drastically reduce flexibility and maintainability.
Why it matters: Hardcoded values make future changes tedious and introduce a high risk of errors.
Example
public class UserService {
private static final String DEFAULT_ROLE = "USER";
public User createUser(String username) {
User user = new User();
user.setUsername(username);
user.setRole(DEFAULT_ROLE); // Hardcoded value
return user;
}
}
Using constants directly in the code can be problematic. If you want to change DEFAULT_ROLE
later, you must revisit and modify each usage in your codebase. This is neither efficient nor scalable.
Best Practice: Use application properties and inject them using Spring’s @Value
annotation to promote configurability.
@Value("${default.user.role}")
private String defaultRole;
4. Overusing @Autowired
Dependency injection in Spring Boot makes code cleaner and more testable. However, over-relying on the @Autowired
annotation can lead to less maintainable code.
Why it matters: It can create hidden dependencies that complicate testing and validate class responsibilities.
Example
@Service
public class OrderService {
@Autowired
private UserService userService; // Overuse of @Autowired
public void processOrder(Order order) {
User user = userService.findUser(order.getUserId());
// process the order
}
}
While it’s straightforward, this usage can lead to a tightly coupled design. Instead, consider constructor injection, which improves testability.
Best Practice: Prefer constructor injection to promote immutability and highlight class dependencies.
@Service
public class OrderService {
private final UserService userService;
@Autowired
public OrderService(UserService userService) {
this.userService = userService;
}
}
5. Not Using Proper Exception Handling
Exception handling is crucial for maintaining a robust application. Simply throwing exceptions without handling them adequately can lead to poor user experiences.
Why it matters: Proper exception handling ensures that issues are reported in a user-friendly way and can also aid with troubleshooting.
Example
@GetMapping("/user/{id}")
public User getUser(@PathVariable String id) {
return userService.findUserById(id); // May throw exception
}
In the above snippet, if a user with the given ID doesn’t exist, the application might throw an unhandled exception that results in a server error.
Best Practice: Use @ControllerAdvice
to centralize exception handling throughout your application.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<String> handleUserNotFound(UserNotFoundException e) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(e.getMessage());
}
}
6. Failing to Optimize Your SQL Queries
Spring Data JPA simplifies database integration but can lead to performance issues if not handled correctly. Developers often overlook optimizing SQL queries, relying instead on the default behavior.
Why it matters: Inefficient queries can lead to high latency and strain on resources, degrading user experience.
Example
Using the default findAll()
method without pagination can be problematic:
List<User> users = userRepository.findAll(); // Fetches all users at once
If the User table contains thousands of records, fetching all records in one go can lead to out-of-memory exceptions.
Best Practice: Use pagination when retrieving results to manage large datasets effectively.
Page<User> users = userRepository.findAll(PageRequest.of(0, 10)); // Fetches a page of users
7. Limited Testing and Ignoring Profiles
Testing is crucial to ensuring the quality of your application before launch. Spring Boot provides several ways to run tests, including integration and unit testing. However, many developers launch without rigorous testing.
Why it matters: Insufficient testing can lead to undiscovered bugs that surface only after deployment, affecting users.
Example
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserServiceTest {
@Autowired
private UserService userService;
@Test
public void testCreateUser() {
// Test the creation of a user
}
}
In this scenario, using @SpringBootTest
will help ensure your application context is loaded for integration tests.
Best Practice: Always implement thorough testing scenarios and utilize Spring profiles to switch between environments for testing and production.
# application-test.properties
spring.datasource.url=jdbc:mysql://localhost:3306/testdb
Wrapping Up
Spring Boot is a robust framework, but skipping fundamental best practices can lead to complications that hinder your application's success. By avoiding these common mistakes—properly configuring application properties, leveraging Spring Boot starters, avoiding hardcoded values, employing constructor injection, handling exceptions correctly, optimizing SQL queries, and thorough testing—you can set a solid foundation.
For more information on Spring Boot best practices, you can refer to the Spring Boot documentation or explore Baeldung’s Spring Boot tutorials.
By implementing the aforementioned strategies, you're not only ensuring a smooth launch but also paving the way for a scalable, maintainable codebase. Happy coding!
Checkout our other articles