Mastering Java Servlets: Common Beginner Mistakes to Avoid
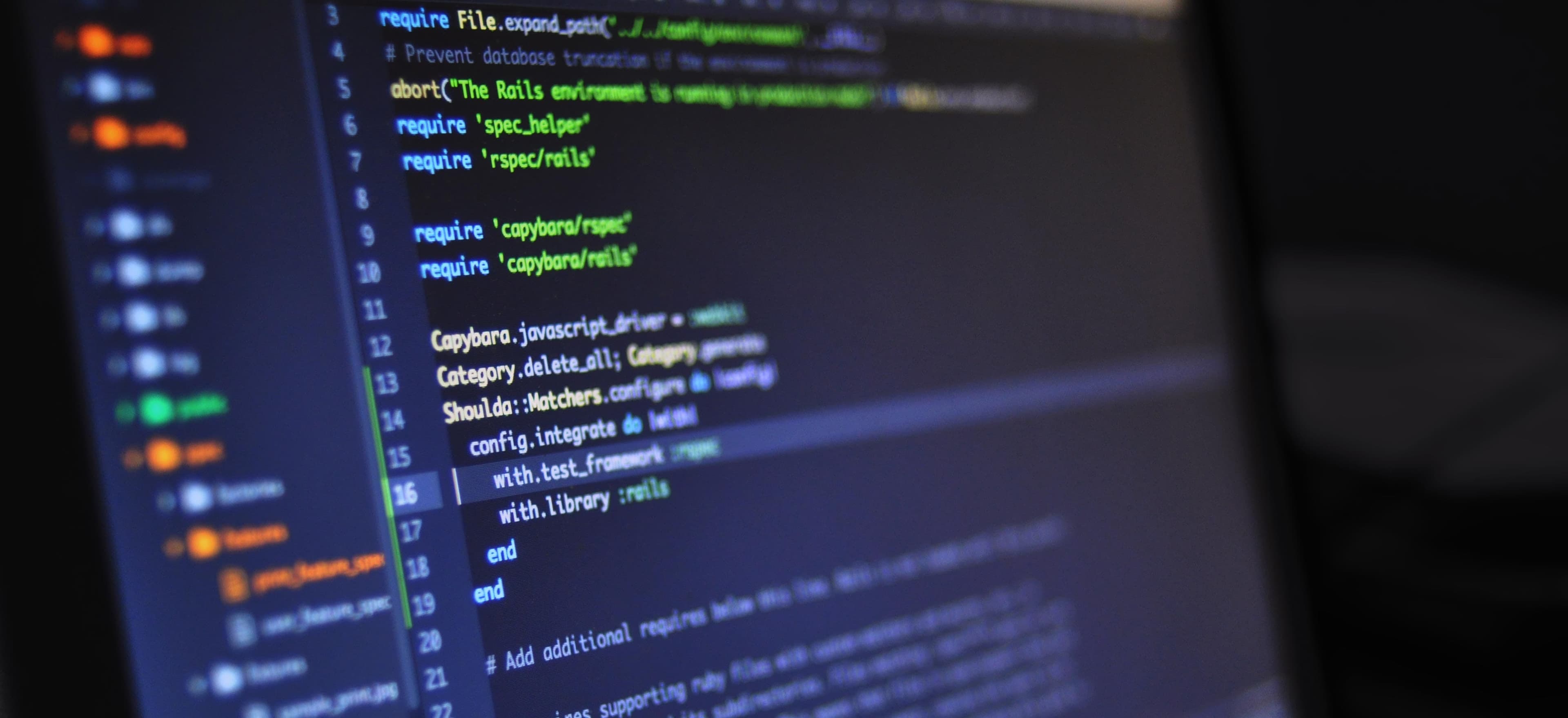
- Published on
Mastering Java Servlets: Common Beginner Mistakes to Avoid
Java Servlets are a fundamental component of Java-based web applications. They provide a standard mechanism to extend server capabilities and handle client requests. While Servlets are powerful, beginners often encounter pitfalls that can lead to frustrating development experiences and performance issues. In this blog post, we will explore common mistakes that developers make when working with Servlets and provide guidance on how to avoid them.
1. Ignoring Servlet Configuration
The Importance of Proper Configuration
One of the first hurdles when working with Servlets is understanding how to configure them properly in web.xml
. Many developers overlook this crucial step and assume that simply creating a Servlet class is enough.
Example Code
<servlet>
<servlet-name>ExampleServlet</servlet-name>
<servlet-class>com.example.ExampleServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>ExampleServlet</servlet-name>
<url-pattern>/example</url-pattern>
</servlet-mapping>
Why This Matters:
Proper configuration ensures that the web container can route incoming requests to the correct Servlet. In this case, requests to /example
will be handled by ExampleServlet
.
Solution
Always define your Servlets in the web.xml
file or use annotations to configure them directly in your code. The use of annotations like @WebServlet
simplifies configuration:
@WebServlet("/example")
public class ExampleServlet extends HttpServlet {
// Servlet implementation
}
2. Failing to Handle Sessions Properly
Session Management Basics
Sessions allow you to maintain user state across multiple requests. A common mistake is to neglect session management or use it incorrectly, leading to unwanted behaviors.
Example Code
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
HttpSession session = request.getSession();
if (session.isNew()) {
session.setAttribute("username", "Guest");
}
// ... further processing
}
Why This Matters: Using sessions effectively ensures user data persists across multiple requests. Failures to manage sessions can lead to unauthorized access or loss of user data.
Solution
Always validate session existence and properly manage session attributes. Avoid storing large objects in sessions, as they can lead to memory leaks.
3. Not Utilizing Context and Initialization Parameters
Understanding Context Parameters
Servlets can gain access to context parameters defined in the web.xml
file. Beginners often forget to leverage this feature, thereby hardcoding values in their code.
Example Code
<context-param>
<param-name>appName</param-name>
<param-value>MyApp</param-value>
</context-param>
In the Servlet:
String appName = getServletContext().getInitParameter("appName");
Why This Matters: Using context parameters makes your application more flexible and easier to maintain. This allows you to change configuration without modifying the actual code.
Solution
Utilize initialization parameters to keep configuration centralized and clean. This practice reduces the need for changes in your source code for simple configuration updates.
4. Poor Exception Handling Practices
Understanding Exceptions
Another common mistake is a lack of proper exception handling in Servlets. This can lead to uninformative error messages and a poor user experience.
Example Code
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
try {
// Possible risky operation
} catch (Exception e) {
log("Exception caught in ExampleServlet", e);
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, "An error occurred");
}
}
Why This Matters: Effective exception handling improves the application's robustness and helps trace issues quickly through logging without exposing sensitive information to users.
Solution
Always use try-catch blocks in your Servlets to handle potential exceptions gracefully. Use logging frameworks like SLF4J or Log4j for better logging practices.
5. Blocking the Main Thread
Understanding Thread Management
Servlets operate in a multi-threaded environment, and blocking the main thread can lead to performance bottlenecks. A beginner error is the use of long-running tasks directly within a Servlet's request-handling method.
Example Code
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Long-running task blocking the thread
for (int i = 0; i < 10; i++) {
// simulate long process
}
}
Why This Matters: Blocking a thread can make the application unresponsive to other requests, leading to a poor user experience and increased load on the server.
Solution
For long-running tasks, consider using separate threads or asynchronous processing. The @Asynchronous
annotation from Java EE can help with that:
@Asynchronous
public Future<String> longRunningTask() {
// perform long-running operation
}
6. Not Closing Resources
Resource Management Basics
Creating database connections, file streams, or other resources without properly closing them can lead to resource leaks. This is a frequent issue for beginners.
Example Code
Connection connection = null;
try {
connection = dataSource.getConnection();
// Use connection
} catch (SQLException e) {
e.printStackTrace();
} finally {
if (connection != null) {
connection.close();
}
}
Why This Matters: Neglecting to close resources can exhaust your system's resource limits, resulting in application crashes or degraded performance.
Solution
Always use finally blocks or try-with-resources statements to ensure resources are closed. This is essential for any database or I/O operations in your Servlets.
try (Connection connection = dataSource.getConnection()) {
// Use connection
} catch (SQLException e) {
e.printStackTrace();
}
A Final Look
Mastering Java Servlets requires understanding not just how to write code but also how to configure it, manage resources, and handle user sessions properly. Avoiding the mistakes discussed in this article will lead to a more robust and maintainable web application.
For further reading on Servlets and their lifecycle, you might find the Java EE Documentation helpful. Additionally, if you're looking to dive deeper into best practices, the Servlet Tutorial on Baeldung can offer a comprehensive guide.
By keeping these best practices in mind, you can streamline your development process and build web applications confidently. Happy coding!