Common HashMap Pitfalls That Every Java Developer Should Avoid
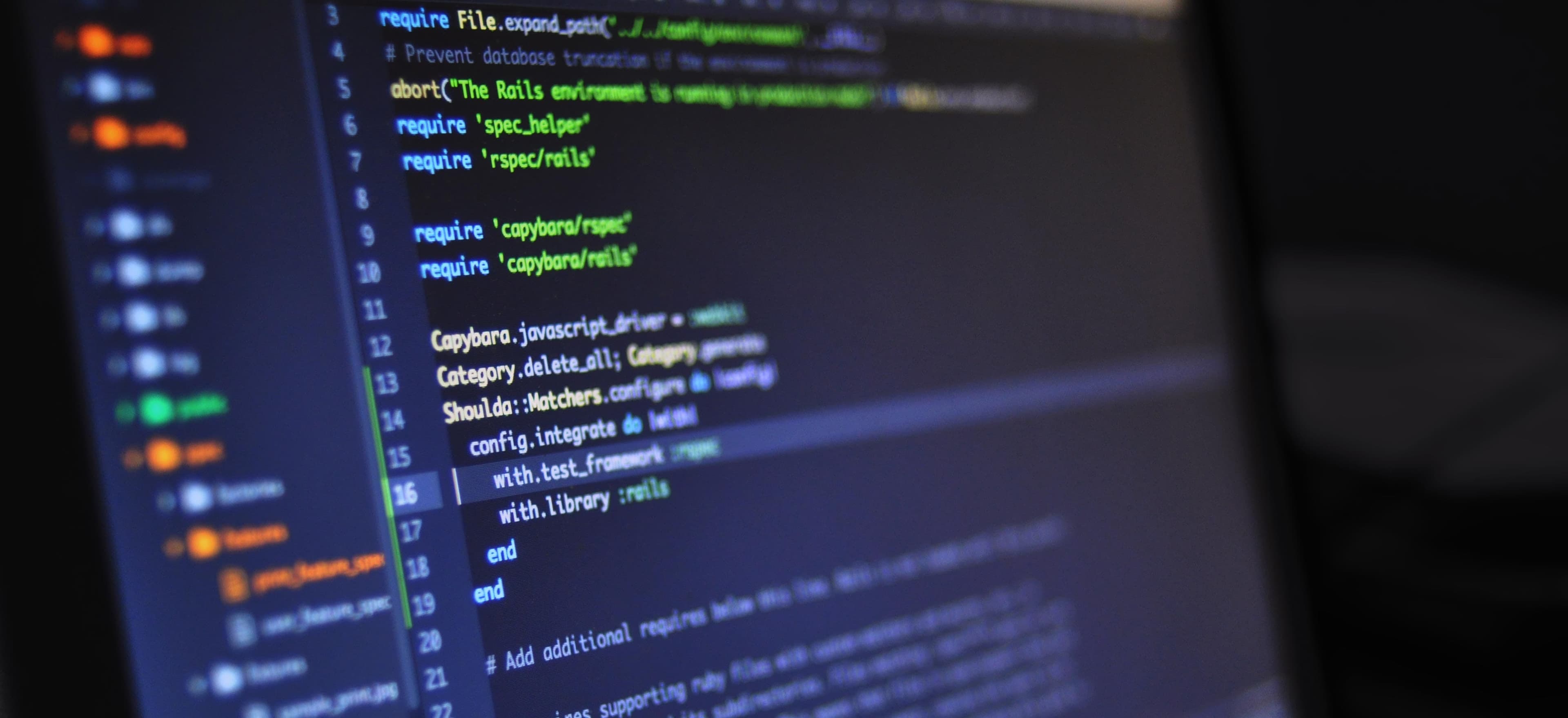
- Published on
Common HashMap Pitfalls That Every Java Developer Should Avoid
Java's HashMap
is one of the most widely used data structures due to its efficient performance in storing key-value pairs. However, it is crucial to understand its intricacies, as improper use can lead to subtle bugs that are hard to trace. In this blog post, we will explore some common pitfalls that every Java developer should avoid while working with HashMap
. We’ll provide code examples and explanations to illustrate the potential issues and best practices to handle them effectively.
What is a HashMap?
A HashMap
is part of Java's Collections Framework and implements the Map
interface. It stores entries as a collection of key-value pairs and allows for constant-time performance (O(1)) for basic operations such as get and put, under ideal conditions.
1. Using Mutable Objects as Keys
One of the most common pitfalls is using mutable objects as keys in a HashMap
. When a mutable object is modified after it has been added to the map, it may lead to unpredictable behavior, primarily because the hash code for the key changes.
The Issue
Consider the following example where we use a mutable object as a key:
import java.util.HashMap;
class MutableKey {
private String keyPart;
public MutableKey(String keyPart) {
this.keyPart = keyPart;
}
public void setKeyPart(String keyPart) {
this.keyPart = keyPart;
}
@Override
public int hashCode() {
return keyPart.hashCode();
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof MutableKey)) return false;
return this.keyPart.equals(((MutableKey) obj).keyPart);
}
}
public class Main {
public static void main(String[] args) {
HashMap<MutableKey, String> map = new HashMap<>();
MutableKey key1 = new MutableKey("Key1");
map.put(key1, "Value1");
System.out.println("Before change: " + map.get(key1)); // Prints "Value1"
key1.setKeyPart("ChangedKey1");
System.out.println("After change: " + map.get(key1)); // Prints null, unexpected behavior
}
}
Explanation
In this example, changing the state of key1
after inserting it into the HashMap
causes it to lose its association with "Value1." Because the hashCode
and equals
methods depend on the keyPart
, modifying it invalidates the original mapping.
Best Practice
Always use immutable objects as keys in a HashMap
. In the example above, if MutableKey
was designed to be immutable, this issue would not arise. Here’s how to make it immutable:
class ImmutableKey {
private final String keyPart;
public ImmutableKey(String keyPart) {
this.keyPart = keyPart;
}
// No setters, only getters for keyPart
}
2. Not Overriding equals
and hashCode
When creating custom objects to use as keys, it is essential to override both equals
and hashCode
correctly. Failing to do so can lead to erratic behavior when retrieving values from the Map
.
The Importance of hashCode and equals
This example illustrates what happens when you don’t override these methods:
class CustomKey {
private String id;
public CustomKey(String id) {
this.id = id;
}
}
public class Main {
public static void main(String[] args) {
HashMap<CustomKey, String> map = new HashMap<>();
CustomKey key1 = new CustomKey("1");
map.put(key1, "Value1");
CustomKey key2 = new CustomKey("1");
System.out.println("Does map contain key2? " + map.containsKey(key2)); // Prints false
}
}
Explanation
In the example, key1
and key2
are distinct objects in memory, and without implementing equals
and hashCode
, the HashMap
cannot determine that they are semantically equivalent.
Best Practice
Always override equals
and hashCode
when your keys are custom objects. Ensure they are consistent; both methods must adhere to their contracts:
@Override
public int hashCode() {
return id.hashCode();
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof CustomKey)) return false;
return this.id.equals(((CustomKey) obj).id);
}
3. Poor Initial Capacity and Load Factor
When initializing a HashMap
, choosing the right initial capacity and load factor can significantly affect performance. The default initial capacity is 16 with a load factor of 0.75.
The Issue
If you expect your HashMap
to grow beyond the default capacity, it may be beneficial to set a higher initial capacity to prevent multiple rehash operations.
Example
public class Main {
public static void main(String[] args) {
HashMap<Integer, String> map = new HashMap<>(10, 0.75f);
for (int i = 0; i < 20; i++) {
map.put(i, "Value" + i);
}
}
}
Explanation
In this snippet, the HashMap
is initialized with a specific capacity to accommodate a larger number of entries upfront, reducing the need to resize as new entries are added.
Best Practice
Estimate your HashMap
size and initialize with that capacity to enhance performance. Utilize the constructor as shown above.
4. Ignoring Synchronization Issues
HashMap
is not synchronized, which means that it is not thread-safe. If multiple threads access the same map concurrently, you can face data inconsistency or a ConcurrentModificationException
.
The Solution
You can use Collections.synchronizedMap()
to create a thread-safe wrapper around your HashMap
:
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<Integer, String> synchronizedMap = Collections.synchronizedMap(new HashMap<>());
synchronizedMap.put(1, "Value1");
synchronizedMap.put(2, "Value2");
synchronized (synchronizedMap) {
for (Integer key : synchronizedMap.keySet()) {
System.out.println("Key: " + key + ", Value: " + synchronizedMap.get(key));
}
}
}
}
Explanation
Using this approach ensures that all accesses to the map are synchronized, preventing inconsistent states or exceptions across threads.
Best Practice
Consider using ConcurrentHashMap
when dealing with high concurrency environments for better performance:
import java.util.concurrent.ConcurrentHashMap;
public class Main {
public static void main(String[] args) {
ConcurrentHashMap<Integer, String> concurrentMap = new ConcurrentHashMap<>();
concurrentMap.put(1, "Value1");
concurrentMap.put(2, "Value2");
concurrentMap.forEach((key, value) -> {
System.out.println("Key: " + key + ", Value: " + value);
});
}
}
Key Takeaways
While HashMap
is undeniably a powerful tool in the Java Collection Framework, it is essential to use it carefully. Avoiding the pitfalls mentioned above, such as using mutable keys, failing to override equals
and hashCode
, not considering initial capacity and load factor, and ignoring synchronization issues, will protect your applications from common bugs.
To learn more about Java Collections and explore additional topics, check out the official Java documentation and the Oracle Java Tutorials.
By adhering to these best practices, you will ensure that your use of HashMap
is efficient and error-free, making your Java development experience much smoother. Happy coding!