Overcoming Latency Issues in Real-Time Message Systems
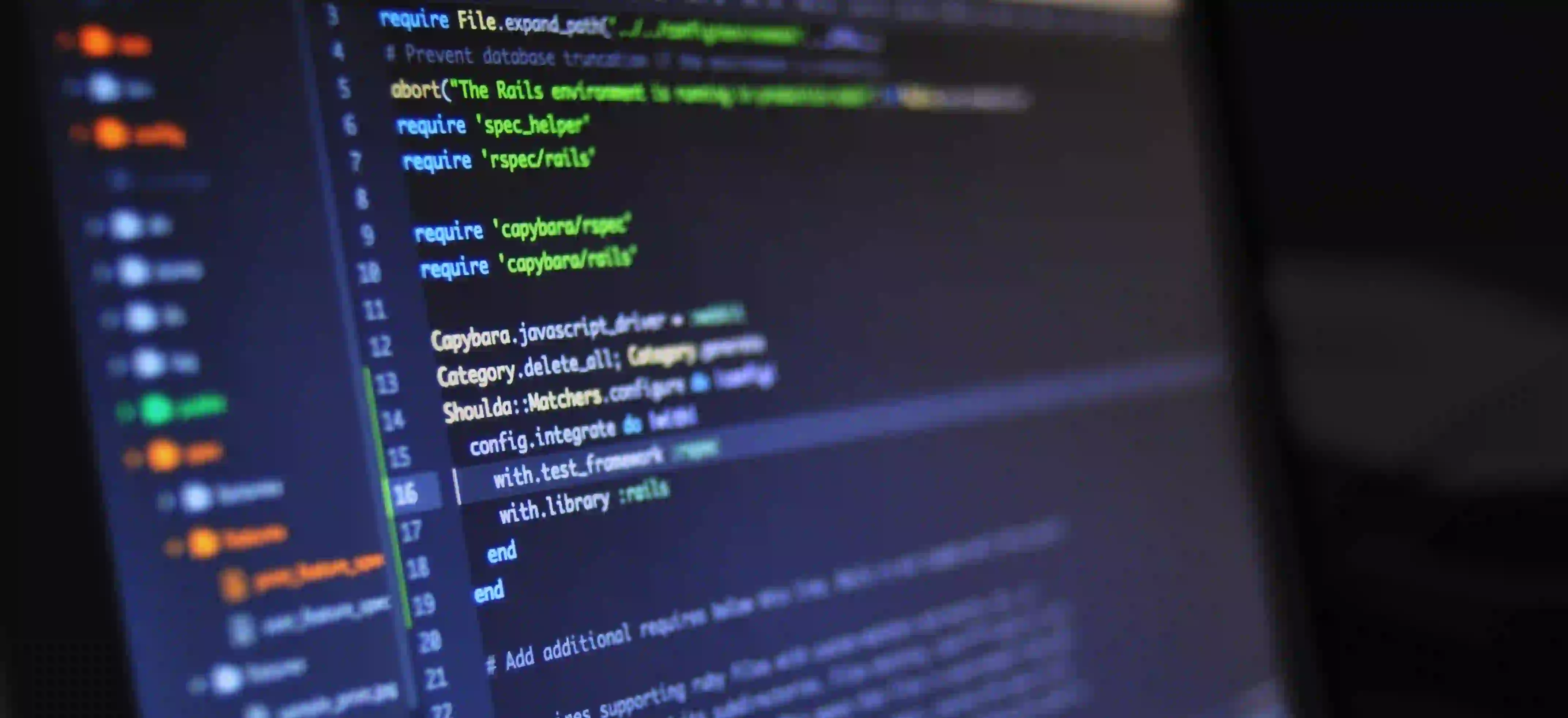
Overcoming Latency Issues in Real-Time Message Systems
In an era where instant communication is paramount, real-time messaging systems must address latency issues effectively. This challenge is particularly pressing in applications such as chat applications, gaming, and financial trading where every millisecond counts. This blog post delves into the causes of latency, discusses the impact it has on real-time messaging systems, and presents strategies and solutions to address these latency problems.
What Causes Latency in Real-Time Messaging?
Latency is the time taken for a message to be sent from the sender to the receiver. Several factors contribute to this delay:
- Network Congestion: Heavy traffic can slow down data transmission.
- Server Response Time: The time it takes for a server to process a request can introduce delays.
- Protocol Overhead: Communication protocols with heavy overhead can slow down message delivery.
- Data Serialization: Converting data into a format suitable for transmission can add to latency.
- Client-Side Processing: If the client's CPU is busy or inefficient, rendering and processing may take longer.
The Impact of Latency
The effects of high latency extend beyond just a minor delay. Here are several consequences of poor latency in real-time messaging systems:
- User Experience: High latency can frustrate users, leading to disengagement.
- Data Integrity: Delayed messages may result in timing issues, risking data integrity, especially in applications like trading where timing is critical.
- Competitive Disadvantage: In a fast-paced digital world, low latency can be a distinguishing factor. Businesses that fail to address it risk losing users.
Strategies to Overcome Latency
Now that we understand what causes latency and its implications, let's explore some strategies to mitigate these issues.
1. Optimize Network Protocols
Choosing the right network protocol can make a significant difference in latency. For instance, using WebSockets can reduce overhead effectively when compared to traditional HTTP requests.
import org.java_websocket.client.WebSocketClient;
import org.java_websocket.handshake.ServerHandshake;
import java.net.URI;
public class MyWebSocketClient extends WebSocketClient {
public MyWebSocketClient(URI serverUri) {
super(serverUri);
}
@Override
public void onOpen(ServerHandshake handshakedata) {
System.out.println("Connected to server");
// Send initial message upon connection
send("Hello Server!");
}
@Override
public void onMessage(String message) {
// Handle incoming messages promptly
System.out.println("Received: " + message);
}
@Override
public void onClose(int code, String reason, boolean remote) {
System.out.println("Disconnected from server");
}
@Override
public void onError(Exception ex) {
ex.printStackTrace();
}
}
Why Use WebSockets?
- It maintains a persistent connection, which reduces the time spent setting up new connections.
- Message format can be smaller due to the absence of HTTP headers, enabling faster communication.
2. Implement Edge Computing
Utilizing edge computing helps minimize latency by processing data closer to the source of generation, rather than relying on a central server.
- Example with a Cloud Service Provider: If messages generated in New York are routed to servers in California, latency increases. Instead, processing them through a service like AWS Lambda at the edge can drastically cut down the time between sending and receiving.
3. Reduce Data Serialization Time
Serialization can be a bottleneck when dealing with complex data structures. Using efficient libraries such as Protobuf or msgpack can minimize serialization time.
Here's a simple Java example using Protobuf:
syntax = "proto3";
package messages;
message ChatMessage {
string sender = 1;
string content = 2;
int64 timestamp = 3;
}
Why Optimize Serialization?
- These libraries are designed for speed and efficiency. This reduces the time spent encoding and decoding messages.
4. Employ Quality of Service (QoS) Strategies
QoS strategies help ensure that messages are delivered in a timely and reliable manner. In systems using MQTT (a lightweight messaging protocol), various levels of QoS can be configured:
- QoS 0: At most once – message delivery is not guaranteed.
- QoS 1: At least once – message delivery is guaranteed, but duplicates can occur.
- QoS 2: Exactly once – ensures no duplicates and is the safest but most resource-intensive.
5. Load Balancing
Implementing load balancing can significantly reduce server response times by distributing incoming requests more evenly.
Here's a simple way to set up a load balancer in Java using a round-robin approach:
import java.util.List;
public class LoadBalancer {
private List<String> servers;
private int currentIndex = -1;
public LoadBalancer(List<String> servers) {
this.servers = servers;
}
public String getNextServer() {
currentIndex = (currentIndex + 1) % servers.size();
return servers.get(currentIndex);
}
}
Why Load Balancing?
- This approach can significantly mitigate high traffic periods, which can compromise performance.
6. Monitor and Analyze
Regular monitoring of your system can help identify potential latency bottlenecks. Tools like New Relic, Datadog, or Prometheus can capture real-time metrics.
- Logging: Instrument your code to log entry and exit times for critical operations. Analyze the logs to pinpoint delays.
Closing Remarks
Latency in real-time messaging systems is a critical issue that can severely affect user experience, data integrity, and business viability. By optimizing network protocols, leveraging edge computing, reducing serialization time, implementing QoS strategies, employing load balancing, and actively monitoring the system, you can greatly reduce latency.
Additional Resources:
By focusing on these solutions, organizations can create responsive, efficient, and reliable real-time messaging systems that meet the demands of today's fast-paced digital world.