Why Static Analysis Tools Often Miss Real Development Issues
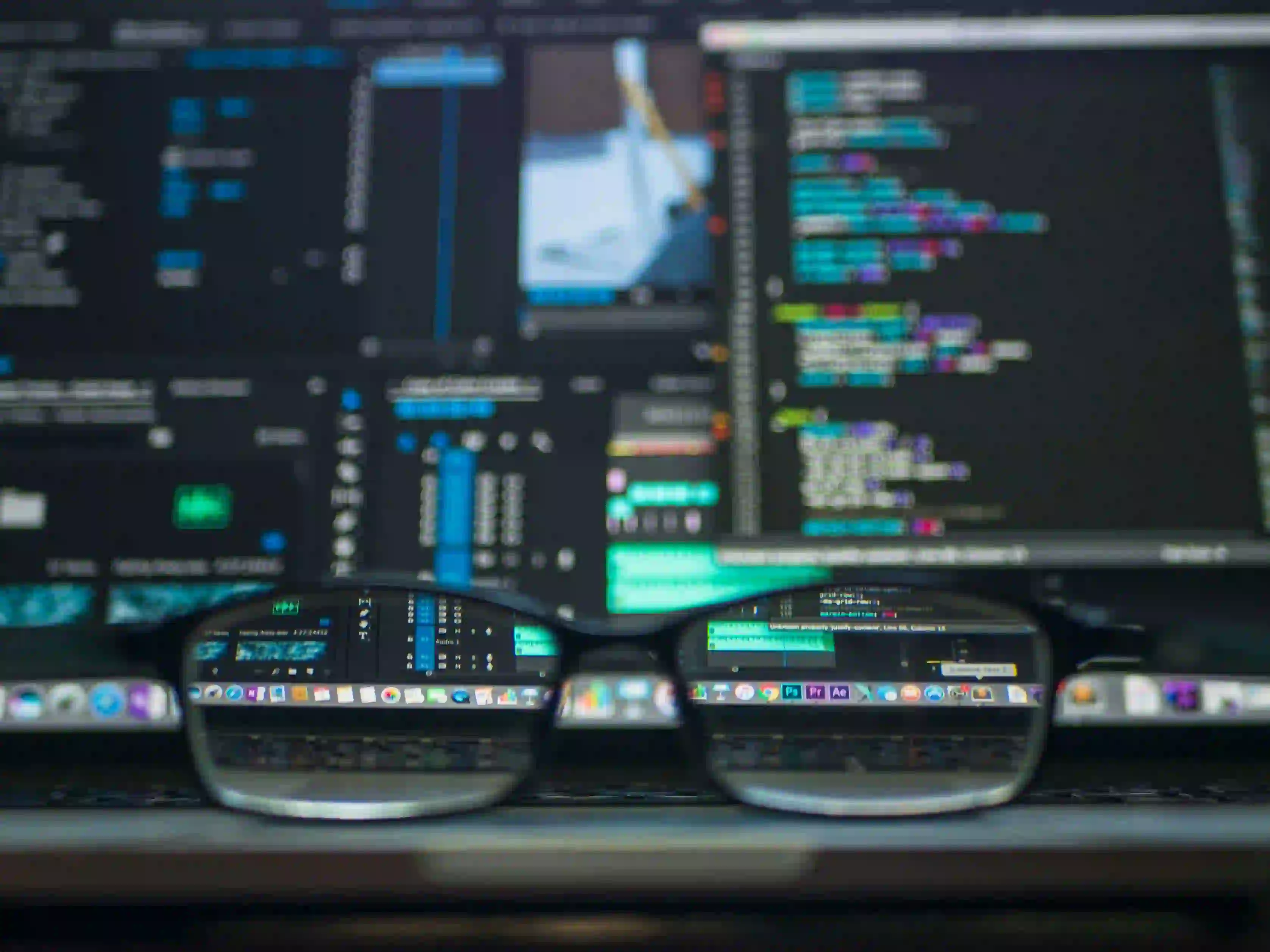
Why Static Analysis Tools Often Miss Real Development Issues
In the landscape of software development, static analysis tools (SATs) have gained significant popularity for their ability to automate code reviews and identify potential issues before code reaches production. However, while these tools are invaluable, they also have their limitations. In this blog post, we will delve into why static analysis tools often miss real development issues, explore the implications of these shortcomings, and suggest measures to enhance your development practices.
What is Static Analysis?
Static analysis is the process of examining code without executing it. Tools like SonarQube, Checkstyle, and FindBugs analyze source code against a set of defined rules to uncover vulnerabilities, code smells, and potential bugs. The primary benefit of SATs is their ability to efficiently scrutinize large codebases, enabling developers to identify issues early in the software development life cycle (SDLC).
Why Use Static Analysis Tools?
Before we explore the limitations, let’s summarize the benefits of static analysis:
- Early Detection: Issues can be caught before the code is deployed.
- Cost-Effectiveness: Fixing bugs early can save time and cost significantly.
- Coding Standard Compliance: Tools help maintain consistent coding standards across teams.
- Non-Intrusive: Static analysis can be integrated into the CI/CD pipeline seamlessly.
Despite these advantages, static analysis tools often miss real development issues for various reasons.
Limitations of Static Analysis Tools
-
False Positives and Negatives
Static analysis tools are notorious for generating false positives—alerts for issues that don't actually exist. Conversely, they sometimes miss false negatives—real issues that require attention. These inaccuracies stem from the tool's limited understanding of the business logic and context in which the code operates.
Example:
☕snippet.javapublic void doSomething(int value) { if (value > 0) { System.out.println("Processing: " + value); } }
A static analysis tool might flag that the
value
variable is not checked for being negative, even though the business context guarantees that it will always be positive at runtime.Why It Matters:
Developers may spend time chasing down phantom issues instead of focusing on genuine concerns—distracting from productivity and innovation.
-
Lack of Context Awareness
Static analysis tools generally possess limited knowledge of the application context. They analyze code at a structural level without understanding its purpose or related functionality. This lack of contextual awareness often leads to missed bugs.
Example:
☕snippet.javaint calculateDiscount(int price) { if (price < 0) { throw new IllegalArgumentException("Price cannot be negative"); } return price <= 100 ? 0 : 10; }
A static analyzer may not catch the possible logical error related to how discounts are computed based on various conditions because it doesn't infer the business rules associated with the price parameters.
Why It Matters:
Code is written to solve business problems, not merely to meet structural requirements. Failing to appreciate context can hinder the identification of genuine problems.
-
Difficulty in Detecting Runtime Issues
Static analysis tools analyze the code statically, which means they won't spot runtime issues, such as concurrency problems, memory leaks, or performance bottlenecks. These issues require dynamic analysis or real-time observation during the application’s lifecycle.
Example:
☕snippet.javapublic synchronized void addElement(List<Integer> list, int element) { list.add(element); }
While the code is syntactically correct and may pass static checks, it could lead to a concurrency problem when multiple threads invoke
addElement
on the same list—something static analysis cannot identify.Why It Matters:
Focusing solely on static analysis might create a false sense of security, leading to missing critical runtime behavior issues that could impact application stability.
-
Limited Coverage of Coding Standards
Static analysis tools are generally rule-based, which means they rely on predefined patterns and practices. If a project diverges from these standards, there might be serious issues that evade detection.
Example:
☕snippet.javapublic void updateUser(User user) { if (user.getId() == null) { System.out.println("User ID is not set!"); return; } // Assume more update logic }
If the coding standards defined in the static analyzer do not cover adequate null checks on custom objects, critical issues could remain undetected.
Why It Matters:
Relying too heavily on prescribed standards can overlook the unique requirements of a project or an organization's expectations.
-
Insufficient Rules and Configurations
A static analysis tool might not cover the full spectrum of issues because of insufficient or poorly configured rules. Misconfigured rules can lead to missed vulnerabilities or generate unnecessary noise.
Example:
☕snippet.javapublic void connectToDatabase(String dbUrl) { // Missing error handling Connection conn = DriverManager.getConnection(dbUrl); }
If the static analysis tool isn't configured to look for unhandled exceptions, the above code could slip through unnoticed, potentially causing runtime failures.
Why It Matters:
A lack of tailored rules that suit individual project requirements decreases the effectiveness of static analysis.
Enhancing Development Practices Beyond Static Analysis
-
Combine Static and Dynamic Analysis
To get a comprehensive view of your application's health, integrate static analysis with dynamic analysis tools. Dynamic analysis gives you insights while the application runs, helping catch runtime errors.
- Tools such as JProfiler and VisualVM can uncover performance issues and memory leaks.
- Automated testing frameworks like JUnit and TestNG should be utilized extensively to cover edge cases.
-
Code Reviews and Pair Programming
Peer reviews and pair programming practices allow developers to assess each other's code and bring different perspectives into the conversation. This human touch often catches issues that automated tools overlook.
-
Emphasize Test-Driven Development (TDD)
Adopting TDD can focus the development on behavior first rather than structure. Writing tests for your code while developing can prevent many common issues from developing in the first place.
- Encourages a mindset of quality and thoroughness.
- Ensures that all code paths are tested.
-
Lifelong Learning and Best Practices
It is crucial for development teams to stay updated on best practices and current tools in the industry. Regular training sessions and workshops can foster a culture of continuous improvement.
A Final Look
Static analysis tools serve as essential instruments in a developer's toolkit, but they are not foolproof. Understanding their limitations allows your team to devise better solutions for identifying real development issues. By combining static analysis with dynamic techniques, code reviews, and best practices, you can cultivate higher code quality and improved application reliability.
For further reading on the integration of static and dynamic analysis, explore SonarQube Documentation and Continuous Testing Best Practices. These resources provide insights into adopting a blend of practices that can improve your code quality significantly.
By leveraging these insights, your development approach can evolve into a more holistic practice that recognizes both the strengths and limitations of static analysis tools, ultimately leading to better software outcomes.