Unlocking Performance: Tackling Distributed Cache Inefficiencies
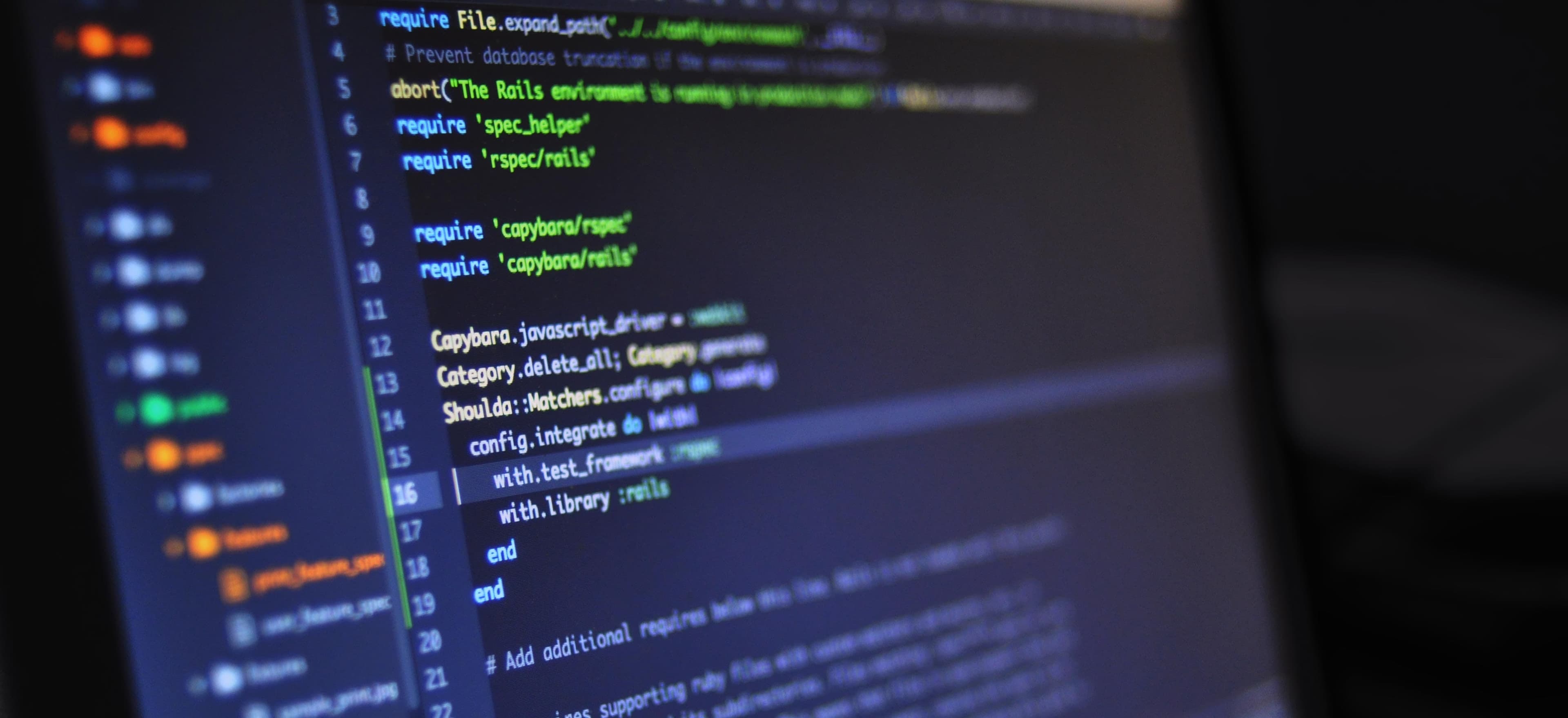
- Published on
Unlocking Performance: Tackling Distributed Cache Inefficiencies
In today's world of cloud computing and distributed systems, performance optimization has never been more crucial. One of the most significant bottlenecks in scaling applications is often tied to data management. Distributed caches are commonly employed to enhance performance and increase application scalability. However, poor management and inefficient use of these caches can lead to several issues that hinder their effectiveness.
In this blog post, we will explore the intricacies of distributed caches, common inefficiencies, and practical solutions to enhance performance. By the end of this article, you'll have a better understanding of how to unlock the full potential of distributed caching in your Java applications.
What is Distributed Caching?
Before diving into inefficiencies, let’s define what distributed caching is. A distributed cache is an in-memory data store that allows applications to access data quickly across a cluster of servers. This approach improves performance by storing frequently accessed data in a temporary storage area, reducing the need to repeatedly fetch data from slower storage like databases.
Benefits of Distributed Caching
- Reduced Latency: Accessing data in memory is significantly faster than hitting the database.
- Scalability: Distributing cache across multiple nodes allows the system to handle more load.
- Fault Tolerance: In most distributed cache systems, data can be replicated, ensuring availability even if a node fails.
Common Inefficiencies in Distributed Caches
While distributed caches offer remarkable benefits, they come with their own set of inefficiencies. Here are some common pitfalls:
1. Cache Stampede
What is it? A cache stampede occurs when multiple requests for the same data hit the cache simultaneously, often leading to a flood of requests to the underlying data source.
How to Tackle It
Use a locking mechanism or a single-entry-cache approach. Here’s how to do it in Java.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
import java.util.HashMap;
import java.util.Map;
public class Cache {
private final Map<String, Object> cache= new HashMap<>();
private final Lock lock = new ReentrantLock();
public Object get(String key) {
// Check if the cache contains the value
if (cache.containsKey(key)) {
return cache.get(key);
}
// Acquire lock to prevent stampede
lock.lock();
try {
// Re-check cache to avoid redundant data fetching
if (!cache.containsKey(key)) {
// Simulate data retrieval from a slow data source
Object value = fetchDataFromDataSource(key);
cache.put(key, value);
}
return cache.get(key);
} finally {
lock.unlock();
}
}
private Object fetchDataFromDataSource(String key) {
// Placeholder for slow database fetch
return "Data for " + key;
}
}
In this code snippet, the get
method checks if the data is in cache. If not, it locks the process until the data is fetched, reducing the risk of multiple calls flooding the data source.
2. Cache Eviction Policies
Cache eviction policies determine how data is removed when the cache reaches its limit. If poorly implemented, effective data can be removed from the cache, leading to increased latency.
How to Tackle It
Choose the right eviction policy based on your application needs (e.g., Least Recently Used - LRU, Least Frequently Used - LFU).
import java.util.LinkedHashMap;
import java.util.Map;
public class LRUCache<K, V> extends LinkedHashMap<K, V> {
private final int capacity;
public LRUCache(int capacity) {
super(capacity, 0.75f, true);
this.capacity = capacity;
}
@Override
protected boolean removeEldestEntry(Map.Entry<K, V> eldest) {
return size() > capacity;
}
public V getCacheValue(K key) {
return get(key);
}
public void addCacheValue(K key, V value) {
put(key, value);
}
}
In this example, LRUCache
extends LinkedHashMap
and utilizes an override of removeEldestEntry
, ensuring that when the cache reaches its capacity, the least recently accessed entry is evicted. Choosing an appropriate cache eviction strategy ensures that your cache remains efficient over time.
3. Data Serialization Overhead
Serialization and deserialization of objects can be expensive in terms of performance.
How to Tackle It
Opt for efficient serialization techniques such as Protocol Buffers or Kryo, which are faster and more compact compared to standard Java serialization.
import com.esotericsoftware.kryo.Kryo;
import com.esotericsoftware.kryo.io.Input;
import com.esotericsoftware.kryo.io.Output;
public class SerializationUtil {
private static final Kryo kryo = new Kryo();
public static byte[] serialize(Object object) {
Output output = new Output(1000);
kryo.writeClassAndObject(output, object);
return output.toBytes();
}
public static Object deserialize(byte[] bytes) {
Input input = new Input(bytes);
return kryo.readClassAndObject(input);
}
}
In this snippet, the SerializationUtil
class uses Kryo for efficient serialization. Reducing serialization overhead can dramatically speed up data storage and retrieval in your cache.
Implementing Distributed Caching in Java
To effectively use distributed caches, it's important to leverage frameworks designed for scalability and resilience, such as Hazelcast or Redis.
Example of Using Hazelcast
import com.hazelcast.core.Hazelcast;
import com.hazelcast.core.HazelcastInstance;
import com.hazelcast.map.IMap;
public class DistributedCacheExample {
public static void main(String[] args) {
HazelcastInstance hz = Hazelcast.newHazelcastInstance();
IMap<String, String> map = hz.getMap("my-distributed-cache");
map.put("key", "value");
String value = map.get("key");
System.out.println("Value from distributed cache: " + value);
}
}
This code initializes a Hazelcast instance and demonstrates how to put and get data from a distributed cache. Leveraging a robust caching solution like Hazelcast can greatly enhance the performance of your application.
Closing the Chapter
Distributed caching can significantly improve application performance, but it requires a thoughtful approach to mitigate common inefficiencies. By addressing issues like cache stampede, poor eviction policies, and serialization overhead, you can unlock the full power of distributed caching in your Java applications.
For further reading, consider delving into the Hazelcast documentation or exploring Java’s concurrency utilities for more tips on handling distributed systems effectively.
Optimizing distributed caches might seem like a daunting task, but with the right strategies and tools in place, it can immensely enhance your system's efficiency and scalability. Unlock the potential of your applications today!
Checkout our other articles