Common Issues with FindBugs and JSR-305 Annotations
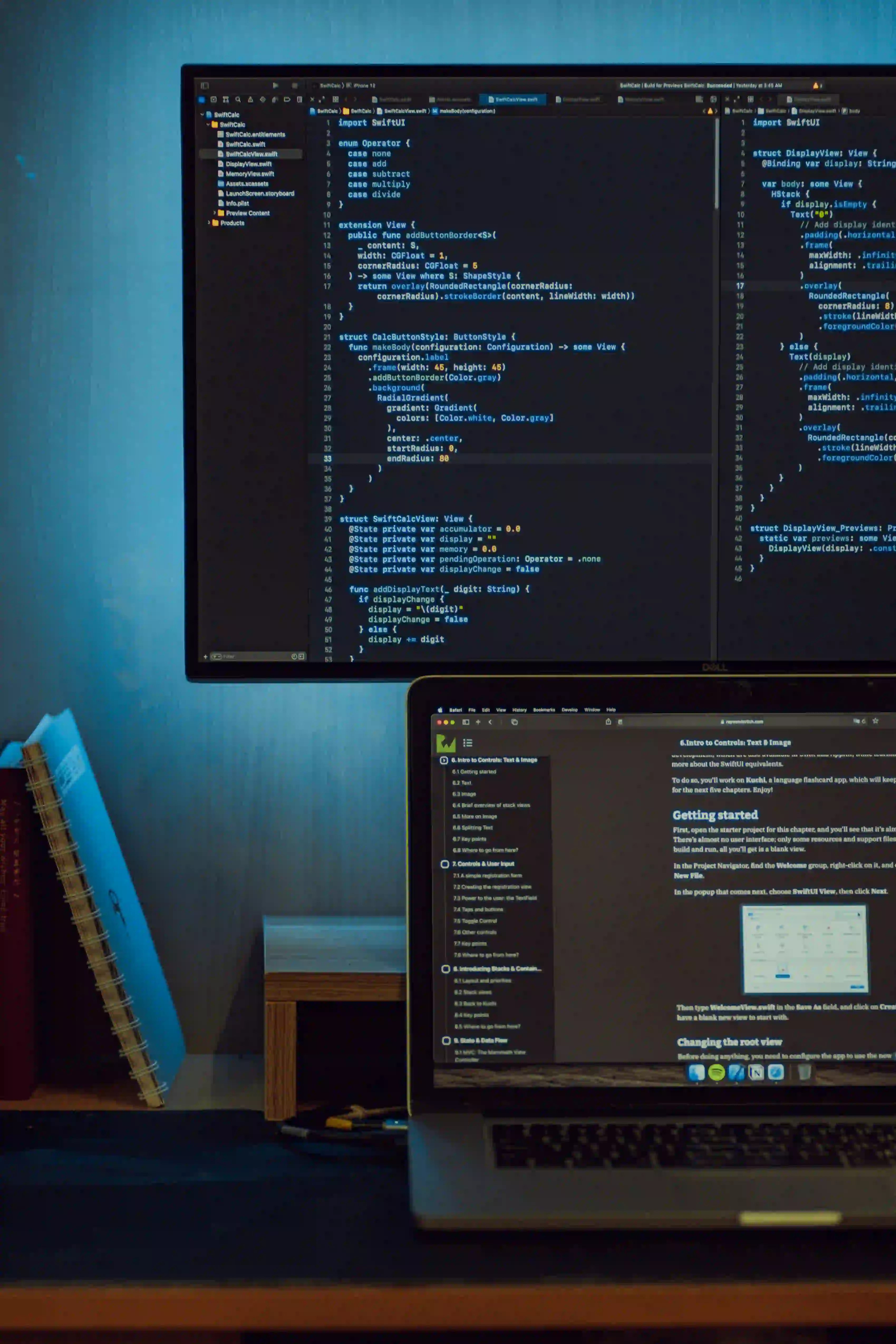
Common Issues with FindBugs and JSR-305 Annotations
When working on Java projects, ensuring code quality is paramount. One of the most effective ways to maintain high-quality code is through static analysis tools like FindBugs. This tool evaluates Java bytecode to identify potential bugs in a codebase. One of its key features is its ability to analyze JSR-305 annotations, which help convey the intended use of API elements, such as methods, constructors, and fields. While FindBugs and JSR-305 annotations offer great benefits, you may encounter a few common issues. In this blog post, we will explore these issues and offer practical solutions.
Understanding JSR-305 Annotations
JSR-305 is a specification that introduces annotations for static code analysis. It enhances Java by providing information about nullability and other properties that can guide developers during code reviews and can greatly improve tooling capabilities.
Key Annotations in JSR-305
- @Nonnull: Indicates that a parameter, return value, or field cannot be null.
- @Nullable: Indicates that a parameter, return value, or field can be null.
- @CheckForNull: Suggests that a value can be null but should be checked before use.
Here's an example of how to use these annotations in your code:
import javax.annotation.Nullable;
import javax.annotation.Nonnull;
public class UserData {
private String username;
public UserData(@Nonnull String username) {
this.username = username;
}
@Nullable
public String getUserData() {
// Simulating a potential null value
return null;
}
}
In this example, the getUserData
method is marked as possible to return null, while the constructor enforces that a valid username must be provided.
Common Issues with FindBugs and JSR-305 Annotations
While FindBugs does a terrific job analyzing code using JSR-305 annotations, some common issues often arise. Below, we will discuss these issues and how to remedy them.
1. Missing Annotations
One of the primary challenges is forgetting to add annotations where they're required. This can lead to false positives in FindBugs, where it flags potential null pointer exceptions.
Solution: Create Coding Guidelines
Establish a set of coding guidelines within your team that encourages all members to use JSR-305 annotations consistently. Implement automated tools that enforce these guidelines. For example, using a linter such as PMD to check for missing annotations.
Here’s a small snippet showing how you can use annotations properly:
import javax.annotation.Nonnull;
public class UserService {
public void createUser(@Nonnull String username) {
// Perform user creation logic
}
}
In this example, if a developer forgets to add the @Nonnull
annotation, it may lead to issues down the line.
2. Incorrect Annotations
Sometimes, the right annotations may not be applied. Mislabeling return types as non-null when they can be null can create hazardous situations.
Solution: Code Reviews
Conduct regular code reviews focusing on proper annotation usage. Utilize FindBugs to identify areas where incorrect annotations might cause issues.
For instance, if a method is annotated incorrectly like:
@Nonnull
public String fetchData() {
return null; // This should not be allowed
}
FindBugs will flag this as an issue, prompting the developer to revise their annotations.
3. Not Configuring FindBugs Properly
In some instances, developers simply do not configure FindBugs to recognize the annotations properly. This might lead to FindBugs missing many potential issues.
Solution: Configuration Adjustments
Ensure that you include the proper libraries in your build tool. For projects using Maven, include JSR-305:
<dependency>
<groupId>com.google.code.findbugs</groupId>
<artifactId>jsr305</artifactId>
<version>3.0.1</version>
</dependency>
Once FindBugs is rightly configured, it can now utilize JSR-305 annotations effectively.
4. Ignoring Warnings
Developers might choose to ignore FindBugs warnings related to JSR-305 annotations due to perceived complexity or time constraints. This can lead to several unresolved issues that compound over time.
Solution: Prioritize Warnings
Set up your development process to prioritize these warnings. Use CI/CD pipelines to fail builds when certain critical issues, like incorrect annotation usage, are found.
FindBugs allows you to categorize warnings by severity, helping your team to focus on critical issues first.
5. Overlapping Tool Compatibility
Some developers may use multiple static analysis tools, and these tools could potentially conflict regarding how to handle JSR-305 annotations.
Solution: Choose Compatible Tools
When selecting additional static analysis tools, ensure they are compatible with JSR-305 annotations. Tools like SonarQube and Checkstyle can work seamlessly alongside FindBugs.
Here's a simple configuration for integrating FindBugs:
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>findbugs-maven-plugin</artifactId>
<version>3.0.5</version>
<configuration>
<failOnError>true</failOnError>
<xmlOutput>true</xmlOutput>
</configuration>
</plugin>
This setup will ensure you're aware of potential annotation issues within your code and maintain the overall quality.
To Wrap Things Up
Using FindBugs alongside JSR-305 annotations can dramatically increase the quality of your code, but it is essential to address common issues that might arise from their integration. By making conscious efforts to include necessary annotations, ensuring proper tool configuration, and implementing a proper review process, you can collaborate continuously towards a robust and maintainable codebase.
To explore more about programming with Java and improve your skills, you may find Oracle's official Java documentation helpful as a resource.
In conclusion, the synergy between FindBugs and JSR-305 annotations stands to elevate the quality of any Java project. Tackle these common challenges, and you will be on the path to creating some truly excellent software.
Stay tuned for more Java insights!