Common Pitfalls When Crawling Websites with Selenide
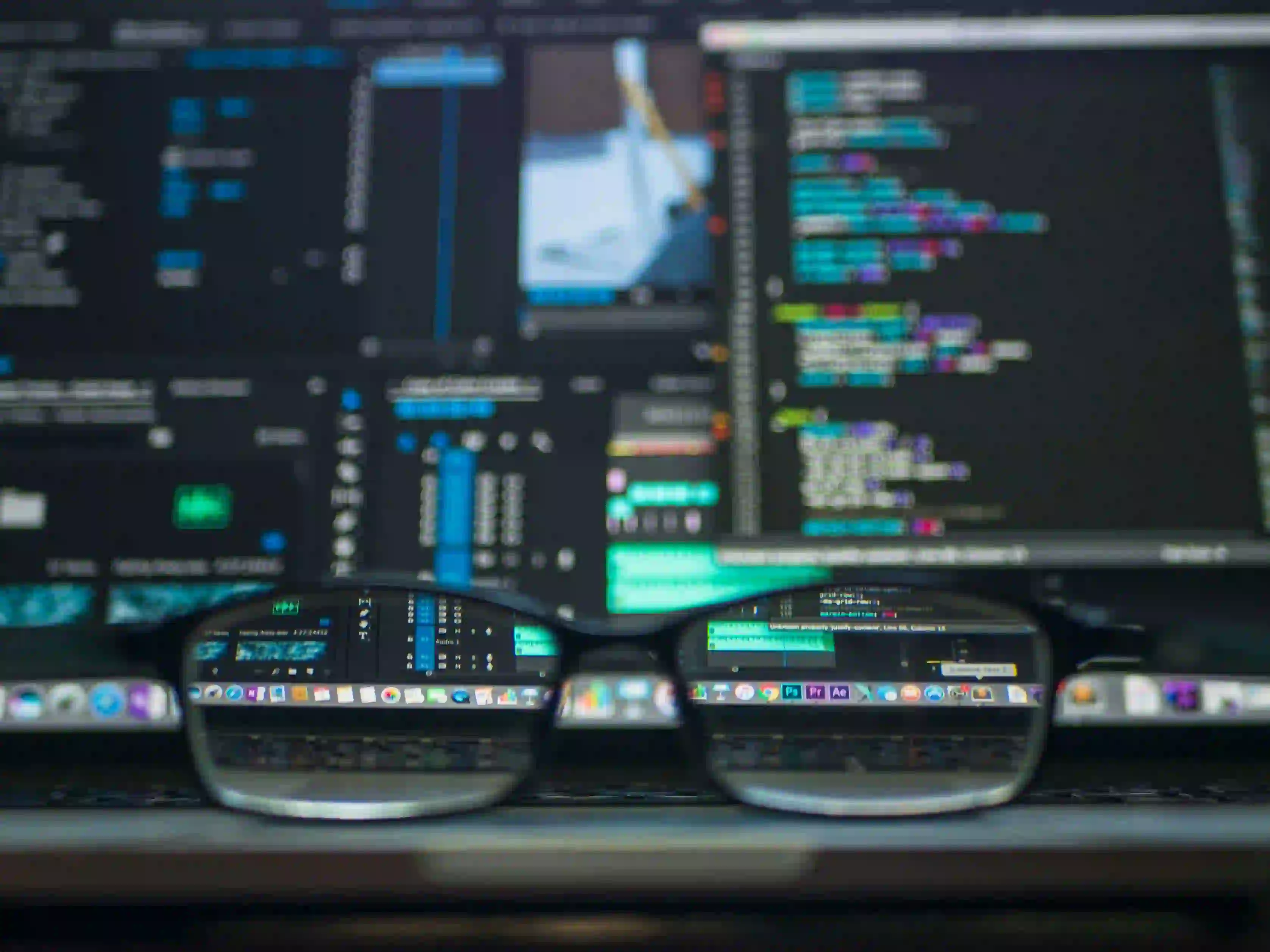
Common Pitfalls When Crawling Websites with Selenide
Web crawling has become an invaluable tool for developers and data analysts seeking to extract information from websites. While there are numerous frameworks available for web crawling, Selenide stands out due to its simplicity and ease of use, especially for those familiar with Selenium. In this blog post, we will discuss common pitfalls when using Selenide for web crawling and how to avoid them.
What is Selenide?
Before diving into the pitfalls, let's clarify what Selenide is. Selenide is a Java-based tool that simplifies the process of writing automated tests for web applications. It provides a clean API and makes it easy to wait for page elements to load. This asynchronous nature of web applications requires tools that can efficiently handle dynamic content, making Selenide a preferred choice among many developers.
Getting Started with Selenide
If you're new to Selenide, here's a sample snippet to help you set up your first crawling project:
import com.codeborne.selenide.Configuration;
import static com.codeborne.selenide.Selenide.*;
public class SelenideExample {
public static void main(String[] args) {
Configuration.startMaximized = true; // Start maximized for better visibility
open("https://example.com"); // The website to crawl
}
}
This code initializes Selenide, starts the browser in maximized mode, and opens the specified URL. But as you start to crawl websites, be wary of the following pitfalls.
1. Ignoring Dynamic Content
Problem
A common mistake is ignoring the fact that many websites use Ajax or other JavaScript frameworks to load content dynamically. If your crawler does not wait for these elements to load, it may fail to locate the required information.
Solution
Utilize Selenide's built-in waiting capabilities to ensure elements are present before attempting to interact with them.
import static com.codeborne.selenide.Selenide.$;
void crawlExample() {
$("div.content").shouldBe(visible); // Waits for the element to be visible before proceeding
String data = $("div.content").getText();
System.out.println(data);
}
In this code, we instruct Selenide to wait until the div.content
element is visible. This prevents "Element Not Found" exceptions, ensuring that your crawler collects the right data.
2. Not Respecting Robots.txt
Problem
Many web crawlers ignore the robots.txt
file, which specifies the rules for web crawlers. Disregarding these directives can lead to undesirable consequences, including being blocked from the site.
Solution
Before you start your crawl, make it a practice to check the robots.txt
file of the website you intend to crawl.
- For example, visit
https://example.com/robots.txt
to see the site's crawler guidelines.
You can programmatically fetch and respect these guidelines. Using an HTTP client in Java would help you do so efficiently.
3. Hardcoding URLs
Problem
Hardcoding URLs can significantly limit your crawler's roving capability. If you need to crawl multiple pages or different websites, a hardcoded URL will impede your flexibility.
Solution
Use configuration files or command-line arguments for URLs to make your crawler more flexible and reusable.
public static void main(String[] args) {
String url = args.length > 0 ? args[0] : "https://default.com"; // Fallback URL
open(url);
}
In this snippet, we allow the URL to be passed as a command-line argument. If no argument is provided, it defaults to a specified URL, making your crawler adaptable.
4. Not Handling Pagination
Problem
When crawling data-heavy websites, navigation through pagination is often required. Failing to account for pagination means you might miss out on significant data.
Solution
Use a loop to traverse pages until no further pages exist.
void crawlPagination() {
while ($("div.pagination .next").isDisplayed()) {
// Extract data
List<String> items = $$("div.items").texts();
items.forEach(System.out::println); // Print extracted items
// Click the next page
$("div.pagination .next").click();
sleep(2000); // Wait for the next page to load
}
}
This code keeps navigating through the pagination while elements exist, ensuring you gather all data over multiple pages.
5. Performance Issues
Problem
Crawling large websites can lead to performance issues if you are not careful with your approach. Excessive waiting times and excessive requests can lead to your IP being blocked.
Solution
Throttle your requests to mimic human behavior. Include delays between actions and be mindful of the limit of requests sent in a short period.
void crawlWithDelay() {
for (String url : getUrlsToCrawl()) {
open(url);
// Perform your scraping logic
sleep(1000); // Wait 1 second between requests
}
}
By integrating a delay, you create a more human-like interaction with the website, helping you avoid flagging by anti-bot mechanisms.
6. Not Handling Exceptions
Problem
Automation isn't foolproof, and websites can throw unexpected errors. Failing to handle exceptions can lead your crawler to stop working entirely.
Solution
Implement a basic try-catch block in your code to manage exceptions gracefully.
void crawlSafely() {
try {
open("https://example.com");
// Scrape data
} catch (Exception e) {
System.err.println("Failed to crawl: " + e.getMessage());
}
}
In this snippet, we capture any exceptions that arise during the crawling process, logging them to ensure we know what went wrong without halting the entire process.
In Conclusion, Here is What Matters
Crawling websites with Selenide can be an incredibly powerful and efficient way to extract data. However, pitfalls are prevalent, especially for newcomers. By avoiding the common mistakes discussed above — like ignoring dynamic content, disregarding robots.txt
, hardcoding URLs, and more — you can create a robust web crawler that performs reliably.
For further insights, you may want to explore the Selenide official documentation here and learn more about web scraping ethics and best practices from resources such as Scrapinghub.
Arming yourself with the knowledge of both pitfalls and effective solutions, you can successfully navigate the world of web crawling and extract the valuable data your projects need. Happy crawling!