Streamline Your Integration Tests Using WireMock Effectively
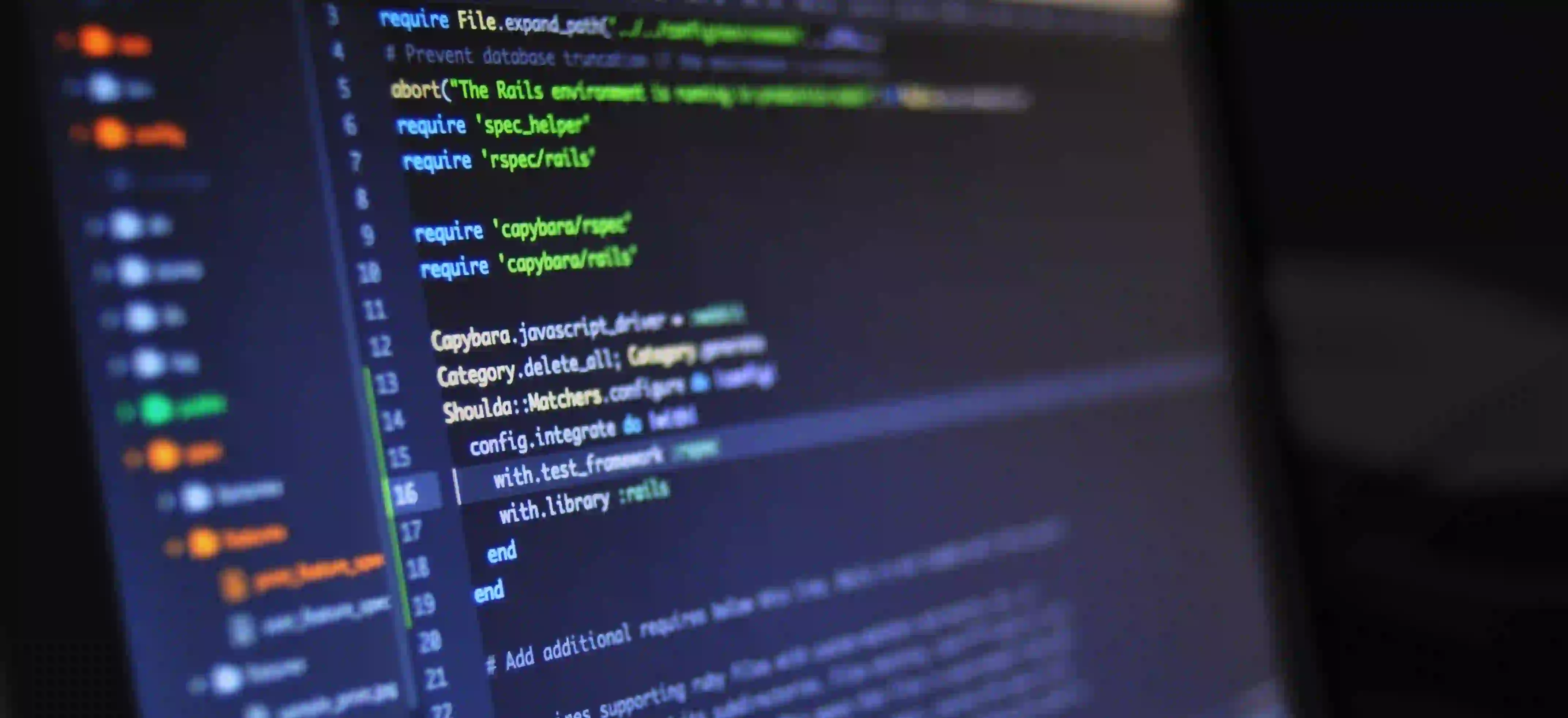
Streamline Your Integration Tests Using WireMock Effectively
Integration testing is a vital aspect of software development, ensuring that components of your application work together as expected. However, it can often be cumbersome and time-consuming, especially when it comes to dealing with external dependencies like APIs. This is where WireMock comes into play.
WireMock is a tool for mocking web services. It allows you to create a simulation of web services that your application interacts with, helping you to test your integration scenarios without the reliability issues of using the actual dependencies. In this blog post, we will delve into the features of WireMock, demonstrate how to set it up, and provide examples of how to leverage it for streamlined integration tests.
What is WireMock?
WireMock is an open-source tool that simulates HTTP-based APIs. It acts as a stand-in for a web service that your application may rely on, ensuring your tests are:
- Fast: No network calls mean quicker test executions.
- Reliable: Tests won't fail due to external service downtime or changes.
- Controlled: You can simulate various responses, including failures, without modifying the actual service.
Before diving deep into examples, make sure you have WireMock ready. You can add it to your Maven or Gradle build.
Maven Dependency
<dependency>
<groupId>com.github.tomakehurst</groupId>
<artifactId>wiremock-jre8</artifactId>
<version>2.32.0</version>
<scope>test</scope>
</dependency>
Gradle Dependency
testImplementation 'com.github.tomakehurst:wiremock-jre8:2.32.0'
Setting up WireMock
Once you have added WireMock to your project, start by creating a simple mock server. WireMock provides a fluent API to set up your server and define the endpoints to mock.
Starting a WireMock Server
import static com.github.tomakehurst.wiremock.client.WireMock.*;
public class WireMockExample {
public static void main(String[] args) {
// Start the WireMock server on port 8080
WireMockServer wireMockServer = new WireMockServer(8080);
wireMockServer.start();
// Configure stubbing for a REST endpoint
wireMockServer.stubFor(get(urlEqualTo("/api/data"))
.willReturn(aResponse()
.withHeader("Content-Type", "application/json")
.withBody("{\"message\": \"Hello, WireMock!\"}")
.withStatus(200)));
}
}
Commentary
In this example:
- We created a new instance of
WireMockServer
and started it on port 8080. - The stub we defined mocks an endpoint
/api/data
, returning a simple JSON response when accessed.
This setup means you can direct your application to call http://localhost:8080/api/data
, and it will receive the mocked response instead of hitting an actual service.
Writing Tests with WireMock
Now that you have the mock server up, you can write integration tests using your favorite testing framework, such as JUnit. Here’s how to do it with JUnit 5.
import com.github.tomakehurst.wiremock.junit5.WireMockTest;
import org.junit.jupiter.api.Test;
import org.springframework.web.client.RestTemplate;
import static com.github.tomakehurst.wiremock.client.WireMock.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
@WireMockTest
public class ApiServiceTest {
private final RestTemplate restTemplate = new RestTemplate();
@Test
void shouldReturnMockedResponse() {
stubFor(get(urlEqualTo("/api/data"))
.willReturn(aResponse()
.withHeader("Content-Type", "application/json")
.withBody("{\"message\": \"Hello, WireMock!\"}")
.withStatus(200)));
ApiService apiService = new ApiService(restTemplate);
String response = apiService.getData();
assertEquals("Hello, WireMock!", response);
}
}
Explanation
- WireMockTest Annotation: This annotation tells JUnit to start a WireMock server before running the test and to stop it afterward.
- Stub Definition: Just like earlier, we defined a stub for the
/api/data
endpoint. - Service Call and Assertion: We made the service call via
ApiService
and asserted that the returned message matched our mocked response.
This complete isolation of external services from our tests allows for focusing solely on the logic of your application.
Simulating Various Scenarios
WireMock isn’t just for happy paths; you can simulate various scenarios such as errors or timeouts easily. For example, you may want to simulate a 500 error response.
stubFor(get(urlEqualTo("/api/error"))
.willReturn(aResponse()
.withStatus(500)
.withBody("{\"error\": \"Internal Server Error\"}")));
In this case, when your service calls /api/error
, it will receive a 500 status code, helping you ensure your application can gracefully handle errors.
Verifying Interactions
Another powerful feature of WireMock is its ability to verify interactions. This helps ensure your application is making the correct calls to the mocked service.
verify(getRequestedFor(urlEqualTo("/api/data")));
With this line, you can check if your application attempted to call the /api/data
endpoint during the test.
Benefits of Using WireMock
- Speed: Tests run faster as they remove the network latency and reduce dependence on external services.
- Consistency: Results are consistent across different runs, eliminating flaky tests caused by variable service states.
- Configurable Responses: Easily manipulate response content and status codes to simulate various scenarios and edge cases.
Best Practices
- Keep Mocks Lightweight: Only mock the services that your test genuinely requires.
- Use Scenarios for Complex States: WireMock supports stateful behavior. Utilize this feature for more intricate integration tests that depend on multiple states.
- Review Logs: Check the WireMock logs to debug the interactions during tests if they do not behave as expected.
My Closing Thoughts on the Matter
Using WireMock effectively in your integration tests brings significant improvements to speed, reliability, and test coverage. It's an invaluable tool that tackles some of the core challenges faced when testing applications that depend on external services.
With the knowledge gained from this blog, you can streamline your integration tests and ensure your applications are thoroughly tested, resilient, and robust.
Still looking to dive deeper? You might find additional resources useful:
- WireMock Documentation
- JUnit 5 User Guide
- Effective Unit Testing
Happy testing!