Common Pitfalls in Android Dynamic and XML Layouts
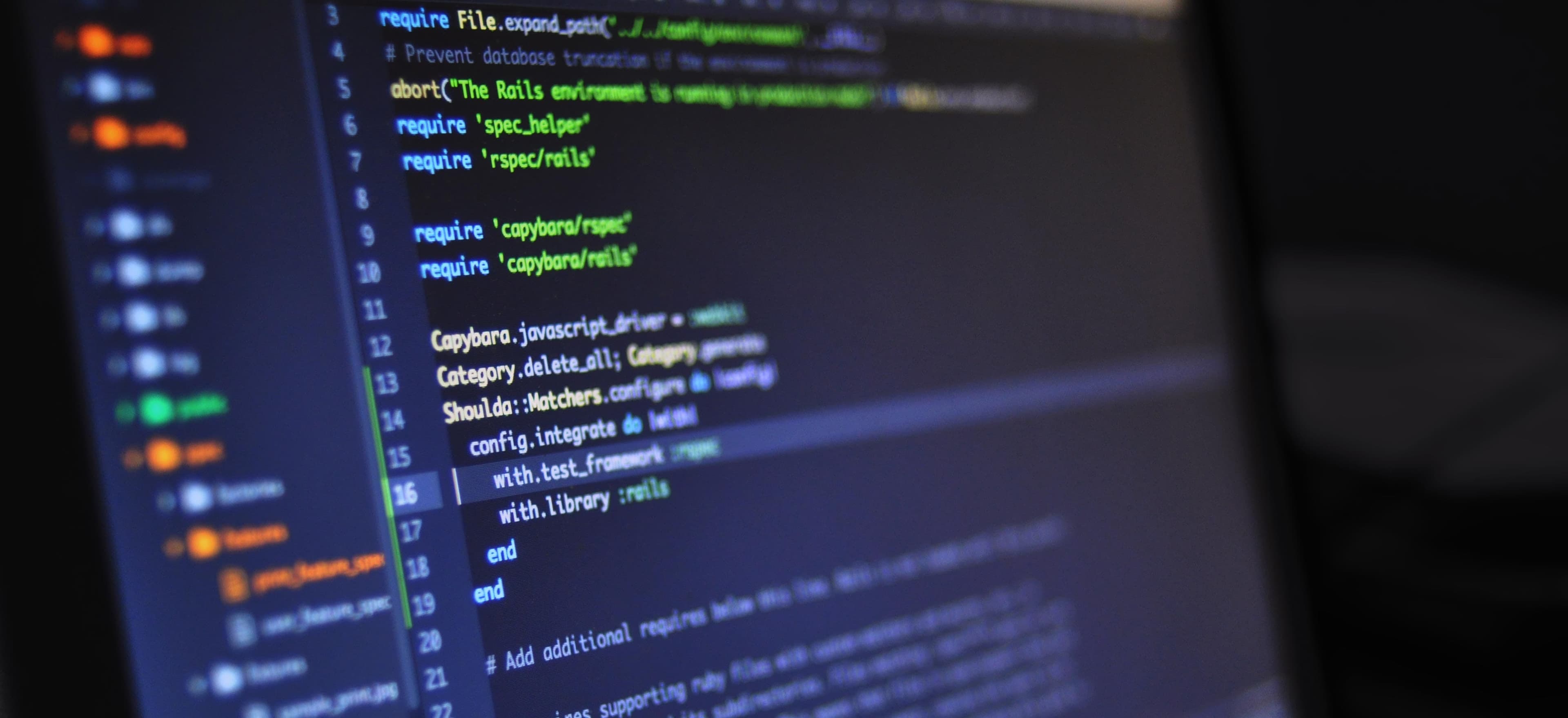
- Published on
Common Pitfalls in Android Dynamic and XML Layouts
Android development is a vast territory where understanding layouts is essential for creating responsive and user-friendly applications. Both dynamic and XML layouts have their own sets of advantages and potential pitfalls. In this blog post, we will explore common pitfalls associated with these two types of layouts and provide you with best practices to avoid them.
Understanding Dynamic and XML Layouts
Before diving into the pitfalls, let’s quickly clarify what dynamic and XML layouts are in the context of Android development:
-
XML Layouts: Static layouts defined using XML files, typically located in the
res/layout
directory. These layouts are defined at design time and loaded into views during runtime. -
Dynamic Layouts: Layouts created programmatically using Java or Kotlin code. This flexibility allows you to modify, add, or remove views at runtime based on user actions or application logic.
While both methods provide unique benefits, developers often run into common issues that can affect app performance, user experience, and maintainability.
Common Pitfalls in XML Layouts
1. Overusing Nested Layouts
Pitfall: One of the most frequently encountered issues is excessive nesting of layouts, which can lead to complex view hierarchies and, ultimately, poor performance.
Avoidance Strategy: Use ConstraintLayout
or LinearLayout
wisely to minimize hierarchy depth. ConstraintLayout is designed to flatten your layout and minimize unnecessary levels of nesting.
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/title"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
android:text="Hello, Android!" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintTop_toBottomOf="@id/title"
app:layout_constraintStart_toStartOf="parent"
android:text="Click Me" />
</androidx.constraintlayout.widget.ConstraintLayout>
Why: Using fewer nested layouts can lead to a more optimized view hierarchy, resulting in lower layout rendering time.
2. Hardcoding Dimensions
Pitfall: Hardcoding specific pixel dimensions can lead to issues with screen size and density variations.
Avoidance Strategy: Instead of hardcoding, utilize wrap_content, match_parent, or dimension resources (e.g., dimens.xml
) to ensure your layouts are adaptable.
<EditText
android:id="@+id/inputField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter your name" />
Why: This approach allows your application to automatically scale across different devices, minimizing the risk of layout breakage and improving usability.
3. Not Utilizing Styles and Themes
Pitfall: Copying and pasting attributes across multiple XML files results in redundancy and makes it challenging to maintain a consistent look.
Avoidance Strategy: Utilize styles and themes in styles.xml
to manage appearance properties centrally.
<resources>
<style name="ButtonStyle">
<item name="android:layout_width">wrap_content</item>
<item name="android:layout_height">wrap_content</item>
<item name="android:background">?attr/colorPrimary</item>
</style>
</resources>
Why: By defining styles, you can easily change the appearance of multiple components without altering each individual XML file, enhancing maintainability.
Common Pitfalls in Dynamic Layouts
1. Memory Leaks from Improper View References
Pitfall: Holding on to references of views in contexts that outlive them can lead to memory leaks.
Avoidance Strategy: Always release references when views are no longer needed. Avoid storing context in static variables.
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Implement your onClick logic
}
});
Why: Clean code helps you maintain application performance and prevents unnecessary memory consumption.
2. Not Managing View States
Pitfall: Failing to handle the state of dynamically added views can lead to inconsistencies in user experience.
Avoidance Strategy: Properly manage the view states by saving and restoring them (consider using onSaveInstanceState
and onRestoreInstanceState
).
@Override
protected void onSaveInstanceState(Bundle savedInstanceState) {
super.onSaveInstanceState(savedInstanceState);
savedInstanceState.putBoolean("buttonVisible", button.getVisibility() == View.VISIBLE);
}
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
if (savedInstanceState.getBoolean("buttonVisible")) {
button.setVisibility(View.VISIBLE);
}
}
Why: Managing view states ensures that users have a consistent experience, contributing to better app usability.
3. Over-Complexity in UI Logic
Pitfall: Creating overly detailed logic for displaying dynamic views results in code that is hard to read and maintain.
Avoidance Strategy: Keep your dynamic view creation logic simple. Use helper methods or separate classes for managing complex view logic.
private void createDynamicButton() {
Button button = new Button(this);
button.setText("Dynamic Button");
button.setLayoutParams(new LinearLayout.LayoutParams(
LinearLayout.LayoutParams.WRAP_CONTENT,
LinearLayout.LayoutParams.WRAP_CONTENT));
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Your logic here
}
});
linearLayout.addView(button);
}
Why: Simple code is easier to test and maintain, enhancing overall project quality.
Best Practices for Both Layout Types
1. Profile Performance
Utilize Android's built-in profiling tools to analyze render times, memory usage, and layout performance. Tools such as Android Profiler can provide invaluable insights into potential issues.
2. Leverage Layout Inspector
Use the Layout Inspector tool in Android Studio to inspect the view hierarchy of your application in real-time. This can help identify any unnecessary nesting or improperly rendered views.
3. Follow Material Design Guidelines
Adhering to the Material Design guidelines ensures that your app remains user-friendly and visually appealing.
A Final Look
Creating layouts in Android is not merely about arranging components on the screen. It involves a delicate balance of performance, usability, and maintainability. By understanding and avoiding common pitfalls in both dynamic and XML layouts, developers can create more robust applications that stand up to scrutiny across a diverse range of devices.
If you found this information helpful, be sure to check out other topics on Android development, such as Android Jetpack for enhancing your app's architecture and Kotlin Coroutines for managing asynchronous programming with elegance.
Remember that effective layout management is a cornerstone of a successful Android application. Embrace these best practices, continue to learn, and elevate your Android development skills!
This article focused on addressing the common pitfalls in Android layouts. For further information or more advanced topics, feel free to reach out or leave questions in the comments section below!
Checkout our other articles