Solving Enum Persistence Issues with JPA 2.1 Type Converters
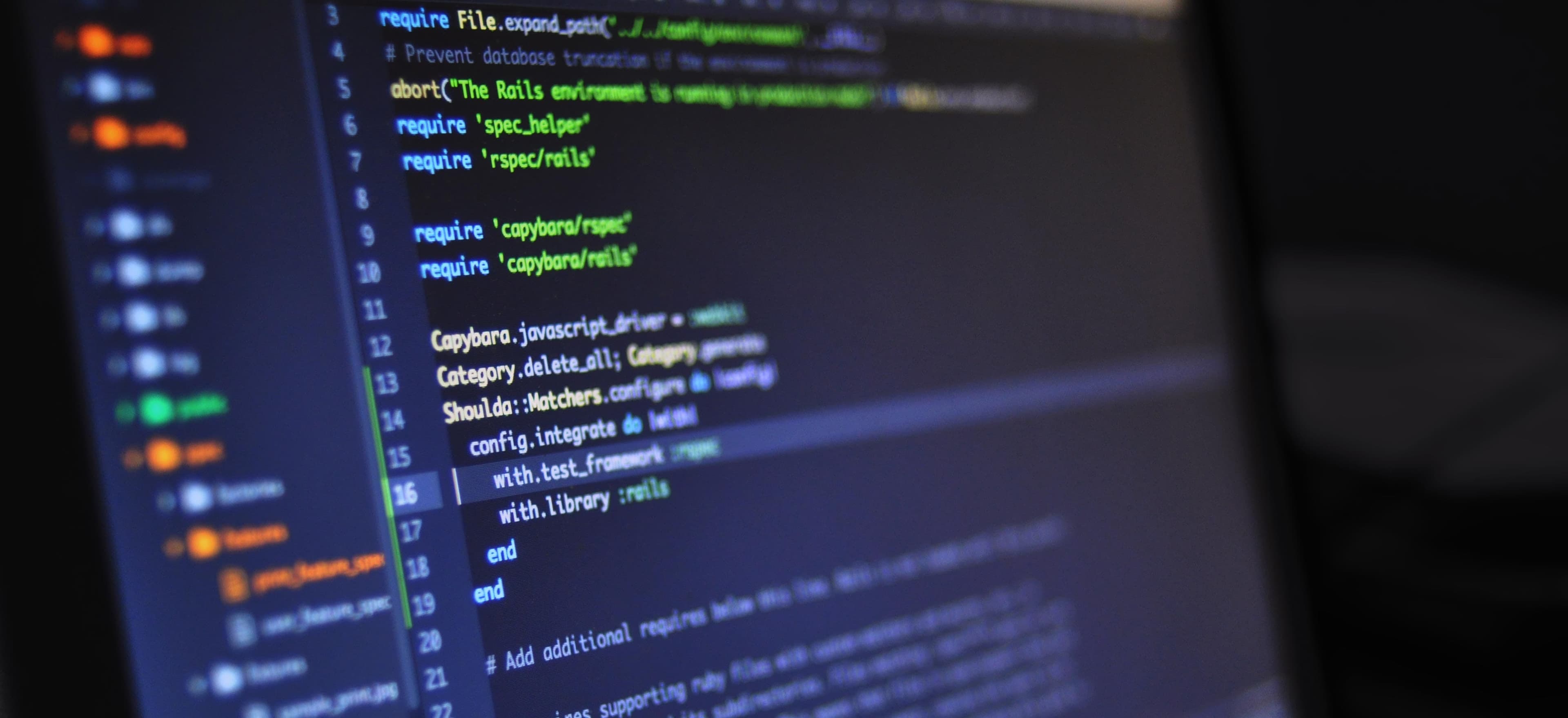
- Published on
Solving Enum Persistence Issues with JPA 2.1 Type Converters
Java Enums provide a robust way to define fixed sets of constants that can make your code more readable and maintainable. However, when it comes to persisting these Enums in a database using Java Persistence API (JPA), developers often encounter issues that can lead to inefficiencies and bugs. Fortunately, JPA 2.1 introduced Type Converters that help us handle these challenges effectively.
In this blog post, we will explore how to utilize JPA Type Converters to solve Enum persistence issues. We will define an Enum, create a JPA entity, and implement a converter to map the Enum to its corresponding database representation. This approach ensures that your code remains clean while improving database interactions.
Understanding Enums in JPA
Before we delve into the solution, let’s understand why using Enums in JPA can be problematic.
In JPA, Enums can be stored in two ways: as ordinal values (which represent the position of the Enum constant) or as string values (which represent the name of the Enum constant). While these methods are straightforward, they are not without limitations:
- Ordinal Values: Using ordinal values can lead to issues when Enum constants are reordered, as this affects their corresponding database values.
- String Values: Storing Enums as strings is more readable, but it can lead to storage inefficiencies and mistakes if there is a typo in the string values.
To overcome these issues, JPA 2.1 introduced Type Converters, allowing us to define how to convert our Enums to and from their database representation.
Example Enum Implementation
Let's create a simple Enum to represent a possible status for an order in an e-commerce application.
public enum OrderStatus {
PLACED,
SHIPPED,
DELIVERED,
CANCELED;
}
This OrderStatus
Enum is easy to read and provides clarity for anyone looking at the code. Now, let’s create a corresponding JPA entity that will make use of this Enum.
Creating a JPA Entity
Here’s how you can create a JPA entity called Order
that uses the OrderStatus
Enum.
import javax.persistence.*;
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String customerName;
@Convert(converter = OrderStatusConverter.class)
private OrderStatus status;
// Getters and Setters
}
In this Order
entity:
- We link the
OrderStatus
Enum with thestatus
field. - We apply the
@Convert
annotation to specify that JPA should use aTypeConverter
to persist this Enum effectively.
Implementing the Enum Converter
Now let's implement the OrderStatusConverter
, which will handle the conversion of the Enum to a database-compatible format, and vice versa.
import javax.persistence.AttributeConverter;
import javax.persistence.Converter;
@Converter(autoApply = true)
public class OrderStatusConverter implements AttributeConverter<OrderStatus, String> {
@Override
public String convertToDatabaseColumn(OrderStatus status) {
if (status == null) {
return null; // Handle null case
}
return status.name(); // Store Enum as String
}
@Override
public OrderStatus convertToEntityAttribute(String dbData) {
if (dbData == null) {
return null; // Handle null case
}
return OrderStatus.valueOf(dbData); // Convert String back to Enum
}
}
Explanation of the Converter
-
Implementation of
AttributeConverter<EnumType, DBType>
:- Here,
OrderStatus
is the Enum type, andString
is the database type we choose to utilize.
- Here,
-
convertToDatabaseColumn() Method:
- This method transforms the Enum to its database representation (in this case, a string).
- When the Enum is null, it returns null, which is crucial for database integrity.
-
convertToEntityAttribute() Method:
- This method converts the database string back to the corresponding Enum.
- It also handles the null case similarly.
Advantages of Using Type Converters
- Decoupling: Separating the conversion logic helps to maintain cleaner code and makes it easier to manage.
- Custom Logic: You can include additional custom logic inside your converter if needed (like mapping different Enum names).
- Automatic Application: With
autoApply = true
, you can avoid repetitive converter annotations on each field.
Practical Example: Using JPA with Type Converters
With our Order
entity and OrderStatusConverter
in place, let's see how to persist and retrieve an Order
object.
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
public class OrderPersistenceDemo {
public void persistOrder() {
EntityManagerFactory emf = Persistence.createEntityManagerFactory("your-persistence-unit");
EntityManager em = emf.createEntityManager();
em.getTransaction().begin();
Order order = new Order();
order.setCustomerName("John Doe");
order.setStatus(OrderStatus.PLACED);
em.persist(order);
em.getTransaction().commit();
em.close();
emf.close();
}
public Order getOrder(Long orderId) {
EntityManagerFactory emf = Persistence.createEntityManagerFactory("your-persistence-unit");
EntityManager em = emf.createEntityManager();
Order order = em.find(Order.class, orderId);
em.close();
emf.close();
return order;
}
}
Closing Remarks
Using JPA 2.1 Type Converters to resolve Enum persistence issues significantly enhances both your code's structure and its maintainability. By separating the conversion logic, you ensure better readability and flexibility, avoiding potential pitfalls with ordinal or string-based storage.
This method can be applied not only to Enums but also to other complex data types that require conversion. If you're dealing with a large number of Enums and persistent types, embracing JPA Type Converters can keep your data layer clean and efficient.
For more details about Java Enums and JPA, you can check out the official JPA documentation or this insightful article on Enums in Java.
Now, it’s time to implement these techniques in your applications and take advantage of the benefits that come along with them. Happy coding!
Checkout our other articles