Common Pitfalls in Generating HTML with Servlets
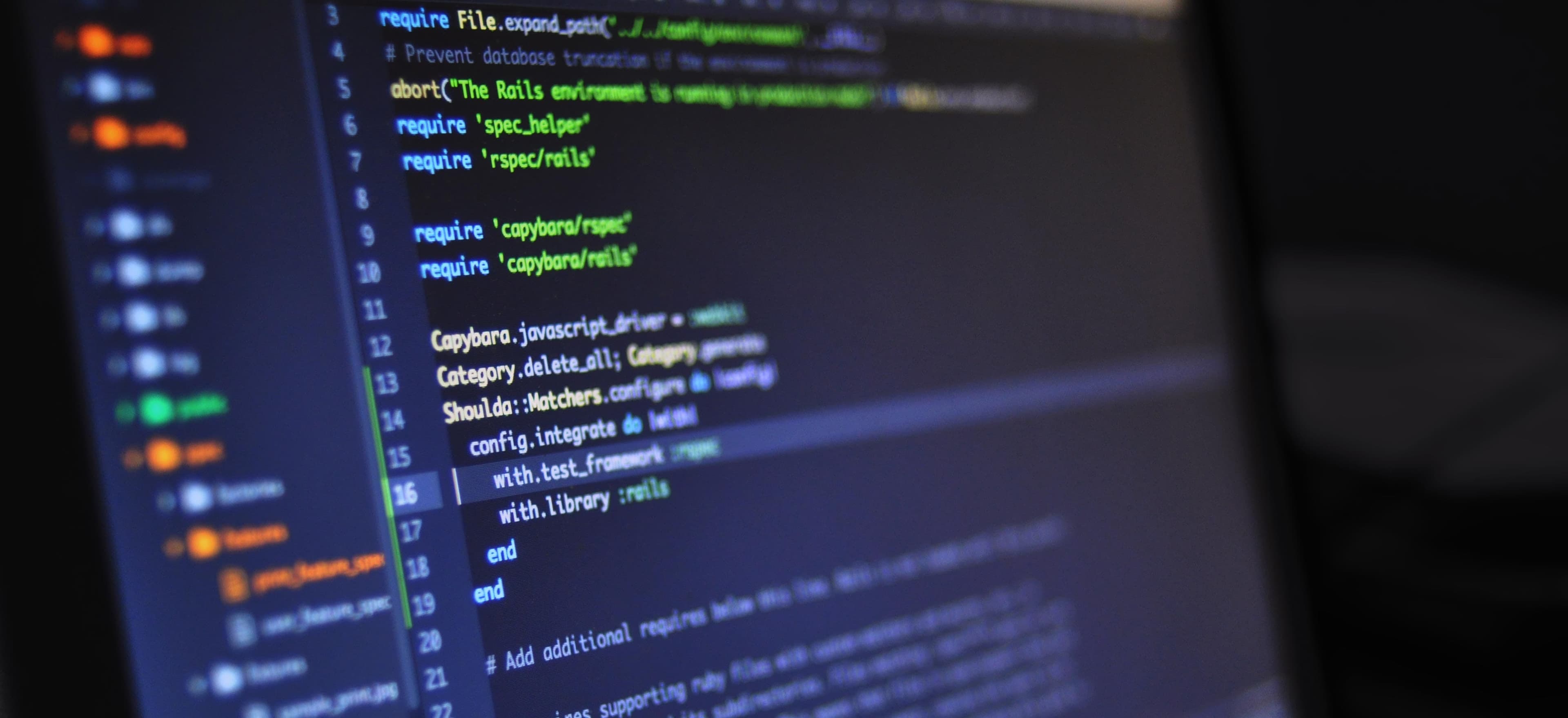
- Published on
Common Pitfalls in Generating HTML with Servlets
Java Servlets are a powerful technology for building web applications. They facilitate interaction between a client and a server, generating dynamic content on-the-fly. However, while working with them, developers often encounter specific pitfalls when it comes to generating HTML. This blog post aims to outline these common issues, provide solutions, and ensure you can create robust, maintainable web applications.
Understanding Servlets
Before we dive into the pitfalls, let's clarify what a servlet is. A servlet is a Java program that runs on a server and handles client requests, producing responses—usually in the form of HTML. It plays a significant role in Java EE web applications. For more on servlets, you can check the official Java EE documentation.
Structure of a Basic Servlet
Here's a simple example of what a basic servlet looks like:
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.PrintWriter;
@WebServlet("/hello")
public class HelloServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h1>Hello, World!</h1>");
out.println("</body></html>");
}
}
This code sets up a servlet that responds with a simple "Hello, World!" HTML message. Let's now explore the common pitfalls when generating HTML.
Pitfall 1: Mixing Logic with Presentation
Problem
One common pitfall is the improper mixing of business logic and presentation. Many developers tend to write Java code directly in the HTML output, leading to cluttered, unreadable code and making it hard to maintain.
Solution
Use the Model-View-Controller (MVC) architecture to separate concerns. The servlet should only handle requests and responses, while JSPs (JavaServer Pages) or other templating engines manage the presentation layer.
// In your Servlet
RequestDispatcher dispatcher = request.getRequestDispatcher("view.jsp");
dispatcher.forward(request, response);
This piece of code forwards the request to a JSP page named view.jsp
, which handles presentation.
Why It Matters
By adhering to MVC principles, you gain maintainability, scalability, and cleaner code. Templated files handle HTML rendering, while servlets manage requests and data flow.
Pitfall 2: Hardcoding HTML Content
Problem
Another frequent pitfall is hardcoding HTML directly into your servlets. It makes your servlet unnecessarily bulky and inflexible.
Solution
Use constructs to manage your HTML templates. For instance, you can define HTML segments in constants or externalize them into separate files.
public class HtmlResponse {
public static String getHeader() {
return "<html><head><title>Welcome</title></head><body>";
}
public static String getFooter() {
return "</body></html>";
}
}
// In your servlet
PrintWriter out = response.getWriter();
out.println(HtmlResponse.getHeader());
out.println("<h1>Welcome to my website!</h1>");
out.println(HtmlResponse.getFooter());
Why It Matters
This approach enhances reusability, making it easier to modify or replace different parts of your HTML response.
Pitfall 3: Outputting User Input Directly
Problem
Outputting user input directly in HTML can lead to security vulnerabilities like Cross-Site Scripting (XSS). Failing to sanitize or escape user inputs exposes your application to attacks.
Solution
Always validate and escape user inputs. Use built-in libraries such as Apache Commons Lang's StringEscapeUtils
to avoid XSS issues.
import org.apache.commons.text.StringEscapeUtils;
// After getting user input
String userInput = request.getParameter("input");
String safeInput = StringEscapeUtils.escapeHtml4(userInput);
out.println("<div>" + safeInput + "</div>");
Why It Matters
Security is paramount in web applications. By sanitizing user inputs, you protect users and their data, ensuring a secure application environment.
Pitfall 4: Not Setting the Content Type
Problem
Many developers forget to set the content type of the response. This may confuse the client about what type of data is being sent, resulting in broken web pages.
Solution
Always set the content type at the beginning of your doGet
or doPost
method.
response.setContentType("text/html;charset=UTF-8");
Why It Matters
Setting the correct content type ensures that browsers interpret the response appropriately, providing the right rendering context for the content.
Pitfall 5: Ignoring HTTP Status Codes
Problem
Failing to acknowledge the significance of HTTP status codes can lead to uncommunicative responses. For example, sending an error page without a proper 404 or 500 status code could mislead users or clients consuming your API.
Solution
Explicitly set the HTTP status code as needed using the setStatus
method.
if (userNotFound) {
response.sendError(HttpServletResponse.SC_NOT_FOUND, "User not found");
}
Why It Matters
Properly indicating the state of responses enhances user experience and assists in debugging and monitoring your application.
Final Thoughts
Generating HTML with Servlets can be a straightforward task, but it is essential to be aware of the common pitfalls that may arise. Using best practices like separating concerns, sanitizing inputs, and appropriately setting content types and HTTP status codes ensures the development of robust web applications.
If you are interested in learning more about Java web development, consider exploring frameworks like Spring MVC or JSF. For a deeper understanding of servlets, you may refer to the Java EE Tutorial.
By applying the tips discussed in this post, you will find yourself avoiding the common pitfalls associated with servlet-based HTML generation, ultimately leading to cleaner, more maintainable, and secure applications. Happy coding!
Checkout our other articles