Managing Java Conflicts: Installing Multiple Versions Smoothly
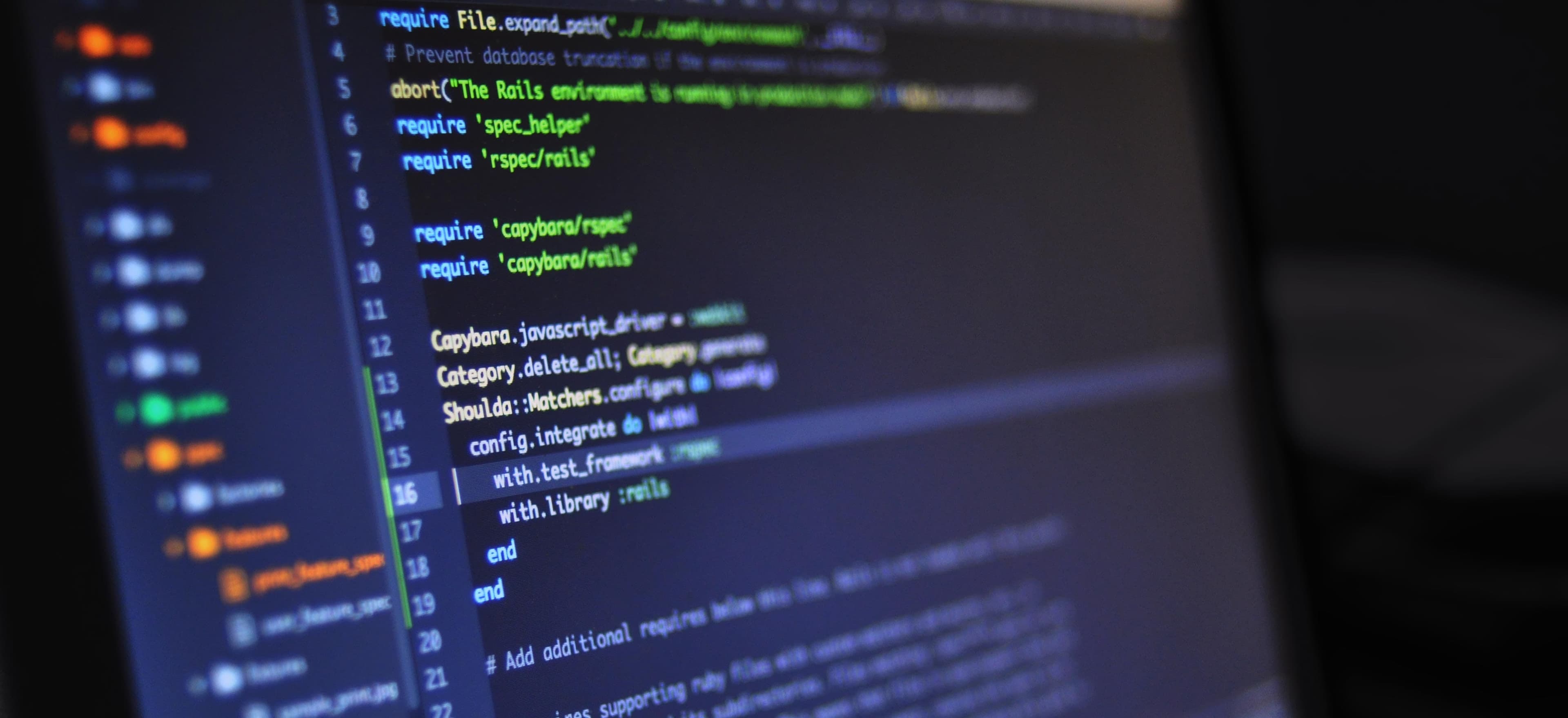
- Published on
Managing Java Conflicts: Installing Multiple Versions Smoothly
Java is a versatile and powerful programming language used across various platforms and applications. However, developers often face challenges when working with multiple Java versions for different projects. Managing these conflicts wisely can significantly enhance productivity and minimize compatibility issues. In this blog post, we'll explore effective strategies to install and manage multiple Java versions smoothly.
Why Manage Multiple Java Versions?
While developing applications, dependencies may require different Java versions. For example:
- Legacy Applications: Some older applications may only run on Java 8.
- New Projects: Newer applications may take advantage of the features of Java 17 or later.
- Testing: You may want to test your application on various Java versions.
To effectively manage these scenarios without creating unnecessary conflicts, you need a strategy.
Preparing Your Environment
Before diving into installations and configurations, let’s clarify how to check your current Java version.
Checking the Installed Java Version
You can check your currently installed version of Java through the command line:
java -version
This command will output the version of Java that is currently in use. If you have multiple versions installed, the output might not reflect the versions you require for different projects.
Method 1: Using the Java Version Manager (JEnv)
One of the most streamlined and robust solutions to manage multiple Java versions is JEnv. This tool allows you to switch between different Java installations effortlessly.
Step 1: Install JEnv
To install JEnv on macOS, you can use Homebrew:
brew install jenv
For other systems, refer to the JEnv GitHub repository for installation instructions.
Step 2: Add Java Versions to JEnv
After installing JEnv, the next step is to add your installed Java versions. Use the following command to add a version:
jenv add /path/to/java-version
For example:
jenv add /Library/Java/JavaVirtualMachines/jdk1.8.0_251.jdk/Contents/Home
Step 3: Setting Global and Local Versions
You can set a global version of Java for all your terminal sessions:
jenv global 1.8
Alternatively, you can set a local version for a specific project:
jenv local 11
This flexibility ensures your environment matches your project needs without creating persistent conflicts.
Example Usage
Imagine you are developing two applications—one for Java 8 and another for Java 11:
- Navigate to your Java 8 project directory.
- Run
jenv local 1.8
. - Switch to your Java 11 project directory.
- Run
jenv local 11
.
Now, JEnv automatically handles the necessary environment changes when you switch directories.
Method 2: Manual Installation and Configuration
In situations where tools like JEnv are not suitable, a manual approach can be adopted. Here's how to get started:
Step 1: Download Java Versions
Visit the Oracle Java SE Downloads page or the Adoptium website to download the Java versions you need.
Step 2: Install Java Versions
- Follow the installation instructions provided by the distribution you choose.
- Each installation should be placed in its dedicated directory (e.g.,
/usr/lib/jvm/java-8-oracle
,/usr/lib/jvm/java-11-openjdk
).
Step 3: Update Environment Variables
Java relies on environment variables to operate correctly. You must configure your PATH
and JAVA_HOME
variables accordingly.
Example Configuration for Linux/MacOS
You can add the following lines to your shell profile (e.g., ~/.bashrc
, ~/.bash_profile
, or ~/.zshrc
):
export JAVA_HOME=/usr/lib/jvm/java-11-openjdk
export PATH=$JAVA_HOME/bin:$PATH
To apply the changes, run:
source ~/.bashrc
This configuration ensures your shell uses the specified Java version. You can change JAVA_HOME
to switch versions.
Managing Conflicts When Switching Versions
Switching between Java versions can lead to conflicts, especially if you have IDEs or build tools that reference a specific version. Here are tips to manage such situations effectively.
Tips for IDEs
- Eclipse: Go to
Window > Preferences > Java > Installed JREs
to configure the Java Runtime Environment. - IntelliJ IDEA: Navigate through
File > Project Structure > SDKs
to add or change SDK versions.
Build Tools
Ensure you update the language level and SDK in your project build file accordingly. For Gradle, you can specify the Java version in your build.gradle
:
sourceCompatibility = '1.8'
targetCompatibility = '1.8'
For Maven, you can set the Java version in your pom.xml
:
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
These configurations will ensure your builds use the Java version you desire, avoiding conflicts.
Testing Your Configuration
After setting up your environment and configurations, it is important to verify that everything works as expected.
Testing with Simple Java Program
You can create a simple Java program to confirm the Java version being used:
public class VersionTest {
public static void main(String[] args) {
System.out.println("Java version: " + System.getProperty("java.version"));
}
}
Compile and execute this code snippet, and it should output the current Java version, confirming your setup is effective.
Execute
To compile and run the program:
javac VersionTest.java
java VersionTest
Key Takeaways
Managing multiple Java versions doesn't have to be a headache. Whether you prefer using JEnv or manually configuring your environment, following best practices and understanding your project requirements will streamline your development process.
By taking the time to correctly set up your environment, you can avoid conflicts and ensure that your applications run seamlessly. For further information, refer to the official Java documentation for more insights on managing Java installations.
Share your experiences with managing Java versions in the comments below! Happy coding!
Checkout our other articles