Mastering Standard Values in Cucumber Java Tests
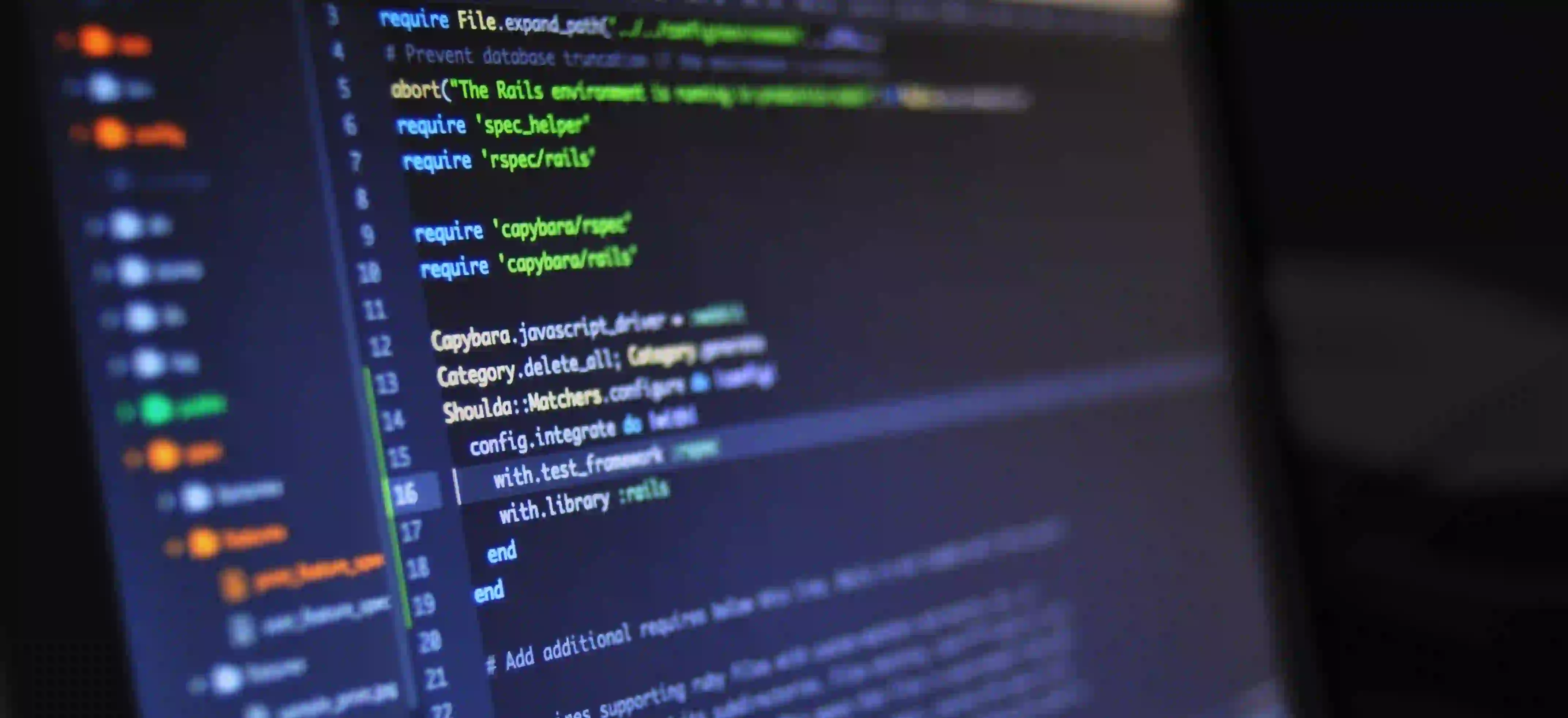
Mastering Standard Values in Cucumber Java Tests
Cucumber is a powerful tool for Behavior-Driven Development (BDD) that enables developers and non-technical stakeholders to collaborate on defining software requirements. One of the core features of Cucumber is its ability to work with standard values, which can enhance both readability and maintainability of your test scenarios. In this blog post, we will discuss how to effectively use standard values in Cucumber Java tests with engaging explanations and practical examples.
What Are Standard Values?
Standard values in Cucumber tests refer to pre-defined constants or variables that represent specific data points used throughout your test scenarios. By employing standard values, you can:
- Improve Readability: It makes your tests easier to understand, especially for non-technical stakeholders.
- Enhance Maintainability: Updating standard values in one place saves you from making changes across multiple scenarios.
- Promote Reusability: Standard values can be reused across different feature files, ensuring consistency.
Setting Up Cucumber with Java
Before we delve deeper, let's ensure you have a basic Cucumber setup using Java. Follow these steps to set up your environment:
-
Add Dependencies: Make sure to include the necessary Cucumber dependencies in your
pom.xml
file if you're using Maven:📄snippet.txt<dependency> <groupId>io.cucumber</groupId> <artifactId>cucumber-java</artifactId> <version>7.2.3</version> <scope>test</scope> </dependency> <dependency> <groupId>io.cucumber</groupId> <artifactId>cucumber-junit</artifactId> <version>7.2.3</version> <scope>test</scope> </dependency>
-
Create Feature Files: Define your test scenarios in a
.feature
file. -
Implement Step Definitions: Write the corresponding Java implementations for the steps defined in your feature files.
Example Scenario Using Standard Values
Let's walk through a practical example where we implement standard values in our Cucumber tests for a simple user registration feature.
Step 1: Define Your Feature File
Create a file named user_registration.feature
:
Feature: User Registration
Scenario: Successful user registration
Given the user is on the registration page
When the user enters valid registration data
Then the user should see a success message
Step 2: Create a Constants Class
Establish a Constants
class to house your standard values:
public class Constants {
public static final String REGISTRATION_SUCCESS_MESSAGE = "Registration successful!";
public static final String VALID_USERNAME = "testUser";
public static final String VALID_EMAIL = "test@example.com";
public static final String VALID_PASSWORD = "SecurePassword123";
}
Using a central location for constants, like our Constants
class, keeps your configurations tidy. If your registration success message changes, you'd update it in one place.
Step 3: Implement Step Definitions
Next, implement the step definitions in a new Java class:
import io.cucumber.java.en.Given;
import io.cucumber.java.en.When;
import io.cucumber.java.en.Then;
import static org.junit.Assert.assertEquals;
public class UserRegistrationSteps {
private String registrationMessage;
@Given("the user is on the registration page")
public void userIsOnRegisterPage() {
// Simulate user navigation to the registration page
System.out.println("User navigated to the registration page.");
}
@When("the user enters valid registration data")
public void userEntersValidData() {
String username = Constants.VALID_USERNAME;
String email = Constants.VALID_EMAIL;
String password = Constants.VALID_PASSWORD;
// Simulate successful registration process
registrationMessage = registerUser(username, email, password);
}
@Then("the user should see a success message")
public void userShouldSeeSuccessMessage() {
assertEquals(Constants.REGISTRATION_SUCCESS_MESSAGE, registrationMessage);
}
private String registerUser(String username, String email, String password) {
// This should contain actual registration logic (e.g., saving to a database)
return Constants.REGISTRATION_SUCCESS_MESSAGE; // Simulate success
}
}
Commentary on the Code
- Constants Integration: By bringing standard values into our step definitions, we enhance portability. If, for example, the success message shifts to "User registration completed!", you only need to change it in the
Constants
class. - Simplicity: The step definitions are straightforward, readily understandable to both technical and non-technical individuals.
- Ease of Testing: By isolating registration logic into its own method, we can easily unit test this function in isolation.
Benefits of Using Standard Values
- Centralized Management: All standard values are maintained in one class, making search and modification straightforward.
- Easier Collaboration: Both technical and non-technical team members can comprehend and modify test scenarios with confidence.
- Less Prone to Errors: Reducing the number of hard-coded strings minimizes the chance of typographical errors.
Best Practices for Standard Values
- Limit Scope: Only define values that are truly shared across multiple scenarios. Overuse can lead to bloated constant classes.
- Use Clear Naming Conventions: Standard value names should clarify their purpose (e.g.,
VALID_EMAIL
vsEMAIL_1
). - Document Changes: When modifications are made to standard values, document the rationale to maintain clarity for future contributors.
Additional Resources
For further reading and in-depth understanding of Cucumber testing, consider the following resources:
In Conclusion, Here is What Matters
Mastering standard values in Cucumber Java tests serves as a game-changer for your testing approach. It simplifies maintenance and enhances collaboration across teams. By incorporating constants into your test scenarios, you not only foster clarity but also streamline the testing process.
With the principles outlined in this post, you are now equipped to implement a more effective and sustainable testing strategy in your Java applications using Cucumber. Embrace standard values and watch your BDD practices flourish!
Happy testing!