Navigating Ethical Dilemmas in Software Development
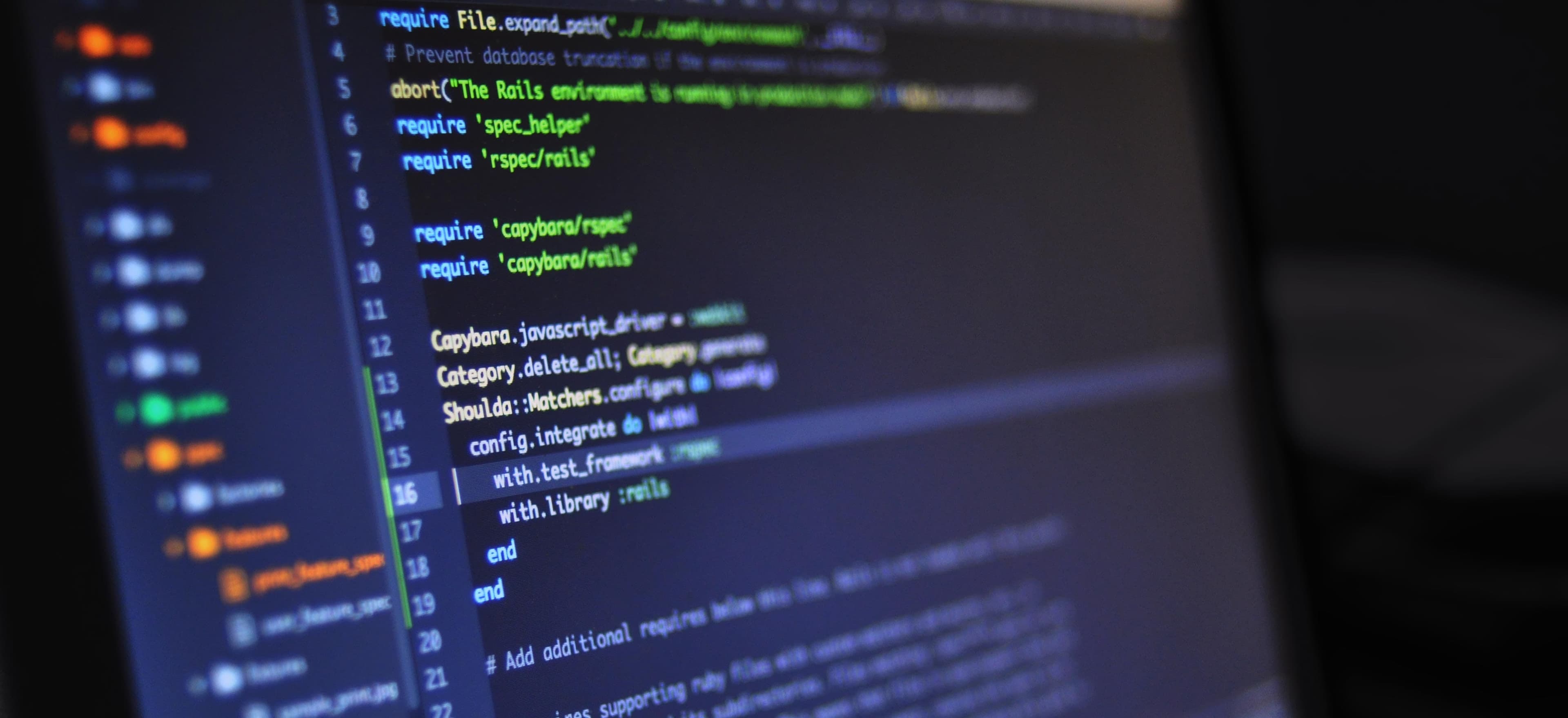
- Published on
Navigating Ethical Dilemmas in Software Development
In an era where technology is omnipresent, software developers wield an immense amount of influence over society. Their decisions can alter how we communicate, work, and even think. However, with great power comes great responsibility. This blog post explores the ethical dilemmas that software developers face and offers a framework for navigating these challenges.
The Importance of Ethics in Software Development
Software ethics encompass the moral principles governing the conduct of software developers. Why is this important? Ethical decisions can have far-reaching implications. Consider:
- Data Privacy: Developers handle sensitive user data. A single oversight in data protection can lead to breaches that compromise user trust.
- Bias in Algorithms: Unchecked algorithms can perpetuate harmful stereotypes or discrimination.
- Impact on Employment: Automation and AI can disrupt job markets. Developers should consider the human cost of their innovations.
These factors emphasize the necessity for ethical considerations. By establishing a robust ethical framework, developers can ensure their creations contribute positively to society.
Common Ethical Dilemmas
Here are some common ethical dilemmas that software developers encounter:
1. Data Privacy Concerns
Handling user data is a significant responsibility. Developers often face a decision between monetizing this data and protecting user privacy.
Example Scenario
Imagine a social media platform that analyzes user behavior to enhance advertising strategies. Should developers prioritize broad data insights or individual privacy?
Suggested Approach
Implement data anonymization techniques. Below is a simple Java example demonstrating how to anonymize data before processing:
import java.util.HashMap;
import java.util.Map;
public class DataAnonymizer {
public static Map<String, String> anonymizeUserData(Map<String, String> userData) {
Map<String, String> anonymizedData = new HashMap<>();
for (Map.Entry<String, String> entry : userData.entrySet()) {
String anonymizedKey = "USER_" + generateUniqueId();
anonymizedData.put(anonymizedKey, entry.getValue());
}
return anonymizedData;
}
private static String generateUniqueId() {
return String.valueOf(System.currentTimeMillis());
}
public static void main(String[] args) {
Map<String, String> userData = new HashMap<>();
userData.put("email", "user@example.com");
userData.put("phone", "123456789");
Map<String, String> anonymizedData = anonymizeUserData(userData);
System.out.println(anonymizedData);
}
}
Why This Matters
Anonymizing user data reduces the risk of privacy violations while still allowing analysis. This keeps user trust intact and ensures compliance with regulations like GDPR.
2. Algorithmic Bias
Algorithms can sometimes reflect the biases of their developers or the data they are trained on. This can lead to unfair outcomes, particularly in critical areas like hiring or law enforcement.
Example Scenario
A company develops an AI hiring tool that scans resumes. If training data reflects past biases, the algorithm may favor candidates from specific demographics.
Suggested Approach
A simple analysis and audit process can help identify biases. Here is a Java snippet for comparing gender representation in candidates:
import java.util.HashMap;
import java.util.Map;
public class BiasChecker {
public static void checkGenderRepresentation(Map<String, String> candidates) {
Map<String, Integer> genderCount = new HashMap<>();
for (String gender : candidates.values()) {
genderCount.put(gender, genderCount.getOrDefault(gender, 0) + 1);
}
System.out.println("Gender Representation Report: " + genderCount);
}
public static void main(String[] args) {
Map<String, String> candidates = new HashMap<>();
candidates.put("Candidate1", "Female");
candidates.put("Candidate2", "Male");
candidates.put("Candidate3", "Female");
checkGenderRepresentation(candidates);
}
}
Why This Matters
Regularly auditing algorithms ensures they are fair and equitable, minimizing the potential for societal harm.
3. Job Displacement Due to Automation
The rise of automation technology can lead to job losses. Developers must evaluate the long-term impacts of their projects.
Example Scenario
When creating an app designed to automate an aspect of a business, developers must consider who will be affected.
Suggested Approach
Developers can design tools that augment human capabilities rather than replace them. Here is an example of a Java-based task automation system that assists, rather than outright replaces, human workers:
import java.util.ArrayList;
import java.util.List;
class Task {
private String description;
public Task(String description) {
this.description = description;
}
public String getDescription() {
return description;
}
}
class TaskAutomation {
public static void assistWithTasks(List<Task> tasks) {
for (Task task : tasks) {
System.out.println("Assisting with: " + task.getDescription());
// Assume some automated processing occurs here
}
}
public static void main(String[] args) {
List<Task> tasks = new ArrayList<>();
tasks.add(new Task("Compile monthly reports"));
tasks.add(new Task("Schedule meetings"));
assistWithTasks(tasks);
}
}
Why This Matters
By designing software to assist human workers, developers strike a balance between technological advancement and societal responsibility.
Building an Ethical Framework
Developers can use the following guidelines to cultivate an ethical mindset:
1. Understand Regulations
Familiarize yourself with privacy regulations like GDPR or CCPA. These guidelines help frame the ethical standards your software must conform to.
2. Foster Open Communication
Encourage discussions within your team about ethical dilemmas. Diverse perspectives can bring new insights and prevent oversight.
3. Implement Continuous Learning
Ethics is an evolving field. Stay updated on the latest discussions surrounding technology and ethics by following resources like the ACM Code of Ethics.
4. Create Transparent Protocols
Implement internal guidelines that outline ethical standards for your software development processes.
In Conclusion, Here is What Matters
Navigating ethical dilemmas in software development is challenging but crucial. As developers, we must recognize the potential impacts of our work and commit ourselves to ethical standards that prioritize user rights and societal welfare. By actively engaging with ethical principles and fostering an open dialogue, we can develop technology that truly serves humanity.
By taking these steps, we can ensure that our innovations benefit society rather than harm it. The future of software development is bright, and through our commitment to ethics, we can help shape it responsibly.
Checkout our other articles