Maximizing Performance: Taming Concurrent Queries in Lucene
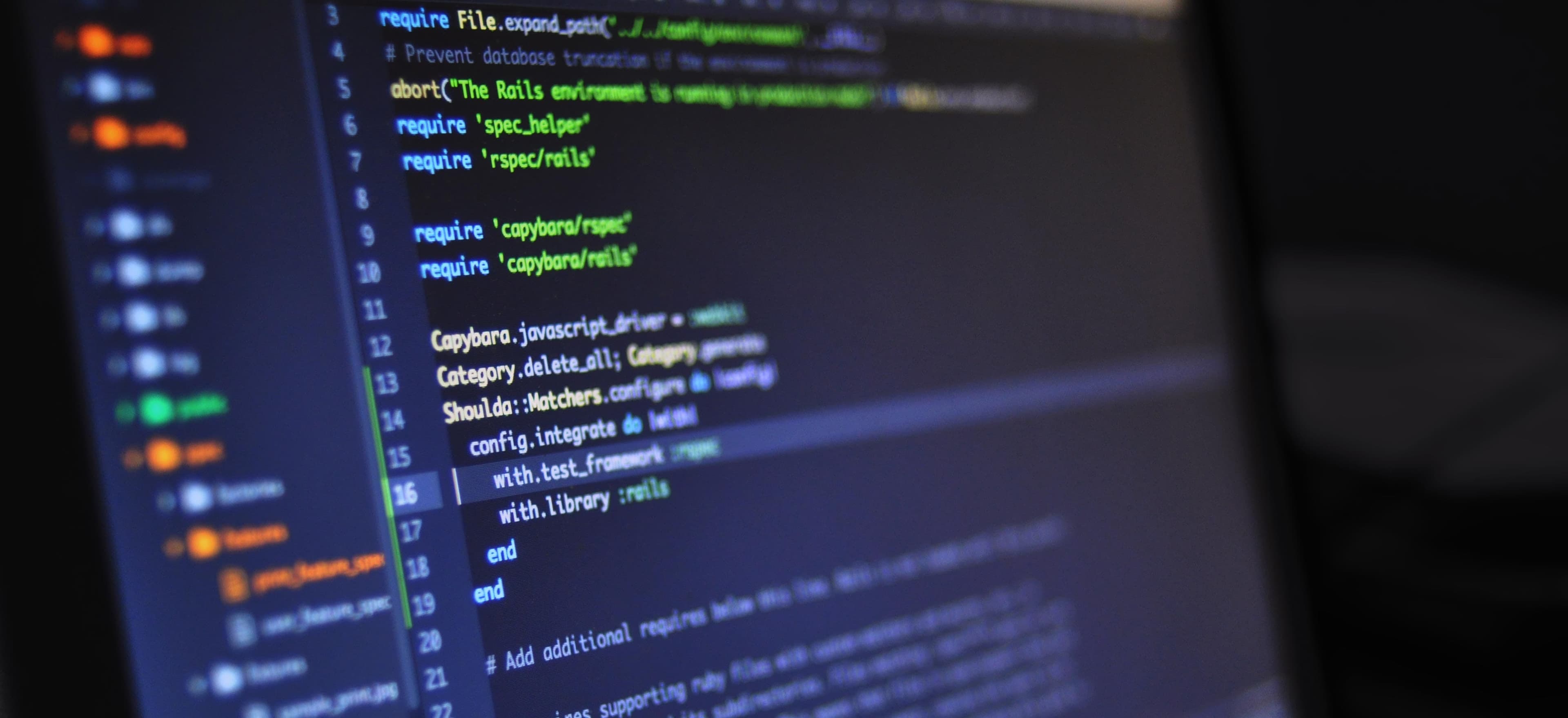
- Published on
Maximizing Performance: Taming Concurrent Queries in Lucene
Apache Lucene is a powerful library for full-text search, providing a range of features that allow developers to build sophisticated search functionalities into their applications. However, with great power comes great responsibility. The performance of search systems can significantly degrade when multiple queries are run concurrently, especially in applications with high user traffic. This article explores how to mitigate performance issues stemming from concurrent queries in Lucene.
Understanding Lucene Querying
Before diving into concurrency, it's essential to understand how querying works in Lucene. Lucene uses an inverted index to provide fast retrieval of documents containing words. When a query is executed, Lucene does the following:
- Parse the query: Turns the query string into an internal representation.
- Query execution: Searches the index and retrieves matches.
- Scoring: Ranks the results based on a scoring algorithm, typically BM25.
Here’s a simple example of executing a query in Lucene:
import org.apache.lucene.analysis.standard.StandardAnalyzer;
import org.apache.lucene.index.DirectoryReader;
import org.apache.lucene.queryparser.classic.QueryParser;
import org.apache.lucene.search.IndexSearcher;
import org.apache.lucene.search.Query;
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.RAMDirectory;
public class SimpleLuceneExample {
public static void main(String[] args) throws Exception {
// Create an index in memory
Directory directory = new RAMDirectory();
StandardAnalyzer analyzer = new StandardAnalyzer();
// Create a QueryParser for parsing the query string
QueryParser parser = new QueryParser("field", analyzer);
// Parse a sample query
Query query = parser.parse("search term");
// Create the IndexSearcher
IndexSearcher searcher = new IndexSearcher(DirectoryReader.open(directory));
// Execute the query
// Note: Handle your results here
}
}
The Performance Challenge
With multiple users performing search queries simultaneously, you may observe increased response times or even time-outs. Concurrent queries can lead to resource contention, particularly around locking mechanisms in the index when writing operations occur.
Optimizing Concurrent Queries
To optimize performance for concurrent queries in Lucene, consider the following strategies:
1. Use Thread Pools
Using thread pools can help manage concurrent query execution limits. Instead of letting every query spawn a new thread, you can reuse threads, thus reducing overhead.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class QueryExecutor {
private final ExecutorService executorService;
public QueryExecutor(int numberOfThreads) {
this.executorService = Executors.newFixedThreadPool(numberOfThreads);
}
public void executeQuery(Runnable queryTask) {
executorService.submit(queryTask);
}
public void shutdown() {
executorService.shutdown();
}
}
Why: This system allows for better resource management, and ensures that only a fixed number of threads are competing for CPU resources at any given time.
2. Caching Results
Caching results can significantly reduce the load on your index. Lucene provides caching mechanisms that allow for stored query results to be quickly retrieved without additional index searching.
import org.apache.lucene.search.Cache;
import org.apache.lucene.search.Filter;
import org.apache.lucene.search.Query;
import org.apache.lucene.search.ScoreDoc;
public class CachingSearch {
private final Cache cache;
public CachingSearch(Cache cache) {
this.cache = cache;
}
public ScoreDoc[] search(Query query) {
// Check if result is cached
ScoreDoc[] cachedResults = cache.get(query);
if (cachedResults != null) {
return cachedResults;
}
// Proceed with search if not cached
// Implement search logic here, store results in cache before return
return new ScoreDoc[]{};
}
}
Why: Caching reduces the required computational power for frequently executed queries, allowing for faster response times.
3. Optimizing Query Structure
How you construct your queries can affect performance. Combining multiple filters into a single query can reduce the need for multiple scans of the index.
import org.apache.lucene.search.BooleanQuery;
import org.apache.lucene.search.Query;
public class OptimizedQuery {
public static Query createBooleanQuery(Query... queries) {
BooleanQuery.Builder builder = new BooleanQuery.Builder();
for (Query q : queries) {
builder.add(q, BooleanClause.Occur.MUST);
}
return builder.build();
}
}
Why: Fewer passes over the index with optimized queries can dramatically increase search time.
4. Asynchronous Query Processing
Introduce asynchronous query processing to allow queries to run without holding up the main thread. This can be accomplished with CompletableFutures in Java.
import java.util.concurrent.CompletableFuture;
public class AsyncQueryProcessor {
public CompletableFuture<ScoreDoc[]> performAsyncSearch(Query query) {
return CompletableFuture.supplyAsync(() -> {
// Perform the search synchronously
return executeSearch(query);
});
}
private ScoreDoc[] executeSearch(Query query) {
// Actual search logic here
return new ScoreDoc[]{};
}
}
Why: This enables the application to maintain responsiveness while long-running queries are executed.
Wrapping Up
Maximizing performance with concurrent queries in Lucene is critical for scalable applications. By employing thread pools, caching results, optimizing your query structure, and introducing asynchronous processing, you can significantly reduce the load on your system while offering users a fast and responsive search experience.
For further reading on best practices and detailed fine-tuning, you can explore the official Lucene 8.0 documentation or check out more on effective search indexing techniques.
By implementing these strategies and keeping an eye on your system’s performance metrics, you’ll ensure that your Lucene-based application can handle high volumes of concurrent queries seamlessly. Happy searching!