Struggling with Batch Request Processing Efficiency?
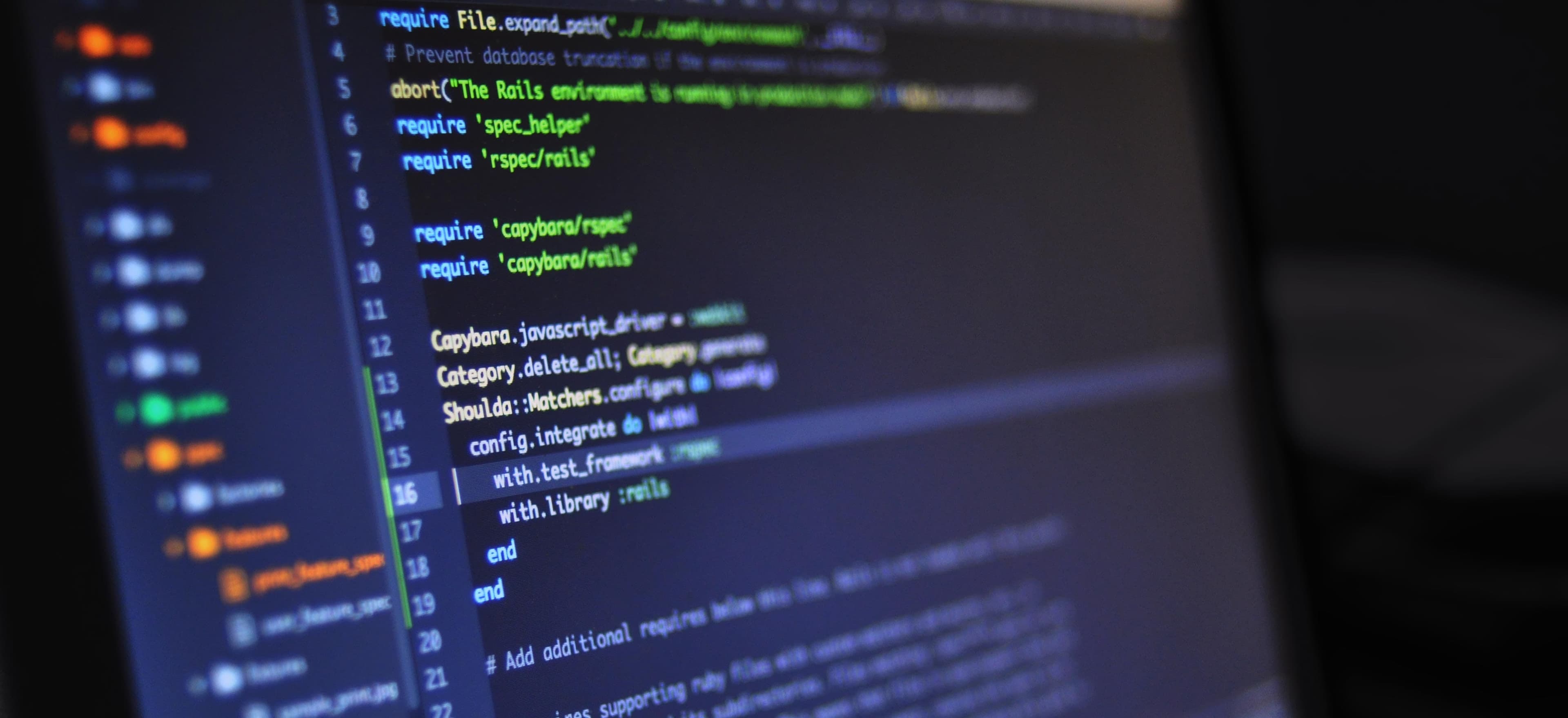
- Published on
Struggling with Batch Request Processing Efficiency?
In today's data-driven world, efficiency in batch request processing is paramount. Whether you're managing user requests in a web application or handling bulk data updates in an enterprise system, optimizing your batch processing can significantly impact your application's performance and user satisfaction. In this blog post, we'll explore strategies for improving batch request processing efficiency using Java, along with code snippets that highlight best practices.
What Is Batch Request Processing?
Batch request processing refers to the handling of multiple requests or transactions in a single batch rather than individually. This approach can reduce the overhead of repeated operations, optimize resource utilization, and improve overall performance.
Why Optimize Batch Processing?
- Performance: Efficient batch processing decreases the time taken to complete multiple requests, enhancing user experiences.
- Resource Utilization: It maximizes CPU and memory utilization, reducing operational costs.
- Scalability: Optimized batches can make your application more scalable, allowing it to handle growing amounts of data and user traffic.
Now, let’s dive into the core elements of creating an efficient batch request processing system.
1. Choosing the Right Data Structure
Using the appropriate data structure can notably enhance the efficiency of batch processing. For instance, ArrayList
is a commonly used structure due to its quick access capabilities. Below, we’ll see how to collect requests using an ArrayList
.
import java.util.ArrayList;
import java.util.List;
public class RequestCollector {
private List<Request> requests;
public RequestCollector() {
requests = new ArrayList<>();
}
public void addRequest(Request request) {
requests.add(request);
}
public List<Request> getRequests() {
return requests;
}
}
Commentary: An ArrayList
is chosen for its efficient random access. Avoid using LinkedList
unless you need to frequently insert or remove elements because it suffers from slower access times.
2. Leveraging Java Streams
Java Streams are a powerful tool for processing collections. When combined with batched processing, they can provide concise and efficient coding. Here’s an example where we filter valid requests and perform an operation.
import java.util.List;
import java.util.stream.Collectors;
public class RequestProcessor {
public List<ProcessedRequest> processRequests(List<Request> requests) {
return requests.stream()
.filter(this::isValid)
.map(this::convertToProcessedRequest)
.collect(Collectors.toList());
}
private boolean isValid(Request request) {
// Validation logic
return request != null; // Example condition
}
private ProcessedRequest convertToProcessedRequest(Request request) {
// Conversion logic
return new ProcessedRequest(request);
}
}
Commentary: Here, we filter valid requests using Streams, which results in cleaner code and potentially better optimizations during execution. The use of functional programming enhances readability.
3. Implementing Multithreading for Concurrent Processing
Sometimes, even with optimal algorithms, processing time can lag. To maximize efficiency, you can implement multithreading:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.List;
public class ConcurrentProcessor {
private final ExecutorService executor = Executors.newFixedThreadPool(10);
public void processInBatches(List<Request> requests, int batchSize) {
for (int i = 0; i < requests.size(); i += batchSize) {
List<Request> batch = requests.subList(i, Math.min(i + batchSize, requests.size()));
executor.submit(() -> processBatch(batch));
}
executor.shutdown();
}
private void processBatch(List<Request> batch) {
for (Request request : batch) {
// Processing logic here
System.out.println("Processing: " + request);
}
}
}
Commentary: This implementation divides requests into smaller batches and processes them in parallel using an ExecutorService. This reduces the time taken for processing overall by utilizing multiple cores of the CPU.
4. Efficient Database Access
One common bottleneck in batch processing is accessing the database. Use batch inserts or updates when interacting with databases:
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class DatabaseBatchProcessor {
public void batchInsert(List<ProcessedRequest> processedRequests, Connection connection) throws SQLException {
String insertSQL = "INSERT INTO requests (data) VALUES (?)";
try (PreparedStatement preparedStatement = connection.prepareStatement(insertSQL)) {
for (ProcessedRequest request : processedRequests) {
preparedStatement.setString(1, request.getData());
preparedStatement.addBatch();
}
preparedStatement.executeBatch(); // Execute the batch
}
}
}
Commentary: Using addBatch()
and executeBatch()
greatly improves database interaction efficiency by reducing the number of individual statements sent to the database.
5. Resource Management
To maintain optimal batch processing, ensure proper resource management. This includes managing memory, threads, and database connections. Use tools like Java Flight Recorder and VisualVM for monitoring resource usage.
Bringing It All Together
Batch request processing can significantly enhance your application's performance, provided you implement the right strategies. From choosing appropriate data structures and leveraging Java Streams to employing multithreading and optimizing database access, the key lies in making informed decisions tailored to your use case.
For additional reading, you may find these resources valuable:
By tackling batch request processing challenges with a multifaceted approach, you can transform your application into a more efficient system capable of handling the demands of modern users. Remember to continually monitor performance and refactor your code as needed to adapt to changing requirements.
Happy coding!
Checkout our other articles