Streamlining User Experience in Android Dashboard Designs
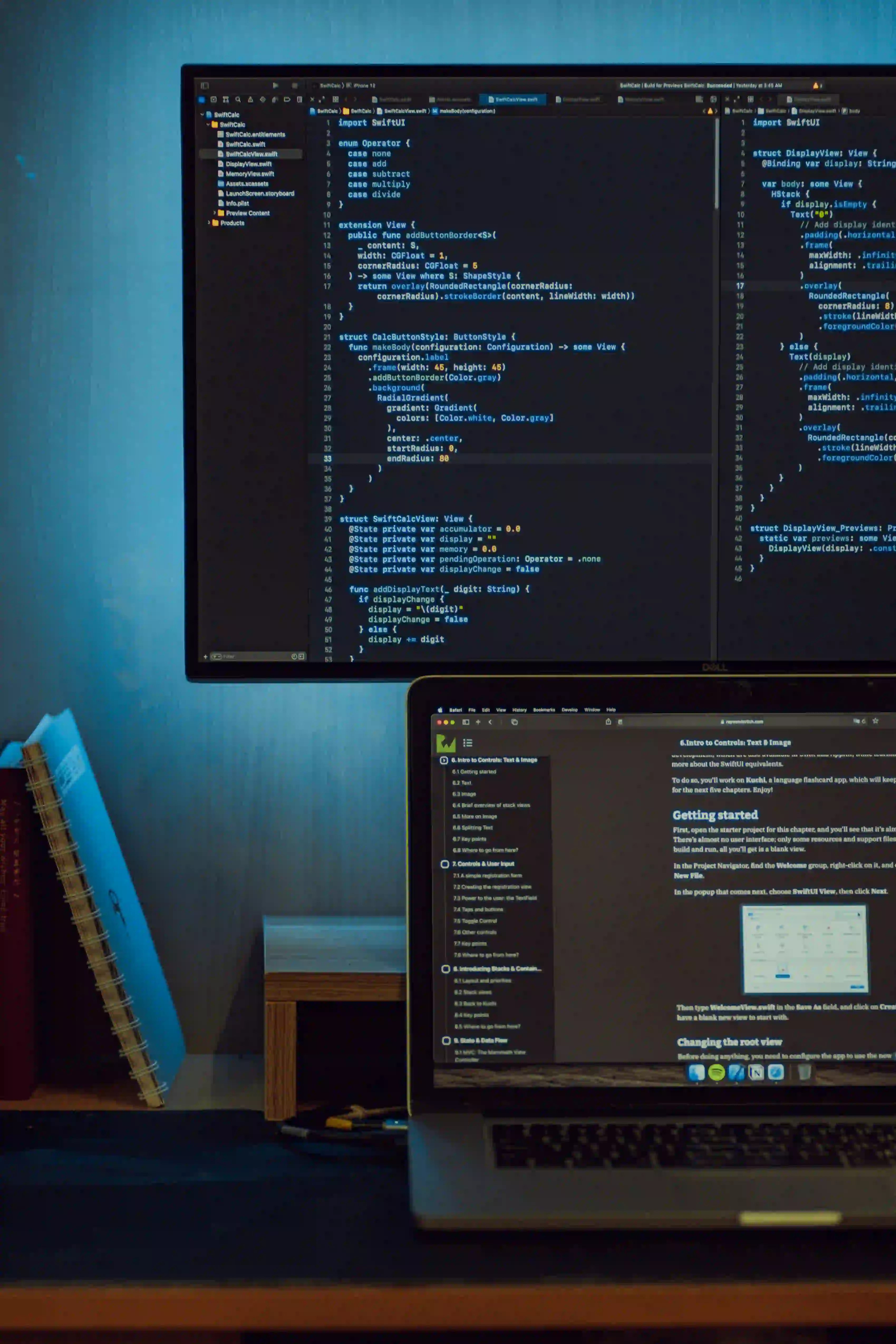
Streamlining User Experience in Android Dashboard Designs
Creating a compelling user experience for Android dashboards is a critical aspect of app development. In today's fast-paced mobile landscape, users expect intuitive interfaces that provide seamless access to data and features. In this blog post, we’ll explore best practices to streamline user experience in Android dashboard designs and discuss some key techniques that developers can apply.
Understanding the User's Needs
Before diving into design practices, it’s essential to empathize with your users. What are their needs? How do they interact with the application? Conducting user research through surveys or interviews can provide invaluable insights. Understanding your audience will inform design decisions and ensure that your dashboard meets their needs.
Key Considerations:
- User behavior: Analyze how users engage with your app.
- User environment: Consider where they will use your app (e.g., on the go, in an office).
- Device compatibility: Ensure that the design is responsive across different devices and screen sizes.
By prioritizing user needs, you set a solid foundation for a compelling dashboard.
Simple and Intuitive Design
Once you have a grasp on user needs, focus on creating a simple and intuitive design. Complexity is the enemy of usability. Here are some strategies:
1. Minimalist Layout
A cluttered dashboard can overwhelm users. Aim for a minimalist layout that presents only crucial information. Google Material Design emphasizes a clean aesthetic with ample whitespace, making it easier for users to focus.
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/dashboard_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="User Dashboard"
android:textSize="24sp"
android:textColor="@android:color/black"/>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="@android:color/darker_gray"/>
</LinearLayout>
In this code snippet, we define a simple layout using a vertical LinearLayout
. The title is clear and prominent, while the separator line establishes visual hierarchy.
2. Consistent Visual Elements
Maintain consistency in your design components, such as buttons, fonts, and icons. This helps build familiarity and makes navigation smoother. Use Material Icons for easily recognizable imagery that users can relate to.
Using the same color palette across your dashboard establishes a cohesive look. For instance, if you opt for a blue theme, keep shades of blue for buttons, highlights, and backgrounds.
3. Clear Navigation
An intuitive navigation structure is critical for any dashboard. Consider:
- Tab layouts for primary sections.
- Navigation drawers for secondary actions.
Keep the labels straightforward to enhance accessibility. An example of implementing tabs in Android would be:
<androidx.tablayout.widget.TabLayout
android:id="@+id/tab_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:tabMode="fixed"
app:tabGravity="fill"/>
<androidx.viewpager.widget.ViewPager
android:id="@+id/viewpager"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
This code sets up a tab layout for easy switching between different sections, facilitating a smoother user experience.
Engaging Visuals and Feedback
Visual elements play a critical role in guiding user attention. Incorporate engaging visuals, but do so judiciously to avoid distraction.
1. Use Data Visualization
For dashboards presenting analytical data, use graphs and charts effectively. Libraries such as MPAndroidChart are excellent for creating interactive charts.
LineChart lineChart = findViewById(R.id.line_chart);
List<Entry> entries = new ArrayList<>();
entries.add(new Entry(0, 1));
entries.add(new Entry(1, 2));
entries.add(new Entry(2, 0));
// Create a dataset and give it a type
LineDataSet dataSet = new LineDataSet(entries, "Label");
// Create a LineData object
LineData lineData = new LineData(dataSet);
lineChart.setData(lineData);
lineChart.invalidate(); // refresh
In this example, we visualize data through a line chart, making it easier for users to interpret trends. Visuals can also reduce the cognitive load on users compared to reading raw figures.
2. Provide Instant Feedback
Instant feedback is vital for user interactions. Whether it’s a button press or completing a task, users should receive immediate visual cues that their input has been acknowledged. For example:
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
v.setBackgroundColor(Color.GREEN); // Change color to indicate action taken
Toast.makeText(getApplicationContext(), "Action Completed", Toast.LENGTH_SHORT).show();
}
});
In this snippet, when a button is clicked, its color changes to green, and a toast message confirms the action. This type of feedback enhances user confidence and engagement with the app.
Optimize for Performance
Performance plays a crucial role in user experience. Slow-loading dashboards can frustrate users.
1. Lazy Loading
For data-heavy dashboards, implement lazy loading. Load only visible portions of data and retrieve additional data on demand. This method conserves resources and enhances performance.
2. Efficient Data Management
Use efficient data structures and algorithms to manage your data. Consider using SQLite databases for storing and retrieving app data efficiently, ensuring your dashboard operates smoothly even with large datasets.
To Wrap Things Up
Crafting a user-friendly Android dashboard requires attention to simplicity, intuitiveness, and performance. Remember:
- Focus on user needs.
- Simplify designs for clarity.
- Create consistent, engaging visuals.
- Optimize performance for a seamless experience.
By adhering to these best practices, you can streamline user experience in your Android app’s dashboard, turning it into a powerful tool that engages and satisfies users. For further reading on improving dashboard designs, check out Material Design Guidelines and Android Developer Documentation.
With the right strategies and user-centric approaches, your Android dashboard can become an essential part of the user’s journey, driving satisfaction and engagement.
By following these techniques and keeping your users at the forefront of the design process, you can create an Android dashboard that not only serves a purpose but also delights users, ensuring they remain engaged and satisfied with your application.