Mastering Java for Seamless Eleventy and Storyblok Connections
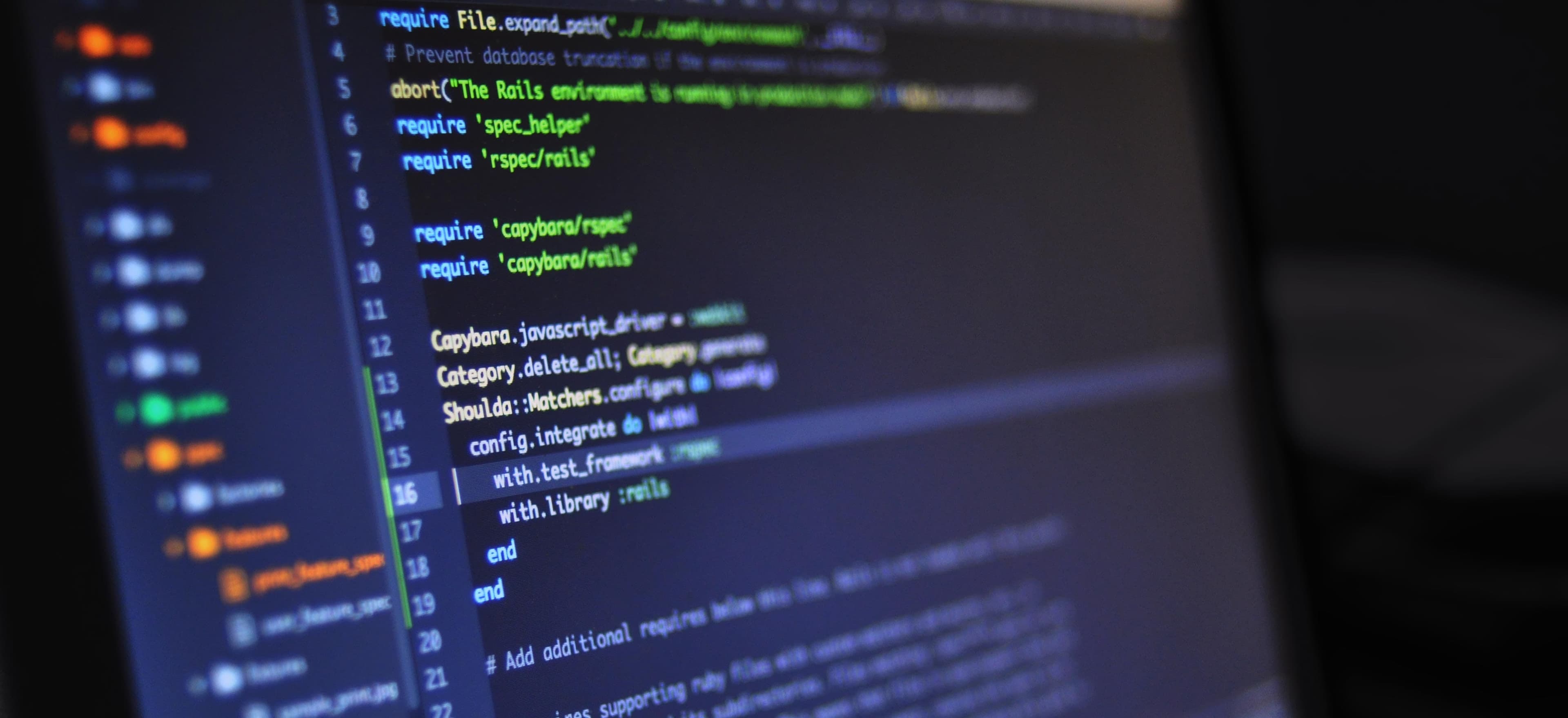
- Published on
Mastering Java for Seamless Eleventy and Storyblok Connections
As web developers continue to adopt modern frameworks and headless CMSs, the need for smooth integrations becomes ever more crucial. Two popular tools in this domain are Eleventy and Storyblok. In our journey today, we will focus on using Java to create seamless connections between these platforms. If you're facing challenges with Eleventy and Storyblok Integration, you can find useful insights in the article Struggling with Eleventy and Storyblok Integration?.
What You Need to Know Before Starting
Understanding Eleventy and Storyblok
- Eleventy (11ty) is a simple static site generator that enables developers to create fast, customizable sites without heavy frameworks.
- Storyblok provides a headless CMS that offers flexibility in how you manage content and integrate it with your projects.
Both platforms are powerful, but their seamless integration often requires some additional coding. Here, we use Java to serve as a bridge or backend solution.
Why Java?
Java is known for its portability, extensive libraries, and strong community support. Its robust ecosystem makes it an excellent choice for backend services that need to interact with APIs like those of Storyblok.
Key Libraries for Integration
To proceed with our integration, understanding a few libraries is important:
- Spring Boot: A powerful framework that simplifies the development of Java applications. It provides an easy way to create RESTful APIs.
- OkHttp: A client for making API calls effectively.
Setting Up Your Java Environment
Before you can start coding, make sure you have the following setup in your development environment:
- Java Development Kit (JDK): Download and install the latest version of the JDK.
- Maven/Gradle: These are build automation tools, and Maven is often used with Spring Boot.
- Integrated Development Environment (IDE): IDEs like IntelliJ IDEA or Eclipse will enhance your coding experience.
Create a Spring Boot Project
Using Spring Boot, you can create a new project quickly. Use the Spring Initializr (https://start.spring.io/) to generate a Maven project.
- Open the Spring Initializr.
- Select project metadata (like Group and Artifact).
- Add dependencies: Spring Web, Spring Boot DevTools.
- Generate the project and unzip it.
Writing Java Code for Storyblok Integration
Now, let's dive into the actual integration. We'll create a basic Spring Boot application that fetches content from Storyblok.
1. Adding Dependencies in pom.xml
Open your pom.xml
file and ensure you have the following dependencies:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.9.1</version>
</dependency>
</dependencies>
This code snippet declares necessary libraries for the web and OkHttp client.
2. Creating a Storyblok Configuration Class
Create a configuration class that handles API interactions with Storyblok. This class will help maintain modularity.
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
import java.io.IOException;
@Component
public class StoryblokService {
private final OkHttpClient client;
@Value("${storyblok.api.key}")
private String apiKey;
public StoryblokService() {
this.client = new OkHttpClient();
}
public String fetchContent(String slug) throws IOException {
String url = String.format("https://api.storyblok.com/v1/cdn/stories/%s?token=%s", slug, apiKey);
Request request = new Request.Builder()
.url(url)
.build();
try (Response response = client.newCall(request).execute()) {
return response.body().string();
}
}
}
Explanation of the Code
In this code snippet:
- OkHttpClient initializes a client to make HTTP requests.
- The
fetchContent
method constructs a URL to fetch a content piece by its slug using the Storyblok API. - @Value is an annotation used to inject the API key from an application properties file.
3. Adding Controller for HTTP Requests
Now, let’s set up a controller that interacts with our StoryblokService
. This will expose an endpoint to the front end.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
@RestController
public class StoryblokController {
@Autowired
private StoryblokService storyblokService;
@GetMapping("/content/{slug}")
public String getContent(@PathVariable String slug) {
try {
return storyblokService.fetchContent(slug);
} catch (IOException e) {
return "Error fetching content: " + e.getMessage();
}
}
}
Analyzing the Controller
What we have here:
- @RestController indicates that this class will handle HTTP requests.
- @GetMapping maps HTTP GET requests to the
/content/{slug}
endpoint. - When a request is made to this endpoint, it invokes the
fetchContent
method ofStoryblokService
.
Running Your Application
- Configure your
application.properties
to include your Storyblok API key:
storyblok.api.key=YOUR_API_KEY
- Run the application: Execute the
main
method in your Spring Boot application class. - Access the endpoint: Visit
http://localhost:8080/content/YOUR_SLUG
in your browser or use a tool like Postman.
Additional Features
The integration doesn't end here. You might consider adding features like:
- Caching responses with Spring Cache.
- Handling authentication if you have protected content.
- Transforming responses for Eleventy's consumption.
Wrapping Up
Integrating Java with Eleventy and Storyblok is a straightforward process when you leverage Spring Boot and OkHttp. As you expand your application's capabilities, keep in mind that proper error handling and security checks will ensure seamless interaction with the Storyblok API.
The knowledge gained from this article should empower you to handle similar integrations in the future. For more insights on Eleventy and Storyblok, be sure to check out the article Struggling with Eleventy and Storyblok Integration?.
Next Steps
- Experiment with the provided code and adapt it to your needs.
- Consider exploring other integration patterns as your application grows.
- Join forums or communities around Eleventy and Java to share knowledge and learn from others.
Happy coding!