Mastering Event Handling in Java: Boost Your UI Efficiency!
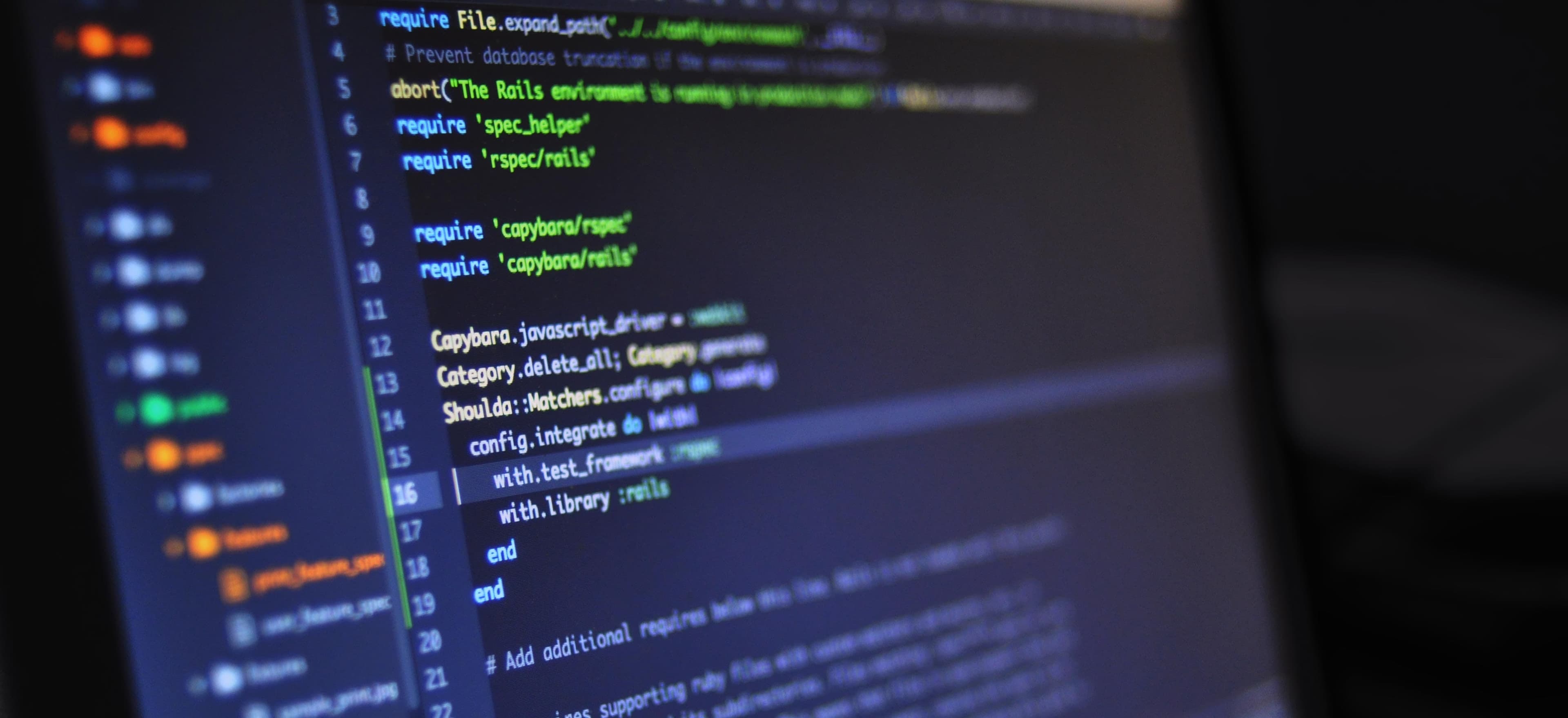
- Published on
Mastering Event Handling in Java: Boost Your UI Efficiency!
In the world of software development, user interactions create the very essence of an application's functionality. For Java developers, effectively managing these interactions through event handling is crucial. This blog post will delve into event handling in Java, explore its importance, and arm you with best practices to optimize your user interface (UI) efficiency.
Understanding Event Handling
At its core, event handling in programming refers to the process of responding to user actions or system occurrences. These may include mouse clicks, key presses, or changes in UI components. In Java, this is primarily managed through the event-driven programming model, where UI components generate events that can be captured and handled.
Why is Event Handling Important?
- User Experience: A smooth, responsive UI enhances user satisfaction.
- Functionality: Proper event handling allows interaction with various components effectively.
- Code Maintainability: A clear event handling structure contributes to better code organization.
Working with Listeners
In Java, event handling is implemented using event listeners. A listener is an interface that receives events and defines how to handle them.
Here’s a basic example showcasing how to handle a button click using an ActionListener:
import javax.swing.*;
import java.awt.event.*;
public class ButtonExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Button Example");
JButton button = new JButton("Click Me");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked!");
}
});
frame.add(button);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Code Explanation
- JFrame: This represents the window.
- JButton: This is the button component.
- ActionListener: It listens for button click events.
In this example, when the button is clicked, a message prints to the console. This basic setup is essential for understanding how Java handles user interactions.
Enhancing Your UI with Advanced Techniques
While the previous example illustrates basic event handling, real-world applications often need more complex interactions. Here, we delve into advanced techniques that can elevate your Java UI experience.
Event Delegation Model
The event delegation model in Java enhances the flexibility of event handling. This model separates event generation from event handling, allowing for more reusable components. Instead of attaching listeners directly to components, you can delegate events to a central controller.
To learn more about the event delegation model, check out the article “Event Delegation Simplified: Why It's a Game-Changer!” available at infinitejs.com/posts/event-delegation-simplified-game-changer.
Using Lambda Expressions
With the introduction of Java 8, lambda expressions can simplify your code and improve readability. Here’s how we can rewrite the previous button click handler:
import javax.swing.*;
public class LambdaExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Lambda Example");
JButton button = new JButton("Click Me");
// Using lambda expression for brevity
button.addActionListener(e -> System.out.println("Button clicked with lambda!"));
frame.add(button);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Why Use Lambda Expressions?
- Conciseness: Reduces boilerplate code.
- Readability: Makes it easier to understand the purpose of the code at first glance.
- Functional Programming Style: Encourages a more functional approach in event handling.
Common Event Types
Java supports various event types relevant to UI applications. Familiarity with these can greatly aid in enhancing application response. Here are some common events:
- Mouse Events: Triggered by mouse actions (clicks, enters, exits).
- Key Events: Occur when keys are pressed or released.
- Focus Events: Pertaining to components gaining or losing focus.
Here’s a quick example handling both mouse and key events:
import javax.swing.*;
import java.awt.event.*;
public class MultiEventExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Multi-Event Example");
JTextArea textArea = new JTextArea();
textArea.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
System.out.println("Mouse clicked at: " + e.getX() + ", " + e.getY());
}
});
textArea.addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
System.out.println("Key pressed: " + e.getKeyChar());
}
});
frame.add(textArea);
frame.setSize(400, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Code Breakdown
- MouseAdapter: A convenience class for mouse event handling, reducing the need to implement all methods of the MouseListener interface.
- KeyAdapter: Similar to MouseAdapter, allowing you to manage key activities more easily.
Best Practices for Event Handling
To enhance UI efficiency further, consider the following best practices:
- Minimize Heavy Calculations: Avoid long-running tasks in event handlers. Use separate threads for intensive calculations to keep the UI responsive.
- Use Event Queues: Leverage Swing’s event dispatch thread (EDT) for thread safety in UI updates.
- Centralize Event Handling: For larger applications, manage events through a centralized controller instead of scattering listeners throughout the codebase.
Example with Threading
Here’s a simple example that handles an event with a separate thread:
import javax.swing.*;
import java.awt.event.*;
public class ThreadingExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Threading Example");
JButton button = new JButton("Start Process");
button.addActionListener(e -> {
new Thread(() -> {
// Simulating a long-running task
try {
Thread.sleep(2000);
} catch (InterruptedException ex) {
ex.printStackTrace();
}
System.out.println("Process Finished!");
}).start();
});
frame.add(button);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Rationale
- Running a lengthy task in a new thread keeps the application responsive. Your UI remains active while processing.
Closing Remarks
Mastering event handling is vital for creating dynamic and responsive user interfaces in Java. By understanding core concepts, leveraging advanced techniques, and adhering to best practices, you can enhance your application's usability.
Whether you're developing a simple application or a more complex system, the principles outlined in this article will serve as a solid foundation for efficiently managing user interactions. For further exploration on delegate methods, you might want to check out the article “Event Delegation Simplified: Why It's a Game-Changer!” at infinitejs.com/posts/event-delegation-simplified-game-changer.
Thanks for reading, and may your Java programming journey be as engaging as the applications you create!
Checkout our other articles