Mastering Java Event Delegation for Cleaner Code Solutions
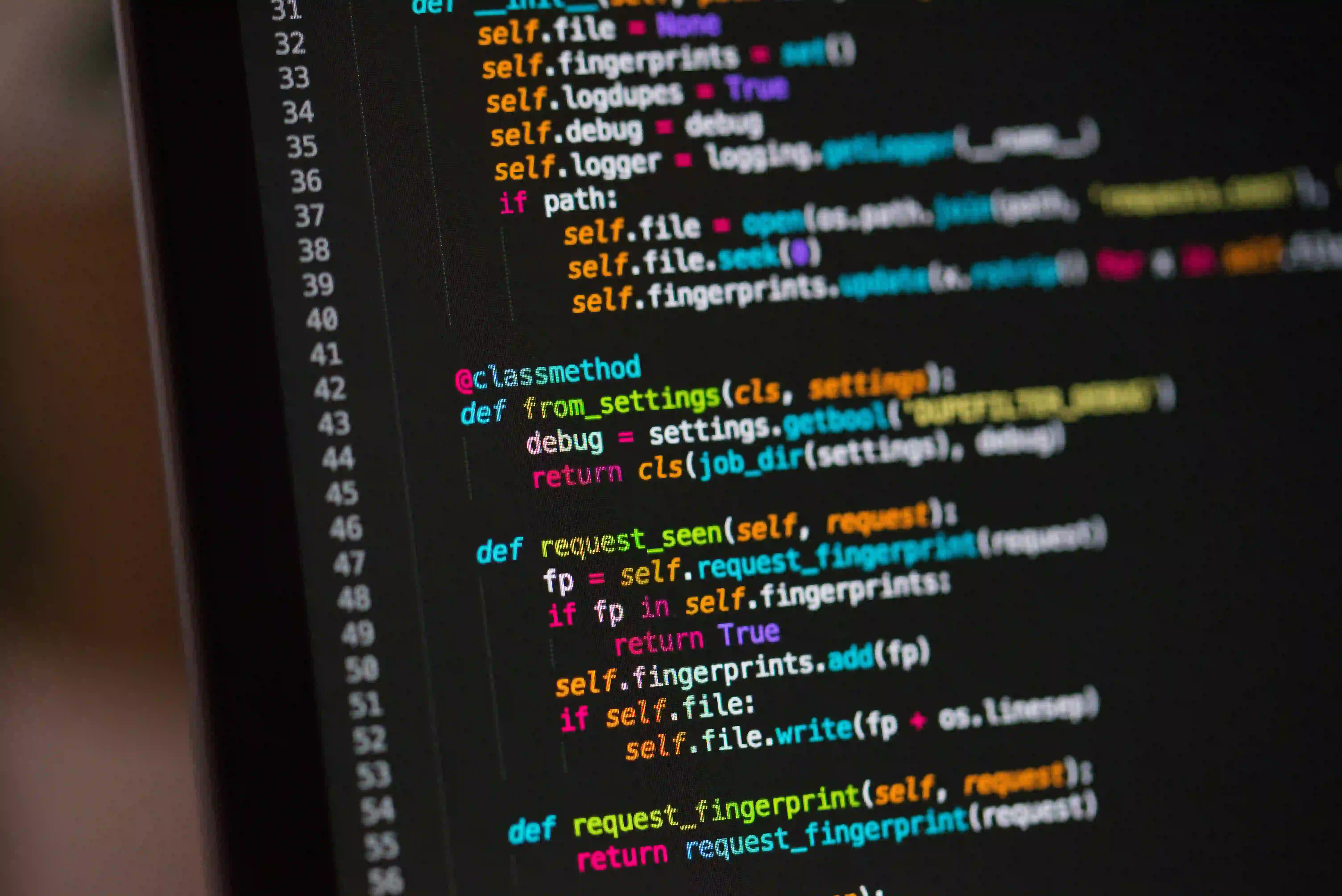
Mastering Java Event Delegation for Cleaner Code Solutions
Java is a versatile programming language that empowers developers to build robust applications. One of the key concepts in Java that significantly enhances code maintainability and performance is Event Delegation. In this blog post, we will explore the essence of event delegation, why it’s crucial for cleaner code, and how to implement it effectively with practical examples.
What is Event Delegation?
Event Delegation is a design pattern that helps in managing events through a centralized mechanism rather than assigning individual listeners to each component. This pattern is particularly useful when dealing with multiple event sources, as it leads to reduced memory usage, cleaner code, and improved performance.
To elaborate, in traditional event handling, each UI component (e.g., buttons, text fields) might have its own event listener. As your application grows, managing these listeners can become cumbersome and error-prone. Event delegation solves this issue by delegating events to a single listener (or a few listeners) higher up in the component hierarchy.
Why Use Event Delegation?
-
Reduced Memory Footprint: Fewer listener instances lead to lower memory consumption. Instead of creating a new listener for every component, one can listen to overall parent events and handle child components therein.
-
Cleaner Code: By centralizing event handling, the code becomes more organized and easier to maintain. This allows developers to separate event logic from UI creation.
-
Improved Performance: Fewer objects mean better performance, especially in complex applications with many components.
-
Easier Maintenance: Modifying event handling logic becomes simpler when managed from a single point.
These benefits can transform the way you manage user interactions in your Java applications. If you're curious about how event delegation is used in JavaScript, consider checking out Event Delegation Simplified: Why It's a Game-Changer!.
Implementing Event Delegation in Java
To illustrate event delegation in Java, we will create a simple GUI application using Swing. Let’s say we have a panel with multiple buttons, and we want to handle their clicks without setting a listener on each button.
Step 1: Setting Up the GUI
First, let’s create a simple frame with a panel and multiple buttons. We will assign a single listener to the panel that will act as the event delegator.
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class EventDelegationExample {
public static void main(String[] args) {
// Create the main frame
JFrame frame = new JFrame("Event Delegation Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
// Create a panel to hold buttons
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(3, 1));
// Add buttons to the panel
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
JButton button3 = new JButton("Button 3");
panel.add(button1);
panel.add(button2);
panel.add(button3);
// Add the panel to the frame
frame.add(panel);
// Set the frame visibility
frame.setVisible(true);
// Add action listener to the panel
panel.addMouseListener(new ButtonClickHandler());
}
}
Step 2: Creating the Event Delegator
Next, we will implement the ButtonClickHandler
. This class will determine which button was clicked by inspecting the event source.
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
class ButtonClickHandler extends MouseAdapter {
@Override
public void mouseClicked(MouseEvent e) {
Object source = e.getSource();
if (source instanceof JButton) {
String buttonLabel = ((JButton) source).getText();
System.out.println(buttonLabel + " clicked!");
}
}
}
Why We Used This Approach
-
Single Listener: By using a single listener for the entire panel, we reduce the number of listener instances. This not only conserves memory but also keeps our code cleaner.
-
Dynamic Handling: The
ButtonClickHandler
checks the source of the event to determine which button was clicked. This dynamic handling is what makes event delegation effective, allowing future changes to button definitions without altering event handling.
Step 3: Running the Application
After implementing the above code, running the application should yield a simple window containing three buttons. Clicking any button will print a message to the console indicating which button was clicked.
Advantages of This Approach
- You can easily add more buttons without changing the core event-handling logic.
- Maintenance becomes straightforward; if changes are needed, they can be executed in a single handler instead of multiple individual ones.
- It allows for cleaner management of UI component interactions.
Going Deeper: Event Delegation and Performance
When building large-scale applications, performance is always a consideration. Event delegation can greatly enhance responsiveness by avoiding unnecessary listener creations and method invocations.
For instance, a scenario with a dynamically generated list of buttons could benefit significantly from delegation because the items are loaded at runtime and may vary:
for (String item : itemList) {
JButton button = new JButton(item);
panel.add(button);
// Normally, you'd add a listener here, but we can skip that!
}
Instead, handling events through a single listener will keep your code vast and flexible.
Bringing It All Together
Mastering event delegation in Java applications fosters cleaner, more maintainable, and performant code. By centralizing the event-handling process, developers not only save resources but also create a more comprehensible structure that is essential for larger projects.
If you're currently using traditional event handling techniques, consider refactoring your approach. Adopting event delegation might be the game-changer your Java applications need.
For further reading into this intriguing design pattern as applied in JavaScript, be sure to check out Event Delegation Simplified: Why It's a Game-Changer!.
Feel free to ask questions or share your own experiences with event delegation in the comments below!