Mastering Event Delegation in Java for Cleaner Code
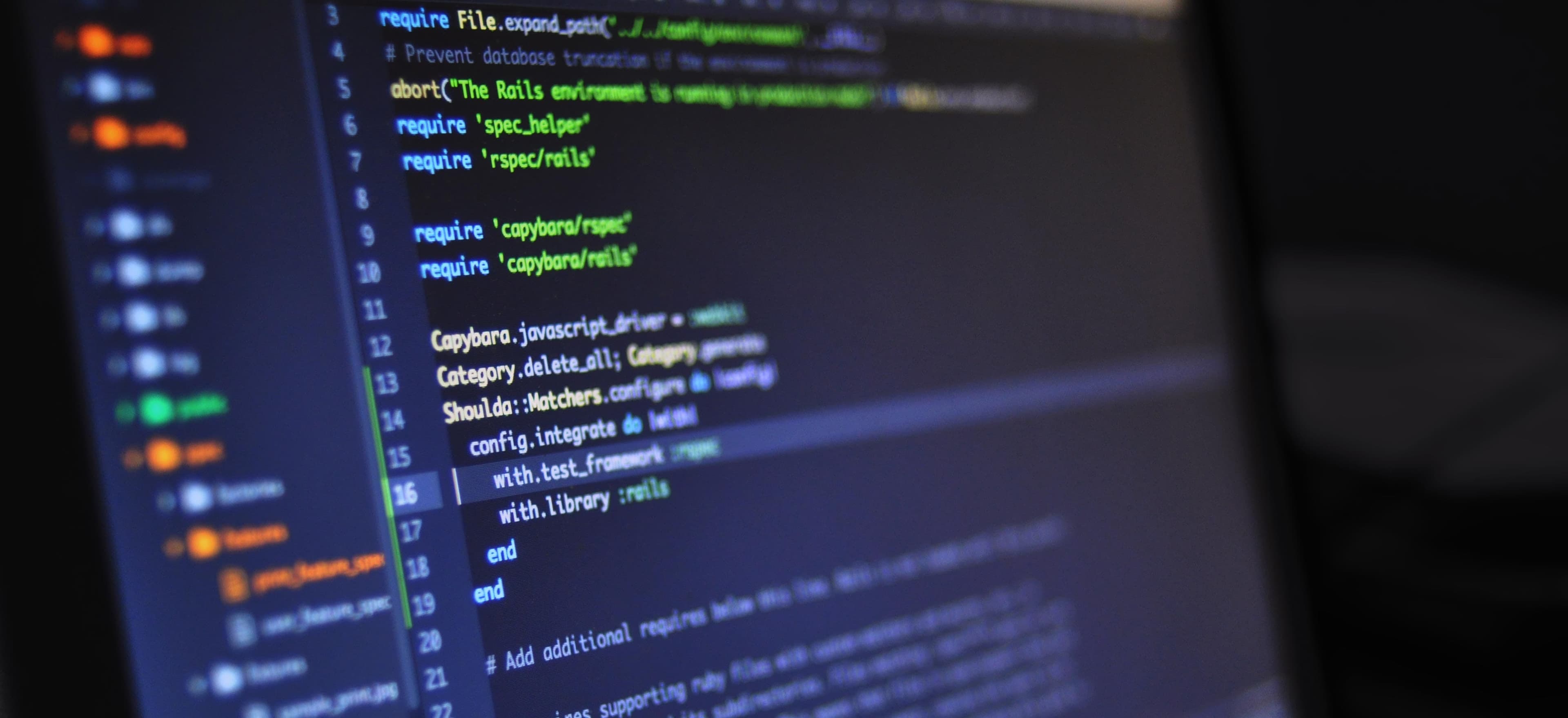
- Published on
Mastering Event Delegation in Java for Cleaner Code
Java is a robust programming language widely recognized for its platform independence and versatility. One of the key aspects of Java programming, especially web applications and software development, is event handling. When building interactive applications, managing events without clutter can be a daunting task. This is where event delegation comes into play.
In this post, we will delve deeply into mastering event delegation in Java, exploring its significance, benefits, and practical applications. We will also discuss why it is deemed a game-changer in handling events efficiently, referring to the excellent article titled "Event Delegation Simplified: Why It's a Game-Changer!" available at infinitejs.com/posts/event-delegation-simplified-game-changer.
Understanding Event Delegation
Event delegation is an event handling pattern where a single event listener is assigned to a parent element instead of individual listeners for every child element. This approach is particularly beneficial in reducing memory usage and enhancing performance. Instead of setting event listeners on multiple child components, you can delegate the event handling to the parent.
The Traditional Approach: On Each Child Component
Here's a typical method of adding event listeners to several buttons:
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TraditionalEventHandling {
public static void main(String[] args) {
JFrame frame = new JFrame("Traditional Approach Example");
JPanel panel = new JPanel();
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
button1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button 1 clicked");
}
});
button2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button 2 clicked");
}
});
panel.add(button1);
panel.add(button2);
frame.add(panel);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
In the above example, individual ActionListener
instances are created for each button. This can quickly become cumbersome, especially when you have many buttons or components.
The Event Delegation Technique
Instead of adding listeners to every individual component, you can define a single listener at the parent level:
Benefits of Using Event Delegation
- Improved Performance: Fewer event listeners mean less overhead.
- Cleaner Code: It streamlines your code, making it easier to read and maintain.
- Dynamic Elements: Adding and removing child components becomes easier since the parent listener remains unchanged.
Implementing Event Delegation
Let's revise the previous example using event delegation:
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class EventDelegationExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Event Delegation Example");
JPanel panel = new JPanel();
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
panel.add(button1);
panel.add(button2);
// Adding a single ActionListener to the panel
panel.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JButton clickedButton = (JButton) e.getSource(); // Identify which button is clicked
if (clickedButton == button1) {
System.out.println("Button 1 clicked");
} else if (clickedButton == button2) {
System.out.println("Button 2 clicked");
}
}
});
frame.add(panel);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Code Breakdown
In this updated code snippet:
- We add an
ActionListener
to the panel instead of individual buttons. This reduces code duplication. - The
getSource()
method determines which component fired the event, allowing for tailored responses based on the button clicked.
Practical Use Cases for Event Delegation
Event delegation shines particularly in applications with dynamic interfaces or lists:
- Dynamic Lists: In Java applications such as Swing, when you have lists of items, employing event delegation allows you to manage clicks on list items seamlessly.
- Form Handling: Dealing with forms where many fields are present can be simplified with event delegation, where only one listener manages all input fields.
- Real-time Applications: In apps requiring real-time updates, like chat or notification systems, having a central event handler is practical.
The Closing Argument
Mastering event delegation not only makes your Java applications cleaner and better organized, but it also enhances performance and code scalability. If you want to dive deeper and understand the fundamental techniques and implications of event delegation further, check out the resource mentioned earlier, "Event Delegation Simplified: Why It's a Game-Changer!" at https://infinitejs.com/posts/event-delegation-simplified-game-changer.
Apply what you've learned here, and you will undoubtedly notice the remarkable difference in your Java code's efficiency and maintainability. Happy coding!
Checkout our other articles