Mastering String Manipulation in Java: Trimming with Delimiters
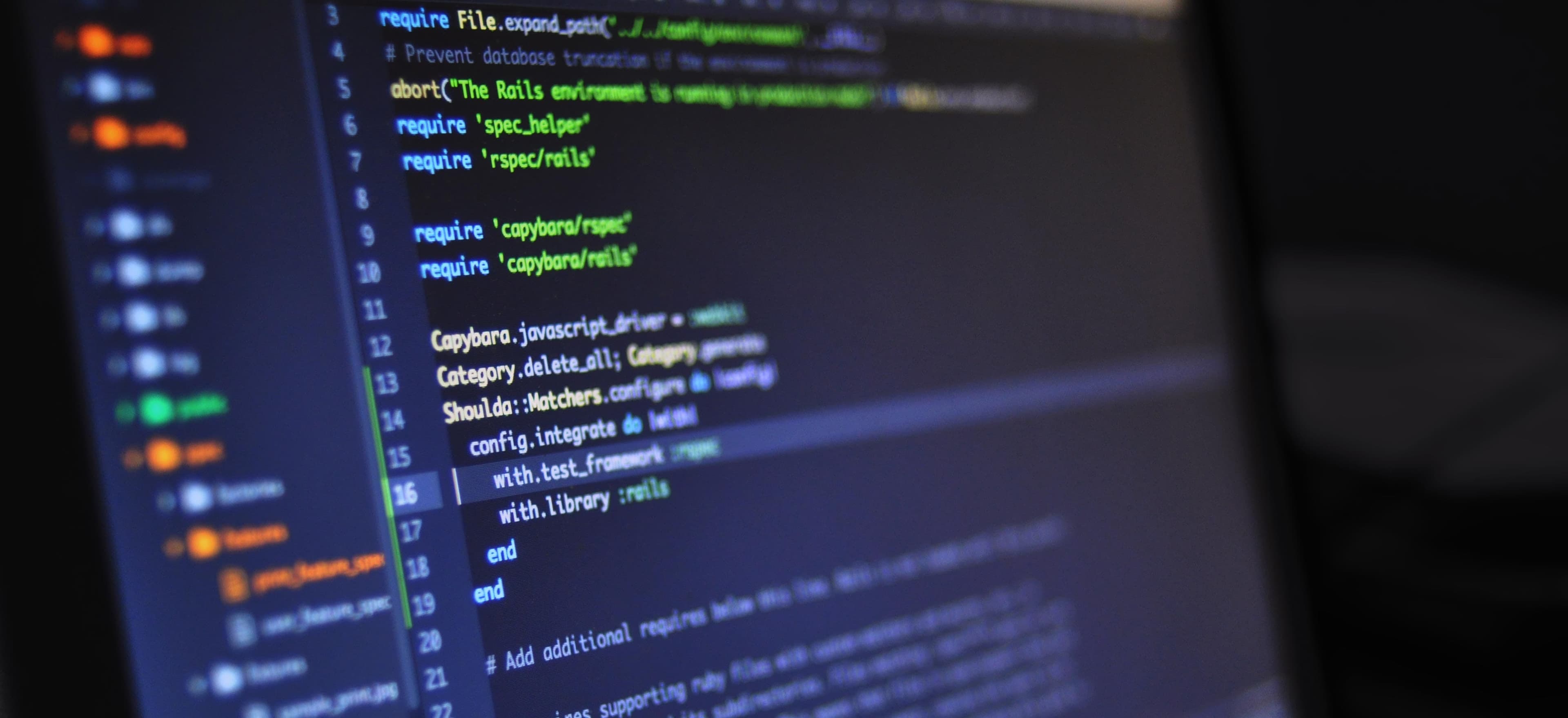
- Published on
Mastering String Manipulation in Java: Trimming with Delimiters
String manipulation is a quintessential aspect of software development, especially in Java. This segment of programming empowers developers to handle, process, and transform textual data effectively. One common string manipulation task you may encounter is trimming strings based on specific delimiters. In this article, we will explore various methods to manipulate strings with delimiters and provide you with code snippets and explanations to enrich your understanding.
Understanding Delimiters
In programming, a delimiter is a character or set of characters that separates tokens within a string. For instance, in the sentence "apple,banana,orange," the comma acts as a delimiter. When manipulating strings, identifying and correctly handling delimiters is essential for accurate data processing.
For practical examples, we'll draw insights from the article "Stripping Numbers Before Delimiters: A How-To Guide" available at tech-snags.com/articles/stripping-numbers-before-delimiters-guide, which discusses effective strategies for handling numbers and delimiters.
Why String Manipulation?
String manipulation allows you to clean, format, and reorganize data, making it a vital skill in many applications. From data parsing to text processing, understanding how to strip, concat, or replace parts of strings can significantly impact your software's performance and usability.
The Basics of String Manipulation in Java
Java provides multiple built-in methods for string manipulation. Some of the commonly used methods include:
length()
: Returns the length of the string.substring(int beginIndex, int endIndex)
: Returns a portion of the string.split(String regex)
: Splits the string based on the given regular expression.trim()
: Trims leading and trailing whitespace from the string.
Let's look at these methods in more depth with examples.
Example 1: Using split()
If your goal is to extract sub-strings from a larger string, the split()
method is your best friend. It allows you to divide a string into an array of substrings based on specified delimiters.
public class SplitExample {
public static void main(String[] args) {
String fruits = "apple,banana,orange";
String[] fruitArray = fruits.split(",");
for (String fruit : fruitArray) {
System.out.println(fruit.trim()); // Output: apple, banana, orange
}
}
}
Why Use split()
?
This method is beneficial because it allows you to easily convert a delimited string into a usable array. This approach simplifies further processing, such as searching or replacing elements in the array.
Example 2: Trimming Whitespace
When dealing with user-input or data from external sources, leading and trailing whitespace can cause issues. The trim()
method can clean up these strings easily.
public class TrimExample {
public static void main(String[] args) {
String text = " Hello World! ";
String trimmedText = text.trim();
System.out.println("|" + trimmedText + "|"); // Output: |Hello World!|
}
}
Why Use trim()
?
Trimming whitespace is a best practice in data handling as it can prevent bugs that stem from unintentional spaces in strings, especially when comparing or storing data.
Advanced String Manipulation: Stripping Numbers Before Delimiters
One common task you might encounter is stripping numbers that appear before delimiters in a string. For instance, given the string "12:apple 34:banana 56:orange", you may want to obtain only the names of the fruits.
This can be elegantly done using regular expressions. Following are the steps and examples.
public class StripNumbersExample {
public static void main(String[] args) {
String input = "12:apple 34:banana 56:orange";
String[] items = input.split(" ");
for (String item : items) {
String strippedItem = item.replaceAll("^\\d+:","");
System.out.println(strippedItem); // Output: apple, banana, orange
}
}
}
Why Use Regular Expressions?
Regular expressions (regex) offer a powerful way to process strings. The pattern ^\\d+:
matches any digits at the beginning of a string followed by a colon. This allows for efficient stripping of numbers, providing a streamlined way to clean data.
For more detailed instructions and examples on stripping numbers before delimiters, consider checking out the previously mentioned article here.
Comprehensive Example: Combining Methods
Often, you will need a combination of string methods to achieve your desired format. Below is an example that combines splitting and removing unwanted characters.
public class ComprehensiveExample {
public static void main(String[] args) {
String input = " 1:apple,2:banana,3:orange ";
String[] items = input.trim().split(",");
for (String item : items) {
String fruit = item.replaceAll("^\\d+:","").trim();
System.out.println("Fruit: " + fruit);
}
}
}
Why This Approach?
This example illustrates how you can ensure the string is appropriately cleaned up at every stage of processing. Using trim()
, split()
, and replaceAll()
together ensures that you receive sanitized output without unwanted characters or spaces.
Final Thoughts
String manipulation is an invaluable skill for any Java developer. By mastering methods like split()
, trim()
, and regular expressions, you can handle complex string processing tasks with ease. Whether you're cleaning data, parsing user inputs, or formatting output, these techniques will serve you well.
Continue exploring string manipulation in Java by practicing various scenarios and using external resources for deeper insights. For instance, refer back to the article "Stripping Numbers Before Delimiters: A How-To Guide" at tech-snags.com/articles/stripping-numbers-before-delimiters-guide for a focused dive into this essential string handling procedure.
Happy coding!
Checkout our other articles