Removing Unwanted Characters: A Java Approach to Clean Data
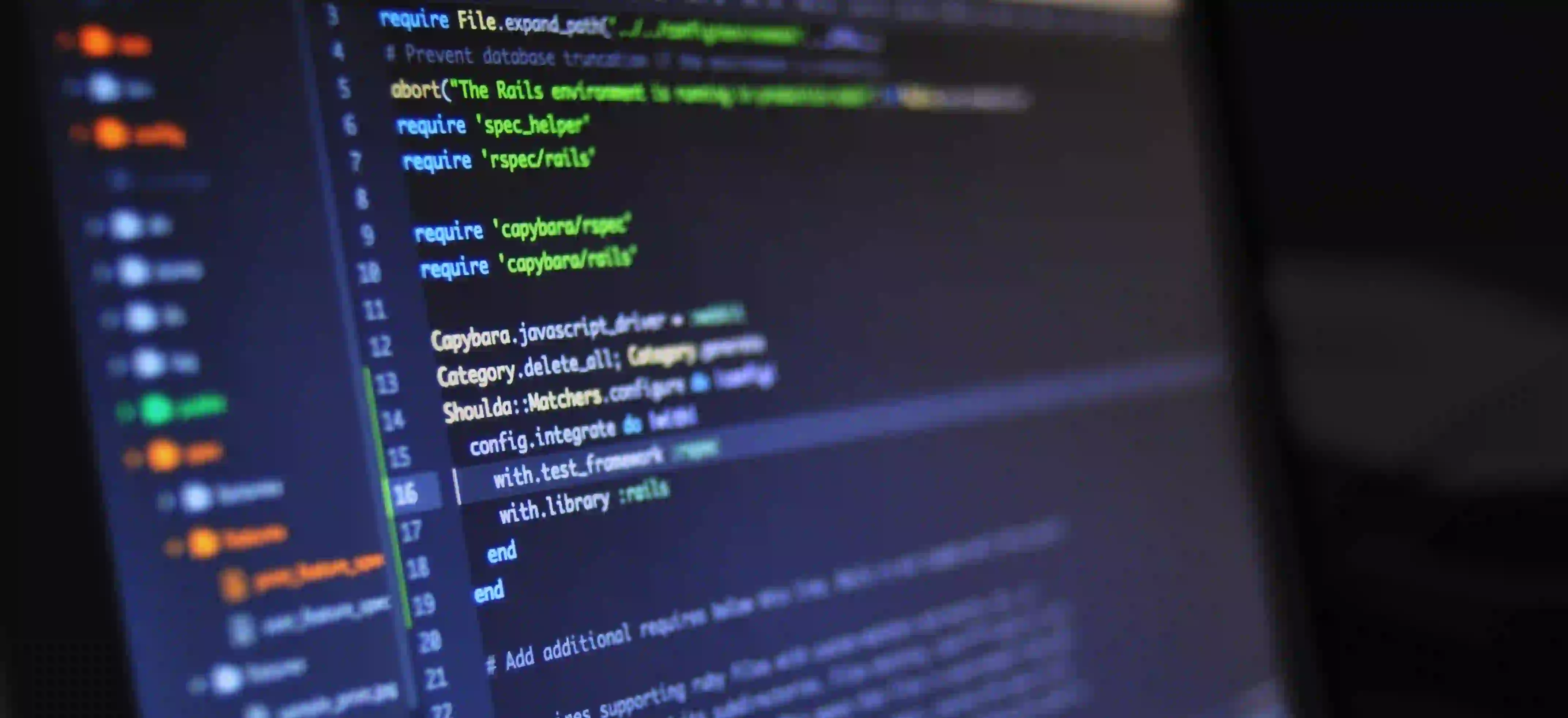
Removing Unwanted Characters: A Java Approach to Clean Data
In the era of big data, one of the most important tasks data engineers and developers face is data cleaning. Specifically, removing unwanted characters from strings can significantly enhance data quality. In this blog post, we will explore various methods in Java to effectively handle this cleaning process. Along the way, we will touch on regex, string manipulation, and practical use cases.
Understanding Unwanted Characters
Unwanted characters can range from whitespace to specific symbols or numbers that don't add any value to the data set. For instance, consider a string from a user form that includes numbers when only letters are expected. Unwanted characters can lead to inaccuracies in data analysis, so learning how to strip them off is crucial.
The Basics of String Manipulation in Java
Java offers multiple ways to manipulate strings. Our focus will be on utilizing simple string methods and regular expressions (regex).
Basic String Manipulation
For a simple implementation, we can use the following Java code to trim whitespace and remove unwanted characters:
public class StringCleaner {
public static String cleanString(String input) {
// Trim whitespace from both ends of the string
String trimmedString = input.trim();
// Remove unwanted characters (e.g., numbers and punctuation)
return trimmedString.replaceAll("[^a-zA-Z]", "");
}
public static void main(String[] args) {
String dirtyString = " Hello,123 World! ";
String cleanString = cleanString(dirtyString);
System.out.println("Cleaned String: " + cleanString); // Output: HelloWorld
}
}
Code Breakdown
- Trimming Spaces: The
trim()
method removes leading and trailing whitespace, which is often present in user input. - Regex Replacement: The
replaceAll("[^a-zA-Z]", "")
method uses regex to eliminate unwanted characters. The pattern[^a-zA-Z]
matches anything that is not an uppercase or lowercase letter.
Why: This method is straightforward for cases where you want to keep only letters. It uses a regex that can easily be modified to meet different needs, such as retaining numbers or symbols.
Advanced String Cleaning Using Regex
While basic cleaning is often sufficient, there are more complex cases where unwanted characters can follow specific patterns.
For instance, if you need to keep certain symbols but remove others, regex becomes incredibly useful. A practical scenario could involve cleaning addresses where spaces and punctuation might be significant:
public class AdvancedStringCleaner {
public static String cleanAddress(String address) {
// Only keep letters, digits, spaces, and certain punctuation
return address.replaceAll("[^a-zA-Z0-9.\\s,-]", "");
}
public static void main(String[] args) {
String dirtyAddress = "123 Main St! Apt# 4B, Somewhere, NY @ 10001";
String cleanAddress = cleanAddress(dirtyAddress);
System.out.println("Cleaned Address: " + cleanAddress); // Output: 123 Main St. Apt 4B, Somewhere, NY 10001
}
}
Code Explanation
- Keeping Specific Characters: The regex pattern
[^a-zA-Z0-9.\\s,-]
allows letters, numbers, spaces, periods, commas, and hyphens to remain in the string. - Flexibility: This demonstrates how regex can be adjusted based on the context of what you consider "unwanted."
Why: This level of specificity is crucial when dealing with address information, where removing too much could alter meaning and clarity.
Integrating Data Cleaning in Your Workflow
Data cleaning is seldom a standalone process. It usually forms a critical step in broader data manipulation tasks. For example, you may encounter cases similar to those discussed in Stripping Numbers Before Delimiters: A How-To Guide. In that scenario, you might not only need to remove unwanted characters but also strip numbers that precede specific delimiters in your data.
Implementing Comprehensive Data Cleaning
You can combine these techniques by chaining multiple string cleaning operations together. Suppose you want to clean a string by both removing unwanted characters and stripping numbers:
public class ComprehensiveCleaner {
public static String cleanData(String input) {
// First, clean the string to remove unwanted characters
String cleanedString = cleanString(input);
// Strip numbers before specific delimiters (like spaces)
cleanedString = cleanedString.replaceAll("\\d+\\s*", "");
return cleanedString.trim();
}
public static void main(String[] args) {
String dirtyData = "123 ABC 456 DEF; GHI 789";
String cleanData = cleanData(dirtyData);
System.out.println("Final Cleaned Data: " + cleanData); // Output: ABC DEF; GHI
}
}
Code Workflow
- Sequential Processing: The
cleanData
method first callscleanString
to strip unwanted characters, followed by removing numbers. - Chained Operation: This chaining allows for handling complex data in one method call.
Why a Comprehensive Approach Matters
Data cleaning is seldom linear. Structured data might require multiple cleaning strategies working together. Using the approach outlined in this section, you can robustly handle diverse data quality challenges.
In Conclusion, Here is What Matters
Cleaning unwanted characters is a vital aspect of preparing data for analysis and ensuring its overall quality. By creatively using Java's string manipulation capabilities and regex, developers can build effective tools to clean their datasets.
For deeper insights on managing numbers before delimiters, I recommend referencing the article "Stripping Numbers Before Delimiters: A How-To Guide." As we continue to grapple with the complexities of data, mastering these techniques will undoubtedly enhance your edge in the tech field.
By adopting these strategies in your Java applications, you not only improve data integrity but also ease subsequent data handling tasks. Remember, clean data leads to accurate insights!